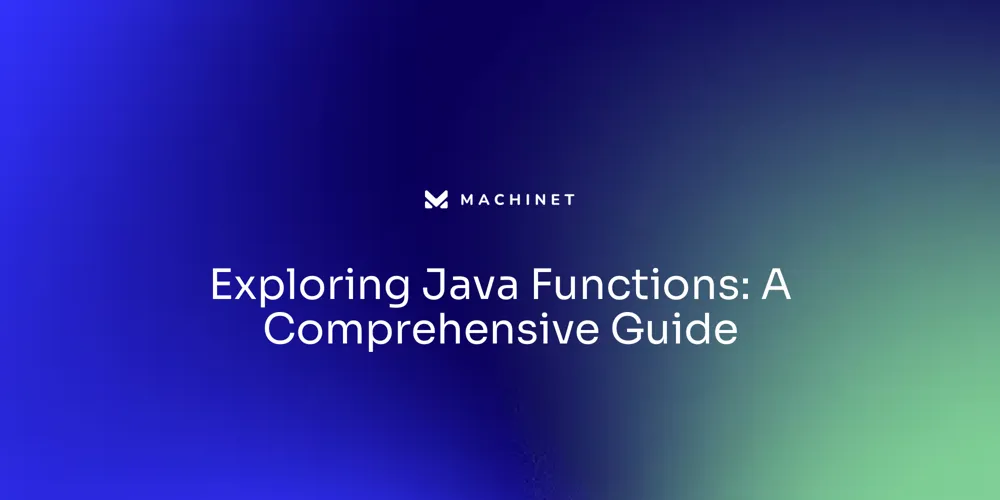
Table of Contents
- Declaring and Calling Functions
- Understanding Method Overloading
- Best Practices for Writing Functions
Introduction
In Java, methods play a crucial role in performing specific tasks and enhancing code reusability. They are declared using a specific syntax that outlines their accessibility, return type, name, and parameters.
Understanding functional programming aspects like functional interfaces and lambda expressions is vital for modern development practices in Java. Method overloading, a powerful feature of Java, allows developers to define multiple methods with the same name but different parameter lists, providing flexibility in how the methods are utilized.
However, it is important to be aware of potential complexities and embrace clean code practices when implementing method overloading. Writing functions using best practices, such as selecting precise and descriptive names, ensuring modularity, and documenting thoroughly, is essential for creating maintainable Java code. By adhering to these practices, developers can construct code that is not only efficient but also a joy to read and maintain.
Declaring and Calling Functions
In Java, methods are essential constructs that enable you to perform specific tasks and enhance code reusability. To declare a method, you'll utilize a specific syntax that outlines its accessibility, return type, name, and the parameters it takes.
An access specifier, such as 'protected', 'private', or 'default', dictates who can invoke the method, ensuring encapsulation and proper usage within an inheritance hierarchy or a package. The return type signifies what the method will return after execution; if no value is to be returned, 'void' is used.
For instance, to create a method that concatenates two strings with a space, you would declare it with the appropriate access level and specify 'String' as its return type. The method would take two 'String' parameters.
Once declared, you call the method using its name and pass the two strings as arguments. Java's functional programming aspects, like functional interfaces and lambda expressions, provide powerful tools for developers. Interfaces such as 'Consumer' and 'Function' allow for passing behavior and chaining functions, respectively. With the prevalence of Java in the industry, evidenced by its consistent top-three ranking and 603K job offers accounting for 20% of programming language-specific roles, understanding these concepts is vital for modern development practices. The recent releases and updates in the Java ecosystem, such as Spring's various versions, further underscore the language's ongoing evolution and the importance of staying current with its features.
Understanding Method Overloading
Method overloading in Java is a powerful feature that exemplifies the concept of polymorphism, allowing developers to define multiple methods within the same class that share the same name but differ in parameter lists. This enables a method to perform various functions, depending on the number of parameters passed to it.
For instance, a method called \'calculateSum()\' could be overloaded to add two integers as well as three integers, providing flexibility in how the method is utilized. As developers harness the versatility of method overloading, they must also be vigilant of the potential complexities it introduces, such as the unpredictable occurrence of deadlocks, particularly when working with newer Java features like virtual threads.
Careful debugging and understanding of underlying libraries are crucial to ensure that the convenience of method overloading does not compromise the robustness of the application. Embracing clean code practices is essential when implementing method overloading.
This involves using descriptive method names and avoiding ambiguous or generic names that can lead to confusion. For example, adopting a clear naming strategy like \'addTwoNumbers()\' and \'addThreeNumbers()\' for overloaded methods can significantly enhance the readability and maintainability of the code. The continuous evolution of Java, marked by the release of libraries such as JobRunr 6.3.4 and EclipseStore 1.0.0, reflects the language\'s adaptability and the Java community\'s commitment to innovation. These updates offer developers new tools and improved features, reinforcing Java\'s position as a vibrant and dynamic programming environment. Method overloading remains a testament to Java\'s enduring design, offering developers the flexibility to write expressive and efficient code.
Best Practices for Writing Functions
Creating clean, maintainable Java code is a craft that hinges on the adept use of functions. By adhering to best practices in function design, you can construct code that is not only efficiently expressive but also a breeze to work with for yourself and your peers. Selecting precise and descriptive names for functions, ensuring they remain succinct and sharply focused on a single task, and documenting them thoroughly are cornerstones of this approach.
A prime example is the abstraction of repetitive operations into helper functions, such as calculating the total price in a shopping cart application, which prevents code duplication and fosters consistency. Mastering the art of function calling is also crucial, as it allows for modular design and the ability to 'call out' to external functions for complex tasks. This modular approach aligns with community-accepted coding standards, which advocate for consistency in naming, spacing, and structure.
As underscored by statistics, clean code is paramount as it is read more often than written, setting the stage for accelerated development and debugging while enhancing maintainability. The sentiment that code serves as a 'love letter' to the next developer is a testament to the importance of writing code with clarity and consideration. With Java's continued prominence and the evolving trends of 2023, it's more important than ever to embrace practices that yield code which is not just functional but also a joy to read and maintain, ensuring it stands the test of time in the dynamic landscape of software development.
Conclusion
In conclusion, methods in Java are crucial for performing specific tasks and enhancing code reusability. By understanding the syntax for declaring methods and the importance of access specifiers and return types, developers can create efficient and encapsulated code.
Method overloading, a powerful feature in Java, allows for the definition of multiple methods with the same name but different parameter lists. While method overloading provides flexibility, it is important to be aware of potential complexities and embrace clean code practices.
This includes using descriptive names and avoiding ambiguity to improve code readability and maintainability. Writing functions using best practices is essential for creating clean and maintainable Java code.
Precise and descriptive names, modularity through helper functions, and thorough documentation contribute to efficient and expressive code. Adhering to community-accepted coding standards ensures consistency and enhances the readability of the code. In the ever-evolving landscape of software development, it is crucial to write code that not only functions correctly but also brings joy to read and maintain. By following these best practices, developers can create code that stands the test of time in the dynamic world of Java programming.
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.