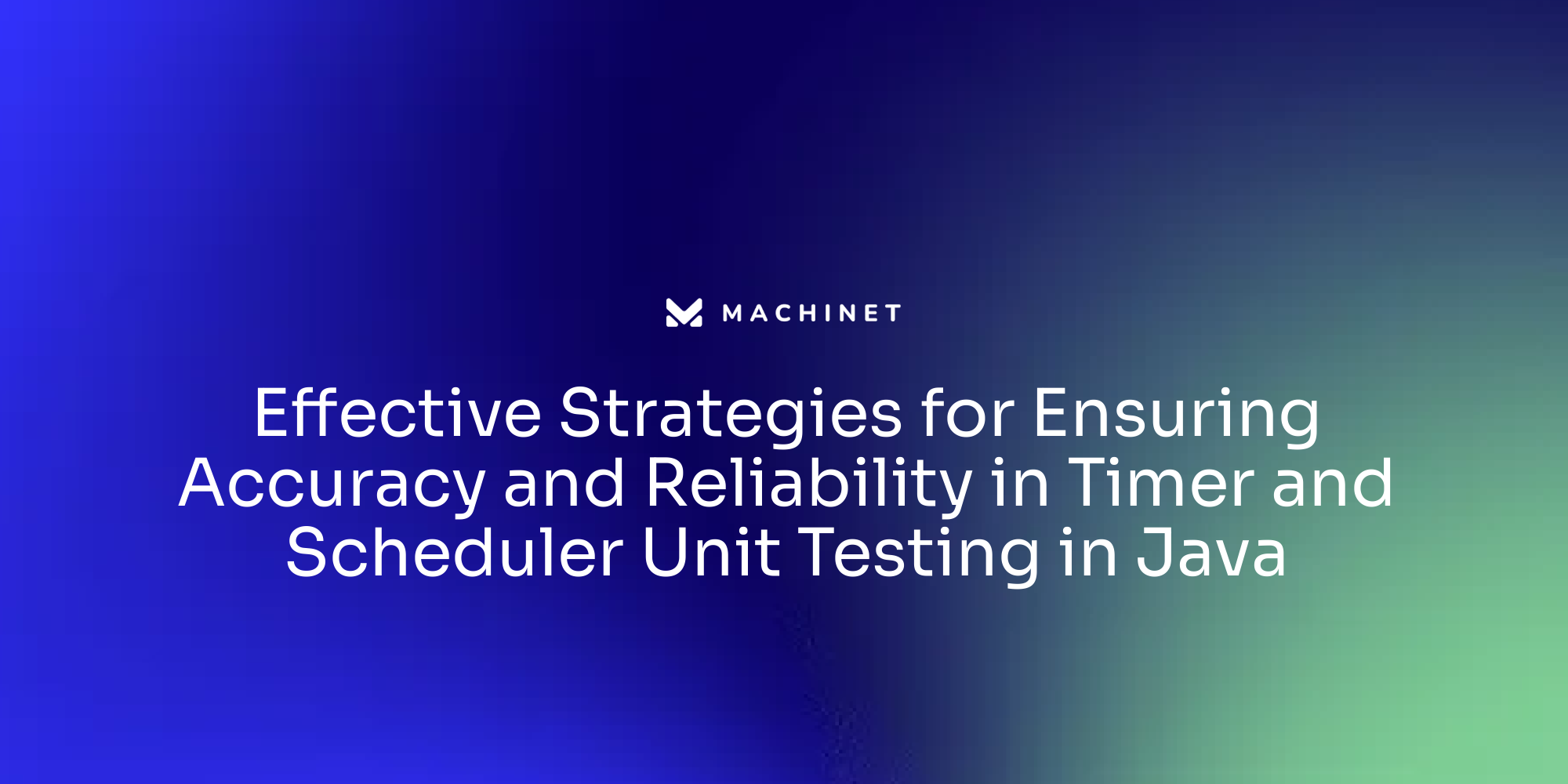
Table of contents
- Understanding the Importance of Accurate Timer and Scheduler Unit Testing in Java
- Common Challenges in Timer and Scheduler Unit Testing
- Strategies for Ensuring Accuracy in Timer Based Unit Tests
- Techniques for Reliable Scheduler Unit Testing
- Implementing Robust and Flexible Testing Frameworks for Timer and Scheduler Tests
- Managing Workload and Balancing Deadlines in Timer and Scheduler Unit Testing
- Case Study: Successful Implementation of Timer and Scheduler Unit Testing
Introduction
The importance of accurate timer and scheduler unit testing in Java cannot be overstated. These tests play a vital role in validating the performance of time-dependent code and identifying potential issues such as race conditions, timezone inconsistencies, and unexpected delays. However, these tests can be challenging to implement due to factors like flakiness caused by improper timeout utilization and the unpredictability of test environments.
In this article, we will explore the significance of precise timer and scheduler unit testing in Java and the challenges faced in achieving accuracy. We will discuss strategies for managing flakiness, aligning the test environment with API needs, and differentiating between performance and functional tests. Additionally, we will provide examples of timer and scheduler unit tests in Java and techniques for reliable testing. By understanding the importance of accurate timer and scheduler unit testing and implementing effective strategies, developers can enhance the reliability and robustness of their software applications
1. Understanding the Importance of Accurate Timer and Scheduler Unit Testing in Java
In the software development lifecycle, the significance of unit testing in Java, particularly when it comes to timers and schedulers, is paramount. These tests act as performance validators for time-dependent code under a myriad of scenarios, aiding in the early detection and resolution of potential issues such as race conditions, timezone inconsistencies, or unexpected delays that may surface in a live environment.
Nonetheless, these tests can oftentimes be "flaky", yielding varying results even when executed in seemingly identical environments. This unpredictability can be attributed to a multitude of factors including network connectivity, system load, library dependencies, and even the type of device on which the test is run. A key source of this flakiness is the improper utilization of timeouts, which are commonly used in tests to ensure tasks are completed within a predefined timeframe.
To illustrate, a test might pass in an environment with network connectivity but fail in its absence. Similarly, a test might pass under normal system load but fail when the load is increased. Other instances include discrepancies arising from dependencies on different libraries and difference between emulators and physical devices. These inconsistencies emerge when the assumptions made in the test are incorrect or when the test environment is subjected to higher load than anticipated.
Addressing these issues necessitates aligning the test environment with the needs and expectations of the APIs used. While more lenient timeouts can be employed if guarantees cannot be established, this is suboptimal. Waiting indefinitely, while sidestepping any assumptions about the operation's execution time, can result in prolonged executions of flawed tests. This calls for a test environment-wide limit on the time allocated for any test execution.
In single-threaded concurrency, deadlocks - a state where a process is unable to proceed due to other processes holding the resources it requires - can occur when indefinite waiting is used. To circumvent this, finite waiting and infinite retries can be combined while yielding control.
It is also crucial to differentiate between performance tests and functional tests. The former should be performed in a representative execution environment, while the latter can be converted by altering timing constraints to completion or order constraints on operations.
Testing for the absence of events should be conducted using proxy events and infinite polling, instead of timed waiting. Misuse of timeouts, stemming from unfulfilled expectations or a non-representative test environment, can lead to flaky tests.
In conclusion, precise timer and scheduler unit testing in Java can enhance the reliability and robustness of software applications, fortifying them against potential issues that may arise in production. Although this process is challenging, it is an integral part of the software development lifecycle and should be approached with the appropriate tools and strategies to ensure success.
To provide examples of timer and scheduler unit tests in Java, consider the following approaches:
-
Timer-based tests: Create a test case where the Timer class from the java.util package is used to schedule and execute a task after a certain delay or at regular intervals. In the test case, verify that the task is executed correctly and that the timing is as expected.
-
Scheduler-based tests: When using a scheduling framework like Quartz, create test cases to verify the scheduling behavior. For example, schedule a job to run at a specific time and then check if the job is executed as expected. Also, test scenarios like rescheduling, pausing, and resuming jobs.
Remember to use appropriate assertions and mock objects when necessary to isolate the behavior being tested and ensure reliable and repeatable tests. To simulate time in Java unit tests, libraries such as Mockito can be used. Mockito provides a way to mock the behavior of objects, including the behavior of time-related classes and methods. By using Mockito's mocking capabilities, you can control the time values returned by methods like System.currentTimeMillis()
or new Date()
. This enables you to simulate different time scenarios in your unit tests, simplifying the testing of time-dependent functionality in your Java code
2. Common Challenges in Timer and Scheduler Unit Testing
Unit testing timers and schedulers in Java is a multifaceted endeavor. It encompasses handling the nuances of multi-threading, managing time zone differences, dealing with real-time clocks, and testing time-dependent code. These complexities can make the process daunting, but there are strategies and best practices that can facilitate the process and ensure reliable and accurate results.
A key practice is isolating the timer and scheduler code to simplify testing. This can be achieved by employing interfaces or using mocking frameworks like Mockito. Also, utilizing a virtual clock instead of the system clock can make your tests more predictable and repeatable. This allows you to control the passage of time during testing, which is particularly useful when dealing with real-time clocks that can introduce an element of unpredictability.
java
Clock clock = Clock.fixed(Instant.parse("2022-01-01T10:00:00Z"), ZoneId.of("UTC"));
Furthermore, it's important to test various scenarios, such as tasks executing correctly, tasks not executing due to incorrect scheduling, and tasks being rescheduled correctly. If your timer or scheduler relies on external dependencies, mock these dependencies during testing. This allows you to focus solely on the timer and scheduler functionality. Also, ensure to test how the timer and scheduler handle errors, such as exceptions thrown during task execution.
java
@Test(expected = RuntimeException.class)
public void testSchedulerExceptionHandling() {
scheduler.schedule(() -> { throw new RuntimeException(); }, 1, TimeUnit.SECONDS);
}
To handle multi-threading issues, consider using synchronized blocks, locks, or thread-safe data structures. These techniques ensure that multiple threads accessing the same resources do not interfere with each other and cause unexpected behavior.
Discover best practices for handling multi-threading issues in timer and scheduler unit testing.
java
synchronized(lock) {
// critical section
}
For managing time zone differences, consider using a library or framework that allows you to mock or simulate time in your tests. Dependency injection can be used to provide a time provider interface to your timer and scheduler classes, allowing you to inject a mock or stub implementation of the time provider in your tests.
java
Clock clock = Clock.fixed(Instant.parse("2022-01-01T10:00:00Z"), ZoneId.of("UTC"));
timer.setClock(clock);
Simulating different time scenarios for testing purposes can be achieved with Mockito's mocking capabilities. You can create a mock object for the timer or scheduler class and then use Mockito's when-then functionality to define the behavior you want for different time scenarios.
java
when(timerMock.schedule(any(TimerTask.class), anyLong())).thenReturn(null);
To reduce technical debt in Java timer and scheduler unit testing, follow best practices for unit testing in Java. This includes using a reliable and robust unit testing framework, such as JUnit, and utilizing mock objects to isolate and test specific components of the timer and scheduler functionality. Regularly reviewing and refactoring the codebase can also help to identify and eliminate any unnecessary complexity or duplication.
In the end, although timer and scheduler unit testing in Java is a complex and challenging task, it is a critical task that can significantly enhance the quality, reliability, and robustness of software applications. With careful planning, meticulous execution, and thorough validation, you can ensure that the timers and schedulers function accurately and consistently under different time scenarios
3. Strategies for Ensuring Accuracy in Timer Based Unit Tests
While crafting precise timer-based unit tests, the key strategy is isolating time-dependent code and manipulating the system clock. This is often achieved by replacing the system clock with a mock or virtual clock during testing. There are numerous techniques to manage time in programming, each with its unique benefits and considerations.
One such technique is the replacement of direct calls to std::chrono::system_clock::now
in unit tests with an alias clock (appClock). This method enables the use of a test clock, bypassing the naive approach of direct invocation of std::chrono::system_clock::now
, which can complicate unit tests.
Another technique is the use of template specialization to encapsulate different clocks for tests and production. This method offers enhanced flexibility and control over time during testing.
Using a clock factory to provide the current time is also an option. In a production environment, the factory returns std::chrono::system_clock
, while during unit tests, a test clock is utilized.
Alternatively, you can pass a clock object to each class that requires time, rather than using singletons. This method ensures a consistent and reliable time source for each class.
Passing timestamps to the code instead of requesting the current time is another strategy that simplifies testing and allows for instrumenting timer code. This method is preferred when the loss of precision is acceptable, making the code more testable and deterministic.
The method of choice will depend on the specific needs of the program and the required precision. For instance, a choice must be made between using a single consistent timestamp or timestamps specific to each processing step, which depends on the program's requirements.
Deterministic scheduling can be beneficial in simulating specific time scenarios, ensuring the code behaves as expected. It's also vital to consider edge cases, such as leap years and daylight saving time changes, to ensure comprehensive test coverage.
Unit tests are critical for identifying regression issues during refactoring, bug fixing, and the integration of new features. They should be fast, independent, repeatable, self-validating, and evolve with the application code. Non-determinism in unit tests can lead to tests occasionally passing and failing. To uncover the root cause of non-deterministic behavior, developers need to thoroughly examine the unit test code and the logic under test.
To isolate time-dependent code in unit tests, you can employ various techniques such as mocking, stubbing, or dependency injection. These techniques allow you to control the behavior of time-dependent code during testing, ensuring consistent and predictable results. By replacing the actual time-dependent code with test doubles, you can simulate different time scenarios and verify the correctness of your code. This approach aids in writing reliable and deterministic unit tests.
However, it's essential to note that the context information provided does not detail the best practices for testing time-dependent code. For such specific information, it is recommended to refer to relevant documentation or resources discussing best practices for testing time-dependent code
4. Techniques for Reliable Scheduler Unit Testing
Unit testing in a time-dependent context, such as testing schedulers or time-based logic, requires a meticulous control over the execution environment. To achieve this, a controlled scheduler can be employed, which simulates the behavior of a real scheduler in a controlled manner. One of the ways to create a controlled scheduler is through the use of a mocking framework like Mockito for Java. Mockito allows you to create a mock object that represents the scheduler, and then define its desired behavior for your unit tests. This way, you can precisely control when and how tasks are scheduled, providing a more reliable test of your code's logic that interacts with the scheduler.
There are also libraries specifically designed for unit testing, such as the NUnit framework for .NET, that provide features for creating and controlling mock schedulers. These tools allow you to simulate the passage of time and control the execution of scheduled tasks, enhancing the reliability of your tests.
Simulating various scheduling scenarios can be achieved by leveraging a test double, such as a stub, for the scheduler. Mockito is particularly useful in this scenario, as it allows you to create a mock object that mimics the behavior of the real scheduler. By defining specific behaviors for the mock object, such as returning certain values or throwing specific exceptions, you can simulate different scheduling scenarios. This approach enables you to test how your code reacts to these scenarios without relying on the real scheduler.
When testing time-dependent code, it is crucial to ensure the scheduler is properly initialized and thoroughly cleaned up after each test to prevent any residual effects. This can be achieved by using a "teardown" or "cleanup" method, which is executed after each test. The teardown method allows you to stop the scheduler, release any resources associated with it, and reset any relevant state, ensuring the scheduler is ready for the next test.
In relation to time management for different test layers in a Java Spring Boot application, a custom datetime provider can be employed to replace all time invocations. This can be implemented as a thread-safe singleton using a DateTimeProvider class, which has methods for getting the current time, setting a fixed time, and resetting the time to the default.
For unit tests, the time can be managed by invoking DateTimeProvider.settime before the test and DateTimeProvider.resettime after the test. For end-to-end testing, a TimeManagementController can be introduced, which exposes a REST endpoint for setting and resetting the time in the application. This approach provides a comprehensive solution for testing time-dependent code in various test layers
5. Implementing Robust and Flexible Testing Frameworks for Timer and Scheduler Tests
As we navigate the intricacies of timer and scheduler unit testing, robust and flexible testing frameworks become invaluable. While some developers may turn to tools like Ditto, the Java ecosystem offers a variety of solutions that can effectively manage the challenges of testing time-dependent code.
One such technique involves the use of stub methods. By replacing or simulating the behavior of certain methods in a unit test, we can control the output of the code being tested. This is particularly useful when testing code that involves time. By stubbing these methods, we can eliminate the dependency on the actual time, ensuring predictable results during testing. This enhances the reliability and repeatability of our tests.
To illustrate, consider a unit test that involves a scheduler. Instead of relying on the system clock, we can stub the scheduler's time-related methods, allowing us to simulate the passage of time and control when tasks are scheduled or executed.
java
// Stubbing the scheduler's time-related methods
Scheduler scheduler = mock(Scheduler.class);
when(scheduler.getCurrentTime()).thenReturn(fakeTime);
In addition to stubbing, spying on real objects is another powerful technique that can be used in timer and scheduler unit testing. Mockito, a popular mocking framework in Java, provides a spy()
method that allows us to create a spy object wrapping around the real object. This spy object can be used to verify and stub specific methods, while retaining the functionality of the real object.
java
// Creating a spy object with Mockito
MyObject myObject = new MyObject();
MyObject spyObject = spy(myObject);
However, we must be mindful of some common issues that can arise when testing timers and schedulers. These include timing dependencies, asynchronous testing challenges, dependencies on external resources, and error handling. By being aware of these issues and addressing them proactively, we can write more reliable and effective unit tests.
Lastly, the practice of continuous integration can further enhance our testing strategy. With continuous integration, we can set our tests to run automatically whenever code changes are made. This allows us to quickly identify and address any issues or regressions, ensuring that our timer and scheduler functionalities are always working as expected.
By leveraging these techniques and practices, we can effectively manage the complexities of testing time-dependent code, leading to higher quality software and a more efficient development process
6. Managing Workload and Balancing Deadlines in Timer and Scheduler Unit Testing
Managing workload and adhering to deadlines in timer and scheduler unit testing can be a challenging venture. It requires a strategic approach, focusing on triaging tests based on their potential impact and associated risks. Prioritizing tests based on impact and risk is a crucial aspect of this testing process. By understanding the potential risks and impact of different components and functionalities, testing resources can be effectively allocated.
It's important to identify critical functionalities that have a high impact on the overall system. These functionalities should be thoroughly tested to ensure their correct behavior and reliability. Also, components with a known history of issues or critical for the overall functionality of the system should be prioritized for testing. A risk-based approach can be used, where tests are prioritized based on the likelihood of failure and the potential impact of those failures. By focusing on high-risk areas, the testing effort can be optimized to identify and address critical issues more efficiently.
The emphasis on automation in the testing process is a strategy that conserves valuable time and ensures a consistent testing environment. Automation eliminates the risk of human error, providing a more reliable testing landscape. Tools such as JUnit, TestNG, Mockito, PowerMock, and EasyMock are some examples of automated testing tools for timer and scheduler unit testing. They provide features and libraries that can be used to test the functionality and behavior of timers and schedulers in a software application.
Scheduling adequate time for testing within the project timeline is another critical aspect to consider. Rushed testing can inadvertently lead to overlooked issues, compromising the quality of the code. Efficient workload management can be achieved by implementing various strategies. These strategies can include prioritizing test cases based on their criticality, optimizing the order of execution, and using parallelization techniques to speed up the testing process.
The inclusion of time abstraction in .NET 8, namely the TimeProvider, has been a significant development. This abstraction encapsulates calls to DateTime.UtcNow and similar APIs, enabling easier testing of time-dependent code. A practical example of this is the Microsoft.Extensions.TimeProviderTesting package, which offers a FakeTimeProvider implementation, allowing developers to control the flow of time during tests.
Similarly, other tools like NodaTime and NodaTimeTesting packages can be employed to address the challenges associated with testing time-dependent classes in unit tests. They offer a FakeClock class, which simulates different points in time, thereby enabling faster and more consistent testing.
In summary, the strategic prioritization of tests, a significant emphasis on automation, and adequate scheduling for testing are crucial in managing workload and balancing deadlines in timer and scheduler unit testing. The utilization of tools such as TimeProvider and NodaTime can make this process more efficient, reliable, and less time-consuming
7. Case Study: Successful Implementation of Timer and Scheduler Unit Testing
The Machinet software development team offers a compelling demonstration of how to effectively incorporate timer and scheduler unit testing into a Java program. They made use of a mock clock, a tool that isolates time-dependent code and allows testers to manipulate the system clock during the testing process. This enabled them to run tests under various time conditions, increasing the robustness of their tests. Libraries such as Mockito in Java can be used for this purpose. Mockito allows for mocking objects and their behavior during unit testing, enabling control over the time returned by the clock object.
Moreover, they utilized a controlled scheduler to manage task execution. This tool allowed them to simulate different scheduling scenarios, further enriching their test cases and enhancing the reliability of their results. JUnit framework and its annotations and assertions can be employed for this purpose. JUnit provides a @Test
annotation for defining a test method that can be executed during the test run. To control the timing of tasks, features such as timeouts, delays, and the Thread.sleep()
method can be used, enabling accurate testing of code behavior under different timing conditions.
Their efforts resulted in a robust and flexible testing framework capable of generating comprehensive tests that thoroughly examined their time-dependent code. The flexibility of the framework ensured the relevance and effectiveness of tests even as the application evolved. JUnit is a reliable testing framework that offers a rich set of features for testing time-dependent code, such as the ability to mock and control the system clock, simulate time-based events, and verify the expected behavior of time-dependent functions and methods.
The team's success underscores the importance of implementing robust testing frameworks and using appropriate tools and techniques in timer and scheduler unit testing. Mocking frameworks can simulate the behavior of the timer or scheduler, enabling control over the timing and scheduling of events in tests. Dependency injection can also be used to decouple code from the actual timer or scheduler implementation, allowing for the simulation of different scenarios. Another effective strategy is the test-driven development (TDD) approach, which ensures that the code is designed to be testable and meets the desired requirements.
The success of their endeavor is a testament to the accuracy and reliability of their time-dependent code. It underscores the importance of implementing robust testing frameworks and utilizing appropriate tools and techniques in timer and scheduler unit testing. This practical example demonstrates the power of these strategies and techniques in ensuring the delivery of high-quality, reliable software applications
Conclusion
In conclusion, accurate timer and scheduler unit testing in Java play a crucial role in ensuring the reliability and robustness of software applications. These tests validate the performance of time-dependent code and identify potential issues such as race conditions, timezone inconsistencies, and unexpected delays. However, implementing these tests can be challenging due to factors like flakiness caused by improper timeout utilization and the unpredictability of test environments.
To overcome these challenges, developers need to align the test environment with the API needs, differentiate between performance and functional tests, and employ techniques for reliable testing. Strategies such as using appropriate timeouts, managing dependencies, and differentiating between performance and functional tests can enhance the accuracy of timer and scheduler unit testing.
By understanding the significance of accurate timer and scheduler unit testing and implementing effective strategies, developers can enhance the reliability and robustness of their software applications. This ensures that potential issues are identified early on in the development process, leading to higher quality software.
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.