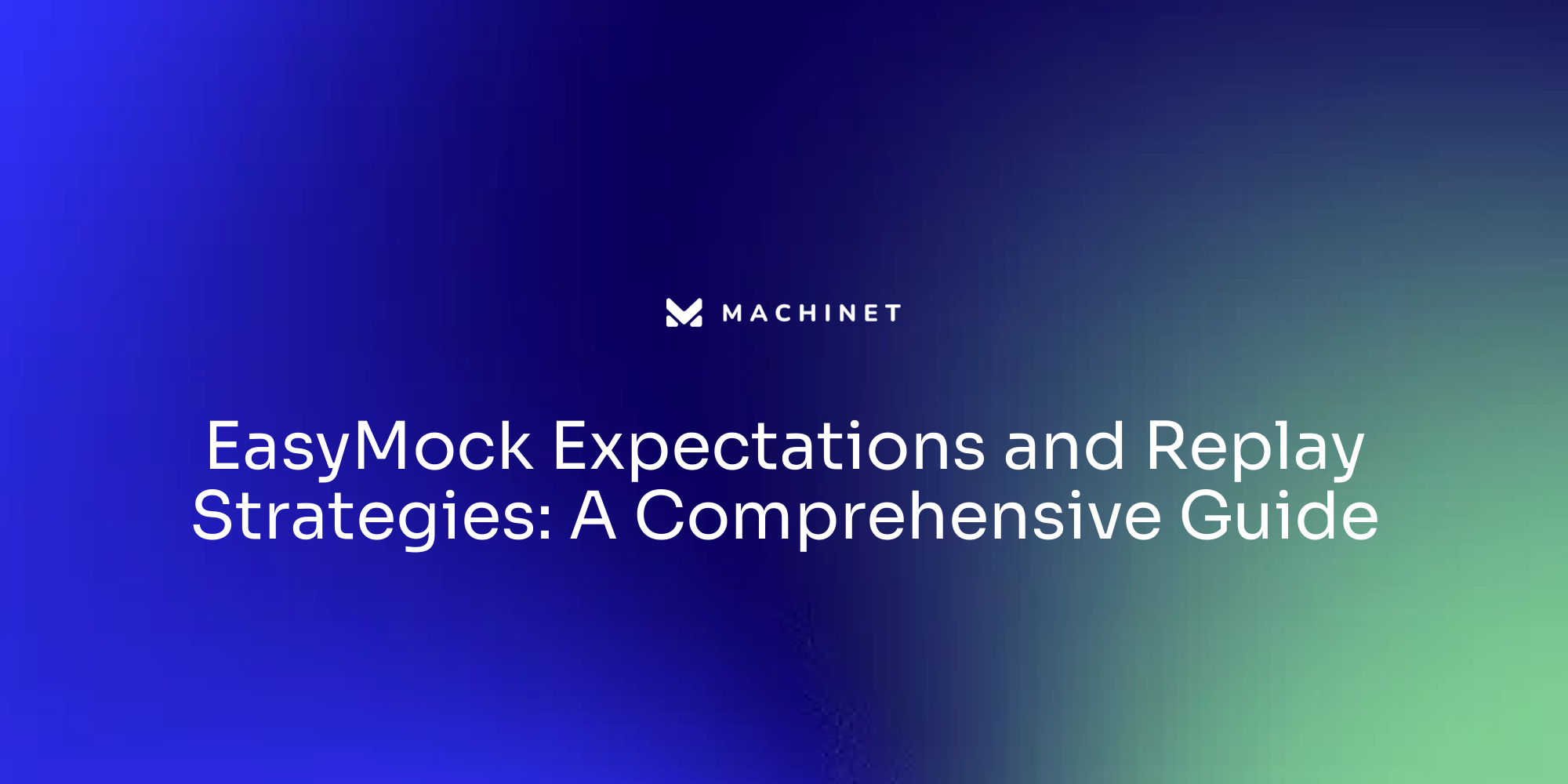
Introduction
Mocking is a crucial technique in software development that allows developers to test their code effectively. By generating mock objects that mimic the behavior of real components, developers can create a controlled environment for testing. Mocks are particularly useful when working with code that has dependencies on external services or resources.
They help isolate the unit of code being tested, ensuring that external factors do not impact the test results. In this article, we will explore the concept of mocking, its benefits, and how to create and manage mock objects using EasyMock, a popular mocking framework. We will also discuss advanced mocking strategies, common pitfalls, and troubleshooting tips.
So, let's dive in and discover how mocking can improve the quality and reliability of your software.
Understanding Mocking and Its Benefits
In the realm of software development, one crucial technique that stands out for its efficacy in testing is mocking. This process involves the creation of substitute objects, which serve as stand-ins for real components, allowing developers to emulate the behavior of those components. Mocks play an instrumental role when working with code that has dependencies on external services or resources like databases and web services. They play a crucial role in establishing a regulated setting that separates the code component under examination, guaranteeing that external variables do not impact the outcomes of the evaluation.
One of the paramount principles in achieving testable code is the separation of concerns, which emphasizes the modularization of code into discrete units that can be individually assessed. These units should be clear in their intent and capable of independent operation without external dependencies. The issue of tight coupling—where parts of the code are interdependent to the point that altering one could adversely affect another—poses a significant obstacle to testability and maintainability. By employing simulated versions, developers can alleviate this problem, enabling each component of the code to function independently during the evaluation stage.
The benefits of mocking are manifold. It not only speeds up the examination process by removing the necessity for real dependencies but also improves coverage, enabling the analysis of edge cases and error scenarios that may be challenging to reproduce with actual objects. Moreover, as stated in a publication by Engineer's Codex, it is essential to maintain a unified source of information within the service, which can be achieved by simulating consistent responses in tests. In the context of code testability, simulated objects are indispensable tools that contribute to the creation of robust, high-quality software.
Creating Mock Objects with EasyMock
EasyMock allows Java developers to create simulated objects effortlessly, preparing for managed and efficient unit or integration assessment. By harnessing the power of EasyMock's API, developers can establish expectations for mock objects, specifying the method invocations they should receive and the outcomes to produce. Its fluent API enhances readability, allowing expectations to be expressed succinctly.
Unit evaluations, pivotal in examining individual components, benefit greatly from EasyMock by verifying that each unit operates as intended, devoid of external dependencies. Integration tests also gain value as EasyMock ensures that various units within an application or external systems interact seamlessly. Shadow Testing, a technique to deploy a new or modified system in parallel with the production environment, leverages tools like EasyMock to assess changes without user impact, thus safeguarding the system's integrity before an official release.
With increasing complexity in software architecture, solutions like EasyMock are essential for developers to maintain system stability while enhancing R&D efficiency. The framework's versatility is demonstrated by its application in diverse evaluation scenarios, from production traffic replication to reducing risks in deployment.
As the technology landscape evolves, tools like EasyMock remain integral, as seen with the recent trend of Java evaluation frameworks gaining popularity for their robust features. These frameworks are instrumental in maintaining Java's relevance amidst the introduction of newer, simplified languages, ensuring that Java continues to be a compelling option for developers.
Setting Expectations with EasyMock
Generating simulated objects in unit testing is crucial for isolating the code under examination and confirming its behavior independently from its dependencies. With EasyMock, developers can utilize the power of expectations to define how simulated objects should respond to method calls, specifying both the number of invocations and the arguments they accept. For instance, you might use expect
to declare a method's expected result, times
to dictate the number of calls, and Return
to specify the return value, and and Throw
when you need the mock to raise an exception. This flexibility allows for the precise tailoring of examination cases to match real-world scenarios, ensuring comprehensive coverage and helping to maintain high standards of software quality and reliability. Furthermore, the flexibility of EasyMock plays a crucial part in tackling industry-wide challenges in unit examination, particularly within the framework of dependency injection frameworks. By emulating various behaviors and outcomes, developers can create strong suites that not only identify issues early but also contribute to the development of more dependable and sustainable code bases.
Using Flexible Matchers in Expectations
EasyMock's ability to handle flexible matchers is a powerful feature that simplifies the verification of dynamic or variable data within software applications. Matchers are versatile tools within EasyMock that enable developers to set up more generalized expectations for method calls. They can be utilized in various ways, such as accepting any argument value or defining a specific range of acceptable values.
For example, the anyObject
matcher is especially helpful when the precise object type is not essential to the success of the evaluation, allowing any object of a specified type to match. Conversely, the eq
matcher is used when the exact value is important, ensuring that the argument matches a specific value. EasyMock also caters to common scenarios with matches tailored for strings, numbers, and collections.
This method of evaluation is advantageous when dealing with code that must operate on a variety of data inputs, as it permits the creation of more adaptable and reusable evaluation scenarios. Furthermore, it aligns with Agile principles, where efficiency and quality are paramount, by supporting the rapid iteration of builds with high-quality features. By employing adaptable matchers, EasyMock enhances an environment for examination that is dynamic and resilient, able to adapt alongside the software it examines.
Replay Mode: Preparing Mocks for Testing
Establishing anticipations on a simulated entity is a crucial phase in the examination procedure, as it specifies the particular actions and results that should happen during an assessment. When using EasyMock, once the expectations are set, the object being tested must transition into replay mode. This mode is essential for the testing phase, as it configures the simulated object to respond only to the predefined method calls, ensuring that it adheres to the scripted interactions and nothing more. Enabling the replay mode is achieved by calling the replay
function, which effectively prepares the simulated object for integration into the testing environment.
During the replay phase, the mock object's behavior is scrutinized to confirm its compliance with the expected outcomes. This rigorous approach aligns with the core principles of test case design, which emphasizes a methodical verification of program behavior through well-defined steps, inputs, and expected results. By concentrating on specific objectives, such as feature validation or bug identification, EasyMock aids in maintaining the robustness of the assessment process.
The significance of such automation tools has been emphasized by recent advancements in the industry, like the declaration of TypeScript 4.9 and its beta release, highlighting the continuous progress in coding practices and tooling. Similarly, tools like Jreleaser have emphasized their mission to streamline release processes and artifact publishing since their inception, illustrating the industry's commitment to enhancing development workflows. As test automation tools evolve, they continue to play a pivotal role in the pursuit of quality assurance excellence, shaping the future of software examination and offering developers innovative ways to refine their code.
Verifying Mocks: Ensuring Expected Behavior
Mock testing is a crucial part of the development process. It allows developers to simulate the behavior of different components that a software module relies on, thus verifying the code's functionality without the need to invoke actual services. With tools like EasyMock, the verification process becomes streamlined. Developers can employ methods such as verify
, times
, and at least Once
to ascertain that the mock objects were called with the correct arguments and in the appropriate sequence. Should there be any divergence from the expected behavior, EasyMock throws an exception, signaling a failure. This process is crucial not only for ensuring that the code interacts properly with other components but also for identifying any unexpected behavior that might lead to issues down the line.
Unit tests serve as a foundational element of verification by focusing on individual pieces of code, usually functions or methods, to confirm their accurate operation in isolation. Automated unit evaluations are conducted regularly throughout the development process, which assists in preserving code quality and functionality. The incorporation of simulated examination within unit assessments enables a more detailed and separated examination environment, liberated from external dependencies. As per a survey, 80% of respondents recognize the significance of conducting tests in their projects, with 58% creating automated tests. This emphasizes the importance of simulating examinations in modern development practices.
In the context of unit evaluation, simulated examination is similar to subjecting the program to a sequence of trials, guaranteeing its durability for real-life uses. Whether it's the complex interactions within an application or the individual components, the practice of simulating examinations, as part of a comprehensive evaluation strategy that includes unit, integration, and other forms of assessment, helps prepare the program for diverse scenarios. As highlighted by industry experts, testing simulations is not only about identifying errors; it is a methodology that enhances the software development process, assisting in the development of excellent APIs and functioning as documentation for new developers to understand anticipated behaviors and design choices.
EasyMockSupport: Simplifying Mock Management
EasyMockSupport is a layer of enhancement for the EasyMock framework, simplifying the creation and administration of simulated objects in automated examinations. Through the incorporation of this tool, programmers are able to define simulated entities within their examination categories, and have them automatically prepared, removing the repetition of manual imitation establishment throughout various examination techniques. This contributes to more readable and maintainable test code.
The tool also introduces methods for verifying interactions with mock objects, thus enhancing the verification process which is crucial in ensuring that systems meet stringent quality and compliance standards—particularly in sectors like banking where M&T Bank has leveraged such standards to maintain robust digital solutions.
In the context of the digital transformation sweeping the banking industry, characterized by a shift to digital customer experiences and the need for heightened security and compliance, tools like EasyMockSupport aid in minimizing maintenance time and costs. They help maintain the Clean Code standards essential for efficient, reliable, and secure applications, as observed in M&T Bank's approach to development.
Utilizing such tools is a component of a wider strategy within development and quality assurance, highlighting the significance of verification early in the development lifecycle. This approach is reflected in the insights from Michael Feathers, who emphasizes the role of tests not only as a safety net but as a guide for improved design and as valuable documentation for new developers.
Moreover, the adoption of new technologies in the software development industry is on the rise. With over 13.4 million professional developers worldwide by 2023, the implementation of dependable evaluation frameworks like EasyMockSupport and TestNG becomes increasingly significant. These frameworks enable streamlined evaluation, from unit to integration levels, and support the current trends where programming languages such as JavaScript, SQL, Python, and HTML/CSS dominate the landscape.
In essence, EasyMockSupport, by enhancing the verification process of mock interactions, plays a vital role in the broader ecosystem of development, where quality, efficiency, and adherence to industry standards are paramount for success in the rapidly evolving digital world.
Advanced Strategies: Partial Mocking and Dynamic Behavior
Utilizing sophisticated mocking techniques in EasyMock can greatly improve the evaluation of software applications, particularly when handling complex codebases or integrating new functionalities. By utilizing partial mocking, developers can selectively choose which methods to simulate and which to leave untouched, operating within their actual scope. This demonstrates immense value in situations where simulating a complete object is either impractical or unnecessary, and can greatly aid in evaluating components that rely on intricate systems or external modules.
Moreover, the capability to define dynamic behaviors in mock objects is a game-changer. It enables the emulation of various outcomes, such as returning diverse results contingent on the arguments passed, or even mimicking delays and exceptions. This level of control allows for more thorough and nuanced evaluation environments, paving the way for more robust and fault-tolerant software systems. As a testament to the effectiveness of dynamic experimentation strategies, consider the case where Etsy's app development teams ran nearly 200 experiments on their listing screen over three years. Despite the high rate of change, they managed to maintain system integrity by employing sophisticated evaluation practices that likely included techniques similar to EasyMock's dynamic behaviors.
In the realm of engineering, the significance of a versatile and all-encompassing quality assurance approach cannot be emphasized enough. The insights from Michael Feathers regarding legacy code highlight the crucial role testing plays not just as a safety net but as a foundation for superior architecture and design. By incorporating sophisticated mocking techniques like those provided by EasyMock, developers can guarantee that their evaluations are not only a protective measure but also serve as a framework for constructing resilient and well-organized software.
Common Pitfalls and Troubleshooting
EasyMock is a versatile tool for mocking dependencies in unit evaluations, but it's not without its challenges. For example, one might have difficulty in establishing the accurate expectations for imitation objects. This is more than a simple pitfall; it's a critical step in ensuring the code behaves as anticipated under examination conditions. To overcome this, developers should define expectations that mirror the actual code behavior closely. In addition, appropriate cleanup of simulated objects is crucial to avoid disruption with other examinations. Utilizing EasyMock's reset
method or the EasyMockSupport utility can help maintain a clean state between evaluations.
Another hurdle could be class loading or compatibility issues when mocking specific objects. This underscores the importance of leveraging EasyMock's documentation and the broader community's knowledge to navigate such complexities. To maintain a commitment to ongoing education, it's crucial to stay updated on the most recent advancements in frameworks such as TestNG, which streamlines a wide range of assessment requirements, spanning from unit to integration assessments. Developers are encouraged to write the logic of their tests, adorn their code with TestNG annotations, and manage test details with the help of a testng.xml file.
In light of recent advancements, tools like JReleaser have emerged, aiming to streamline the release process and artifact publishing to various package managers, providing a glimpse into the industry's direction towards efficiency and modularity. As testing paradigms evolve, it's crucial for developers to adapt and incorporate these tools and methodologies to ensure the delivery of robust, high-quality software.
Conclusion
In conclusion, mocking is a crucial technique in software development that allows developers to effectively test their code. It generates mock objects that mimic real components, creating a controlled testing environment and isolating the code being tested.
The benefits of mocking are numerous. It accelerates testing by eliminating dependencies and enhances test coverage, including edge cases and error scenarios. EasyMock simplifies mock object creation and management with its fluent API and flexible matchers.
EasyMock's replay mode configures mock objects to respond to predefined method calls, ensuring expected behavior. Verifying mocks helps identify unexpected behavior and ensures code interacts properly with other components.
EasyMockSupport streamlines mock object creation and management in automated tests, improving test code readability and maintainability.
Advanced mocking strategies like partial mocking and dynamic behavior enhance software testing, allowing selective simulation of methods and dynamic outcomes.
Developers should be aware of common pitfalls and troubleshooting challenges when using EasyMock, such as setting correct expectations and proper cleanup of mock objects. Staying informed about the latest testing framework developments is essential for adapting to industry trends.
In the rapidly evolving digital world, mocking and tools like EasyMock are vital for improving software quality and reliability. They contribute to creating superior APIs, serve as documentation for new developers, and ensure the success of software development projects.
Try EasyMock today and experience the benefits of improved software quality and reliability!
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.