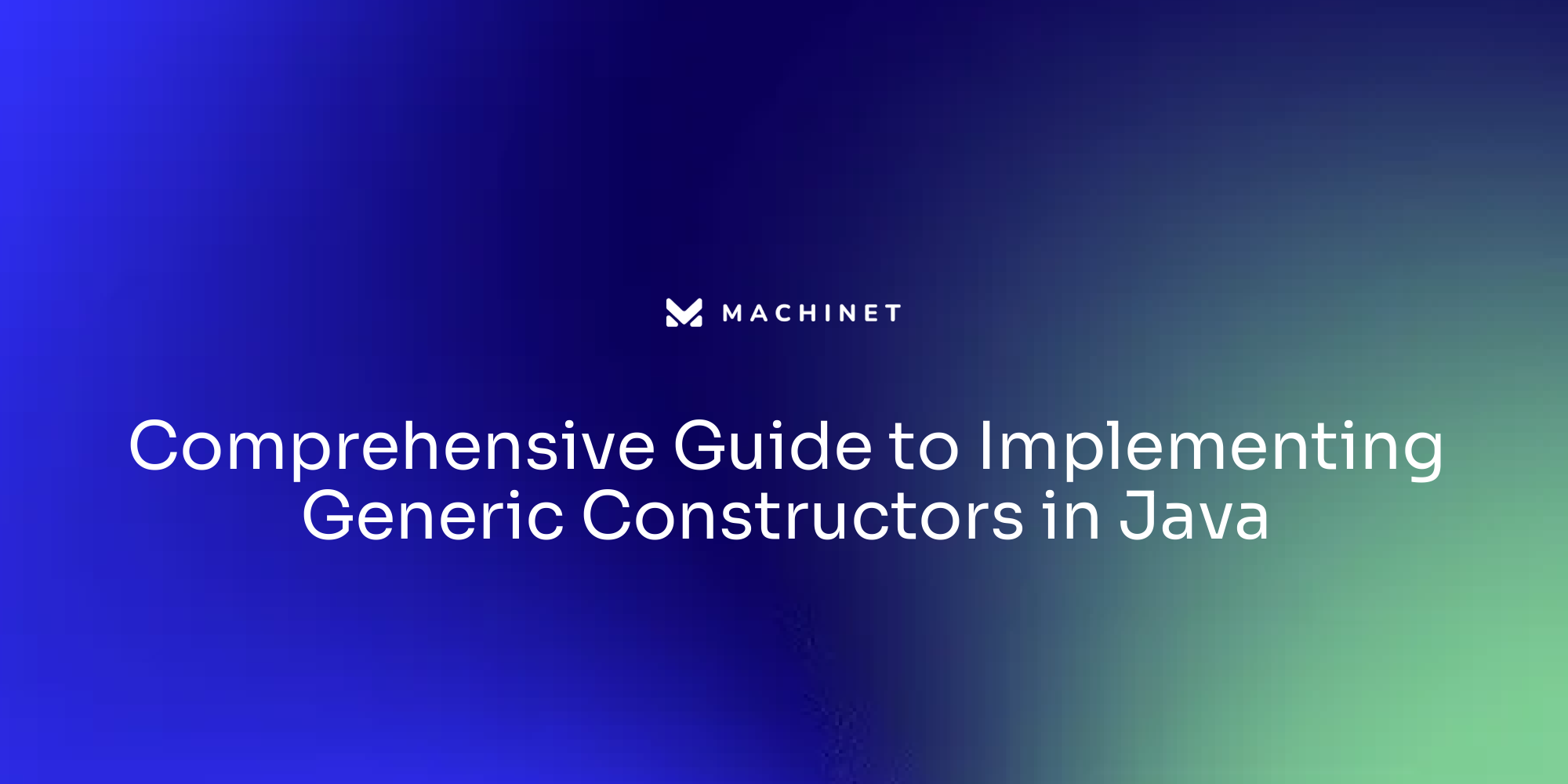
Introduction
Java's generic constructors are a versatile feature that empower developers to design constructors adaptable to various object types, enhancing code organization and reducing redundancy. This article delves into the declaration of generic constructors, illustrating with practical examples how to specify type parameters and utilize them effectively. It also explores the integration of generic constructors within generic classes, showcasing their benefits in creating flexible, reusable components.
Furthermore, the article highlights common use cases and best practices for implementing generic constructors, ensuring efficient and maintainable code that adheres to key programming principles. By embracing these techniques, developers can significantly improve the adaptability and robustness of their software architectures.
Declaring Generic Constructors
Java's versatile builders are a strong aspect that enable programmers to develop builders suited for various kinds of objects. This is particularly useful for enhancing code organization and reducing redundancy. To announce a flexible initializer, indicate the parameter category prior to the initializer's title. For example:
```java
class Box
public <U> Box(U item) {
this.item = (T) item;
}
} ```
In this example, the Box
class constructor is defined with a type parameter U
, enabling it to accept any type when creating a Box
instance. This adaptability in managing categories is one of the numerous ways Java templates enhance more effective and versatile software structure. As mentioned in the development community, templates not only simplify programming but also aid in identifying patterns that improve overall functionality.
Using Generic Constructors in Generic Classes
Non-specific builders inside non-specific classes provide a robust method to manage various categories while maintaining your programming structured and effective. Consider the following example of a generic class with type parameters that define the constructor's behavior:
```java
class Pair
public Pair(K key, V value) {
this.key = key;
this.value = value;
}
} ```
In this Pair
class, the type parameters K
and V
allow developers to create pairs of any types, providing flexibility and reusability. This approach not only simplifies the process of creating objects but also enhances the adaptability and comprehensibility of your software architecture. By utilizing generics, you can effectively handle various data categories, decrease code repetition, and reveal patterns that promote improved code structure.
Common Use Cases for Generic Constructors
Generic builders play a pivotal role in creating flexible and reusable components. They are especially beneficial in data structures such as lists, maps, and sets, where the category of elements can differ. For example, a universal list class can utilize a universal constructor to accept any category of element. This method not only improves code reusability but also guarantees safety by allowing developers to create functions, classes, and interfaces that work with various data formats. This idea, referred to as parametric polymorphism, is essential to type parameters and can be observed in various situations. For instance, a universal function that repeats the input value can manage any data category, owing to the parameter T, which signifies a random data category established during execution. 'This adaptability is essential when creating frameworks or libraries, as it enables new categories of objects to be added without altering existing implementations, following the Open/Closed Principle.'. By utilizing templates, programmers can develop more maintainable, robust, and flexible solutions that suit a broad array of applications.
Best Practices for Using Generic Constructors
When implementing generic constructors, follow these best practices to ensure your code remains efficient and maintainable:
- Use meaningful and contextually relevant type parameters to enhance readability and maintainability. For instance, if you're designing a universal class for a collection, a parameter such as
<T>
clearly shows that it can manage any data category. - Avoid unnecessary complexity by using generics only when they provide clear benefits. Generics should make your programming easier by enabling it to manage various data forms while preserving safety of the data.
- Record your constructors in detail to explain the anticipated categories and behaviors. This makes it easier for other developers to understand and use your code correctly.
- Be cautious with data conversion to prevent runtime exceptions. Improper handling of type casting can lead to unpredictable behavior and bugs, so always ensure that type casts are safe and necessary.
Understanding how to effectively use generics can significantly improve the flexibility and robustness of your code. By following these practices, you can create more adaptable and understandable software architectures.
Conclusion
Java's generic constructors provide a robust mechanism for developing adaptable and efficient code. By allowing constructors to accept various object types, they enhance code organization and reduce redundancy. The ability to declare type parameters before the constructor name facilitates greater flexibility, as illustrated in the examples of the Box
and Pair
classes.
Such versatility not only streamlines object creation but also fosters better management of diverse data types.
Integrating generic constructors within generic classes exemplifies the power of this feature in creating reusable components. The approach allows developers to design systems that are both comprehensible and efficient, making it easier to implement structures like lists, maps, and sets. This adaptability is crucial in modern software development, especially when adhering to principles such as the Open/Closed Principle, which promotes extensibility without altering existing code.
To maximize the benefits of generic constructors, adhering to best practices is essential. Meaningful type parameters, avoiding unnecessary complexity, thorough documentation, and safe type casting are all critical components of effective implementation. By following these guidelines, developers can create code that is not only robust and maintainable but also aligns with key programming principles.
Embracing generics ultimately leads to more flexible and resilient software architectures, enhancing overall development efficiency.
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.