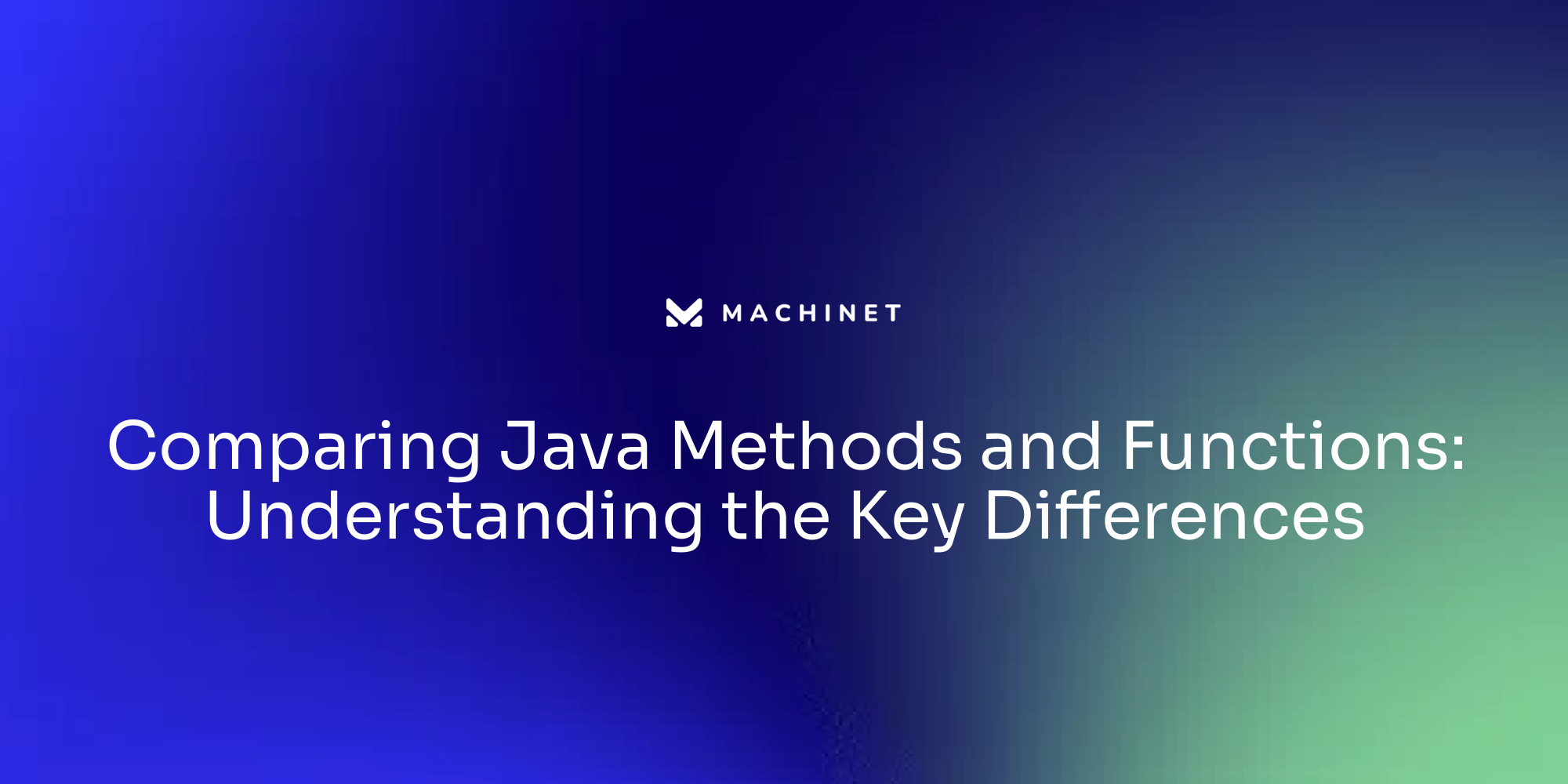
Introduction
Java, with its robust and structured approach to software development, relies heavily on the use of methods and functions. These constructs are essential for developers to create efficient and well-organized code. In this article, we will explore the differences between methods and functions in Java, their definitions and usage, practical examples of their implementation, and performance considerations when choosing between them.
By understanding and effectively utilizing methods and functions, developers can enhance their coding skills and navigate the ever-evolving landscape of software development. So, let's dive in and explore the world of Java's methods and functions together.
Terminology and Context: Methods vs. Functions in Java
Comprehending the intricacies of the functions and procedures in the Java programming language is pivotal for any developer seeking to master the language. Object-Oriented Programming (OOP), a reliable pillar, guarantees that the implementation is not only strong but also well-arranged and structured through these constructs. In Java, an approach is essentially a block of code consisting of a series of statements designed to perform a specific task. Declared within a class, these methods showcase a formal structure:
- accessSpecifier: Governs the method's visibility to other classes.
- returnType: Specifies the data type of the returned value, or 'void' if nothing is returned.
- methodName: The distinct identifier for the way, followed by parentheses.
- parameters: Optional variables passed into the method.
For example:
java
public int addNumbers(int a, int b) {
return a + b;
}
In this snippet, 'public' is the access specifier, 'int' the returnType, 'addNumbers' the methodName, and 'int a, int b' the parameters.
Pattern Matching, a concept introduced to programming language, enhances readability and intuitiveness by allowing values to be matched against defined patterns. It's an advanced technique that can simplify the code by reducing the need for multiple conditional statements.
The continuous development of the programming language over almost thirty years has witnessed its syntax and capabilities expand considerably. Originally conceived as 'Oak' in 1995, the programming language has grown from a technology envisioned for interactive television to a foundational element of modern software development. Its consistent updates and improvements have kept it relevant for contemporary programming needs.
For developers, especially those eyeing roles in big tech, understanding Java's overloading and overriding of functions is essential. These OOP concepts, while different in application, contribute to code's flexibility and maintainability—qualities that are particularly valuable in data science and AI.
Overloading permits multiple functions to have the identical name but with dissimilar parameter lists, enabling a form of polymorphism. On the contrary, overriding happens when a subclass offers a particular implementation for a function already defined in one of its parent classes.
As you explore deeper into the realm of programming, remember that the success of this language is embedded in its structured approach to software development. Methodologies such as Agile and Waterfall provide systematic frameworks that guide you through the software development lifecycle, from planning to maintenance, ensuring efficiency and quality.
Whether you're refreshing your knowledge for an interview or fortifying your skills for industry success, understanding the nuances of methods and functions in this programming language is a definitive step towards mastering this enduring language.
Key Differences: Methods and Functions in Java
From the busy streets of enterprise-level applications to the complex circuits of mobile apps, the presence of this programming language is deeply embedded in the fabric of software development. At the central part of the functionality of this programming language are its building blocks—functions and methods—which are crucial for developers to construct smooth, efficient code.
Methods in programming language are similar to employees in a company, each with a specific role that contributes to the larger goal. They are associated with an object or class and often manipulate the state of that object. For instance, consider a delivery company that has expanded its operations. Similar to how the company adapts its delivery strategies - utilizing bicycles, motorcycles, or cars - based on the city's terrain and distances, the functions in the Java programming language are tailored to the context of the class they reside in, ensuring optimal performance.
Functions, on the other hand, are more like independent contractors, providing a specific service without the need to be attached to a company's hierarchy. In the programming language of Java, these are static functions, which do not operate on objects but perform an action and return a result. An example of this would be a mechanism that calculates a discount based on customer loyalty points, regardless of the customer's other attributes.
As developers, the decision between utilizing a technique or feature in the programming language can be as important as selecting the appropriate means of transportation for a delivery service in an unfamiliar city. Streams, as an example, showcase the capabilities of functions, handling data from collections or arrays in a declarative way without storing the data themselves. This is particularly useful in modern multi-core computers where efficiency is key.
Moreover, the recent enhancements in Java have introduced capabilities like switch expressions, which improve the language's capacity to handle operations more succinctly and expressively. This is similar to a delivery company optimizing its processes to better serve a growing city.
In the constantly changing field of programming, comprehending when to utilize a technique or approach is similar to choosing the most efficient delivery plan. A technique is deeply integrated, capable of nuanced interaction with its environment, while a function is a tool of precision, executing a specific task with detachment from a broader context. As the language continues to evolve and expand, with features like limited functions in the Foreign Function & Memory API, programmers have a versatile set of tools to navigate the structure of their software with the same skill as a delivery company navigating the intricacies of urban growth.
Methods in Java: Definition and Usage
Programming functions serve as the foundation of a program's functionality, enabling developers to encapsulate intricate logic into manageable blocks of code that can be utilized repeatedly. Each function in Java is a sequence of instructions bundled together within a class to achieve a particular operation. The syntax for declaring a function includes an access specifier, which determines who can invoke the function, a return type indicating the data returned by the function, and the function's name followed by parentheses that may enclose parameter declarations.
For example, a method could look like this:
java
public int add(int number1, int number2) {
return number1 + number2;
}
In this snippet, public
is the access specifier, allowing the method to be called from outside the class. The return type int
signifies that the function returns an integer value. add
is the name of the procedure, and int number1, int number2
are the inputs the procedure accepts.
Understanding the proper use of methods prevents 'code smells,' which are indicators of deeper issues in the codebase, often reflecting poor design or complexity that may hinder maintenance and scalability. By following software development methodologies that promote modular programming, developers can create cleaner, more maintainable software.
Modular programming, highlighted by software development methodologies, is a practice that involves breaking down a program into separate, interchangeable modules, where each module handles a specific aspect of the program's functionality. This approach aligns with the Agile principle of continuous improvement, as it allows for easier program updates and bug fixes, leading to a more robust and adaptable application.
Functions in the Java language are essential for enabling developers to write versatile and efficient software, which is particularly important in the context of recent advancements in the Java ecosystem. For example, the OpenJDK JVM's Just-In-Time compilers, like C2, implement optimizations to generate highly efficient compiled output. As software development continues to evolve, effectively leveraging the constructs of the Java programming language becomes increasingly vital for crafting high-quality applications.
Functions in Java: Functional Interfaces and Lambda Expressions
In the programming language of Java, functions play a vital role in achieving modularity and promoting the reuse of code. They become especially potent when combined with functional interfaces—interfaces that define a single abstract procedure. This design pattern is crucial for creating lambda expressions, a feature introduced in Java 8, which empowers developers to write programs that are both concise and expressive.
For instance, consider a scenario where we have a CSV text processor that must handle various separators, such as commas or semicolons. We create a functional interface with a solitary approach to analyze the text, and for every distinct separator, we implement this interface. Therefore, a technique assigned with producing a report would only require to receive this parser object and the input string, streamlining the programming and making it resistant to modifications in separator types.
Lambda expressions represent a significant advancement from Java's conventional object-oriented approach, where each piece of functionality had to be linked to an object or class, frequently resulting in the generation of unnecessary classes. These anonymous functions enable for more direct and less cluttered programming, improving readability and maintainability. The significance of lambdas is evident in the context of cloud technology, where scalability and flexibility are paramount. As David Linthicum, a cloud computing expert, articulates, 'Cloud technology allows organizations to quickly scale up or down based on their needs.' Lambda expressions align with this principle by enabling lightweight and adaptable code.
The incorporation of lambda expressions and functional programming in the language showcases its growth and the community's reaction to the fast-paced advancements in technology, as demonstrated by industry trends and insights. The programming language continues to adapt, ensuring its relevance in the modern landscape of software development and offering a robust platform for developers embarking on their careers.
Comparing Methods and Functions: Practical Examples
Exploring the intricacies of Java's techniques and operations can provide valuable insights into the language's design philosophy and practical applications. A programming language highly regarded for its object-oriented principles, portability, and performance, provides a strong framework for enterprise applications and Android mobile app development. Its syntax, while considered verbose relative to languages like Python, is designed with readability in mind, a boon for developers familiar with object-oriented concepts.
For example, overloading in Java enables the same function name to be used with different parameters, improving code readability and reusability. This is particularly useful in scenarios where the technique's core functionality remains consistent, but its input requirements vary. On the other hand, method overriding enables a subclass to provide a specific implementation of a method that is already defined by its superclass, a powerful feature that facilitates polymorphism and dynamic method dispatch.
Illustrative cases, such as the IntelliJ IDEA by JetBrains, emphasize the significance of the programming language in the contemporary development landscape, despite the emergence of newer, more simplified languages. 'JetBrains' ongoing innovation and dedication to the programming language through their top IDE demonstrate the enduring value of the language to both experienced developers and those who are just starting their coding careers.
This fondness for the programming language is further strengthened by industry trends, where it continues to be a key player among languages used by developers who also work with JavaScript, SQL, Python, and HTML/CSS. This data highlights the language's compatibility and the significance of comprehending its fundamental principles, such as procedures and operations, to develop adaptable, dependable, and sustainable software.
To summarize, a procedure in programming language is more than simply a piece of code; it represents the language's ability for organized, object-oriented design that matches the requirements of extensive, efficient applications. Comprehending and excelling in the utilization of techniques and procedures in the programming language is not just evidence of a developer's technical expertise but also a valuable advantage in the constantly changing field of software development.
Performance Considerations: Methods vs. Functions
Grasping the intricacies of the methods and procedures in Java programming language is essential for attaining peak efficiency in your applications. Methods in Java, which are synonymous with functions in other languages, are essential building blocks. They are used to execute blocks of code and can be called upon multiple times, which can be either synchronous or asynchronous. Synchronous execution blocks the calling thread until the operation completes, while asynchronous approaches enable the thread to continue processing other tasks.
For example, think about a technique that executes a web request. A synchronous request would halt the thread until a response is received, potentially leading to inefficiencies if the response is delayed. On the other hand, an asynchronous request would let the thread execute other tasks concurrently, thus improving performance.
When evaluating performance, it's crucial to take into account the kind of task being executed by the approach. If the task is I/O-bound, involving operations like network requests or file reads, asynchronous methods can significantly enhance performance by preventing a thread from being idle. However, for CPU-bound tasks that are computationally intensive, the benefits of asynchronous execution may be less pronounced.
Furthermore, the performance of the programming language has been finely tuned since its inception nearly three decades ago. The progression from a technology for set-top boxes to a staple in modern software development is a testament to its robustness and adaptability, with performance being a key factor in its enduring popularity.
Choosing between synchronous and asynchronous approaches ultimately depends on the specific requirements of your application and the nature of the tasks. To compose extremely illustrative and ideal programming, it's crucial to grasp the fundamental principles of Java. Providing clear names to procedures and variables can clarify their purpose and actions, enabling the creation of code that is easier to read and maintain.
To sum up, selecting the appropriate form of execution in programming language is not just about syntax but a strategic choice that can influence the performance and responsiveness of your application. By harnessing the powerful features of the Java programming language and making informed decisions, you can create software that not only operates effectively but also performs efficiently.
Best Practices for Using Methods and Functions in Java
Applying best practices in programming functions and procedures is similar to selecting the appropriate vehicle for various delivery scenarios. Much like how a delivery company might use bicycles for short, simple trips and cars for longer, complex routes, developers must use the appropriate structures for clarity and maintainability. A clearly defined approach in Java, consisting of an access specifier, return type, and function name, along with its parameters, resembles a meticulously planned delivery process: it's evident about where it starts, what it carries, and where it ends.
For example, contemplate a technique for handling various types of gifts in an application, which could range from simple postcards to more elaborate presents. The signature of this approach must clearly convey its purpose and the types of gifts it handles. Ensuring approaches are as simple as possible not only eases current development but also future maintenance.
Bear in mind, the main objective of coding isn't to showcase technical expertise with intricacy but to generate programming that's simple to understand and sustain. This is much like writing a program description at the start: it should clearly describe what the program does, its main features, and any unique methods employed, all aimed at reducing errors and enhancing readability. By adhering to these best practices, you'll be better prepared to tackle the challenges of scalability, data governance, and the ethical considerations of handling sensitive information, ensuring your Java code remains robust, efficient, and, most importantly, understandable.
Conclusion
In conclusion, understanding the differences between methods and functions in Java is crucial for developers. Methods are blocks of code within a class that perform specific tasks, while functions are independent and return results. Choosing between them requires strategic consideration based on the specific needs of the application.
Java's continual evolution has introduced features like switch expressions and lambda expressions, enhancing the language's ability to handle functions more concisely and expressively. Understanding when to use methods or functions is key in navigating the ever-evolving programming landscape.
Java methods promote modular programming and adhere to software development methodologies, leading to cleaner and maintainable code. Functions, when coupled with functional interfaces and lambda expressions, contribute to modularity, code reuse, and improved readability.
Performance considerations are crucial when choosing between synchronous and asynchronous methods. Asynchronous methods enhance performance for I/O-bound tasks, while synchronous methods are more appropriate for CPU-bound tasks.
Adopting best practices in using methods and functions is essential for clarity and maintainability. Writing clear and descriptive code, adhering to coding standards, and focusing on readability are key principles to follow.
In summary, understanding and effectively utilizing methods and functions in Java enhance coding skills and enable developers to create efficient and well-organized code. Choosing the right construct and following best practices lead to high-quality applications. Java's structured approach to software development provides a robust framework for developers in the ever-evolving landscape of software development.
Try using Java methods to promote modular programming and create cleaner and maintainable code!
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.