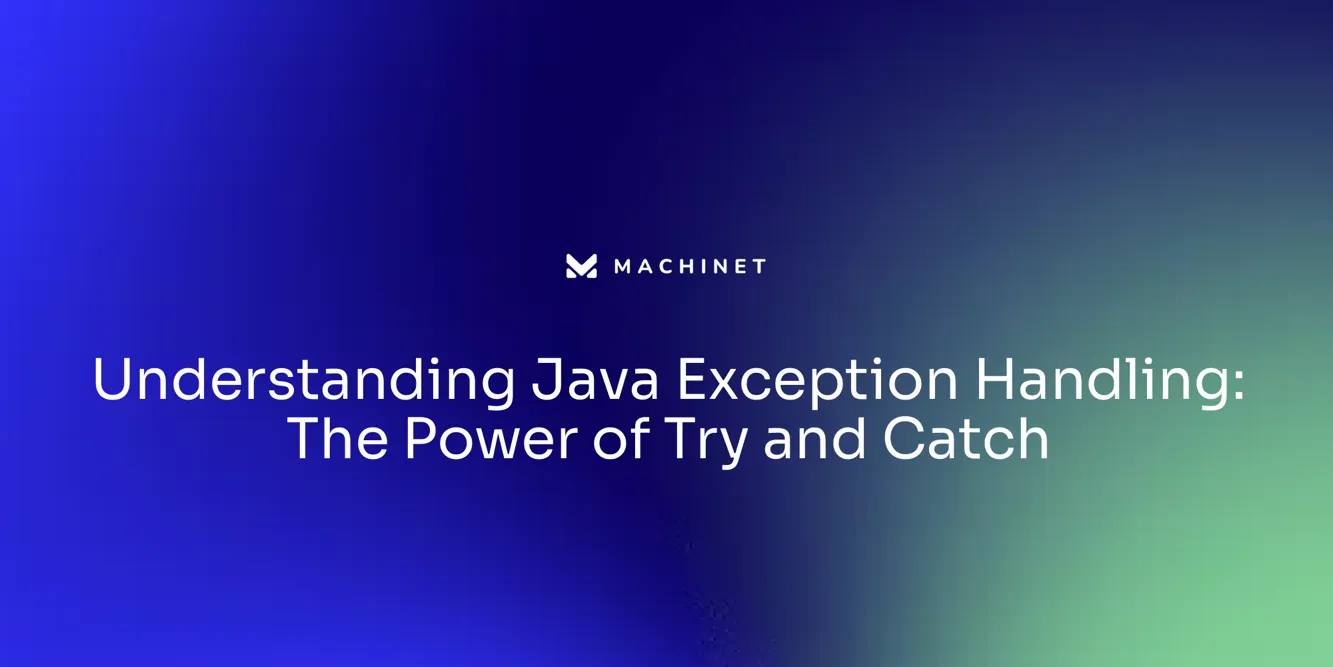
Table of Contents
- Java Exception Handling: The Basics
- Understanding the Try Block
- Using the Catch Block
- The Power of Try and Catch: Examples and Best Practices
Introduction
Exception handling is a crucial concept in Java programming that helps manage disruptions during runtime and improve the reliability of applications. This article provides an overview of Java exception handling, including the basics, the try block, the catch block, and best practices. By understanding and implementing proper exception handling techniques, developers can create more robust and maintainable code.
Java Exception Handling: The Basics
Exception handling in Java is a fundamental concept that bolsters the robustness of applications by managing disruptions during runtime. It involves the creation of an exception, an object thrown at runtime when an unusual event occurs.
If not well-managed, exceptions can abruptly halt a program. Exception handling thus improves the reliability of applications by effectively dealing with these runtime errors.
There are two main categories of exceptions in Java: checked and unchecked exceptions. The Java runtime system stops the execution of the current method when an exception arises, then passes along an exception object with information about the error to the nearest catch block that can handle the exception.
This process is crucial for maintaining the smooth operation of the program. In Java exception handling, catch blocks are examined from top to bottom, with each block handling the exception that matches its condition.
For instance, FileNotFoundException catch block comes into play when the file does not exist at the specified path. If the data format in the file isn't what the application expects, the FormatException catch block is used. The General Exception catch block is a broader one that catches any other types of exceptions not previously handled. This block is particularly useful for catching unexpected exceptions that might not have been foreseen during code development. To handle these exceptions, techniques such as logging, notifying end-users of the issue, and providing appropriate error messages can be employed. This understanding of Java exception handling is vital for developing robust applications and writing more reliable, maintainable code.
Understanding the Try Block
In Java, a try block is a construct used to encapsulate code that might lead to an exception, a disruptive event that interrupts the program's normal flow. The try block contains the code that's intended to be executed.
If an exception arises within this block, it's intercepted and managed by an associated catch block that follows the try block. Each catch block is designed to handle a specific type of exception and to execute a particular set of instructions when that exception occurs.
Exception handling enhances the reliability of Java applications by enabling them to manage runtime errors effectively. This robust mechanism contributes to the smooth execution of applications, ensuring they don't terminate abruptly when an exception is thrown at runtime.
In addition to improving application stability, proper exception handling also makes the code easier to read and maintain, aligning with the principles of clean code. The presence of the var keyword in modern Java, a strongly typed language, also makes it friendlier to use, especially for beginners. This keyword allows developers to define a reference within a method, with the compiler keeping track of the type. The adoption of this feature by Java developers worldwide illustrates the continual evolution of the language to meet the demands of today's programming environments.
Using the Catch Block
In the realm of Java programming, the catch block is an integral part of error handling, playing a critical role in catching and managing exceptions that may arise within the try block. It's key to understand that exceptions are events that disrupt the normal flow of a program.
When an exception occurs, the Java runtime system halts the current method's execution and delivers an exception object to the nearest catch block capable of handling the exception. Within the catch block, programmers can implement code to manage the exception, whether it's to display an error message or take corrective measures.
It's crucial to remember that catch blocks follow a sequential execution order, meaning the arrangement of catch blocks is significant when dealing with multiple exception types. A well-handled exception can prevent abrupt program termination, contributing to the robustness and fault-tolerance of Java applications.
This is part of Java's powerful exception handling mechanism, which not only aids in managing runtime errors but also enhances the application's reliability. Beyond the conventional try-catch construct, there are alternative approaches to exception handling that can make your code cleaner and easier to comprehend. For instance, you might catch an exception within a method, perform some additional actions, and then re-throw it to be managed further up the call stack. This allows for flexible and centralized error management, making your code more robust and maintainable.
The Power of Try and Catch: Examples and Best Practices
To comprehend the full potential of try and catch blocks in Java, let's delve into how they can be utilized to handle a variety of exceptions effectively. For instance, consider the complex situation faced by Netflix when dealing with multiple services.
The traditional approach of managing thread fanout became convoluted quickly due to fault tolerance. This is where the power of reactive programming and libraries like Hystrix came into play, managing threads and ensuring a smooth flow of operations.
When an exception occurs during a program's execution, it disrupts the normal flow of instructions. The Java runtime system halts the execution of the current method and passes an exception object, containing information about the error, to the nearest catch block that can handle the exception.
This mechanism not only helps in dealing with runtime errors but also enhances the application's reliability. In addition to handling exceptions, it's crucial to know how to throw them effectively.
When you throw an exception, you're creating a new exception object. This object contains information about the event that occurred, reflected by the exception type and other properties like the exception message, which provides a more detailed description of what happened. While it's important to handle exceptions gracefully, it's equally crucial to avoid overly broad catch blocks and log exceptions for troubleshooting and debugging purposes. For example, the NumberFormatException, which can be thrown by Integer.parseInt, is a runtime exception. Although this code compiles, we can still choose to handle the exception, thereby providing a more robust and fault-tolerant application. In conclusion, proper understanding and implementation of try and catch blocks can ensure that your Java applications are robust and fault-tolerant, enhancing the overall efficiency and maintainability of your codebase.
Conclusion
Java exception handling is a crucial concept that improves the reliability of applications. By implementing proper techniques, developers can create more robust and maintainable code.
Exception handling involves using try blocks to encapsulate code that may cause exceptions. Catch blocks play a critical role in catching and managing exceptions, preventing abrupt program termination.
Alternative approaches to exception handling, such as re-throwing exceptions, can make code cleaner and easier to comprehend. Throwing exceptions with detailed information about the event enhances application reliability.
Understanding and implementing try and catch blocks properly ensures that Java applications are robust and fault-tolerant. This enhances the overall efficiency and maintainability of the codebase. In conclusion, exception handling is vital for creating reliable Java applications. It allows developers to effectively manage runtime errors and improve application stability. By mastering exception handling techniques, developers can write cleaner, more maintainable code.
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.