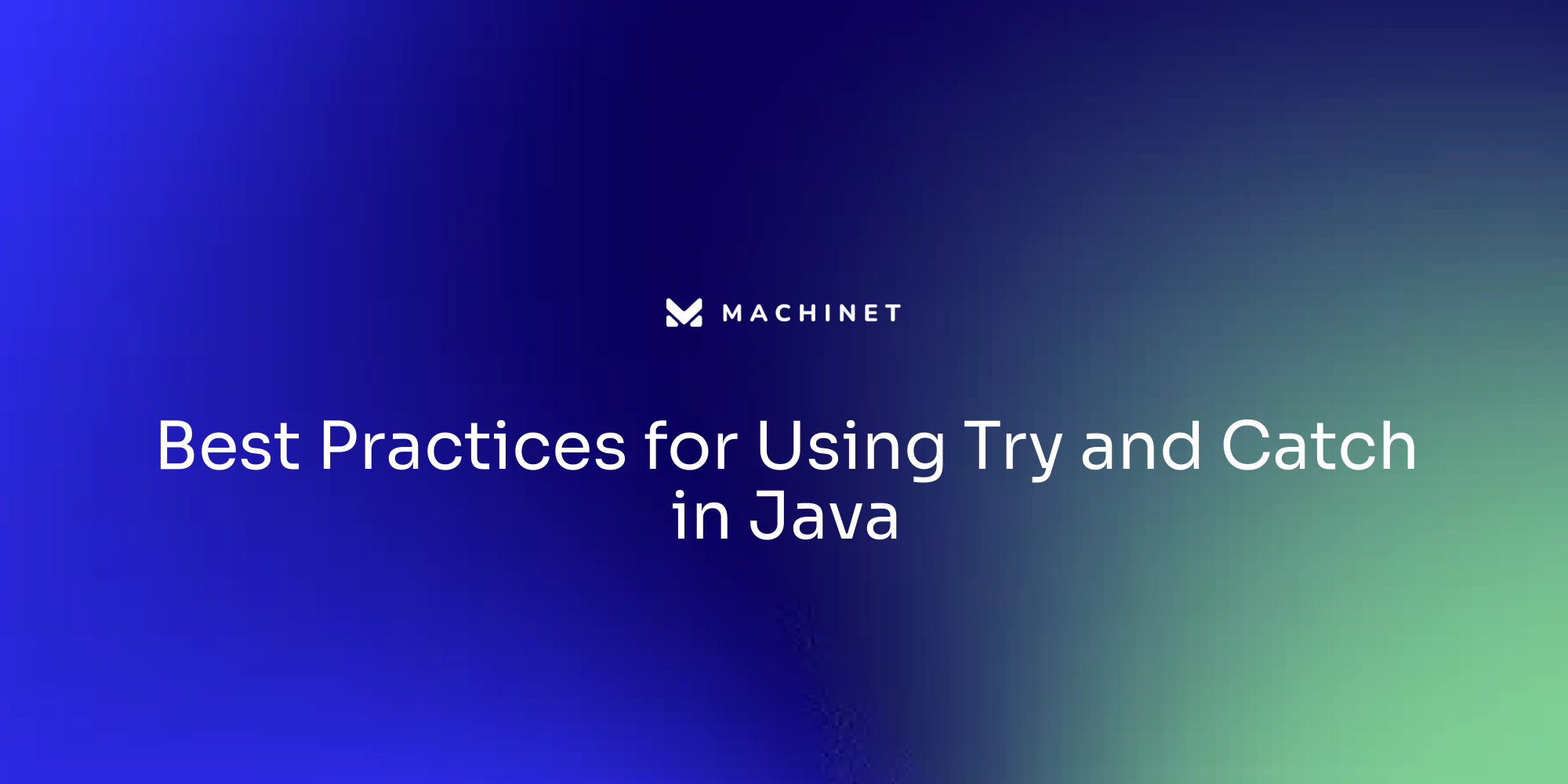
Introduction
Effective exception handling is a cornerstone of robust software development, enabling applications to manage errors gracefully and maintain stability. This article delves into various best practices for exception handling, emphasizing the importance of using specific exception classes to target different types of errors. It highlights the significance of catching exceptions at the appropriate level of abstraction, ensuring sufficient context for resolution, and the critical role of proper logging for diagnosing issues in production systems.
Additionally, the discussion covers the pitfalls of catching generic exceptions, the benefits of the try-with-resources statement for automatic resource cleanup, and the principle of throwing exceptions early while handling them late. It underscores the importance of checking for suppressed exceptions and catching the most specific exception first to prevent overshadowing more detailed errors. Furthermore, the article advises against using try-catch blocks as logical operators and provides insights into best practices for managing nested try-catch structures.
These guidelines aim to enhance code readability, maintainability, and overall application resilience, drawing from expert recommendations and real-world examples.
Use Specific Exception Classes for Different Types of Errors
'Utilizing particular specialized classes enables programmers to manage issues more efficiently and retain improved control over the program's progression.'. By catching specific errors such as IOException
, SQLException
, or custom issues instead of a generic Exception
, one can pinpoint the exact problem and respond appropriately. This approach not only aids in identifying problems with greater clarity but also facilitates the implementation of targeted solutions. For example, the Spring Integration 6.2.0 version tackled issues caused by 550 FTP Response failures by enhancing the Redraw()
and finalizeR()
methods. Similarly, Spring Vault 3.1 introduced features to handle authentication errors more robustly. These examples emphasize the importance of utilizing detailed error classes to improve software robustness and reliability.
Catch Exceptions at the Appropriate Level of Abstraction
Efficient error management is essential for creating strong applications that can smoothly handle unforeseen circumstances. Handling errors at the appropriate level guarantees that you possess enough context to resolve the problem efficiently. Catching errors too early can strip away valuable context needed for resolution, while catching them too late can result in unhandled issues and unpredictable application behavior. Strive to capture errors when you possess sufficient information to take significant measures and reduce possible problems, improving the application's reliability and user experience.
Log and Handle Exceptions Properly
Proper logging of exceptions is essential for diagnosing problems in production systems. Establishing a strong logging system assists in capturing the stack trace and pertinent issue details, which can be vital for efficient troubleshooting. For example, CloudFlare's logging pipeline manages millions of log events per second, demonstrating the importance of a reliable logging system. It is vital to ensure that logs do not expose sensitive information and provide sufficient context for debugging. Automating log analysis with tools like Python can streamline the process, allowing for quicker detection and resolution of issues. Moreover, redundancy in logging pipelines, as practiced by CloudFlare with their dual data centers, can prevent data loss and ensure continuous log availability. By adopting comprehensive logging strategies, you can safeguard your application's health and enhance the user experience.
Avoid Catching Generic Exceptions
Capturing general issues, like Exception
or Throwable
, can hide the real problem and result in inadequate handling. Specific exceptions provide more information about what went wrong and help in debugging. For instance, differentiating between mistakes and failures is essential, particularly in critical systems such as financial software. Mistakes, such as syntax or logical issues, directly result from problems within the code, while failures may occur due to external factors like third-party provider downtime. For example, if a payment provider's API is unavailable, it is a failure rather than an error, highlighting the importance of handling such scenarios properly to maintain application integrity. Always strive to catch the most specific error possible to address the exact issue effectively, enhancing the resilience of your application against unexpected scenarios.
Use try-with-resources for Automatic Resource Cleanup
The try-with-resources statement in Java is an essential tool for managing resources like files or database connections efficiently. By automatically closing resources at the end of the statement, even when exceptions occur, it significantly reduces the risk of resource leaks. This feature not only enhances the reliability of the program but also improves its readability. For instance, rather than manually shutting a database connection, which can result in possible issues if overlooked, the try-with-resources statement manages this procedure effortlessly. This approach aligns with best practices in programming, such as encapsulation and the Single Responsibility Principle (SRP), ensuring that each class or method has a clear and focused responsibility, thereby making it easier to maintain and debug.
Throw Early and Handle Exceptions Late
Raising exceptions right away upon identifying a fault condition is essential for preserving tidy and comprehensible programming. This approach allows developers to address potential issues at their source, reducing the time spent on bug fixing and enhancing overall code quality. By isolating mistake handling logic from business logic, developers can create more maintainable and robust applications capable of dealing with unexpected situations effectively. This proactive error management aligns with the Agile principle of continuous attention to technical excellence, ensuring that the system remains resilient and responsive to real-world conditions, ultimately leading to fewer bugs and more efficient development cycles.
Check for Suppressed Exceptions
When an error arises while managing another error, it can be suppressed. This means the second error won't be propagated up the call stack, but it can still provide valuable insights. To access these details, use the getSuppressed()
method. This method is crucial for comprehending the complete context of faults, as hidden issues might uncover further problems that arose during the initial handling process. For example, when incorporating with intricate systems such as JobRunr or transferring outdated code to more reliable cloud-oriented frameworks, verifying for hidden issues can be essential for upholding strong error management.
Catch the Most Specific Exception First
When dealing with several catch blocks, it is essential to capture the most specific errors first. Java's error handling mechanism follows a top-down approach, meaning if a more general issue is caught first, the specific ones will not be reached. This guarantees that each category of issue is managed suitably and avoids more particular situations from being eclipsed by broad ones. As James Gosling, the father of Java, emphasized, understanding the motivation behind checked and unchecked exceptions can significantly enhance the robustness and maintainability of your programming. This practice not only helps in managing errors gracefully but also in maintaining a clear and organized codebase.
Best Practices for Nested Try-Catch Blocks
Deeply nested try-catch blocks can significantly increase the complexity of your program, making it harder to read and maintain. Refactoring your code to minimize these nested structures can enhance overall code quality. One effective approach is to separate mistake handling into distinct methods. This practice not only simplifies the main logic but also isolates error management, contributing to a cleaner and more maintainable codebase.
Improving structure during evaluations can be especially advantageous. It enables developers to recognize and tackle potential problems, ensuring that the program is both clean and maintainable. Regularly scheduled refactoring, as part of routine maintenance, keeps the codebase efficient and reduces technical debt. The primary objective is to consistently enhance software quality, making development more predictable and efficient.
James Gosling, the father of Java, emphasized the importance of error handling in maintaining robust software. By understanding and applying best practices for refactoring, developers can ensure their work remains efficient and easy to maintain. Simplifying conditional expressions and breaking down complex methods into smaller pieces are key techniques that aid in this process.
Avoid Using Try-Catch as Logical Operators
Using try-catch blocks for control flow can degrade performance and obscure code readability. Exceptions should indicate unforeseen problems, not regular conditions. Instead, use conditional logic to handle predictable scenarios. This approach ensures your application remains robust and capable of dealing with real-world situations, rather than just success paths. According to James Gosling, the father of Java, unchecked errors should address programming faults or invalid inputs, whereas checked errors should manage problems beyond the developer's control, such as network failures. By distinguishing between routine failures and critical errors, developers can better manage and resolve issues, especially in scenarios involving third-party interactions or financial software integrity. Implementing proper exception handling helps build resilient applications that can effectively respond to unforeseen problems without compromising user experience.
Conclusion
Effective exception handling is essential for creating robust and resilient software. By utilizing specific exception classes, developers can enhance clarity and control over error management, leading to more targeted solutions. Catching exceptions at the appropriate level of abstraction ensures that sufficient context is available for effective resolution, contributing to a stable application experience.
Proper logging and handling of exceptions play a vital role in diagnosing issues, particularly in production environments. A reliable logging framework captures critical error information, facilitating quicker troubleshooting and maintaining application integrity. Avoiding the pitfalls of catching generic exceptions is crucial, as it allows for more precise debugging and better error management.
Adopting best practices such as using try-with-resources for automatic resource management, throwing exceptions early, and checking for suppressed exceptions can significantly improve code quality and maintainability. Prioritizing the most specific exceptions in catch blocks prevents overshadowing of important errors, while minimizing nested try-catch structures enhances code readability.
Ultimately, avoiding the use of try-catch blocks as logical operators promotes clearer and more efficient error handling. By implementing these best practices, developers can create applications that are not only more resilient to unexpected issues but also easier to maintain and enhance over time.
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.