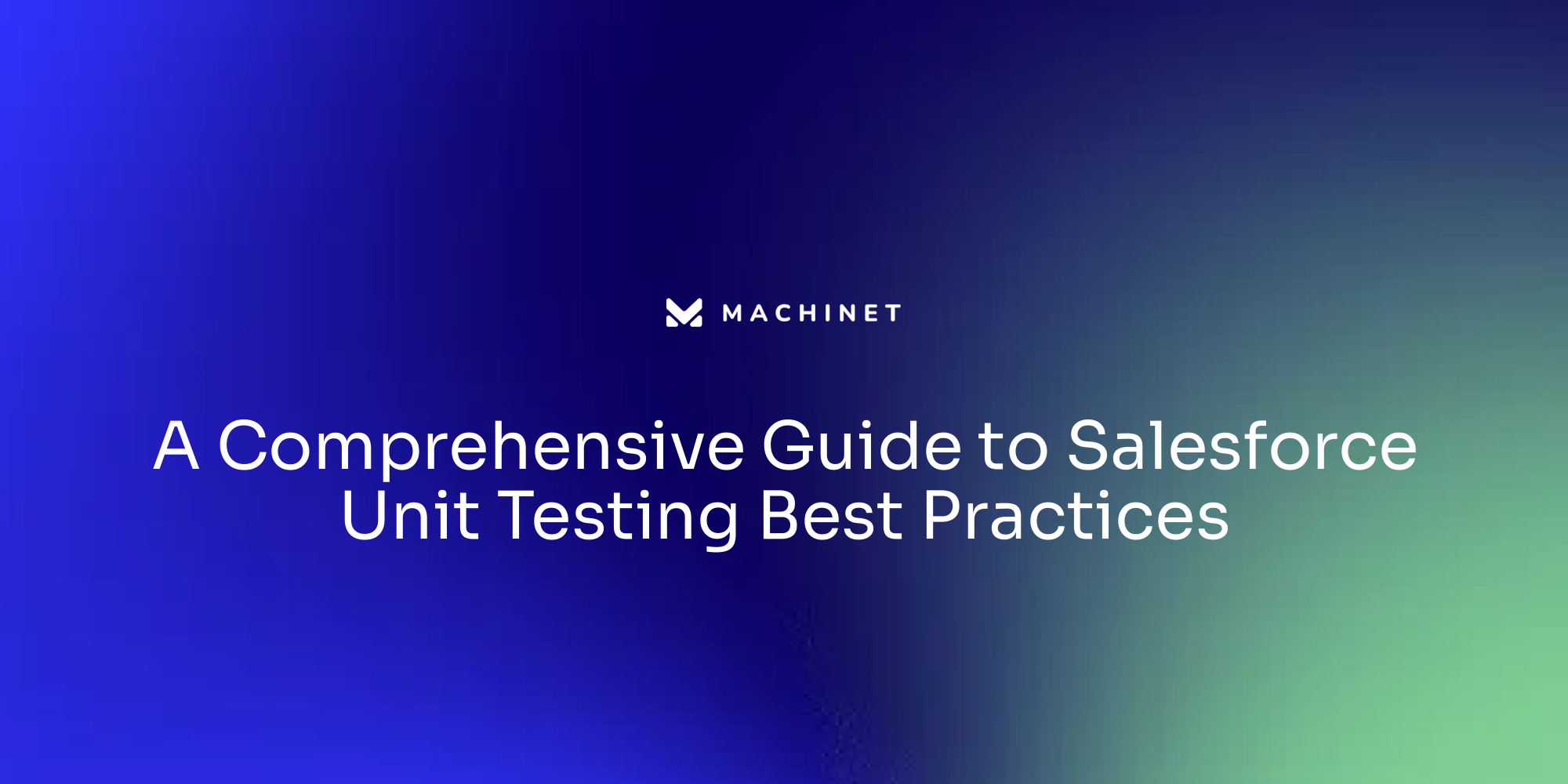
Introduction
In the dynamic world of Salesforce development, ensuring robust and reliable software through effective unit testing is paramount. This article delves into the core principles and best practices that underpin successful unit testing, providing a comprehensive guide for developers to enhance code quality and software performance. From understanding the intricacies of business logic to meticulously crafting test cases, each section offers valuable insights into achieving thorough test coverage and maintaining test integrity.
The discussion begins with key principles that highlight the importance of well-defined test cases, covering functional aspects, edge cases, and potential failure points. It then moves on to best practices for writing unit tests, emphasizing the need for small, focused tests that run in isolation. Additionally, the article explores strategies for covering all scenarios and edge cases, utilizing System.assert() statements for validation, and leveraging Test.startTest() and Test.stopTest() methods to manage governor limits.
Further sections address the creation of test data using Test Data Factory methods, the importance of handling exceptions and errors, and the necessity of testing both bulk and single records. The article also underscores the significance of maintaining test isolation with the @isTest(SeeAllData=false) annotation and the benefits of using Test.loadData() for external data.
Finally, the discussion turns to ensuring code coverage and test completeness, avoiding common pitfalls, and the advantages of parallel testing. By adhering to these guidelines, Salesforce developers can achieve higher code quality, reduce the risk of defects, and ensure their applications perform reliably under various conditions.
Key Principles of Effective Unit Testing
Effective unit testing in Salesforce hinges on a deep understanding of business logic and the anticipated outcomes of the code. Well-designed evaluations are the backbone of this process, systematically confirming various aspects of software behavior. It is crucial to create evaluations that encompass functional elements, edge situations, and possible failure points.
Each test case should have clear objectives, such as validating specific features or identifying bugs. Components like a unique Test Case ID, descriptive title, preconditions, input data, steps to reproduce, and expected results are crucial. Tests should be repeatable, isolated, and independent to avoid unintended side effects.
Furthermore, incorporating evaluations early in the Software Development Lifecycle (SDLC) assists in recognizing problems before they become deeply embedded, lowering the chance of expensive rework. Code should also be written with testability in mind, allowing for easy mocking and stubbing of dependencies, ensuring robust and effective testing.
Best Practices for Writing Unit Tests
Creating unit evaluations in Salesforce necessitates a systematic method to guarantee dependability and sustainability. To start off, unit evaluations must be small and focused, targeting specific functionalities. This precision helps in quickly identifying issues and maintaining the codebase. Furthermore, evaluations should operate independently to prevent reliance on real data or conditions, guaranteeing uniformity and dependability across various settings. Utilizing descriptive names for examination methods improves readability and clarifies the purpose of each evaluation. This clarity is essential for anyone evaluating or upholding the assessments, ensuring they comprehend the specific functionality being validated and the anticipated results.
Covering All Scenarios and Edge Cases
A thorough unit assessment collection must encompass all potential situations, including standard flows and edge conditions. Every examination should have particular goals, such as confirming features, guaranteeing integration, or detecting potential errors. This includes creating evaluations for anticipated inputs, unforeseen inputs, and boundary conditions.
Key components of a test case include: - Test Case ID: A unique identifier for easy reference and tracking. - Test Case Title: A concise and descriptive title that reflects the purpose of the examination. - Preconditions: Required criteria that must be met prior to carrying out the evaluation. - Input Data: Specific data inputs or conditions required for the evaluation. - Steps to Reproduce: A step-by-step guide on how to perform the case.
By considering all possible paths through the code, developers can ensure that their logic is robust and can handle real-world situations. As stated in the World Quality Report, merely 56% of organizations possess adequate evaluation coverage, emphasizing the necessity for comprehensive assessment. AI-powered evaluation can expand assessment coverage by as much as 85%, enhancing software quality considerably. Additionally, organizations using AI-driven testing reported a 30% reduction in testing costs and a 25% increase in testing efficiency.
Using System.assert() Statements
System.assert() statements are essential for validating outcomes in unit evaluations. These assertions ensure that the actual results align with the expected outcomes, effectively verifying the functionality being evaluated. Utilizing several assertions within the same method can offer thorough validation of various aspects, preserving clarity and focus. Each test scenario should have specific objectives, outlining what aspect of the software's functionality or performance it aims to evaluate. This organized method guarantees strength and efficiency in the evaluation process. Test cases are meticulously crafted, featuring components like a unique Test Case ID for tracking, a descriptive Test Case Title, necessary Preconditions, specific Input Data, and detailed Steps to Reproduce. These elements collectively contribute to a thorough verification of the software's behavior, enhancing the reliability of the evaluation process.
Utilizing Test.startTest() and Test.stopTest()
The Test.startTest()
and Test.stopTest()
methods play a crucial role in Salesforce unit testing by managing governor limits and simulating user interactions. By placing code that consumes resources between these two methods, developers can reset the limits, ensuring that the evaluations are not influenced by limits set by previous operations. This is essential for maintaining the integrity and reliability of the case scenarios, which are designed to systematically verify different aspects of the software's behavior and performance.
Creating Test Data with Test Data Factory Methods
Generating sample information is a crucial element of unit evaluation, guaranteeing that assessments are consistent, dependable, and repeatable. Employing Test Data Factory techniques enables efficient and reusable sample generation. This method not only encourages uniformity across evaluations but also lessens the difficulty of handling information within separate assessment procedures. By automating the generation of information sets needed for evaluating applications, a Test Data Generator (TDG) can replicate real-world scenarios without the need for live production information.
The uniformity provided by automated information generation guarantees that the same set is utilized across various examination cycles, which is vital for regression evaluation and confirming that solutions do not bring about new problems. As software applications grow more complex, the volume of evaluation information needed has risen dramatically. Test engineers can load applications with information or stress them with large amounts of invalid inputs to check breakpoints and other aspects of performance.
Furthermore, keeping experimental information organized guarantees its reproducibility. Key components of information storage include permissions, ensuring that many teams can access the information while only a few can edit it, and traceability, where each point is uniquely identifiable. Improved information masking methods also contribute to safeguarding sensitive details while preserving the functionality and significance of the sample information.
In essence, leveraging Test Data Factory methods and automated data generators plays a critical role in modern software development and evaluation, improving software quality and ensuring comprehensive evaluation.
Handling Exceptions and Errors
Unit assessments must confirm both the anticipated results and the appropriate management of exceptions. Every evaluation scenario should have clear goals, such as ensuring that the appropriate exceptions are triggered under faulty conditions. This is essential for validating the robustness and reliability of the code. For example, a well-structured examination scenario should feature a distinctive identifier (Examination Scenario ID), a detailed title, preconditions, particular input data, and a step-by-step guide to replicate the situation. According to the Consortium for Information & Software Quality (CISQ), the cost of defective software surpassed $2 trillion in 2022, highlighting the critical need for thorough testing. By meticulously crafting examination scenarios, developers can minimize the occurrence of bugs and enhance software quality.
Testing Bulk and Single Records
Salesforce applications often operate on both single and bulk records, making it crucial to write tests that efficiently handle both scenarios. Effective performance evaluation can identify any bottlenecks in your code, whether dealing with one record or thousands. This ensures that your application maintains optimal performance and reliability, regardless of the load. By detecting and addressing performance issues early in the development cycle, businesses can safeguard productivity and profitability. As noted, "Performance evaluation can help to identify performance bottlenecks in Salesforce, such as slow-loading pages, poor database design, or inefficient code." This proactive approach not only improves code quality but also ensures compliance with SLAs, ultimately leading to a seamless user experience. Moreover, performance testing aids scalability, enabling your Salesforce configuration to expand alongside your business requirements, managing higher information loads and user quantities without sacrificing efficiency.
Using @isTest(SeeAllData=false) for Isolation
'To maintain isolation during assessments and ensure precise outcomes, developers should utilize the @isTest(SeeAllData=false) annotation.'. This practice prevents evaluation methods from accessing actual data in the organization, thereby ensuring assessments are independent of the current state of the database. Using this annotation contributes to more reliable and consistent test outcomes. Moreover, utilizing sandbox environments for development and evaluation can further lower risks to the production environment. Sandbox environments, which are replicas of the production environment, allow for safe planning, building, testing, and deploying of new features or settings without impacting live information. This approach not only reduces IT costs but also accelerates the development process by allowing updates and changes to be tested thoroughly before deployment, safeguarding the production environment from potential disruptions.
Leveraging Test.loadData() for External Data
When handling intricate information frameworks, utilizing the Test.load Data()
function enables developers to import external content into their evaluations effectively. This method streamlines the setup procedure for evaluations that necessitate particular information configurations, making it simpler to execute and uphold evaluations. As Mudit Singh from LambdaTest highlighted at the Spartans Summit, automation and structured testing processes are revolutionizing the effectiveness of software quality assurance. By utilizing the Test.loadData()
method, developers can avoid repetitive setup across multiple test cases, thus reducing the risk of inconsistencies and ensuring that all tests are aligned with the latest structures. This method is particularly beneficial in scenarios where mock data needs to be consistent yet flexible enough to accommodate changes, embodying the builder design pattern principles that promote easily created instances with custom data overrides.
Ensuring Code Coverage and Test Completeness
Salesforce mandates a minimum of 75% code coverage for deployment, but aiming for higher coverage can significantly enhance code quality. Code coverage measures the percentage of code executed during testing and is crucial for identifying untested areas. To achieve comprehensive coverage, developers should ensure their unit examinations encompass all branches of logic and edge cases. Regularly updating tests as the codebase evolves is vital to maintaining test completeness and adapting to new functionalities.
The significance of comprehensive evaluation is emphasized by the swift speed of software advancement and the necessity for strong quality assurance. According to a McKinsey report, organizations equipped with top-tier tools experience a 47% increase in developer satisfaction and retention rates. Moreover, integrating modern DevOps practices can lead to a 30% increase in deployment rates and a 28% boost in developer productivity. These statistics highlight the importance of investing in efficient evaluation and coverage strategies to ensure high-quality software delivery.
Avoiding Common Pitfalls and Best Practices for Parallel Testing
Frequent mistakes in unit evaluation involve depending too much on information from the organization, overlooking exception management, and not assessing edge scenarios. To enhance evaluation efficiency, implementing parallel assessment practices is essential. Simultaneous evaluations enable several assessments to operate at the same time, greatly decreasing total execution duration. This method ensures that assessments remain independent and do not interfere with one another, enhancing reliability.
Parallel evaluation, also referred to as shadow evaluation, involves deploying a new or modified system alongside the existing production system in a parallel environment. This setup enables developers to observe and evaluate the behavior of the new system in a real-world setting before it is officially released. Such an approach helps mitigate risks by identifying potential issues before they can disrupt normal operations.
It's a good practice to run your tests on various combinations of platforms, devices, and browsers to ensure comprehensive coverage. By doing so, you can maximize the return on investment (ROI) and enhance the quality assurance process. As noted by experts, parallel evaluation not only reduces the budget but also increases coverage in the release cycle.
In essence, parallel testing provides a controlled environment for thorough evaluation and validation, ensuring a smoother deployment process and enhancing the quality and reliability of software systems.
Conclusion
Effective unit testing in Salesforce is essential for ensuring robust software performance and reliability. By adhering to well-defined principles and best practices, developers can enhance code quality and mitigate the risk of defects. Key aspects such as crafting clear test cases, managing governor limits, and utilizing structured test data play a significant role in achieving comprehensive test coverage.
The importance of covering all scenarios, including edge cases, cannot be overstated. By meticulously designing tests that account for both expected and unexpected inputs, developers can create resilient applications capable of handling real-world conditions. Employing tools like System.assert()
for validation and leveraging methods such as Test.startTest()
and Test.stopTest()
ensures that tests are both effective and efficient.
Moreover, maintaining test isolation with the @isTest(SeeAllData=false)
annotation and utilizing Test.loadData()
for external data further contribute to the integrity of the testing process. Emphasizing thorough exception handling and testing for both bulk and single records solidifies the reliability of the code, ensuring optimal performance under varying conditions.
In conclusion, achieving high code coverage and test completeness is not just a regulatory requirement but a fundamental practice that significantly enhances software quality. By avoiding common pitfalls and embracing parallel testing strategies, developers can streamline their testing processes, ultimately delivering superior applications that meet user expectations and business needs.
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.