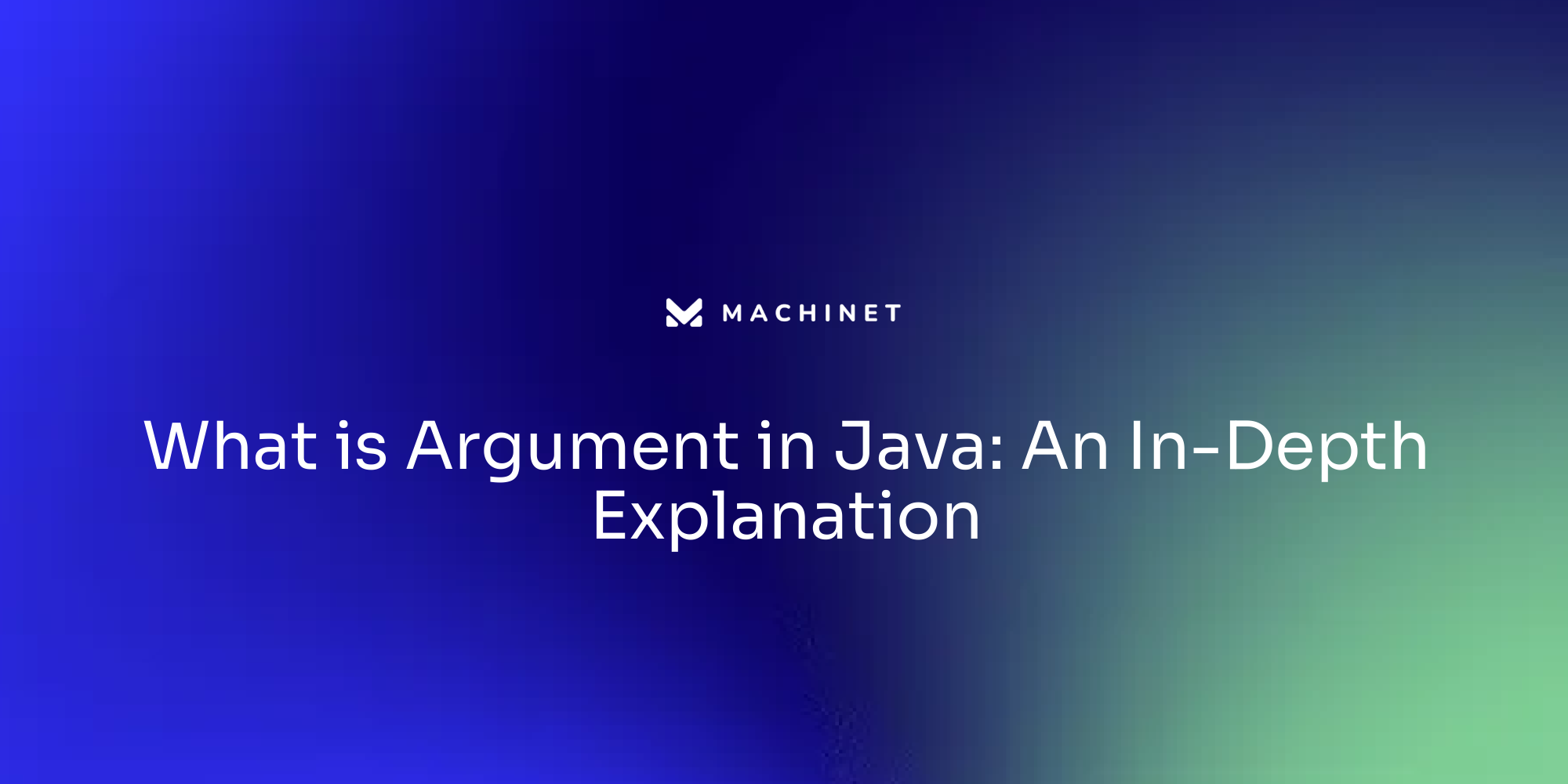
Introduction
In the realm of Java programming, distinguishing between 'argument' and 'parameter' is a fundamental yet often perplexing task for beginners. While parameters serve as placeholders in a method's signature, arguments are the actual values passed during method calls. This distinction is essential for writing clear, maintainable code.
Java supports different types of arguments, including primitive data types, objects, and varargs, each with unique behaviors. Understanding how arguments are passed—either by value or by reference—can significantly impact the efficiency and correctness of code execution. Common pitfalls such as confusing parameters with arguments, passing null references, and type mismatches can hinder program functionality.
Following best practices like using descriptive parameter names, limiting the number of parameters per method, and effectively employing varargs can enhance code readability and maintainability. Additionally, the capability to handle command line arguments offers Java programs an added layer of flexibility and dynamic behavior, making them more versatile in real-world applications.
Argument vs. Parameter: Understanding the Basics
In Java, 'argument' and 'parameter' are terms that often confuse beginners, yet they have distinct roles. A parameter is a variable defined in a function's signature, serving as a placeholder for the values the function will process. For example, in the function definition public void add(int a, int b)
, a
and b
are parameters.
When the method is called, the actual values passed to it, such as add(5, 10)
, are referred to as parameters. Here, 5 and 10 are the factors. Understanding this distinction is crucial for debugging and writing clear, maintainable code. Well-defined parameters and clear reasoning enhance code readability and facilitate smoother collaboration among team members.
java
public void add(int a, int b) {
// Method body: statements to perform the addition
}
In this example, a
and b
are the parameters, while add(5, 10)
would pass 5 and 10 as arguments to the method. This differentiation assists in understanding function calls and their expected inputs, making the debugging process more intuitive.
Types of Arguments in Java
Java supports several types of arguments, each with distinct behaviors:
-
Primitive Data Types: These include types like
int
,char
, andboolean
. When given to a function, a copy of the value is sent. This ensures that the original data remains unchanged, which enhances type safety and reduces the risk of unintended side effects. As noted by A N M Bazlur Rahman, a Java Champion, Java's type-checking mechanisms are designed to be intuitive and efficient, simplifying syntax and aligning closely with the language's principles. -
Entities: When entities are transmitted to functions, a reference to the entity is sent. This means that changes made to the object within the method will affect the original object. This behavior is fundamental in the programming language and is crucial for managing complex data structures and enabling sophisticated operations. According to DNAStack's Staff Software Developer, Java's handling of reference types fosters a more intuitive and efficient development experience.
-
Varargs: This feature enables you to provide a variable quantity of inputs of the same type to a function. Varargs simplify function calls when the count of parameters is unknown at compile-time, making the code more adaptable and easier to manage. This is especially beneficial in APIs where a function must manage an arbitrary number of inputs without compromising performance or readability.
Passing Arguments to Methods
Arguments can be passed to methods in Java in two primary ways:
-
By Value: This is the default method of passing arguments in Java. When a function is invoked, a copy of the variable's value is created, and any alterations made to the parameter within the function do not influence the original variable. This approach ensures that primitive data types, like
int
,float
, orchar
, are passed safely without altering the original values. -
By Reference: For entities, Java passes the reference to the entity rather than a copy of the entity itself. This indicates that any modifications made to the entity within the function will be shown in the original entity. 'This is especially helpful when dealing with intricate data arrangements or when a function needs to alter the entity given to it.'. For instance, updating the properties of an object or manipulating elements within a collection can be efficiently handled using this approach.
Common Mistakes When Passing Arguments
Several common mistakes can occur when passing arguments in Java:
-
Confusing Parameters and Arguments: Misunderstanding the distinction can lead to errors in method calls. Parameters are the variables outlined in the function definition, while arguments are the actual values provided to the function. As one expert notes, “Arguments are the specific values that you pass to a method’s parameters when calling it.”
-
Passing Null References: This can lead to NullPointerExceptions if not handled correctly. A NullPointerException happens when you attempt to utilize a variable that doesn’t refer to any actual entity in memory, like a blank sticky note. To prevent these, always make sure that objects are properly created before passing them as inputs. Best practices for preventing NullPointerExceptions include checking for null values and using Optional where appropriate.
-
Type Mismatch: Providing a value of an incompatible type can lead to compile-time errors. Java is a strongly-typed language, so the types of the arguments must match the types of the parameters. For example, if a function anticipates an integer, providing a string will lead to a compile-time error. This highlights the significance of comprehending the signature and ensuring compatibility of the data types.
Best Practices for Using Arguments in Java
To ensure effective use of arguments in Java, consider the following best practices:
- Use Descriptive Parameter Names: This enhances code readability and maintainability. For instance, remarks can clarify the intent of each parameter, making it evident what values are anticipated and what the function computes.
- Limit the Number of Parameters: Aim for approaches with a maximum of three parameters to simplify calls and reduce complexity. Overloading functions with parameters can lead to confusion and errors.
- Employ Varargs When Suitable: This can enhance function calls' clarity when handling several inputs. Varargs (variable-length input lists) enable functions to accept a variable number of values, enhancing flexibility.
Additionally, consider using comments judiciously to break down complex logic or algorithms. Comments can serve as reminders for future improvements or known issues but should not clutter the codebase.
Command Line Arguments in Java
Java programs can accept command line inputs, allowing users to provide information directly when executing the program. This feature is essential for enhancing flexibility and enabling dynamic behavior in applications. The inputs are passed as an array of Strings to the main method and can be accessed by iterating over the array and processing each input as needed. Notably, tools like Apache Commons CLI simplify the parsing of command line arguments, which is beneficial for various Apache projects, including Kafka, Maven, Ant, and Tomcat.
Conclusion
Understanding the distinction between arguments and parameters is crucial for any Java programmer. Parameters serve as placeholders within method signatures, while arguments are the actual values provided during method calls. This differentiation not only aids in debugging but also enhances code clarity and collaboration among developers.
Java offers various types of arguments, including primitive data types, objects, and varargs, each exhibiting unique behavior. Recognizing how arguments are passed—by value for primitives and by reference for objects—can significantly impact code efficiency and functionality. Additionally, being aware of common pitfalls such as confusing parameters with arguments, passing null references, and type mismatches can help avoid runtime errors.
Implementing best practices, such as using descriptive parameter names, limiting the number of parameters, and effectively utilizing varargs, can lead to cleaner, more maintainable code. Furthermore, the ability to handle command line arguments adds a layer of flexibility, making Java applications more dynamic and user-friendly.
In summary, grasping the nuances of arguments and parameters, along with adhering to best practices, empowers developers to write more robust and effective Java code. This foundational knowledge is essential for fostering a deeper understanding of Java programming and enhancing overall software development skills.
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.