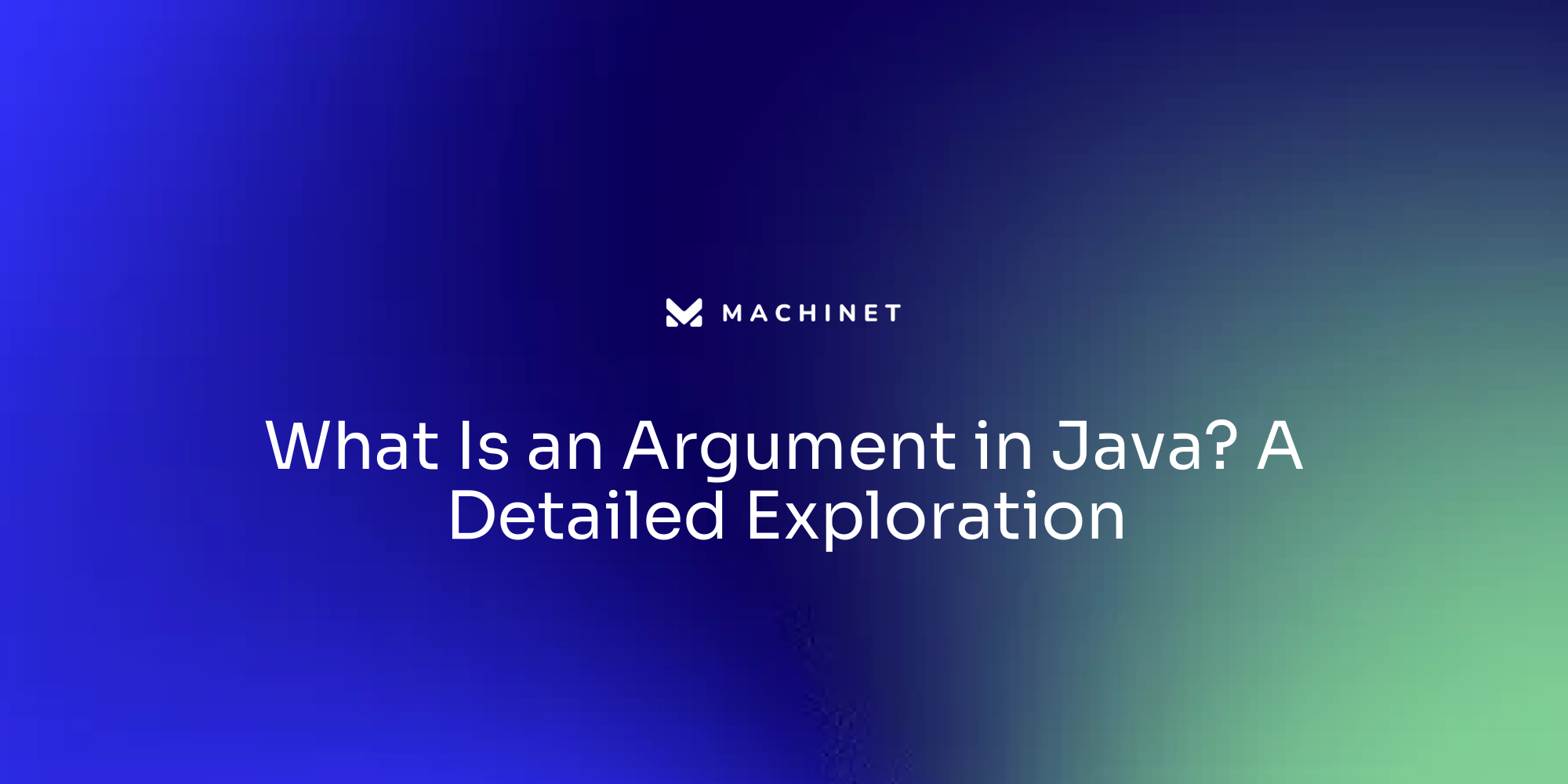
Introduction
In the world of Java programming, understanding how to work with arguments is fundamental to writing efficient and dynamic code. Arguments in Java are the values passed to methods during invocation, allowing these methods to process external data seamlessly. This concept is crucial for creating flexible and maintainable applications, particularly in domains like data science and artificial intelligence where adaptable and efficient code is essential.
Java offers a range of argument types, including primitive arguments, object arguments, and the versatile varargs. Each type serves different purposes, enhancing code flexibility and readability. Additionally, Java's pass-by-value mechanism for method arguments necessitates a clear understanding of how data is manipulated within methods, especially when distinguishing between primitive types and object references.
Common issues like IllegalArgumentException highlight the importance of validating method inputs to maintain application integrity. Effective handling of such exceptions, coupled with best practices like using descriptive parameter names and encapsulating related parameters, ensures the development of robust and maintainable code. Advanced techniques such as using builders and generics further enhance Java's capability to manage complex data structures efficiently, promoting type safety and reducing redundancy.
What Is an Argument in Java?
In the programming language, inputs are values provided to functions when they are called, allowing these functions to operate with external data and display dynamic behavior. These claims can be literals, variables, or expressions that correspond to the parameter types specified in the function's signature. Understanding this concept is essential for writing flexible and maintainable code, particularly in fields like data science and artificial intelligence, where efficient and adaptable code is paramount. According to recent analysis, array usage in programming often involves small sizes accessed by one or two classes, highlighting the importance of efficient data handling in dynamic programming environments.
Types of Arguments in Java
Java offers various kinds of parameters for functions, each serving distinct purposes and increasing the adaptability of your code.
-
Primitive Arguments: These include basic data types such as
int
,char
, andboolean
. 'They are passed by value, meaning any changes to the parameter within the function do not affect the original value.'. -
Object Arguments: Instances of classes or interfaces can be passed as arguments. This enables techniques to engage directly with the entities, offering a robust approach to handle and use intricate data arrangements. For instance, when passing an object of a class, the function can modify the object's state, which will be reflected outside the function.
-
Varargs (Variable Arguments): Introduced in version 5, varargs enable functions to receive zero or more inputs of a specified type. This feature improves flexibility by allowing you to provide a variable quantity of inputs without requiring you to overload the function several times. For example:
java
public void printNumbers(int... numbers) {
for (int number : numbers) {
System.out.println(number);
}
}
These argument types facilitate more readable, maintainable, and flexible code, aligning with Java’s goal of providing a robust and efficient development environment.
Passing Arguments in Java: Pass by Value vs. Pass by Reference
Java utilizes a pass-by-value mechanism for function arguments, meaning it transmits a copy of the argument's value to the function. For primitive types like int and float, this translates to copying the actual value. However, for entities, what is duplicated is the reference to the entity, not the entity itself. As a result, any modifications made to the object's characteristics within the function will influence the original object. On the other hand, reassigning the reference itself within the method does not impact the original reference outside the method. This distinction is crucial for understanding how data is manipulated within programming environments and ensures developers can predict the behavior of their code more accurately.
Common Issues with Arguments in Java: IllegalArgumentException
One of the common pitfalls in Java programming is the IllegalArgumentException. This exception happens when a function gets a parameter that is unsuitable or beyond the anticipated range. Acting as a safeguard, IllegalArgumentException guarantees that functions operate with valid data, preserving the integrity of the application.
When an IllegalArgumentException is thrown, it signals that the provided argument doesn't meet the method's requirements. This exception is part of a broader mechanism in the programming language that uses exceptions to handle unexpected errors gracefully. By invoking exceptions, developers can prevent the program from crashing and provide a structured response to erroneous situations. As noted by Sharat Chander, senior director at Oracle, Java's robustness largely comes from its community's contributions and the effective use of its error-handling mechanisms.
For instance, consider a method that expects a positive integer as input. If it receives a negative value, an IllegalArgumentException can be thrown to alert the developer or the user of the invalid input. This proactive approach helps in identifying and resolving issues promptly, ensuring that the software behaves as expected under various conditions.
Handling IllegalArgumentExceptions in Java
To effectively handle IllegalArgumentException, developers should validate inputs before processing them. This can be achieved using conditional statements to check for valid input values or by employing try-catch blocks to manage exceptions gracefully. Providing clear and informative error messages is crucial, as it aids in debugging and enhances code reliability. James Gosling, the creator of the programming language, highlights the importance of handling exceptions properly to maintain the integrity of the application. By ensuring that inputs are validated and exceptions are handled with informative messages, developers can avoid frequent traps and enhance the maintainability of their code.
Best Practices for Using Arguments in Java
When working with arguments in Java, it's essential to maintain clarity and readability. Using descriptive parameter names helps others understand the code's purpose quickly, facilitating collaboration and reducing the chances of errors. For instance, instead of using vague names like arg1
or val2
, opt for names that convey meaning, such as username
or transaction Amount
. This practice aligns with the guideline that code is read more often than it's written, making future modifications smoother.
Furthermore, steer clear of overloading functions with too many parameters. Excessive parameters can lead to confusion and increased complexity. Instead, consider encapsulating related parameters within entities or data structures. This not only simplifies function signatures but also enhances maintainability. For instance, creating a UserDetails
structure to hold multiple user-related parameters can make the code more intuitive and easier to manage.
Moreover, adding comments for clarity can be beneficial, especially when dealing with complex logic. However, it's crucial to use comments judiciously. Over-commenting can clutter the codebase without adding significant value. A well-placed comment explaining the purpose of a function or the role of a parameter can make a significant difference, particularly in collaborative environments where multiple developers work on the same codebase.
In summary, clear and descriptive parameter names, thoughtful use of entities or data structures, and strategic commenting are key practices for enhancing the readability and maintainability of Java code.
Advanced Topics: Using Builders and Generics with Arguments in Java
Builders provide an organized approach for constructing items gradually, making the process more intuitive and less prone to mistakes. This pattern is particularly beneficial when dealing with objects that have multiple optional parameters, as it separates the construction process from the object's representation. For example, in a financial service application, using the Builder Pattern ensures that transaction details are immutable once recorded, enhancing security and reliability.
Generics in Java allow you to write methods and classes that can operate on various data types while maintaining type safety. This flexibility is essential for creating reusable code components. For instance, in a healthcare data management system, generics enable a unified API to manage different types of medical records without needing separate codebases. By leveraging generics, developers can write more versatile and maintainable code, reducing redundancy and potential errors.
Conclusion
Understanding how to effectively utilize arguments in Java is essential for developing robust and dynamic applications. The article highlighted the significance of arguments as values passed to methods, facilitating interaction with external data. With types such as primitive, object, and varargs, Java provides flexibility that enhances code readability and maintainability, particularly in complex programming scenarios.
The distinction between pass-by-value and pass-by-reference was emphasized, clarifying how data manipulation occurs within methods. This understanding is crucial for developers to predict the behavior of their code accurately. Additionally, the article examined common issues like IllegalArgumentException, underscoring the importance of input validation to maintain application integrity.
Best practices for handling arguments were discussed, advocating for descriptive parameter names and the encapsulation of related parameters to simplify method signatures. Advanced techniques such as the Builder Pattern and the use of generics were also explored, showcasing ways to create more intuitive and type-safe code. By adhering to these principles, developers can enhance the flexibility, readability, and overall quality of their Java applications.
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.