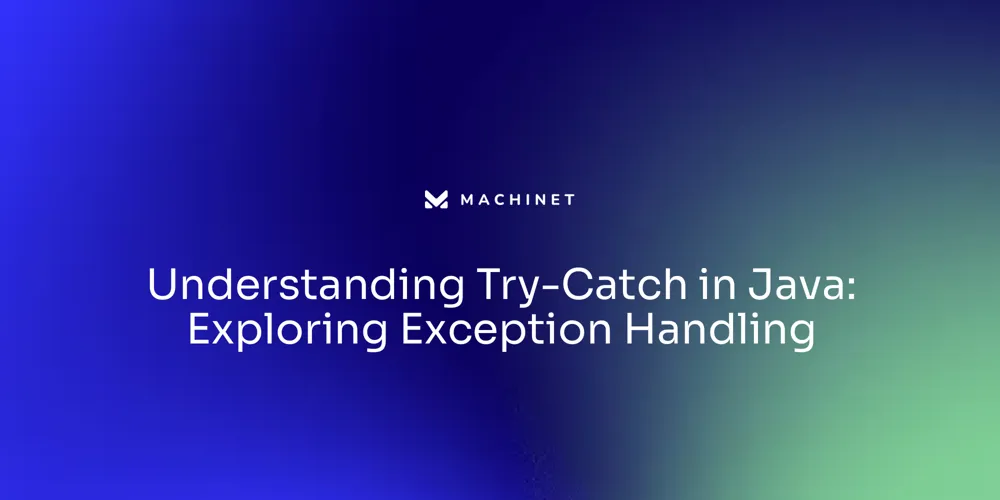
Table of Contents
- What is Exception Handling?
- Understanding Try-Catch Blocks
- Types of Exceptions
- Best Practices for Exception Handling
Introduction
Exception handling is a crucial aspect of Java programming, providing a structured approach to managing runtime errors and ensuring the resilience of applications. In this article, we will explore the fundamentals of exception handling in Java, including the different types of exceptions, the use of try-catch blocks, and best practices for effective error management. Whether you are a beginner or an experienced Java developer, this article will equip you with the knowledge and skills to create robust and fault-tolerant applications.
What is Exception Handling?
Java's exception handling is a cornerstone of its robustness, providing a structured way to detect and manage runtime errors, ensuring applications run smoothly. In Java, exceptions are events that interrupt the normal flow of execution by 'throwing' an object that encapsulates the error details.
This thrown object can be 'caught' and handled gracefully to prevent abrupt program termination. There are primarily two categories of exceptions in Java: checked and unchecked.
Checked exceptions must be declared in a method's signature or handled within a method, while unchecked exceptions, like RuntimeException
, can occur without such declarations. For instance, a [NumberFormatException](https://reflectoring.io/do-not-use-checked-exceptions/)
may arise if Integer.parseInt
is given an improper format.
Although it's a runtime exception, developers can choose to handle it explicitly to prevent potential crashes. Exception handling enhances not only the error management but also the reliability and robustness of the application.
As stated, Java is unique in its implementation of checked exceptions, a concept introduced at its inception and still debated today. While some argue against their use, others praise them for enforcing error handling and improving code reliability. Errors can stem from various sources, such as incorrect user input formats, resource limitations, external system failures, or programming errors. Effective exception handling in Java allows developers to anticipate these issues, providing a safety net for operations like reading data from a file. By handling exceptions like file not found, lack of permissions, or unreadable content, Java programs can maintain stability and provide a better user experience. In conclusion, proper exception handling is not just about catching errors; it's about creating a resilient ecosystem for your Java applications, enabling them to withstand and recover from the unexpected.
Understanding Try-Catch Blocks
Exception handling is an integral feature of Java, providing a structured pathway for managing runtime errors to maintain the seamless execution of an application. When an event occurs that interrupts the normal flow of instructions, the Java runtime system halts the current method and creates an exception object that encapsulates details about the issue.
This object is then relayed to an appropriate catch block that is equipped to address the exception. In Java, exceptions are categorized into two primary groups: checked and unchecked exceptions.
Checked exceptions must be declared in a method or constructor's throws clause if they can be thrown by the execution of the method or constructor and propagate outside the method or constructor boundary. Unchecked exceptions, on the other hand, include runtime exceptions and errors, which are not subject to the Catch or Specify Requirement.
The throw keyword is used to explicitly signal the occurrence of an exception, effectively invoking the exception handling mechanism within the Java Virtual Machine (JVM). Proper exception handling is crucial for crafting robust and fault-tolerant Java applications. It enhances the reliability of the software by ensuring that potential runtime errors do not lead to abrupt termination. According to recent statistics, the adoption rate of Java 17, which contains enhanced features for exception handling, has seen a significant increase, with 35% of applications using it in 2023—a nearly 300% growth rate in one year. This data underscores the importance placed by developers on utilizing modern Java versions that offer advanced capabilities in error management.
Types of Exceptions
In Java, exceptions serve as a signal that an unexpected event has occurred, interrupting the flow of program execution. To communicate such events, Java provides the throw
keyword, which when used, instantiates a new exception object encapsulating details about the occurrence.
This object includes the exception type and a message, offering a clearer understanding of the issue at hand. The Java runtime halts the current method’s execution upon an exception and forwards the exception object to a catch
block equipped to handle it.
Java distinguishes between two principal categories of exceptions: checked exceptions, which are scrutinized at compile-time, and unchecked exceptions, which are checked at runtime. Checked exceptions are unique to Java, conceived with the intention of enhancing robustness, particularly in networked environments.
They compel developers to confront potential errors, thereby fostering more fault-tolerant applications. However, checked exceptions have been a polarizing feature since their inception in 1996; while they were groundbreaking, they are now often seen as a hindrance, particularly in the context of functional programming. An example of unchecked exception is the [NumberFormatException](https://dev.to/codenameone/everything-bad-in-java-is-good-for-you-3fhd/)
, which may be thrown by Integer.parseInt
. This type of exception does not mandate handling at compile-time, thus the following code compiles without issues:
java
int number = Integer.parseInt("123");
Conversely, developers may opt to handle it explicitly:
java
try {
int number = Integer.parseInt("123");
} catch (NumberFormatException e) {
// Handle exception
}
It's important to note that exceptions not only aid in addressing runtime errors but also play a crucial role in ensuring the robustness of Java applications.
Best Practices for Exception Handling
Java's exception handling is a cornerstone of its robust programming capabilities, providing a structured pathway for managing runtime errors and ensuring the resilience of applications. At the heart of this system are 'exceptions,' events that interrupt the program's usual sequence of operations.
When an event occurs that a program doesn't anticipate or can't process, Java creates an exception object containing details about the issue and halts the current method's execution. This object is then passed to a 'catch block' equipped to handle the situation.
To effectively communicate an issue within your code to the Java runtime, one must utilize the 'throw' keyword, which instantiates the exception handling machinery of the Java Virtual Machine (JVM). This initiated object not only carries the exception type but also includes a message detailing the occurrence for better clarity.
A stack trace often accompanies an exception, pinpointing the exact code location where the problem surfaced, serving as a guide for developers to trace back to the issue's origin. Despite the debate over the use of checked exceptions in Java—a feature unique to the language since its inception in 1996—understanding how to throw and handle both checked and unchecked exceptions remains crucial. Such knowledge enhances code readability, reliability, and maintainability. As developers navigate through different types of exceptions and learn to create custom ones, they elevate their proficiency in Java, crafting more fault-tolerant applications. Adherence to the best practices is not just a recommendation but a stepping stone towards becoming a more adept Java developer.
Conclusion
In conclusion, proper exception handling is crucial for creating robust and fault-tolerant Java applications. By using try-catch blocks, developers can effectively manage runtime errors and prevent abrupt program termination. Java distinguishes between checked exceptions, which must be declared or handled, and unchecked exceptions, which can occur without such declarations.
Exception handling not only improves error management but also enhances the reliability and resilience of the application. Understanding the different types of exceptions in Java, such as checked and unchecked exceptions, allows developers to anticipate potential issues and handle them appropriately. While checked exceptions have been a polarizing feature since their introduction, they enforce error handling and contribute to the overall robustness of the code.
On the other hand, unchecked exceptions provide flexibility but should still be handled to ensure a stable user experience. To effectively handle exceptions in Java, it is important to follow best practices. This includes using the throw keyword to signal the occurrence of an exception and providing clear messages that describe the issue at hand.
Additionally, understanding stack traces can help developers trace back to the origin of the problem and improve code readability and maintainability. In summary, mastering exception handling in Java is essential for creating resilient applications. By utilizing try-catch blocks, understanding different types of exceptions, and following best practices, developers can enhance their skills and create fault-tolerant software that can withstand unexpected events.
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.