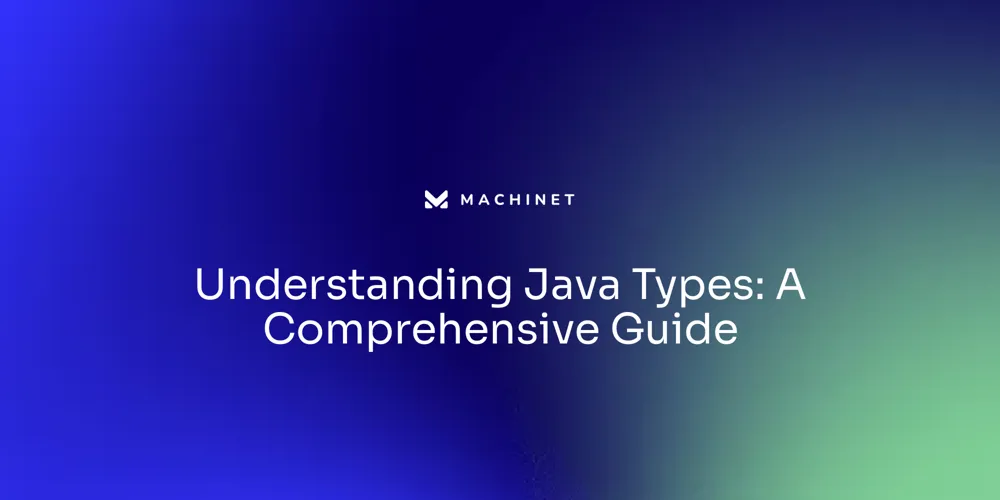
Table of Contents
- Java Data Types
- Primitive Data Types
- Reference Types
- Type Inference
Introduction
Java's strong typing system is a cornerstone of its reliability and performance. Every variable in Java must be associated with a specific data type, which governs the kind of data it can hold and the operations that can be performed on it.
This article will explore the two main categories of data types in Java: primitive and reference types. It will delve into the characteristics, usage, and importance of each type, highlighting how a clear understanding of these types can enhance the readability and maintainability of code. So, let's dive into the world of Java data types and uncover their significance in the programming realm.
Java Data Types
Java's strong typing system is a cornerstone of its reliability and performance. Every variable in Java must be associated with a specific data type, which governs the kind of data it can hold and the operations that can be performed on it.
There are two main categories of data types in Java: primitive and reference types. Primitive data types are the basic types of data built into the language.
They include int, char, byte, and others, each of which has a fixed size and stores its value directly in memory. These types represent single values and are not composed of other objects.
They are efficient both in terms of memory usage and execution speed, being atomic and non-divisible. For example, an int in Java typically occupies 32 bits of memory and can store values from -2,147,483,648 to 2,147,483,647.
In contrast, reference data types, also known as non-primitive or derived types, include classes, arrays, and interfaces. These types store references to the memory locations where the actual objects are kept.
This means that when you manipulate an object of a reference type, you're working with a pointer to the data rather than the data itself. Reference types default to null if not explicitly initialized, indicating the absence of an object.
Understanding the distinction between these two data types is crucial. Primitive types are passed by value, meaning that a copy of the actual data is made when used in method calls. On the other hand, reference types are passed by reference value, meaning that a copy of the reference is made, but the object it points to is the same. To illustrate, calling a method with an object as a parameter doesn't create a new object but allows access to the original object through its reference. As Uncle Bob, the author of 'Clean Code,' puts it, "We are constantly reading old code as part of the effort to write new code. Therefore, making it easy to read makes it easier to write." This principle is particularly relevant when dealing with Java's data types. A clear understanding of the types and how they are used can significantly enhance the readability and maintainability of code.
Primitive Data Types
Java's data types are categorized into two main types: primitive and reference. Primitive data types, which include boolean, byte, short, int, long, float, double, and char, are the basic data types that store values directly in memory. They have predefined sizes and value ranges; for instance, an int in Java is a 32-bit signed integer.
These types are non-nullable and have default values if not initialized, ensuring type safety and efficiency in handling data. Reference data types, conversely, store memory addresses pointing to their objects, which can be null if not initialized. The distinction between these data types is crucial for Java developers to understand, as they influence how data is stored, accessed, and manipulated within applications.
In the context of Java's evolution since its inception in 1995, understanding these data types is more relevant than ever. With Java's strong typing system, every variable needs a declared type, accommodating a variety of data and operations. The language continues to adapt, maintaining its foundational elements while integrating new features to meet modern software development needs.
Reference Types
Java's type system is robust, ensuring that every variable is defined with a type that dictates the kind of data it can hold and the operations that can be performed. Among Java's data types, reference types are crucial for object-oriented programming, as they store references to the complex structures rather than the actual data itself.
These types include classes, interfaces, arrays, and enums, each serving a unique purpose within the language's ecosystem. - Classes provide the templates for object creation, encapsulating related data and behavior into a single entity.
Objects are instances of these classes, and their properties and methods define their state and functionality. - Interfaces establish contracts that classes can implement, ensuring a consistent API and enabling polymorphism - a core concept in Java's inheritance model.
- Arrays offer a means to store multiple items of the same type, facilitating easy access and manipulation of collections of data. - Enums represent a group of constants, improving code readability and type safety by providing a restricted set of possible values.
Understanding the distinction between reference types and primitive types is essential. Primitive types hold the actual value, while reference types point to an object's location in memory. This is a critical aspect of Java's parameter passing mechanism, which operates on a 'pass-by-value' basis. When a method is called with a primitive type, its value is copied, leaving the original unaffected. Conversely, passing a reference type means copying the reference, not the object, allowing modifications to the object without altering the reference itself. This subtle yet vital difference underscores the importance of clear communication regarding Java's handling of data types and the mutability of objects passed to methods.
Type Inference
Java's evolution has led to the introduction of type inference in Java 10, a feature that simplifies the developer's job by allowing the compiler to deduce a variable's type from its initializer. This innovation eliminates the need for explicit type declarations, streamlining the code to be more succinct. The use of type inference is not confined to local variables alone but extends to lambda expressions and method references as well, contributing to enhanced code readability and a reduction in repetitive code structures.
Reflecting on the mathematical foundation of type inference, it's akin to formulating a list of ordered pairs, each comprising a type and an associated name, which ensures clarity and prevents confusion between components. For instance, a variable of some_type could be represented as (int, "val1"), distinctly naming each integer component. The Java ecosystem continues to innovate with features such as Switch Expressions introduced in Java 14 and the revolutionary Pattern Matching for Switch in Java 21.
Pattern Matching, in particular, facilitates a more intuitive way of extracting and operating on data, by matching values against patterns with embedded variables and conditions. This not only makes the code more legible but also aligns with the systematic approach of solving type equations, which is now a simpler process akin to high school algebra. Adding to Java's dynamic landscape are the anticipated features in JDK 22, such as the simplification of unnamed classes, which now behave like normal top-level classes without necessitating additional tooling or runtime support.
Moreover, the Jakarta Data 1.0.0, set for inclusion in Jakarta EE 11, and updates like Hibernate Reactive 2.0.3.Final, further demonstrate the ongoing enhancements in the Java world. Java remains a dominant force in the programming realm, securing a solid top three spot in popularity despite the emergence of modern languages. Its widespread use in frameworks like Spring maintains its relevance in top-tier companies, showcasing its enduring significance in the tech industry.
Conclusion
In conclusion, Java's strong typing system with its two main categories of data types, primitive and reference types, plays a crucial role in the reliability and performance of the language. Primitive data types are built-in and store values directly in memory, while reference types store references to memory locations where objects are kept.
Understanding the distinction between these types is essential for developers as it affects how data is stored, accessed, and manipulated within applications. Java's type system also includes reference types such as classes, interfaces, arrays, and enums.
These types serve different purposes in object-oriented programming and contribute to code readability and maintainability. The introduction of type inference in Java 10 simplifies the developer's job by allowing the compiler to deduce variable types from initializers.
This feature enhances code readability and reduces repetitive code structures. Java continues to evolve with new features like Switch Expressions and Pattern Matching for Switch, making code more intuitive and legible. The ongoing enhancements in the Java ecosystem further demonstrate its enduring significance in the tech industry. Overall, a clear understanding of Java's data types enhances the readability and maintainability of code, making it easier for developers to read and write high-quality software.
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.