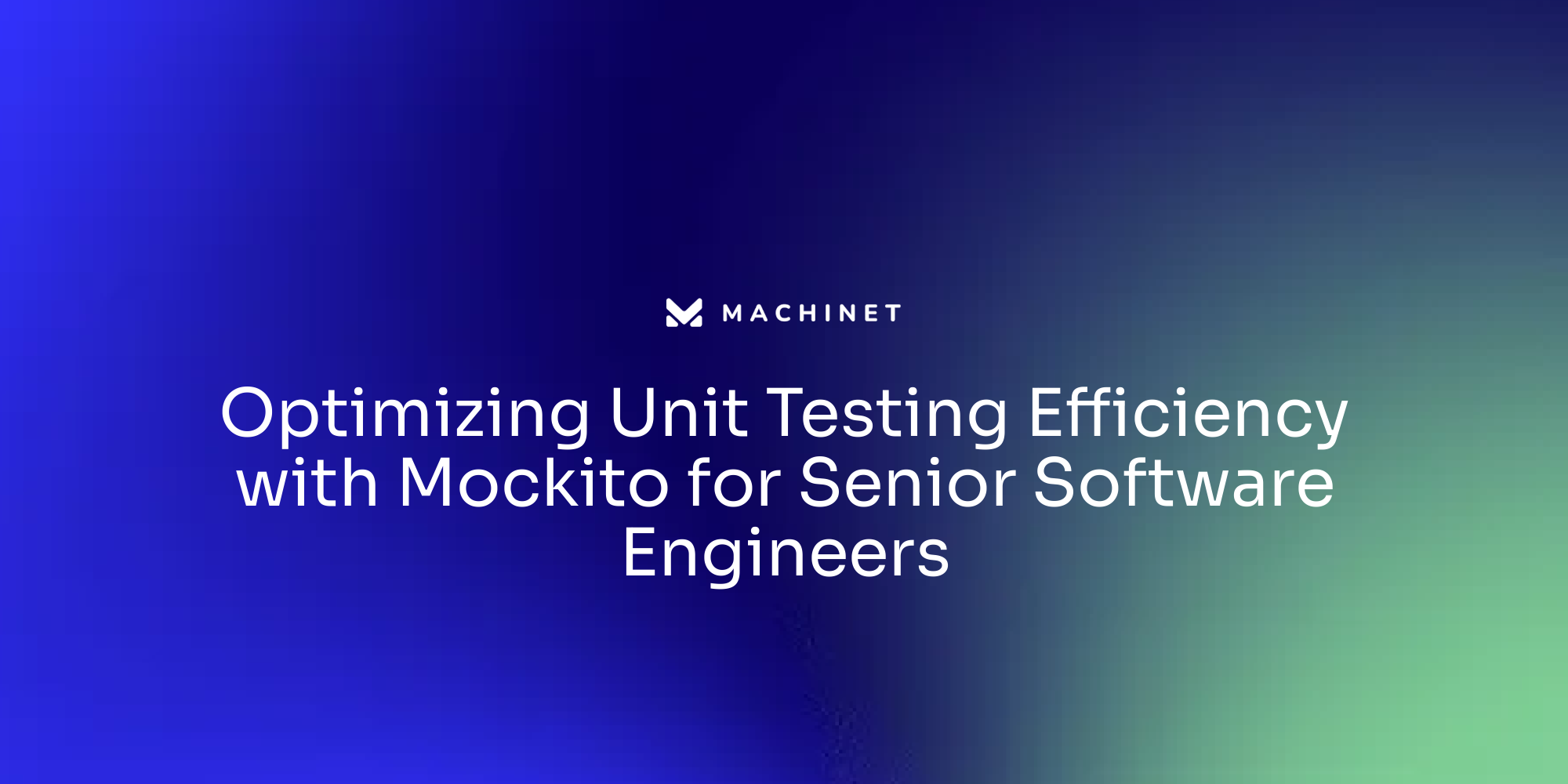
Table of Contents
- Adding Mockito to a Project: A Step-by-Step Guide
- Configuring the Return Values of Methods Calls on Mock Objects with Mockito
- Advanced Techniques: Mocking Final Classes and Static Methods Using Mockito
- Utilizing InjectMocks for Dependency Injection via Mockito in Complex Projects
- Verifying the Calls on Mock Objects: Ensuring Test Accuracy with Mockito
- Using Spy from Mockito: Enhancing Test Coverage and Flexibility
- Capturing the Arguments in Unit Tests with Mockito: A Deep Dive
- Writing a New Mockito Test for ConfigureThreadingUtil: A Practical Example
Introduction
The process of unit testing is crucial for ensuring the quality and reliability of software applications. Mockito, a popular Java testing framework, provides powerful tools and features that can enhance the effectiveness of unit tests. From mocking dependencies and controlling method behavior to verifying method calls and capturing arguments, Mockito offers a comprehensive solution for testing complex projects.
In this article, we will explore various aspects of Mockito and how it can be utilized to improve unit testing. We will discuss topics such as adding Mockito to a project, configuring return values of method calls on mock objects, mocking final classes and static methods, utilizing @InjectMocks
for dependency injection, verifying method calls on mock objects, using spies to enhance test coverage and flexibility, and capturing arguments in unit tests. By understanding these advanced techniques and best practices, software engineers can write more effective and reliable unit tests using Mockito. So let's dive in and explore the power of Mockito in unit testing!
1. Adding Mockito to a Project: A Step-by-Step Guide
To utilize Mockito in your Java project, the initial step is to add it as a dependency.
For Maven users, this can be achieved by modifying the pom.xml file to contain the subsequent lines:
Try Machinet for faster code generation!
xml
<dependencies>
<dependency>
<groupId>org.mockito</groupId>
<artifactId>mockito-core</artifactId>
<version>3.11.2</version>
<scope>test</scope>
</dependency>
</dependencies>
Remember to replace 3.11.2
with the desired version of Mockito. Specifying the scope as test
ensures that the Mockito dependency is utilized only during testing and not included in the final build of your project.
For Gradle users, the build.gradle file should be edited to incorporate these lines:
groovy
dependencies {
testImplementation 'org.mockito:mockito-core:3.11.2'
}
This will add the Mockito library to your project's test scope, enabling you to use it for unit testing.
After the inclusion of the dependency, Mockito can be imported into your test classes using import org.mockito.Mockito;
.
Boost your unit testing with Machinet's AI-powered code generation!
The addition of Mockito as a dependency is crucial for mocking static methods and constructors, which can sometimes be a requirement in the process of writing tests. Mockito's static constructor mocking feature is a handy alternative to the introduction of layers of indirection like the strategy or factory pattern.
Mockito's mockStatic
method is employed to mock static methods, and the behavior of the mocked static method is altered only within the scope of the try
block. Examples of this include mocking static methods on the Paths
class and the Instant
class.
A similar pattern is followed when mocking constructors. An example of this would be mocking the Random
constructor. While static and constructor mocking can be powerful tools, they should be used judiciously.
Additionally, the Mockito testing framework is covered in depth in the book "Mockito Made Clear," which is currently in beta from Pragmatic Programmers. This book includes access to code examples in a GitHub repository, which includes a Gradle build file. This build file includes dependencies for various libraries, such as JUnit 5, Mockito, AssertJ, Retrofit, Jackson, and Gson. It also includes settings for using Java 11 features, specifically the HttpClient class, while still being compatible with Java 8.
The dependencies block in the build file includes the necessary dependencies for JUnit 5, Mockito, AssertJ, Retrofit, Jackson, and Gson. The build file also includes a test block that specifies the use of JUnit 5 for tests and running them in parallel using multiple threads
2. Configuring the Return Values of Methods Calls on Mock Objects with Mockito
Mockito, a popular testing framework in Java, offers an assortment of tools for managing mock objects' behavior. These mock objects are often utilized to simulate the behavior of complex, real objects during testing. One of the key features of Mockito is the ability to dictate the return values of method calls on these mock objects. This is achieved via the when()
method, which is part of the Mockito API.
Here's a rudimentary example demonstrating its use:
java
Mockito.when(mockObject.method()).thenReturn(value);
In the above code snippet, mockObject.method()
represents the method call we want to control, and value
is the designated return value for this method when invoked. This mechanism, while simple, provides substantial control over our mock objects, enhancing the reliability and predictability of our tests.
This method is particularly effective when testing classes with external dependencies. By employing Mockito to mock these dependencies, we can isolate the class under test, ensuring our tests remain unaffected by the behavior of external systems.
Additionally, Mockito's ability to record interactions with mock objects also enables us to implement behavior testing. This allows us to confirm that our code interacts with the mock objects as expected, further bolstering the robustness of our tests.
However, it's essential to note that while Mockito offers a range of methods for creating mock objects (such as the @Mock
annotation, the mock()
method, and the spy()
method), private methods remain invisible for tests and hence cannot be mocked.
The ability to define return values of method calls on mock objects is a powerful feature of Mockito, making it an indispensable tool for writing effective and reliable unit tests.
To specify the return value of a method call on the mock object when invoked with specific arguments, the when
method is used. This helps in effectively stubbing the behavior of the mock object and controlling its return when certain methods are called. For example:
java
when(mockObject.methodName()).thenReturn(expectedValue);
In this syntax, mockObject
is the object on which the method is called, methodName
is the name of the method to stub, and expectedValue
is the value that the method should return when called.
The when
method is used to specify the behavior of a mocked object when a certain method is called. You can use it to define the return value of the method call. Here is another example:
```java // Create a mock object of the desired class MyClass myMock = Mockito.mock(MyClass.class);
// Specify the return value for a method call using the when method Mockito.when(myMock.myMethod()).thenReturn("mocked value");
// Now, when the myMethod() method is called on the myMock object, it will return "mocked value" ```
In the example above, MyClass
is the class whose method you want to mock, and myMethod()
is the method you want to mock. The thenReturn
method is used to specify the return value of the method call. In this case, it is "mocked value".
Using the when
method in Mockito allows you to control the behavior of mocked objects during unit testing, making it easier to test specific scenarios and simulate different outcomes
3. Advanced Techniques: Mocking Final Classes and Static Methods Using Mockito
Mockito, a Java framework widely adopted for its robust unit testing and mocking capabilities, provides a rich feature set that includes mocking of interfaces, abstract classes, and standard classes. However, a limitation of Mockito 3 is that it doesn't support the mocking of final classes and methods by default. To overcome this, Mockito provides an extension known as "mock maker".
To enable the mock maker extension, you have two options: create a configuration file or use Mockito's inline artifact. If you opt for the first method, you will need to create a configuration file named org.mockito.plugins.MockMaker
in the resources/mockito/extensions
directory. This file is used to configure the mock maker implementation, specifying the fully qualified class name of the mock maker implementation that Mockito should use.
java
// File: src/test/resources/mockito-extensions/org.mockito.plugins.MockMaker
mock-maker-inline
The second method, Mockito's inline artifact, provides a more convenient alternative. This artifact comes pre-configured and does not require a separate configuration file. It leverages the Java Instrumentation API and subclassing to generate mocks for final types and methods. To use Mockito-inline, you can add the following dependency to your project:
xml
<dependency>
<groupId>org.mockito</groupId>
<artifactId>mockito-inline</artifactId>
<version>2.x.x</version>
</dependency>
Once the dependency is added, Mockito-inline takes care of the necessary bytecode manipulation, allowing you to mock final classes and methods as usual. It's important to note that Mockito-inline only works with Mockito 2.x and above.
Mockito also supports a variety of features such as verifying interactions, setting up stubbing, capturing arguments, and facilitating behavior-driven development. It allows spying on objects, resetting mocks, and mocking with argument matchers.
For example, Mockito 3.4.0 introduced the MockedStatic
class to enable the mocking of static methods. Here's how you can use it:
java
try (MockedStatic<ClassName> theMock = Mockito.mockStatic(ClassName.class)) {
theMock.when(ClassName::method).thenReturn(value);
// Your test code here
}
In this example, ClassName::method
is the static method being mocked, and value
is the return value you wish to stub.
While Mockito is a powerful testing tool, it's worth noting that it has some limitations when it comes to spying on objects. Specifically, it's unable to spy on final classes or static methods. Despite these limitations, Mockito is commonly used alongside JUnit and Spring Boot for unit testing in Java projects, providing a comprehensive testing environment
4. Utilizing InjectMocks for Dependency Injection via Mockito in Complex Projects
The Mockito framework, with its @InjectMocks
annotation, serves as a powerful tool for Java unit testing, especially when working on complex software projects with numerous dependencies.
The @InjectMocks
annotation allows automatic injection of mock or spy fields into the object under test, simplifying the process of preparing your test environment.
Let's consider a simplified example:
```java @Mock DependencyClass dependency;
@InjectMocks TestClass testClass;
@Before public void setup() { MockitoAnnotations.initMocks(this); } ```
Here, DependencyClass
is a dependency of TestClass
. The @Mock
annotation creates a mock instance of DependencyClass
, which @InjectMocks
then injects into TestClass
. The setup()
method initializes these mocks before each test.
Mockito's injection happens via one of three methods: constructor-based, setter methods-based, or field-based injection. It first attempts constructor-based injection if a constructor exists. If not, it tries setter methods-based injection. Finally, if neither constructors nor setter methods are available, Mockito defaults to field-based injection, injecting the dependencies directly into the fields.
If there's only one matching mock object, Mockito injects it. However, if there are multiple mock objects of the same class, Mockito uses the mock object's name to identify and inject the dependencies.
Beyond instance method mocking, Mockito supports static methods and constructors mocking as well, using mockStatic
and mockConstruction
methods respectively. It's important to use these powerful tools responsibly to avoid overcomplicating your tests.
To create a Mockito @InjectMocks
example with multiple dependencies, ensure Mockito and JUnit dependencies are added to your project. Annotate the class you want to test with @RunWith(MockitoJUnitRunner.class)
, allowing Mockito to initialize the mocks and inject them into the class under test. Mock objects for the dependencies are created and annotated with @Mock
. The class under test is annotated with @InjectMocks
, and Mockito will automatically inject the mock objects into the class.
In terms of best practices, @InjectMocks
should be used judiciously. It's generally preferable to manually inject dependencies using constructor injection or setter methods. Ensure all the dependencies required by the class being tested are properly initialized before invoking any methods. In integration tests, it's often better to use real instances of dependencies rather than mocks. Keep tests focused and independent, focusing on testing the expected behavior and interactions with the injected dependencies.
Remember, Mockito's @InjectMocks
annotation can greatly enhance testing capabilities, but it should always be used responsibly and judiciously
5. Verifying the Calls on Mock Objects: Ensuring Test Accuracy with Mockito
The core of Mockito unit testing lies in the validation of certain method calls on your mock objects.
The verify()
method is an essential tool for this task. The code snippet below serves as an example:
java
Mockito.verify(mockObject).method();
This line verifies that method()
was invoked on mockObject
. If this condition is not satisfied, the test will not pass. The times()
function allows you to specify the number of times a method should have been called for the test to pass. Here's an example:
java
Mockito.verify(mockObject, Mockito.times(2)).method();
This line ensures that method()
was invoked exactly two times on mockObject
.
In a real-world scenario, such as an e-commerce application, you might want to verify that customer data is stored in the session following a successful login. In this case, you would use a mock data access object (DAO) and a service to interact with the DAO. The login method would validate user credentials and return the appropriate customer object. For the purpose of this guide, we will keep things simple and avoid using frameworks like Spring.
The test code utilizes a spy instead of a mock, represented by the spy annotation. A method named "testsuccessfullogin" is included in the test code, which performs a login using arbitrary credentials. The line of code that employs the "verify" method is responsible for confirming if the "saveinsession" method was called. The "verify" method accepts the spy or mock object as a parameter and checks if a specific method got called. The example code indicates that the "saveinsession" method should be invoked with any customer object.
Beyond verifying that a specific method was called, the verify methods in Mockito can also be used to test the number of method invocations, verify the order of method invocations, confirm that a method is called a specific number of times, at least once, or at most a certain number of times. It can also verify that a method is never called. Other verify methods such as verifyNoMoreInteractions
and verifyZeroInteractions
can be used to confirm that all interactions with a mocked object are verified. The inorder
method can be used to verify the order of method invocations. These methods are critical in ensuring that the expected behavior is being tested and that all necessary method invocations are being made.
With these tools at your disposal, you are encouraged to experiment with the code and incorporate Mockito into your own unit tests. The verify()
method is a crucial component of Mockito, allowing you to create assertions on the behavior of the mock object during test execution. By using verify()
, you can ensure that the desired behavior of the code under test is being met. It is a powerful method in Mockito that lets you verify if a specific method has been called on a mock object, providing various options for checking the number of times and arguments of the method call. This is just a simple example of how the verify()
method can be used in Mockito for verifying method calls. Mockito provides various options and features for verifying method invocations with different arguments, number of invocations, and more
6. Using Spy from Mockito: Enhancing Test Coverage and Flexibility
As an experienced software engineer, you might find yourself dealing with the challenge of verifying method calls and stubbing methods during unit testing. Mockito, a popular Java testing framework, offers a powerful tool to address this: the spy()
function. This function allows you to create a 'spy' of a real object, retaining the original behavior of the object while also permitting the stubbing of certain methods and the verification of method calls.
To illustrate, let's consider a class Invoice
with two dependencies: TaxCalculation
and ExportInvoiceLibrary
. The Invoice
class contains a processInvoice
method that computes the total value and then calls the sendToGovernment
method from the ExportInvoiceLibrary
dependency. To verify that the sendToGovernment
method is being called correctly, we can create a spy of the ExportInvoiceLibrary
and use it to monitor the sendToGovernment
method.
java
ExportInvoiceLibrary exportInvoiceLibrary = new ExportInvoiceLibrary();
ExportInvoiceLibrary spyExportInvoiceLibrary = Mockito.spy(exportInvoiceLibrary);
Here, spyExportInvoiceLibrary
is a spy of exportInvoiceLibrary
. All method calls on spyExportInvoiceLibrary
will be delegated to exportInvoiceLibrary
, but we can also verify calls on spyExportInvoiceLibrary
.
This is how you can create a spy object using Mockito's spy()
function:
```java import static org.mockito.Mockito.*;
// Create an instance of the object you want to spy on MyObject myObject = new MyObject();
// Create a spy object using the spy() function MyObject spyObject = spy(myObject);
// Use the spy object in your test cases // You can mock specific methods of the spy object using when().thenReturn() or doReturn().when() syntax
// For example, mock the return value of a method when(spyObject.someMethod()).thenReturn("mocked value");
// Call the method on the spy object String result = spyObject.someMethod();
// Assert the result assertEquals("mocked value", result);
// Verify that the method was called on the spy object
verify(spyObject).someMethod();
``
By using the
spy()` function in Mockito, you can create a spy object that allows you to mock specific methods while still using the original implementation for other methods. This can be useful in situations where you want to test the interaction between different methods of an object.
It's important to understand the distinction between a spy and a mock in Mockito. Spying on an object means creating a real object and then intercepting some of its methods to track their invocations. This can be useful when you want to partially mock an object and keep the real behavior of certain methods. On the other hand, mocking an object means creating a dummy object that simulates the behavior of the real object. You can define the expected behavior of the mocked object's methods and verify that they are called correctly during testing.
In conclusion, Mockito's spy()
function is a versatile tool that offers the capability to create a 'spy' of a real object, allowing for method stubbing and call verification. This functionality can be particularly useful in unit testing scenarios, where it's necessary to verify the correct interaction between different parts of the codebase
7. Capturing the Arguments in Unit Tests with Mockito: A Deep Dive
As we delve into the realm of advanced software solutions, unit tests frequently encompass methods that take arguments. Occasionally, these arguments need to be captured for further assertions to fortify the software's resilience. Mockito, a freely available testing framework, provides the ArgumentCaptor
class to address this need.
Consider the following code snippet as an example:
java
ArgumentCaptor<String> captor = ArgumentCaptor.forClass(String.class);
Mockito.verify(mockObject).method(captor.capture());
String argument = captor.getValue();
In this code segment, captor.capture()
is utilized to seize the argument that method()
accepts. Subsequently, the captured argument can be fetched using captor.getValue()
. This action paves the way for additional assertions to be conducted on the argument, thereby elevating the effectiveness of the unit test.
Mockito is a flexible framework that facilitates the generation of mock objects, the configuration of return values from method calls on these objects, and the validation of specific methods invoked on the mock objects. With regards to the ArgumentCaptor
class, Mockito logs the interaction with mock objects, enabling the capture of arguments during verification of method calls.
The latest release of Mockito Core version 4.9.0 continues to evolve the framework, introducing features to mock static methods and final classes, and providing support for spies and reflection to modify private fields or invoke private methods. Mockito has thus become a comprehensive tool for software engineers, assisting in the handling of technical debt and adapting to ever-changing requirements.
To elaborate on the use of ArgumentCaptor in Mockito, an ArgumentCaptor object is created, and the capture()
method is employed to seize the arguments passed to the method under test. This is beneficial when the values of the arguments need to be verified or additional assertions need to be performed on them. Here's an example of how this process works:
```java
ArgumentCaptor
// Execute the method call for which you want to capture the arguments yourMockObject.yourMethod(argumentCaptor.capture());
// Retrieve the captured argument(s) String capturedArgument = argumentCaptor.getValue();
// Perform assertions or verifications on the captured argument(s) // ... ```
The call to argumentCaptor.capture()
prompts Mockito to capture the argument(s) passed to the method and store them in the ArgumentCaptor object. The captured argument(s) can then be retrieved using the getValue()
method. It's important to note that ArgumentCaptor can only capture the arguments of the most recent method call. If the method is called multiple times, the previously captured arguments will be overwritten. In cases where arguments for multiple method calls need to be captured, multiple ArgumentCaptor objects can be created
8. Writing a New Mockito Test for ConfigureThreadingUtil: A Practical Example
An exploration into Mockito testing brings us to a practical scenario involving a ConfigureThreadingUtil
class and its configure()
method. We aim to aptly test this method, leveraging the robust features of Mockito.
We begin by creating a mock of ConfigureThreadingUtil
:
java
ConfigureThreadingUtil util = Mockito.mock(ConfigureThreadingUtil.class);
Following this, we stub the configure()
method:
java
Mockito.when(util.configure()).thenReturn(true);
In our test code, we call the method and verify its execution:
java
boolean result = util.configure();
Mockito.verify(util).configure();
Our test culminates with an assertion that the method returned the expected value:
java
Assert.assertEquals(true, result);
This simple example demonstrates Mockito's effectiveness and versatility in unit testing. From Mockito version 3.8.0, features for mocking static methods and constructors have been introduced, further enhancing its flexibility for more intricate tests. For instance, Mockito's mockStatic
method can be employed to mock static methods, aiding in testing code that relies on fixed values or system properties.
The verify
method in Mockito serves as an efficient tool to ascertain if a specific method has been called in your test. This can be especially beneficial in cases like testing an ecommerce application where the customer's data gets stored in the session following a successful login. Using a spy instead of a mock could prove advantageous when there's no actual database integration occurring.
It's crucial to remember to use Mockito's static and constructor mocking responsibly and sparingly. While these features are potent, they should be utilized wisely to uphold the readability and maintainability of your tests.
The examples discussed here are available on GitHub for further exploration. Moreover, resources and blog posts related to unit testing basics and best practices for Java unit testing are available on the Machinet.net website. These can provide additional insights and tutorials to enhance your understanding of Mockito and its applications in unit testing.
To generate unit tests using Machinet's unit test generation feature, the code snippets provided on Machinet.net can be a valuable resource. By referring to these snippets, you can effectively leverage Machinet's unit test generation feature and bolster your unit testing process.
Integration of Machinet into existing development workflows could also contribute to enhancing the overall quality and efficiency of your software development process. Identification of specific workflows to be integrated with Machinet, determination of integration points, utilization of the Machinet API or SDK for interaction, configuration of Machinet to fit specific requirements, and training of the development team on effective usage of Machinet are steps that can guide this integration.
Remember, Mockito's capabilities, when combined with resources from platforms like Machinet.net, can significantly improve the quality and efficiency of your unit testing process
Conclusion
In conclusion, the article provides a comprehensive overview of the Mockito testing framework and its various features. It emphasizes the importance of adding Mockito as a dependency to a Java project and provides step-by-step instructions for Maven and Gradle users. The article also highlights how Mockito can be used to configure the return values of method calls on mock objects, including mocking static methods and constructors. It discusses the usage of @InjectMocks
for dependency injection and demonstrates how to verify method calls on mock objects using the verify()
method. Additionally, the article explores the use of spies in Mockito for enhancing test coverage and flexibility. Lastly, it explains how to capture arguments in unit tests using the ArgumentCaptor
class.
The ideas discussed in this article are significant because they provide software engineers with powerful tools and techniques for writing effective and reliable unit tests. By utilizing Mockito's features, developers can mock dependencies, control method behavior, verify method calls, and capture arguments, ultimately improving the quality and reliability of their software applications. These advanced techniques enable developers to isolate components, test specific scenarios, and ensure that their code interacts correctly with external systems. Incorporating Mockito into the unit testing process can lead to more robust software development practices.
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.