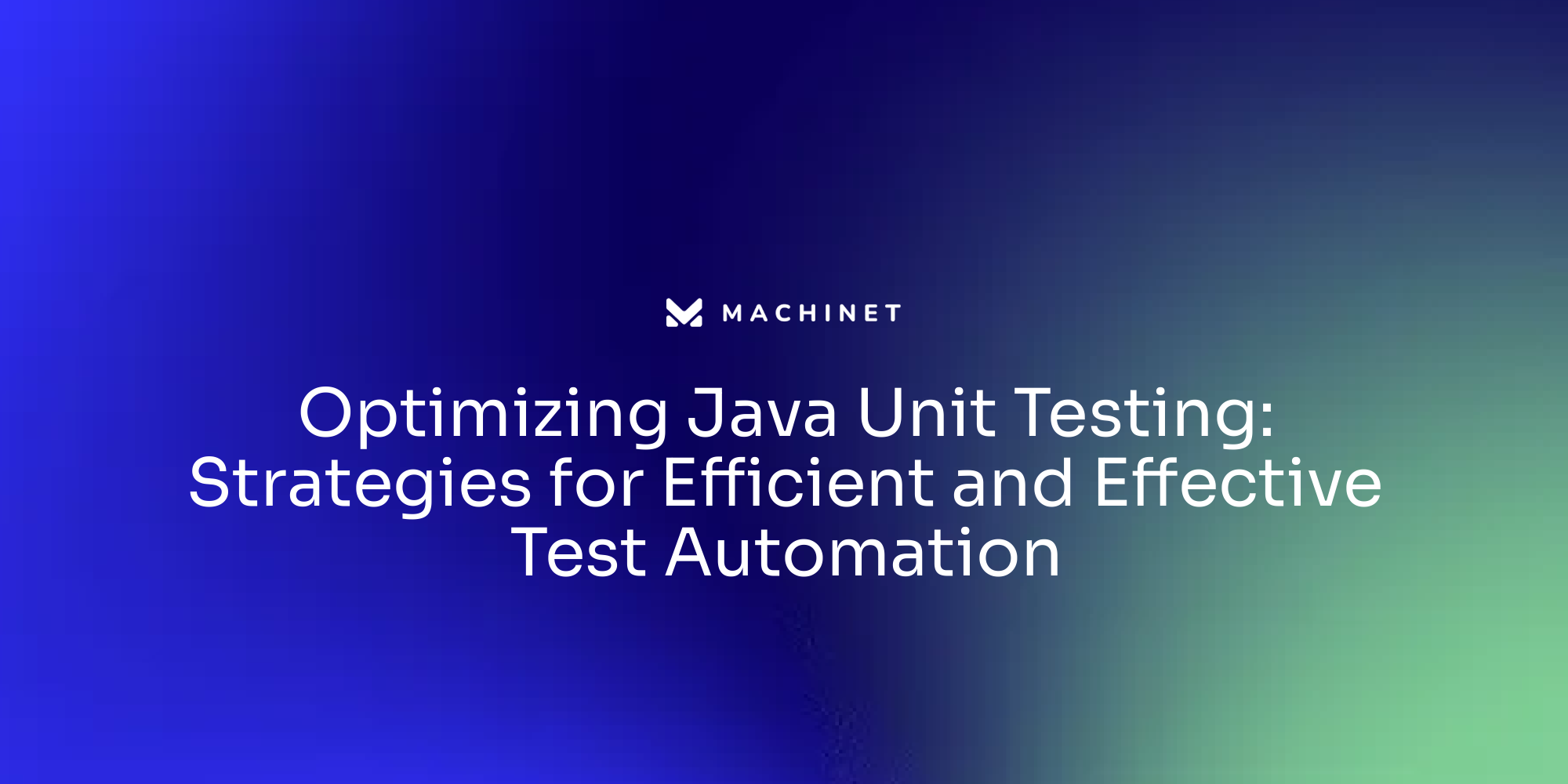
Table of Contents
- Understanding the Basics of Java Unit Testing
- Identifying High Value Candidates for Test Optimization
- Debugging and Profiling: Key Steps in Optimizing Java Unit Tests
- Techniques for Speeding up Java Unit Tests
- Implementing Robust and Flexible Testing Frameworks for Evolving Project Needs
- Managing Workload and Balancing Deadlines in Test Automation
- Strategies to Effectively Refactor and Improve Existing Test Suites
- Tips for Faster Java Unit Tests: Maximizing Optimization Effort vs Benefit
Introduction
Unit testing is a critical element in the world of Java software development, providing a reliable method to verify the functionality of individual components within a larger system. A solid foundation in unit testing involves understanding testing frameworks, creating effective tests, and embracing the principles of Test-Driven Development (TDD). In this article, we will explore the basics of Java unit testing, including the widely accepted JUnit framework and its annotations and assert methods. We will also discuss the importance of unit testing in Test-Driven Development and how mocking can be used to handle external dependencies. By implementing robust unit tests, developers can ensure the quality and functionality of their Java applications.
Unit testing is an integral part of Test-Driven Development (TDD), an approach that emphasizes writing tests before writing code. By following TDD principles, developers can ensure that new functionality does not disrupt existing functionality and identify bugs early in the development process. In this article, we will explore the strategies and best practices for implementing effective unit tests in Java, including the importance of test organization, using meaningful test names, and avoiding dependencies on external resources. We will also discuss techniques for speeding up unit tests, such as running tests concurrently and minimizing interactions with databases. By following these strategies, developers can optimize their unit tests for better performance and enhance the overall efficiency of their Java applications
1. Understanding the Basics of Java Unit Testing
Unit testing within the sphere of Java is a critical element, offering a reliable method for verifying the functionality of individual components within a broader software system. This verification process is achieved by focusing on the smallest testable parts of an application, primarily individual methods, to confirm their correctness. A robust foundation in unit testing involves a comprehensive understanding of testing frameworks, the creation of effective tests, and the principles of Test-Driven Development (TDD).
The unit testing framework JUnit is a widely accepted tool within Java application development. It is supported by a plethora of Java Integrated Development Environments (IDEs) and build tools. For example, Eclipse provides robust support for JUnit, incorporating it as its default testing library. In JUnit, a test class is structured with methods marked with unique JUnit annotations. For JUnit 4, annotations include @BeforeClass, @Before, @Test, and @After, while JUnit 5 utilizes @BeforeAll, @BeforeEach, @Test, and @AfterEach. These test methods use assert statements to verify if the code under test produces the expected results. JUnit offers a variety of assert methods such as assertEquals, assertNotEquals, assertTrue, assertFalse, assertNull, assertNotNull, assertSame, and assertNotSame.
Learn more about JUnit assert methods.
Unit testing is a crucial part of Test-Driven Development (TDD), assisting in the early detection of code issues. It ensures that new functionality does not disrupt existing functionality and aids in identifying bugs early in the development process. Test cases in unit testing are written to compare the expected output of a method with the actual output, using assertions to determine whether a test passes or fails by comparing expected and actual values.
Mocking is another vital aspect of unit testing, particularly when dealing with external dependencies. It involves creating mock objects that mimic the behavior of these dependencies, often using libraries like Mockito. Method stubbing is used to influence the behavior of mocked objects and return specific values. For example, test cases for methods that involve database queries or other external operations can use mocking to simulate the behavior of those dependencies.
The unit testing process in Java encompasses initializing necessary parameters, creating mock objects, stubbing methods, and calling the method being tested. Assertions are then used to evaluate the outcome of the test and determine whether it passes or fails. To ensure thorough testing, test cases should cover different scenarios and conditions. Therefore, when executed using the JUnit framework and the Mockito library, unit testing provides a reliable method to ensure the quality and functionality of software, aiding in the early identification of bugs and issues.
When writing unit tests, it is important to follow best practices such as writing small, focused tests, using meaningful test names, and organizing tests into logical groups. Use assertions to validate expected behavior and test doubles like mocks and stubs to isolate dependencies. Avoid testing implementation details and run tests frequently as part of the development process.
Unit tests should be clear, concise, and focus on a specific unit of functionality.
Discover best practices for writing unit tests.
Using annotations such as @Test to mark test methods and @Before, @After to set up and tear down test environments. Utilize assertions like assertEquals, assertTrue, assertFalse to validate expected results. Follow the Arrange-Act-Assert pattern, where the test case sets up the initial conditions, performs the action, and then verifies the expected outcome. Implement tests that cover both positive and negative scenarios, edge cases, and boundary conditions. Regular refactoring of the code to improve its design and maintainability is also recommended.
To optimize unit tests for better performance, several techniques can be considered.
Explore techniques for optimizing unit tests.
These include reducing unnecessary setup and teardown, using test data efficiently, running tests in parallel, mocking external dependencies, optimizing assertions, and using appropriate testing frameworks.
Comprehensive unit testing is crucial in Java to ensure the quality and reliability of software. It aids in identifying and fixing bugs, improving code coverage, maintaining code integrity, promoting code reusability and modularity, facilitating collaboration among team members, providing documentation and examples for future reference, and ensuring that changes or updates to the code do not introduce new bugs or break existing functionality
2. Identifying High Value Candidates for Test Optimization
Unit tests play a pivotal role in software development, but their value is not uniform. Some tests are more vital to the project's success than others. By identifying these high-value tests and optimizing them, you can significantly enhance your testing process. High-value tests usually cover essential functionality, have a high likelihood of failure, or are frequently executed. Focusing your optimization efforts on these tests can amplify your enhancements, ensuring your most crucial tests are efficient and effective.
Unit tests serve as a safety net against regression issues that might unexpectedly arise during refactoring, bug fixing, or the integration of new features. They need to be fastβideally in seconds, not minutesβto maintain a smooth workflow. Slow tests can extend the time required for developers to refactor the logic, as they may need to run the entire test suite to ensure no new regression issues have appeared.
Unit tests should be independent, meaning they should not share state. If a test stores data in shared memory and subsequent tests rely on that data, running individual tests or parallel tests becomes complicated.
Tests should be repeatable and not reliant on external factors like databases or file systems. This ensures that unit tests don't require pre-setup and can produce consistent results across different environments.
Ideally, unit tests should be self-validating, producing a boolean value after execution to indicate whether the test has passed or failed. This eliminates the need for developers to sift through logs or similar resources to determine the test execution results.
Your unit test suite should evolve over time with the application code. Tests should be written timely, not just before going into production. Avoid non-determinism in unit tests, which refers to the scenario where the same logic under test sometimes passes and sometimes fails. To identify the root cause of non-deterministic behavior, developers need to thoroughly examine both the unit test code and the logic under test.
To identify high-value unit tests for optimization, consider the purpose and impact of the tests. High-value unit tests provide significant coverage and contribute to the overall quality and stability of the codebase. Prioritize tests that cover critical or complex functionality. These tests are likely to catch important bugs and ensure that key features of the code are functioning as expected. Tests that cover code paths that are frequently used or have a high likelihood of failure should also be considered high-value.
When optimizing high-value unit tests, there are several strategies that can be employed.
These strategies include identifying and focusing on the most critical and high-risk areas of the codebase, prioritizing tests that provide the most coverage and value, and leveraging techniques such as parallel test execution and test data management to improve efficiency.
In software development, high-value unit tests are essential for ensuring the quality and reliability of the code. These tests focus on critical functionalities and scenarios that have a significant impact on the overall performance and functionality of the software. They are designed to identify and prevent potential issues, bugs, and regressions in the codebase.
To improve the efficiency of high-value unit tests, it is important to follow certain practices. This means identifying and prioritizing the areas of the code that are most prone to errors, have the highest impact on the overall system, or are critical for business requirements. Unit tests should not have dependencies on external systems or resources, as this can slow down the execution time and make the tests more brittle. Instead, use techniques such as mocking or stubbing to isolate the code being tested.
To optimize high-value unit tests, there are several tools and techniques that can be employed. These include using mocking frameworks, such as Mockito or PowerMock, to simulate dependencies and isolate the unit under test. Another technique is to use code coverage tools, such as JaCoCo or Cobertura, to identify areas of code that are not being adequately tested. Additionally, performance testing tools like JMeter can be used to measure the performance of unit tests and identify any bottlenecks.
Optimizing high-value unit tests can have a significant impact on overall project efficiency. By focusing on optimizing tests that provide the most value, developers can ensure that they are spending their time and resources on the most critical aspects of the codebase. This can lead to faster and more reliable builds, as well as quicker feedback on any potential issues or regressions in the code. Additionally, optimizing high-value unit tests can help reduce the overall testing time, allowing developers to iterate and deploy changes more quickly. This can ultimately lead to increased productivity and efficiency in the development process
3. Debugging and Profiling: Key Steps in Optimizing Java Unit Tests
As a seasoned software engineer, you're no stranger to the nuances of Java unit tests. A key aspect of ensuring the efficiency and effectiveness of these tests lies in the dual practices of debugging and profiling. Debugging helps identify and rectify any errors within your tests, while profiling facilitates a thorough understanding of your test's performance characteristics.
Consider, for instance, the IntelliJ IDEA Profiler, a tool known for its proficiency in CPU profiling. The value it brings to even the simplest of applications is significant. It provides a detailed analysis of the runtime behavior of your code, helping you pinpoint areas of inefficiency and hot spots.
Let's delve into an example. Assume we have an application that repeatedly attempts to create a filesystem path, measuring the throughput using a benchmark. Profiling this application using the IntelliJ IDEA Profiler, we can glean valuable insights into its performance. The tool provides a flame graph that represents sampled stacks and the time spent in different parts of the code. This allows us to identify the removeIf
method as a performance bottleneck. By replacing this method with a loop, we can markedly enhance the application's performance.
But the profiling doesn't end there. A closer look reveals exceptions related to file existence in the createDirectories
method, which are negatively impacting performance. A simple solution here is to wrap the call to createDirectories
in a filesExists
check. This prevents unnecessary exception handling and further boosts the application's performance.
The IntelliJ IDEA Profiler proves invaluable in detecting and rectifying these performance issues. It offers various ways to analyze the profile report, with the flame graph being just one of them.
Apart from the IntelliJ IDEA Profiler, tools such as JProfiler or VisualVM can also be leveraged to profile Java unit tests and pinpoint performance bottlenecks. They provide insights into CPU usage, memory allocation, and execution time, enabling you to identify areas ripe for optimization.
For example, VisualVM is a powerful tool that provides detailed information about CPU usage, memory usage, thread activity, and more. This information can help you identify bottlenecks and optimize your code accordingly. With VisualVM, you can also take heap and thread dumps, monitor garbage collection activity, and even profile remote applications.
Moreover, consider the repository "jfincher42/debugtutorial" on GitHub, a platform known for automation of workflows, hosting, and management of packages. This repository, written in Java, provides a practical guide to debugging and profiling Java unit tests.
The process of fixing errors in Java unit tests involves identifying the specific error message or behavior, reviewing the code for syntax errors, logical errors, or missing dependencies, analyzing the error message or behavior to understand the root cause, making necessary changes to the code, and running the unit tests again to verify that the error has been resolved. Remember, fixing errors in unit tests is an iterative process and it may need multiple iterations to completely resolve the issue.
To optimize Java unit tests with profiling, you can use a variety of techniques.
Profiling tools can provide insights into the performance of your code and help identify areas that can be optimized. By analyzing the execution time of different methods or sections of code, you can focus on optimizing the slowest parts.
By regularly debugging and profiling your tests, you can ensure they are not only correct but also efficient. This practice will help you manage technical debt, deal with constantly changing requirements, and balance workload and deadlines, ultimately leading to higher quality software products
4. Techniques for Speeding up Java Unit Tests
Optimizing the speed of Java unit tests is a multi-pronged task, encompassing a variety of strategies. A key area to focus on is testing the most crucial aspects of the code, which helps reduce the scope of tests and subsequently, the time taken for their execution. This can be achieved by using test doubles, such as mocks or stubs, to simulate dependencies and isolate the code under test.
Another critical strategy is to minimize interactions with the database as much as possible, as these can often lead to delays in test execution. Here, the use of mock objects or in-memory databases can be extremely beneficial. Mocking frameworks like Mockito allow developers to create mock objects that mimic the behavior of real objects, but with predictable responses. This makes it easier to test specific functionality without having to rely on external dependencies, thus minimizing database interactions.
Speeding up Java unit tests can also be achieved by tapping into the power of parallelism in test execution. Running tests concurrently can drastically cut down the total time taken for test execution. This can be achieved using frameworks and tools such as JUnit or TestNG, which provide built-in support for running tests in parallel.
Maintaining high-speed builds in software development is vital, as slow builds can significantly impact team productivity. Performance tips like concurrent test execution, avoiding expensive setup and cleanup in tests, distinguishing between unit and integration tests, and fixing flaky tests can help improve build speeds.
For instance, running tests concurrently and using more threads than CPUs can help reduce build times. Additionally, minimizing the use of external resources, such as databases or network calls, during unit tests can also speed up the process.
Distinguishing between unit tests and integration tests is another strategy for speeding up Java unit tests. Unit tests are typically faster and more isolated, while integration tests involve more components and are slower. By separating these two types of tests, builds can fail faster, and developers can address issues more quickly.
Flaky tests, which unpredictably fail or pass, often slow down the testing process and waste time. Therefore, fixing these tests is another strategy for speeding up Java unit tests.
Moreover, keeping up with modern build tools and ensuring they are up-to-date can help improve build speeds. Good build tools continuously work on performance issues, and using the latest versions can help take advantage of these improvements.
Lastly, investing in faster Continuous Integration (CI) machines can also contribute to faster build times. Every second counts in build times, and faster CI machines can help save valuable time. For instance, switching from Travis CI to AWS CodeBuild with a proper instance type can quadruple the CI performance, reducing the build time significantly.
While these strategies can be highly effective, it's vital to remember that they may not be suitable for every team or project. Therefore, it's crucial to adapt these strategies to fit the specific context and needs of the team and the project.
In summary, improving Java unit tests' speed involves a blend of strategies, including reducing the scope of tests, minimizing database interactions, running tests concurrently, distinguishing between unit and integration tests, fixing flaky tests, and investing in faster CI machines. By implementing these strategies, teams can significantly improve their build speeds and productivity
5. Implementing Robust and Flexible Testing Frameworks for Evolving Project Needs
The significance of testing frameworks in the ever-changing landscape of software development is undeniable. They form the backbone that accommodates the fluidity of project requirements, enabling the seamless integration, modification, or removal of tests. A truly flexible framework should support various testing types, including unit, integration, and functional testing, catering to the dynamic needs of the project.
Java, in particular, demands a robust testing framework to ensure the consistency and reliability of your tests, regardless of any changes in project specifications or the codebase. This robustness is instrumental in maintaining the software's integrity, providing accurate feedback, and facilitating the success of Continuous Integration/Continuous Deployment (CI/CD) processes.
For example, when dealing with Swing applications, testing is paramount to ensure their reliability and stability. Libraries such as Fest Swing have become popular tools for testing these applications, allowing developers to simulate user actions and verify results using matchers. Other tools, like AssertJ and JUnit 5, provide a comprehensive and expressive testing framework. AssertJ's fluent assertions and JUnit 5's test annotations enable developers to create readable and effective tests. These tools, when integrated, simplify the setup and teardown of resources.
A common challenge in testing is executing UI tests in a headless environment. However, solutions like TightVNCServer can address this issue. The use of Maven configuration further aids in separating UI tests from unit tests, thereby preventing concurrent running and potential conflicts.
AssertJ enhances the failure reporting process by providing detailed messages about assertion failures, ensuring developers have all the necessary information to identify and rectify issues promptly. Furthermore, AssertJ and JUnit 5 support parameterized tests, facilitating the testing of a wide range of scenarios with clarity and readability.
However, building an effective testing framework isn't merely about selecting the right tools. It involves managing test data and state, test reporting and visualization, and dynamic test environments. Integration with code quality tools, continuous monitoring, and test case management systems also plays a significant role in overall software quality.
A robust and flexible testing framework construction requires a comprehensive approach. Utilizing built-in Java testing tools in conjunction with third-party libraries can result in a framework that is not only robust and flexible but also efficient and effective.
To build such a flexible testing framework in Java, developers can use annotations and assertions provided by the JUnit framework. Annotations allow the definition of test methods and test classes, while assertions enable the verification of the expected behavior of the code. Other features of JUnit, such as test fixtures, parameterized tests, and test suites, can be leveraged to create a comprehensive and flexible testing framework in Java.
To maintain a robust testing framework, it's crucial to follow best practices and techniques, including using a combination of unit tests, integration tests, and end-to-end tests to thoroughly test the different components of your application. It's also essential to use a reliable testing framework that supports features like test automation, test data management, and test reporting. Writing testable code that is modular, loosely coupled, and follows the principles of dependency injection, regularly maintaining and updating your test suite, and incorporating code coverage analysis can also contribute to the robustness of your testing framework.
There are several third-party libraries available for building a robust Java testing framework. These libraries, including JUnit, TestNG, Mockito, PowerMock, and AssertJ, offer features such as test case management, assertion libraries, mocking frameworks, and test execution, and reporting tools. These libraries can be chosen based on specific requirements to build a robust testing framework for Java applications.
Finally, to ensure reliable and consistent test results in a Java testing framework, it's important to follow some best practices. Use appropriate annotations, write independent test cases, use assertions effectively, test edge cases, use mocking and stubbing, and run tests in isolation. Following these tips can improve the reliability and consistency of your test results in a Java testing framework
6. Managing Workload and Balancing Deadlines in Test Automation
In the sphere of test automation, managing workloads and balancing deadlines is a consistent challenge. This necessitates a strategic allocation of tests, execution planning, and efficient resource utilization. An effective way to manage the workload is by prioritizing tests based on their significance and risk level. This approach ensures focused attention on the most critical aspects of the project.
Prioritizing tests involves considering the criticality and impact of each test case. By assigning priority levels, the most important and high-risk scenarios are tested first, enabling early identification and resolution of critical issues. Additionally, tests can be prioritized based on their dependency on other test cases or functionalities. This planned order of execution optimizes test execution and enhances the overall efficiency of the test automation process.
To minimize the impact on development activities, scheduling test execution during non-peak times allows for smoother execution without additional strain on system resources during peak development times.
The incorporation of automated testing tools, such as Selenium, JUnit, TestNG, and Apache JMeter, can considerably streamline workload management. These tools execute tests swiftly, providing timely feedback, thus enabling more efficient resource and task management. They offer test case management, test script creation, test execution, and result analysis features.
Balancing deadlines involves careful planning and scheduling of testing activities, ensuring that the testing phase does not impede the development process. This requires a well-structured plan aligned with the project timeline to prevent testing from becoming a bottleneck. Careful consideration of factors such as project scope and complexity, available resources, and timeline is necessary. Identifying specific testing objectives, creating a detailed test plan, and allocating necessary resources accordingly is crucial. Regular communication and coordination with project stakeholders ensure testing activities align with project goals and objectives.
Resource management is vital to ensure efficiency and reliability in test automation. Best practices include using resource pooling, implementing resource cleanup, using dependency injection, handling resource conflicts, and monitoring resource usage.
The concept of maintaining a "sustainable pace" is key in agile software development. This means frequently delivering small value chunks to customers at a consistent pace. Good development practices such as test-driven development and continuous integration contribute to maintaining a sustainable pace.
However, many software organizations overlook the importance of working at a sustainable pace, leading to challenges in delivering valuable changes to customers frequently and predictably. Teams need to assess their process to avoid falling into the trap of overworking and skimping on good practices.
Teams have more influence than they think and can set boundaries, such as agreeing to not work more than a standard work week. Making overtime visible and capturing "real" hours worked can help highlight the extent of overwork and create awareness.
Slicing stories into small, consistently sized increments and limiting work in progress can contribute to a predictable and consistent cadence. Managers should educate business stakeholders about the negative consequences of not maintaining a sustainable pace, such as technical debt and decreased productivity.
Nurturing a learning culture and investing time in continuous learning and improvement can lead to high-performing teams that deliver small changes consistently. It is important for teams to discuss and address workload challenges in retrospectives and brainstorm small experiments to promote a more sustainable approach.
Not maintaining a sustainable pace can lead to burnout and turnover, which is costly for organizations. Institutions like the Agile Testing Fellowship offer courses and training led by experts in agile testing to help teams navigate these challenges
7. Strategies to Effectively Refactor and Improve Existing Test Suites
The continuous evolution of a codebase requires the consistent refinement of existing test suites to maintain their value and effectiveness. This refinement process involves eliminating redundant tests, breaking down extensive tests into more manageable segments, and updating tests to reflect changes in the codebase.
Test suite enhancement can also involve increasing test coverage, refining test organization, and integrating new testing techniques or tools. The concept of "packing light" in software development, as advocated by Kent Beck in "Extreme Programming Explained," suggests that carrying only the necessary code and tests can streamline the development process.
Tests can become "cruft," a term used in software development to describe outdated or incorrect elements that hinder progress. An analytical approach is crucial to determining the usefulness of each test and identifying those that have become crufty. Factors such as test setup time, test execution time, recent bugs discovered, and the value and complexity of the test are integral to this analysis.
Creating a comprehensive spreadsheet containing all relevant data about the tests and sorting them based on various criteria can help identify the least efficient tests. Redundant tests, such as those testing identical logic at different levels (unit, integration, end-to-end), can be either retired or pushed down to a lower level to enhance efficiency.
Tagging can further categorize tests, allowing for the execution of specific subsets of tests based on the feature being worked on or the type of test being conducted. The decision to retire tests should be based on a pain-versus-value analysis, with data collection being paramount for making informed decisions.
While running tests in parallel can boost efficiency, it may also increase the maintenance burden. Hence, the importance of data gathering and informed decision-making in retiring tests cannot be overstated.
Maintaining the same standard of quality for test code as for production code is essential. The technique of refactoring against the red bar, as discussed in a presentation by M. Scott Ford at AgileDC 2016, can be instrumental in confidently refining test code without introducing false positives.
In the constant review and enhancement of test suites, several strategies can be employed to improve existing tests. Identifying and prioritizing the most critical test cases and focusing on enhancing their coverage can be one approach. This involves analyzing the existing test suite and identifying any gaps or areas that need improvement. Automation can be introduced into the testing process to improve the efficiency and effectiveness of the test suite significantly. By automating repetitive and time-consuming tasks, such as test data setup and teardown, the efficiency and effectiveness of the test suite can be significantly improved.
Refactoring test suites involves improving the structure and organization of existing test cases to make them more efficient, maintainable, and readable. It is a best practice in software development to periodically review and refactor test suites to ensure their effectiveness. Grouping related test cases into separate test classes or test methods can make it easier to understand and maintain the test suite.
To maintain test effectiveness and efficiency, it is important to follow best practices for unit testing in Java. This includes techniques such as writing small, focused tests that cover specific functionality, using mocking frameworks to isolate dependencies, and using assertions to verify expected behavior. Regularly reviewing and refactoring test code to ensure it remains clean and maintainable can ensure that tests are effective in catching bugs and efficient in terms of execution time and resource usage.
In conclusion, the regular review and enhancement of test suites is a crucial aspect of maintaining their effectiveness and efficiency over time. This involves reviewing and updating tests to ensure they remain relevant and effective as the codebase evolves
8. Tips for Faster Java Unit Tests: Maximizing Optimization Effort vs Benefit
Unit test optimization in Java requires a systematic approach, focusing on areas that yield the most impact. Prioritize test cases that are frequently executed or consume a significant amount of time. To pinpoint areas ripe for improvement and bottlenecks, leverage profiling tools. These tools can provide insights into code performance, execution time, memory usage, and resource consumption, aiding in identifying potential bottlenecks.
Automation is a powerful tool in the test optimization process. Automated testing tools can accelerate the execution of tests, reduce manual efforts, and improve test execution speed. Strategies such as parallel test execution, test data optimization, test environment optimization, and test suite optimization can be employed to enhance the speed of test execution.
However, remember that optimization is a continuous process requiring regular monitoring and adjustments. As your project grows and evolves, your optimization strategies should adapt accordingly. Regularly review and refactor your tests to eliminate redundant or unnecessary code. This can improve overall efficiency and maintainability of the test suite. Prioritize the tests based on their significance and impact on the system, ensuring that critical parts of the code are thoroughly tested.
Unit testing is a fundamental aspect of modern software development. It plays a key role in bug detection and prevention, code refactoring, and maintenance. Effective unit tests adhere to best practices such as the AAA (Arrange-Act-Assert) pattern, minimizing dependencies, avoiding test interdependencies, and maintaining test coverage.
Automated unit testing tools offer numerous benefits, including support for different test frameworks, code coverage analysis, integration with CI/CD pipelines, and generation of test execution reports. These tools can significantly augment the efficiency and effectiveness of unit testing processes.
On the other hand, manual unit testing involves a series of steps, including choosing the unit to test, setting up the test environment, defining test cases, running the unit test, and analyzing the results. Despite being more labor-intensive, manual unit testing can provide valuable insights and help uncover issues that automated tests might miss.
In the end, effective unit testing significantly contributes to code quality, bug detection, maintainability, and collaboration, fostering continuous improvement in software development. Whether you choose to automate your tests or write them manually, remember that the key lies in striking a balance between quantity and quality, and maintaining your test code as your codebase evolves. By following these best practices and strategies, you can optimize your Java unit tests for maximum efficiency, reducing the overall testing time while still ensuring the quality of your code
Conclusion
In conclusion, Java unit testing is an essential aspect of software development that ensures the quality and functionality of applications. By understanding testing frameworks like JUnit, creating effective tests, and embracing Test-Driven Development (TDD) principles, developers can verify the correctness of individual components within a larger system. Unit testing also plays a crucial role in identifying bugs early in the development process and preventing new functionality from disrupting existing functionality. Additionally, techniques like mocking can be used to handle external dependencies and improve the efficiency of unit tests. Overall, implementing robust unit tests is vital for ensuring the reliability and effectiveness of Java applications.
The ideas discussed in this article have broader significance for developers and software engineering teams. By following best practices for implementing effective unit tests in Java, such as organizing tests, using meaningful test names, and avoiding dependencies on external resources, developers can optimize their testing process. Techniques like running tests concurrently and minimizing interactions with databases can also speed up unit tests and enhance overall efficiency. Adopting these strategies not only improves the quality of software but also boosts developer productivity by providing faster feedback on code changes. It is crucial for developers to prioritize unit testing as an integral part of their development process to ensure the success of their projects.
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.