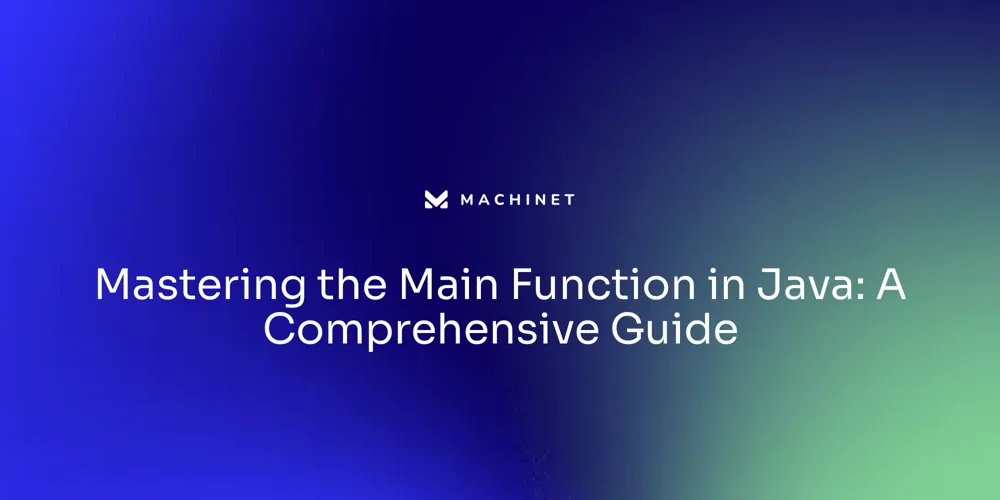
Table of Contents
- Syntax and Structure of the Main Method
- Understanding the 'public static void main' Declaration
- The Role of the 'String[] args' Parameter
- Examples of Using the Main Method
- Common Errors and Troubleshooting
- Best Practices for Writing the Main Method
- Advanced Topics: Overloading and Method Signature
Introduction
The main method in Java is a crucial component of a program, serving as its entry point and laying the foundation for execution. Understanding the syntax and structure of the main method is essential for navigating the complexities of Java and its syntax.
This article explores the main method in detail, covering its declaration, the role of the 'String[] args' parameter, common errors and troubleshooting, best practices for writing the main method, and advanced topics such as overloading and method signature. By grasping the concepts and principles outlined in this article, developers can create code that is not only functional but also comprehensible to colleagues and future maintainers. So let's dive into the world of Java's main method and unlock its potential for efficient and effective programming.
Syntax and Structure of the Main Method
In Java, the main
method is akin to the central nervous system of a program, laying the groundwork for its execution. It's structured as public static void main(String[] args)
and each part of this signature serves a specific role.
The public
modifier ensures that this method is globally accessible, while static
allows it to run without the instantiation of its class. void
signifies that it won't return any values, and main
is the conventional name that identifies this method as the program's launch point.
The String[] args
parameter is particularly noteworthy as it introduces the ability to accept command-line arguments, which are akin to the various paths a bicycle delivery service might take to ensure timely deliveries in a bustling cityscape. This method's design embodies the Single Responsibility Principle (SRP), ensuring that it remains focused and uncluttered, much like the clear instructions needed for efficient package delivery in a growing business.
This principle is crucial for maintaining simplicity within methods, preventing them from becoming convoluted and unwieldy. Furthermore, understanding the purpose and structure of the main
method is essential for junior developers who are navigating the complexities of Java and its syntax. To illustrate, Eleftheria, an experienced business analyst, emphasizes the importance of clear and accessible content for those delving into technical subjects. This is mirrored in the way we write our code - with clarity and precision, ensuring that each method, like a well-documented delivery route, is understandable and serves a distinct purpose. By following these guidelines, developers can create code that is not only functional but also comprehensible to colleagues and future maintainers, akin to a well-oiled delivery system that adapts and scales with the demands of a growing city.
Understanding the 'public static void main' Declaration
In Java, the method signature public static void main
is more than just a random collection of keywords; it's a precise instruction to the Java Virtual Machine. Each keyword plays a crucial role in the program's execution:
-
public
: This accessibility modifier ensures the entry point is visible across different classes, allowing the JVM to invoke the main method from any location. -static
: By marking the main method as static, it eliminates the need for instantiating its class, facilitating a seamless launch of the program. -
void
: It signifies that the main method concludes without returning any data, aligning with Java's conventions. Methods are the backbone of Java programs, organizing related instructions into cohesive units. They adhere to the Single Responsibility Principle, ensuring each method addresses a distinct aspect of the program's functionality.
This approach simplifies complex processes into smaller, more manageable tasks. Moreover, well-documented methods, through comments, provide clarity on the codeβs purpose and structure, assisting in its understanding and maintenance. Interestingly, Java developers often work with a variety of other languages and technologies, including JavaScript, SQL, Python, and HTML/CSS, highlighting the interconnected nature of modern software development.
The Role of the 'String[] args' Parameter
In the realm of Java development, mastering the main method's 'String[] args' parameter is a pivotal skill for software developers. This parameter empowers your program to accept command-line arguments, offering a dynamic way for users to influence the program's behavior at runtime.
For instance, consider a program designed to print user-provided arguments:
java
public static void main(String[] args) {
for (String arg : args) {
System.out.println(arg);
}
}
When executed with java MyProgram arg1 arg2
, the console will output:
arg1
arg2
This feature not only adds interactivity but also serves as a bridge between the user and the program, allowing for customized input without the need for a graphical user interface. It's a demonstration of Java's inherent strength in performance and control, as you directly engage with the application's core functionality.
Understanding such native Java capabilities is essential, as they form the foundation upon which frameworks build. While frameworks offer streamlined development for complex tasks, the underlying principles of Java, such as handling command-line arguments, remain crucial. According to a survey, the most popular languages and technologies used alongside Java include JavaScript, SQL, Python, and HTML/CSS. This highlights the versatility and broad application of Java in the software development ecosystem, underscoring the importance of a solid grasp of its syntax and semantics for both seasoned professionals and those new to the field.
Examples of Using the Main Method
Java's main method serves as the entry point for program execution, showcasing its flexibility through various applications. For instance, it can display messages, as seen in a simple greeting:
```java public static void main(String[] args) { System.out.println("Hello, World!
"); } ```
It is also adept at handling calculations, allowing for arithmetic operations to be performed and results displayed:
java
public static void main(String[] args) {
int a = 5;
int b = 10;
int sum = a + b;
System.out.println("The sum of " + a + " and " + b + " is " + sum);
}
Moreover, the main method can facilitate user interaction by prompting for input, then utilizing that data within the program:
java
import java.util.Scanner;
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("Enter your name: ");
String name = scanner.nextLine();
System.out.println("Hello, " + name);
}
These examples underscore the method's adaptability in executing diverse tasks, serving as a microcosm of software development methodologies which emphasize structured, systematic approaches to design and development. The main method's versatility mirrors the adaptable nature of these methodologies, be it Agile, Waterfall, or Kanban, which guide developers through a project's lifecycle, from initiation to completion. As a result, understanding the main method's applications is akin to grasping the foundational elements of software development, where each line of code aligns with the broader goals and procedures outlined by the chosen methodology.
Common Errors and Troubleshooting
When using the main method in Java, it's crucial to ensure that it's correctly structured to avoid common errors. A properly defined main method should follow the signature public static void main(String[] args)
.
This allows Java to recognize it as the entry point of the application. If you encounter the Error: Main method not found in class MyProgram
, this signature may be incorrect or missing.
Remember that the Single Responsibility Principle (SRP) applies here; the main method should serve as the singular starting point without bearing additional complexity. Another frequent issue is the Error: Illegal modifier for the main method
.
This can occur if you've added extra modifiers that are not permitted. The main method should be straightforward and accessible, declared with only the necessary public
and static
modifiers to be executed by the Java Virtual Machine (JVM).
If you come across the Error: class not found
, it's often a sign that the class containing the main method hasn't been compiled or is not present in the classpath. Ensuring that your class is compiled and correctly located within the classpath is akin to organizing your code with clear comments that delineate sections or modules. This organization aids in navigating the codebase and troubleshooting such errors more effectively. By understanding these errors and their solutions, you can streamline your development process, akin to following a well-documented recipe. This approach not only saves time but also aligns with best practices in software development, such as method encapsulation and clear documentation through comments, which are vital for maintaining an organized and understandable codebase.
Best Practices for Writing the Main Method
Crafting the main method in Java is akin to setting the cornerstone for a building; it must be done with precision and clarity. To write an effective main method, consider the following guidelines:
1.
Conciseness is Key: The main method should be a gateway, initiating the execution of the program. It is not a place for intricate logic or additional features.
By keeping it concise, you create a clear path for program execution, avoiding unnecessary complexity. 2.
Descriptive Variable Names: Opt for variable names that convey their purpose. For instance, instead of 'Ctr', use 'Customer' to eliminate ambiguity.
This practice aligns with the adage, 'Code is read more often than it is written,' ensuring that your code remains accessible and comprehensible to all who encounter it. 3.
Exception Handling: Incorporating exception handling within your main method is vital. It equips your program to manage unexpected errors gracefully, safeguarding against unforeseen interruptions during runtime. 4. Modularity through Methods: Embrace the Single Responsibility Principle (SRP) by breaking down complex tasks into distinct methods, each with a focused role. This principle fosters simplicity and focus, preventing methods from becoming convoluted and difficult to maintain. By adhering to these practices, you contribute to the world of high-quality software development, where clear requirements and effective communication are paramount. These guidelines serve as the blueprint for crafting software that is both impactful and enduring, steering clear of the pitfalls of ambiguity and complexity.
Advanced Topics: Overloading and Method Signature
Java's capability to overload methods, including the main method, is a powerful feature that enhances the language's flexibility. Method overloading allows multiple methods to share the same name but differ in their parameter lists.
For example, the main method traditionally accepts a single argument, String[] args
, which serves as the entry point for the application. However, you can also define additional main methods with different parameters:
```java public class MyClass { public static void main(String[] args) { System.out.println("Main method with String[] args"); }
public static void main(int arg) {
System.out.println("Main method with int arg");
}
} ```
In the above code snippet, the second main method won't be executed by the Java Virtual Machine (JVM) as an entry point, but it illustrates the concept of method overloading.
This technique is in line with the Single Responsibility Principle, ensuring that each method in a class serves a unique purpose and maintains simplicity. Method overloading is not to be confused with method overriding, where a subclass provides a specific implementation for a method that is already defined in its superclass. Both practices are key to object-oriented programming and contribute to writing maintainable and modular code, which is particularly beneficial in domains such as data science and AI that demand efficient code structure. Remember, while Java remains a staple in the industry, it is often used in conjunction with technologies like JavaScript, SQL, Python, and HTML/CSS, reflecting the importance of a polyglot approach in modern software development.
Conclusion
In conclusion, the main method in Java is a crucial component of a program, serving as its entry point and laying the foundation for execution. Understanding its syntax and structure is essential for navigating the complexities of Java.
The 'String[] args' parameter allows for accepting command-line arguments, providing a dynamic way to customize program behavior. Troubleshooting common errors ensures the main method is correctly structured.
Best practices for writing the main method include keeping it concise, using descriptive variable names, incorporating exception handling, and embracing modularity through methods. These guidelines contribute to high-quality software development.
Advanced topics like overloading methods enhance Java's flexibility and maintainability. By grasping these concepts, developers can create code that is functional and comprehensible to colleagues. In summary, mastering the main method in Java unlocks its potential for efficient programming. Understanding its syntax, troubleshooting errors, following best practices, and exploring advanced topics contribute to creating high-quality code.
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.