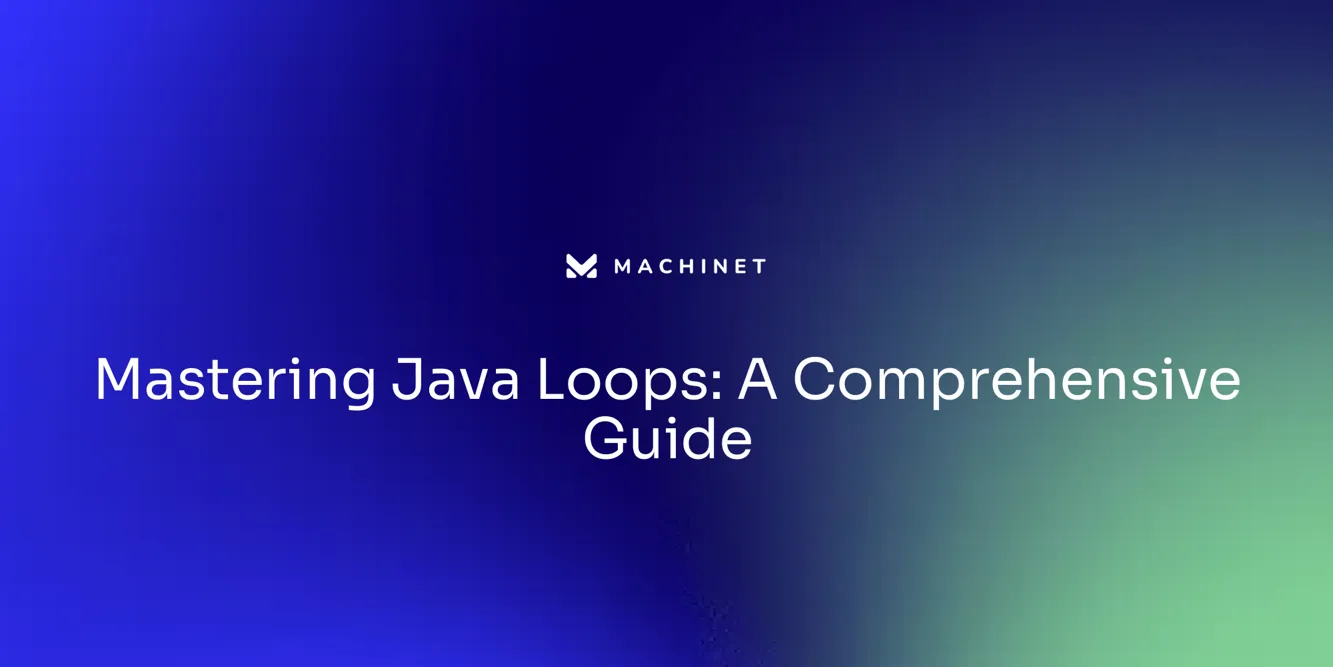
Table of Contents
- For Loop in Java
- Understanding the While Loop
- Nested Loops in Java
- Jump Statements in Loops
- Loop Control Statements
- Common Loop Patterns in Java
- Best Practices for Using Loops in Java
Introduction
The article discusses various aspects of loops in Java, including the 'for' loop, the 'while' loop, nested loops, jump statements, loop control statements, common loop patterns, and best practices for using loops. It explains the purpose and functionality of each type of loop and provides practical examples and applications.
The article also highlights the importance of understanding loop structures and offers tips for optimizing loop performance and avoiding common mistakes. Whether you are a beginner or an experienced Java developer, this article will enhance your understanding of loops and help you improve your coding skills.
For Loop in Java
The 'for' loop is a crucial part of Java's control flow, serving as a well-structured and efficient mechanism to execute a block of code multiple times. It consists of three parts: initialization, a condition, and an increment/decrement statement.
The loop continues until the condition is met, or indefinitely if no condition is specified. Let's consider a practical example: outputting a string parameter a specified number of times.
However, make sure to pass at least two arguments, failing to do so will result in an exception. Java has evolved significantly since its inception in 1995, constantly adding new features to stay relevant and efficient.
One of these is the introduction of lazy versions of numeric range-based 'for' loops, such as 'IntStream' and 'LongStream.' These offer an object-oriented approach to 'for' loops, providing versatile and high-performing alternatives.
Interestingly, Java's popularity remains high, despite the emergence of modern languages like Kotlin. This is largely due to frameworks like Spring, which is used by many top-tier companies. Java's versatility extends beyond simple loops and control flow. For instance, the 'Happy Number' problem is a unique challenge often faced by Java developers. Although it seems like a mathematical curiosity, it has practical applications in algorithm design and testing in Java.
Understanding the While Loop
In Java, the 'while' loop serves as a powerful tool, executing a specific block of code repeatedly as long as the stated condition remains true. Unlike its counterpart, the 'for' loop, a 'while' loop does not depend on initialization or increment/decrement statements, relying solely on the condition to dictate the loop's execution. To illustrate its utility, consider the 'Happy Number' problem often encountered by Java developers.
This mathematical curiosity has practical applications in Java and computer science, often used in algorithm design and testing. The 'while' loop is instrumental in solving this problem, as it can continuously iterate until the number reaches 1, indicating a happy number. Further, a 'while' loop is crucial in designing games, such as the Number Guessing Game, where the loop keeps prompting the player for their guess until the correct number is identified.
The shift in Java over the years, such as the introduction of the 'var' keyword, has made the platform easier to use, making 'while' loops more accessible for both beginners and veterans alike. Remember, a 'while' loop is a key component of control flow in Java and is highly useful for executing a block of code a specific number of times. It is a fundamental aspect of Java syntax and semantics that every developer should master.
Nested Loops in Java
Java's nested loops, defined within the scope of another loop, offer a means to traverse multidimensional or nested data structures. This capability allows the execution of repetitive operations on each element of the inner loop while the outer loop iterates.
Such a feature has a significant impact on the performance of your program, particularly when the number of iterations is large. However, with the increase in nested loops, the time complexity of your code can grow exponentially.
Furthermore, code with many nested loops tends to be less modular and more tightly coupled, making it challenging to reuse specific parts of the code in other contexts or applications. An interesting application of nested loops in Java is in algorithm design and testing, as seen in the 'Happy Number' problem, a common challenge for Java developers.
This problem showcases the practical applications of nested loops in Java and computer science beyond its mathematical curiosity. Another example is the use of a for loop in Java, a well-structured and extremely useful statement for executing a block of code a specified number of times. It's part of control flow in programming, used until a condition is met or infinitely if no condition is specified. Finally, it's worth noting that Java offers versatile and performant eager for statement and lazy versions of numeric range-based for loops in the form of IntStream and LongStream, providing alternatives to modeling for loops in an object-oriented programming language.
Jump Statements in Loops
Java's jump statements, namely 'break' and 'continue', offer a unique way to manipulate the regular execution sequence within loops. These statements give you the power to either exit a loop prematurely or bypass the current iteration and move on to the next, based on specific conditions.
In the realm of Java, the 'for' loop is a particularly well-structured and robust statement that is often utilized to execute a block of code multiple times until a certain condition is met, or even indefinitely if no condition is specified. For instance, the 'for' loop can be employed to output a specific string parameter a predetermined number of times.
An exception will be thrown if fewer than two arguments are provided. It is important to note that each loop iteration is contingent upon a loop-continuation-condition, a Boolean expression that dictates the execution of the loop body.
If the condition evaluates to true, the loop body is executed; if false, the loop terminates, and control is transferred to the subsequent statement. For example, a 'while' loop can be used to display the phrase 'Welcome to Java!' a hundred times. Such a counter-controlled loop is characterized by a control variable that counts the number of executions. However, it's worth noting that programmers often fall into the trap of off-by-one errors, executing a loop one more or fewer times than intended.
Loop Control Statements
Java's loop control statements, including 'break', 'continue', and 'return', offer unique control over the execution of loops. These statements can be used to entirely terminate a loop, bypass to the next iteration, or exit the enclosing method or function.
For instance, the 'for' loop, a crucial part of Java's control flow, is structured to execute a block of code repeatedly until a certain condition is met. This loop is particularly useful, especially when the precise number of iterations is known beforehand.
For instance, when iterating over numbers from 0 to 10 and printing them, the 'for' loop excels. However, it's important to avoid common pitfalls like the off-by-one error, where a loop is inadvertently executed one time less or more than intended due to an error in the condition. For example, to display a message 100 times, the condition should be 'count < 100' rather than 'count <= 100'. Leveraging these control statements correctly can enhance the efficiency and accuracy of your Java code.
Common Loop Patterns in Java
Java, being a robust and versatile programming language, offers a myriad of features, one of which is the concept of loops. Loops, specifically 'for' loops, are integral to control flow in Java, allowing code blocks to execute multiple times until a specified condition is met.
An interesting application of 'for' loops is the 'Happy Number' problem, which showcases the practical applications of mathematical concepts in Java. To understand loops better, let's explore some common patterns.
A common use-case is iterating over arrays or collections, where the loop helps traverse through each element. Additionally, loops can perform calculations on elements or search for specific elements within the array or collection.
Another aspect of Java's 'for' loops is their structure. They are well-structured and extremely useful for executing a block of code a specified number of times.
For instance, a 'for' loop can be used to output a string parameter a specified number of times. Furthermore, Java provides lazy versions of numeric range-based 'for' loops in the form of IntStream and LongStream. These provide a more object-oriented approach to looping, offering both versatility and performance. However, it's essential to be careful with the conditions specified for the loop to avoid common mistakes, such as the off-by-one error, where the loop executes one more or less time than intended. In the dynamic world of Java development, understanding these loop patterns and knowing when and how to apply them can enhance your programming skills and efficiency.
Best Practices for Using Loops in Java
Enhancing the efficiency and readability of your Java code is crucial, especially when dealing with loop structures. Proper initialization of loop variables, suitable use of loop constructs, elimination of unnecessary calculations within loops, and loop performance optimization are essential practices.
Let's delve into these aspects in detail. An effective strategy is to consider early exit or optimization, where you can program your loops to terminate prematurely under certain conditions, thus reducing the number of necessary iterations.
A case in point can be seen in the 'Happy Number' problem, a common challenge faced by Java developers. In this scenario, the loop terminates once the process reaches 1, indicating a happy number.
You can also explore alternative algorithms or data structures that may solve the problem with fewer iterations. The Java Collection Framework, for instance, allows you to choose the right collection based on the specific functionality you need, thereby eliminating the need for nested loops.
Moreover, it's advisable to leverage built-in functions or libraries that provide efficient solutions for common operations. The IntStream and LongStream classes, for example, offer lazy versions of numeric range-based for loops, which can be a boon for performance. Lastly, it's critical to avoid common pitfalls such as off-by-one errors, where a loop runs one time more or less than intended. This is a frequent mistake, as highlighted by a survey that showed a loop displaying 'Welcome to Java' 101 times instead of the intended 100. By incorporating these principles into your Java development workflow, you can heighten the quality of your code and become a more proficient Java developer.
Conclusion
In conclusion, this article provides a comprehensive overview of loops in Java, covering various types of loops such as the 'for' loop and the 'while' loop. It explores the purpose and functionality of each loop and offers practical examples and applications.
The article emphasizes the importance of understanding loop structures and highlights best practices for optimizing loop performance and avoiding common mistakes. It discusses nested loops and jump statements, showcasing their utility in solving problems and manipulating the execution sequence within loops.
Furthermore, the article touches on loop control statements and common loop patterns in Java, demonstrating their versatility and usefulness in different scenarios. It also encourages developers to follow best practices such as proper initialization, suitable use of constructs, elimination of unnecessary calculations, and leveraging built-in functions or libraries for efficient solutions. Overall, this article serves as a valuable resource for both beginners and experienced Java developers. By enhancing our understanding of loops and adopting best practices, we can improve our coding skills and efficiency in Java development.
Start optimizing your loop performance and improving your coding skills today!
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.