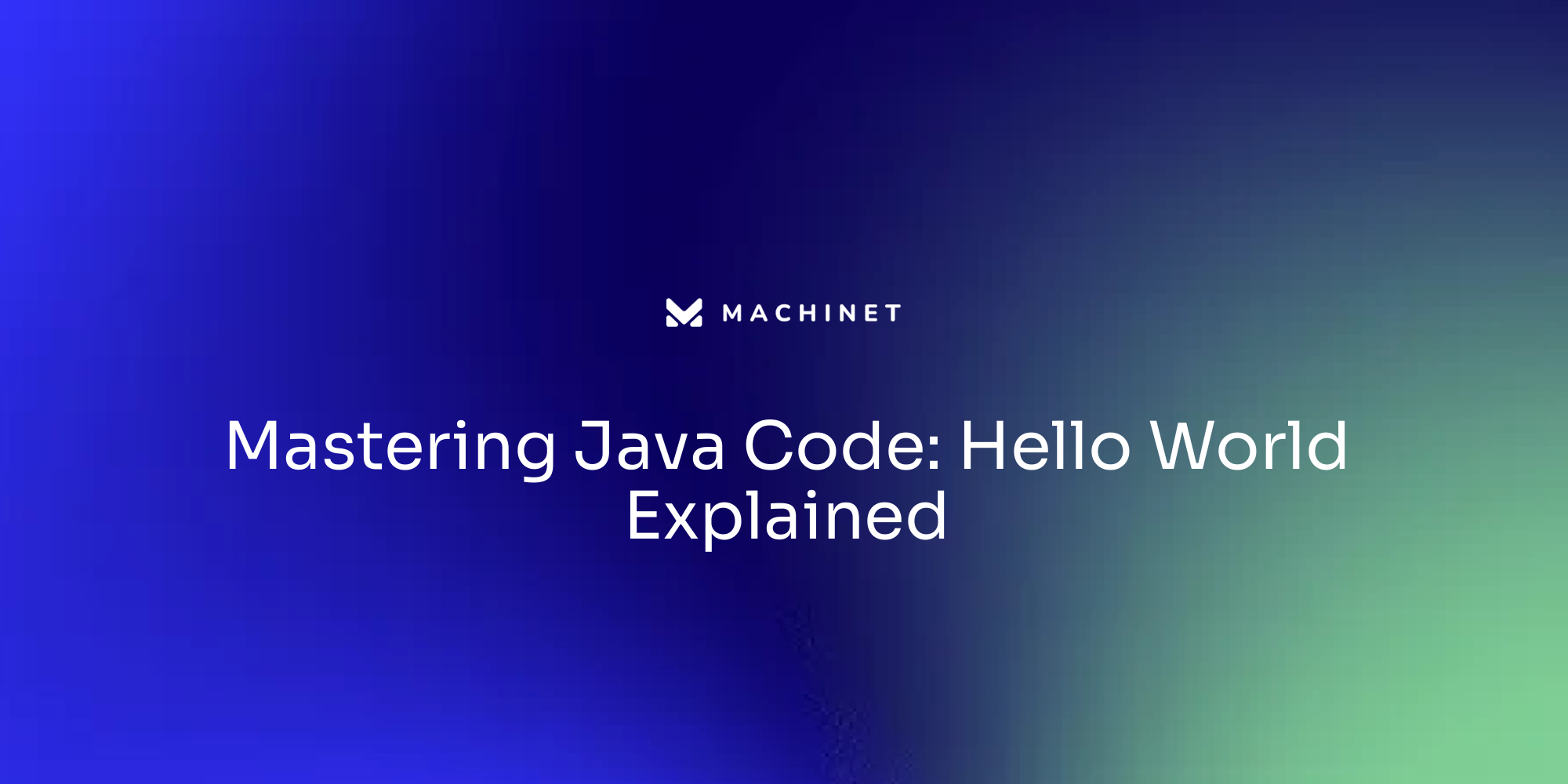
Introduction
Java is a powerful and widely used programming language that requires the right tools and understanding to write efficient and effective code. In this article, we will explore the basic requirements for writing Java code, understand the concept of Java classes, delve into the main method and its significance in Java programs, explain the System.out.println() statement, and learn how to compile and run a Java program. We will also provide a step-by-step guide to writing a Hello World program, discuss key concepts such as public, static, and void, and offer troubleshooting tips for common mistakes.
So, whether you're a beginner or an experienced developer looking to enhance your Java skills, this article will provide valuable insights and guidance to help you succeed in the world of Java programming.
Basic Requirements for Writing Java Code
Before you dive into writing programming language, it's essential to have the right tools and understand the process. To begin, you'll need the Development Kit (JDK) for the programming language, which is the cornerstone for any developer. It's more than just a set of libraries; it's the very framework that allows you to compile and run Java-based applications.
Next, an Integrated Development Environment (IDE) like Eclipse, IntelliJ IDEA, or NetBeans can significantly streamline your coding experience. These powerful platforms provide comprehensive facilities for software development, including a source text editor, automation tools, and a debugger.
For those who favor simplicity, a text editor such as Notepad++ or Sublime Text is sufficient for writing in the Java programming language. These editors are lightweight and often preferred for smaller projects or quick edits.
Understanding the process of writing and executing Java programs is crucial. At first, you write your programming instructions in a .java
file. The compiler for the programming language, which is part of the JDK, then converts your program into bytecode by generating a .class
file. This bytecode is the language spoken by the Java Virtual Machine (JVM), making your programming instructions platform-independent and ready to execute anywhere.
As you start programming, keep in mind that writing a program is not only about typing lines of instructions; it's about solving problems through the design of algorithms. For example, if tasked with calculating the area of a circle, you would first outline the steps required in an algorithm, which could be expressed in natural language or pseudocode. This approach helps to crystallize your thoughts and plan your structure in advance.
Embracing significant variable names is another essential aspect for clarity and maintainability in your programming. It allows anyone, even those without prior knowledge of your codebase, to quickly grasp the logic and purpose of different parts of your code.
Keeping up with the most recent advancements in programming is also crucial. OpenJDK, the open-source reference implementation of SE, releases a new major version every six months, with quarterly updates. These releases ensure that the programming language remains vibrant and up-to-date with current trends in technology.
In the context of the tech industry, keeping your skills sharp and current is essential. With trends rapidly evolving and the tech sector experiencing significant growth, leveraging the latest tools and programming languages like Java is essential for modern software development. As per recent insights, a vast majority of developers are adopting AI-integrated tools such as ChatGPT and GitHub Copilot, highlighting the integration of cutting-edge technology in everyday coding practices.
To summarize, commencing with programming in the language of Java entails equipping oneself with the appropriate resources, strategizing one's approach with algorithms, composing concise and manageable code, and keeping up-to-date with the latest updates and advancements in the Java environment.
Understanding Java Classes
Java is an object-oriented programming language, where classes act as the essential components. An encapsulation encompasses a collection of characteristics and actions, serving as a blueprint from which instances are created. Let's explore how to define a class, step by step:
- Begin by announcing the Category using the keyword 'class' followed by the name of the category you select.
- Outline the structure and capabilities of the objects created from this definition by specifying variables, procedures, and constructors within the body of this definition.
- Create instances of the defined type by utilizing the 'new' keyword.
Imagine we're developing a parking lot system. The class might represent a parking lot with properties such as a unique ID, number of floors, and slots per floor, while behaviors could include methods to park or remove vehicles.
The syntax of this programming language, reminiscent of C and C++, is engineered for clarity and organization, promoting a consistent coding style. The Development Kit (JDK) provides the essential toolkit for programming language development, including libraries and tools for application building.
Understanding the concepts in programming is crucial not only for code organization but also for aligning with the language's conventions. As emphasized by experts, using simple, descriptive names for classes and their components is paramount for readability and maintenance, particularly in complex systems.
As we witness the ecosystem of this programming language progress, with contributions from the worldwide developer community, it's crucial to stay updated with the most effective techniques and adhere to naming conventions like the JavaBeans standard. This commitment ensures that as the programming language continues to evolve to meet current requirements, your programming remains comprehensible and effective.
The Main Method in Java
Each program in the Java programming language starts with the 'main' function, which establishes the starting point for the application. As you write 'public static void main(String[] args)', you're setting up a gateway for the programming language environment to enter and start interpreting your code. The main
function is universally acknowledged by the JVM as the initial touchpoint of any programming application. Here's a closer look at each piece of this pivotal method:
public
: This keyword makes themain
method accessible from anywhere, ensuring the JVM can invoke it regardless of the class it resides in. 'By declaring thestatic
keyword, you're indicating to the programming language that this function can be invoked without the need to create an instance of the class - it is associated with the class itself.'.void
: This indicates that themain
routine will not return any data once it completes its execution.main
: The conventional name recognized by the JVM as the starting point of the program.String[] args
: Theargs
array captures any command-line arguments passed to the program, allowing users to influence the program's behavior when it's run.
Comprehending the structure and purpose of the main
function is not just academic; it's a fundamental aspect of Java that aligns closely with the very essence of object-oriented programming (OOP). In OOP, everything revolves around creating and manipulating objects, and the main
function is where this action begins, setting the stage for classes to be instantiated and various functions to be invoked.
Reflecting on the joy of running your first 'HelloWorld' program, remember that it's not just about getting the code to execute—it's about laying the groundwork for all the complex applications you'll develop in the future. As you become more acquainted with Java and its object-oriented attributes, you'll understand the importance of the main
function more with each program you write.
The 'main' function is similar to the starting point in a recipe; it's where you initiate the process of executing a well-structured set of instructions that lead to a gratifying outcome, much like the initial steps in calculating the area of a circle. Just as a recipe guides the cook through each step towards a delicious dish, the 'main' function guides the JVM through the execution of a program, ensuring a successful and error-free run.
When studying the language, it is crucial to grasp that the main
method is more than just a function—it's the cornerstone upon which all applications are built. So every time you write it out, you're not just starting a program; you're embracing the very philosophy that underpins programming in this language.
System.out.println() Statement Explained
In programming, one of the basic tasks is to show information to the user, which is where the System.out.println()
command comes into play. This command prints the specified message to the console, providing an essential way for developers to communicate output or debug issues. For example:
java
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
In the snippet above, System.out.println()
is invoked to output the greeting 'Hello, World!'. This simple yet powerful tool is part of the standard output class System
and is instrumental in the initial stages of learning programming and throughout the development process. By using test cases, developers can ensure that their System.out.println()
statements are outputting the correct information under various conditions, which is vital for both functionality and security, particularly in environments where precision and reliability, such as in banking systems or embedded systems, are non-negotiable. Furthermore, as the digital landscape progresses with a shift towards cloud computing and the continuous advancement of programming technology, mastering the effective use of System.out.println()
plays a crucial role in maintaining and scaling complex applications.
Compiling and Running a Java Program
Creating and executing a program involves several key steps that transform your written instructions into a form that your computer can understand and run. First, you'll need to create your Java program using a text editor or an Integrated Development Environment (IDE). Once your program is prepared, you save it with a .java
extension, like HelloWorld.java
.
The next step is to compile your program. This is done using the javac
command in the terminal or command prompt. Go to your program's directory and enter javac HelloWorld.java
. This compilation process is crucial as it turns your human-readable programming language code into bytecode, which is represented as a .class
file.
Finally, to run your program, you'll execute the bytecode using the java
command followed by the class name without the .class
extension, such as java HelloWorld
. This step starts the Runtime Environment (JRE), which interprets the bytecode and executes your program.
Comprehending this procedure is crucial, as it guarantees the seamless implementation of programming language programs across diverse computing environments, embodying the programming language philosophy of 'Write Once, Run Anywhere'. This concept is a cornerstone of the programming language's design, allowing programs like your Hello World
application to run on any device that supports it without needing to rewrite the code.
For example, the programming community recently demonstrated its capability to handle large-scale data processing. A challenge to process temperature data from a billion-row text file was met with enthusiasm, showcasing how a programming language can manage extensive datasets efficiently. Participants included a variety of responses and solutions, contributing to a vibrant ecosystem of developers and technologists.
Truly, the journey of Java from source code to execution is a testament to the language's robustness and the crucial role of the Java Compiler in ensuring high-performance applications. By understanding each step from scripting to executing your programming language, you position yourself to effectively participate in challenges and contribute to the evolving landscape of development, as seen in community initiatives and continuous improvements to the language.
Step-by-Step Guide to Writing a Hello World Program
To create a Java Hello World program, you begin by launching an integrated development environment (IDE) or a text editor of your choice. Next, initiate a new file and name it HelloWorld.java
. The essence of the program lies within these lines of code:
java
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
Once you've inputted the code, save the file. Proceed by opening a command line interface and navigate to the directory containing your new programming file. This is the place where compilation happens, transforming your readable Java program into bytecode understood by the Java Virtual Machine (JVM), achieved by executing 'javac HelloWorld.java'. After successful compilation, the program is ready to run. You bring it to life with the command java HelloWorld
, and the console greets you with 'Hello, World!'
This process isn't just about following steps; it's embodying the rich history and evolution of the programming language since its inception nearly three decades ago. It's also about understanding the foundational practices of programming, where designing algorithms is key—even for something as simple as printing out a greeting to the world. This method of problem-solving in code development is what forms a strong and effective program, and it's a testament to the resilience and adaptability of the programming language that continues to be essential in modern software development, despite the rise of newer languages.
Understanding Key Concepts: public, static, void
When embarking on programming in the language, it's essential to grasp the significance of certain keywords such as 'public', 'static', and 'void', especially when crafting the entry point of your applications. The 'public' keyword is an access modifier ensuring that the main function is accessible from any other entity, not just the one it resides in. This is important because the main function serves as the gateway for the Java Virtual Machine (JVM) to begin executing your application. The 'static' keyword attaches the main function directly to the class, signifying that it can be called without creating an instance of the class, which is crucial since at the start of your application, there are no objects yet to call functions on. Lastly, 'void' indicates that the main method will not return any data; its purpose is to govern the flow of execution, not to supply output. Together, these keywords form the foundation of an application, allowing the 'HelloWorld' program, a rite of passage for developers, to run seamlessly ensuring that the foundational setup is correct. Remember, the main objective of programming is clarity and maintainability. By comprehending and utilizing these keywords effectively, you lay the foundation for writing readable and functional programming code.
Common Mistakes and Troubleshooting Tips
As a developer, you're likely to encounter a few hiccups along the way. Let's talk about some common stumbling blocks and how to navigate them:
-
Syntax Errors: These are the 'grammar' mistakes of Java, like missing semicolons or mismatched parentheses. They're usually easy to fix once you spot them, so keep a keen eye on the structure of your program.
-
Logic Errors: Have you ever written a program that just doesn't perform as intended? That's a logic error. It's time to play detective - review your algorithms, check your assumptions, and make sure everything adds up.
-
Debugging: Embrace the debugging tools in your Integrated Development Environment (IDE). They're like a GPS for navigating through your program, helping you pinpoint where things go awry.
-
Stack Overflow Errors: If you're seeing 'StackOverflowError', think of it as a red flag that you might be in a loop-de-loop. This could be due to infinite recursion or too many method calls - a common challenge when dealing with concurrency and ensuring data consistency across multiple users.
Remember, clear code is key. Complexity might seem impressive, but it's the enemy of maintainability. Code should be a clear map for others to follow - not a maze. Keeping this in mind will not only help you avoid errors but also make debugging a breeze.
Encountering a 'NullPointerException'? Imagine a sticky note that's supposed to lead you to an object, but it's blank. That's what accessing a null reference in a programming language is like. To avoid this common programming pitfall, ensure your references point to actual objects.
Always start by understanding your data. It's the foundation upon which all your coding is built. Without a solid grasp of your data, you might miss out on key insights or make incorrect assumptions that can lead to errors down the line.
And remember, even the best of us run into bugs, especially when scaling up to distributed systems and juggling multiple concurrent users. Tools like Amazon Q Developer can be invaluable in diagnosing and fixing those tricky distributed system bugs.
Keep these tips in hand, and you'll be well on your way to smoother Java development.
Conclusion
In conclusion, writing efficient and effective Java code requires the right tools and understanding. The basic requirements include having the Java Development Kit (JDK) and an Integrated Development Environment (IDE) or a text editor. Understanding the journey of Java code from writing to execution is crucial, as it involves writing code, compiling it into bytecode, and running it on the Java Virtual Machine (JVM).
Java classes are the fundamental building blocks in object-oriented programming. They encapsulate properties and behaviors and promote readability and maintainability through meaningful names.
The main method in Java serves as the entry point for the application, allowing class instantiation and method invocation.
The System.out.println() statement is a fundamental tool for displaying output and aiding in debugging and feedback.
Compiling and running a Java program involves several steps, including writing code, compiling it into bytecode, and executing it on the JVM. This process ensures platform independence.
Writing a Hello World program in Java involves creating a file, defining the code, compiling it, and running it. This process emphasizes the importance of algorithm design.
Understanding key concepts like 'public', 'static', and 'void' is crucial for crafting the entry point of applications and writing readable code.
Common mistakes in Java programming include syntax errors, logic errors, and null pointer exceptions. Paying attention to code structure and utilizing debugging tools can help avoid these errors.
In conclusion, by following the basic requirements, understanding Java classes, the main method, the System.out.println() statement, and the process of compiling and running Java programs, developers can succeed in writing efficient and effective Java code.
Learn more about crafting the entry point of applications and writing readable code in Java.
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.