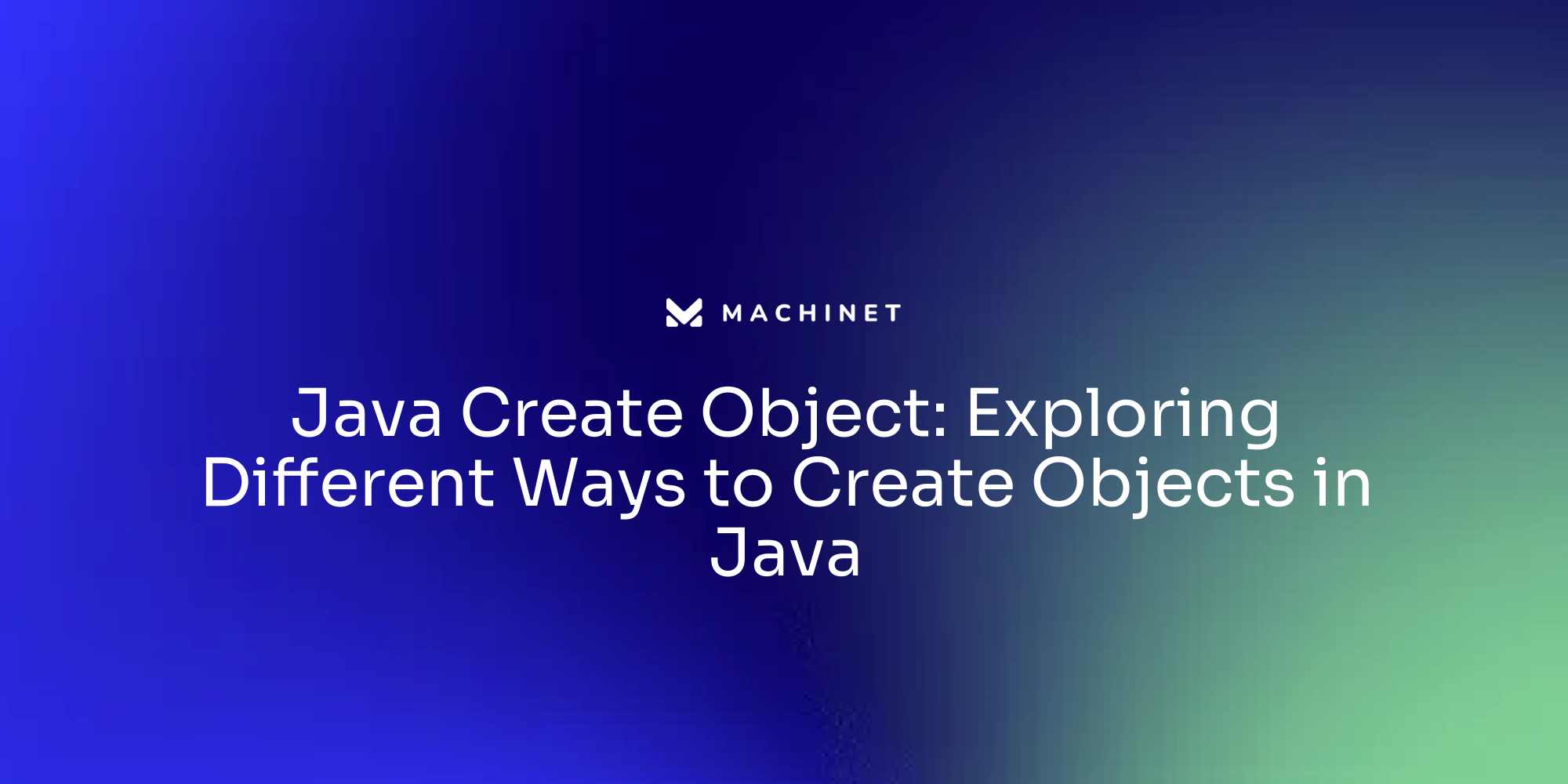
Introduction
Java, as an object-oriented programming language, relies heavily on the concepts of classes and objects. Classes serve as blueprints for objects, defining their attributes and behaviors. Objects, on the other hand, are instances of classes, created with specific values for their attributes, enabling them to interact with other objects.
In this article, we will explore the fundamentals of classes and objects in Java. We will discuss how classes provide a structured framework for organizing software programs and how objects encapsulate data and functionality. Additionally, we will delve into various ways to create objects in Java, such as using the 'new' keyword, the Class.forName() method, the clone() method, and the newInstance() method.
By understanding the creation and management of objects and classes in Java, developers can build software that meets industry standards and is adaptable to future changes. The principles of object-oriented programming provide a structured approach to software development, fostering reusable and adaptable code.
Join us as we explore the world of classes and objects in Java, uncovering the power and flexibility they bring to software development.
Understanding Classes and Objects in Java
At its essence, the programming language Java is based on objects, which considers nearly everything as a form of data. This approach is a foundation for a programming language, providing a framework for structuring software programs. Every element in programming language is formed from a category, which is essentially a plan containing the element's qualities and actions. For example, in the context of a banking application, an account would be a entity, defined by a structure with characteristics such as account number and balance, and actions such as deposit and withdrawal.
In Java, a template defines the structure of an entity, providing information about its variables (state) and functionality (operations). Consider a structure as a blueprint for a house, where each house constructed from that blueprint is an entity with its distinctive features, yet sharing the same architectural design. Classes encapsulate the data for the entity and the methods to manipulate that data, providing a high level of abstraction.
The connection between entities and categories is essential in Java. Entities are instances of categories, formed with particular values for their characteristics, empowering them to communicate with other entities in a purposeful manner. The condition of an entity refers to its current state, determined by the values of its attributes at any given time. It's the unique data that differentiates one object from another, even if they stem from the same class.
The object-oriented nature of this programming language emphasizes readability and organization, inherited from its C and C++ ancestry. This allows the development of complex software systems that are easier to manage and extend over time. The adoption of this programming language remains robust, despite the introduction of newer languages, thanks to continuous updates and enhancements that keep it competitive.
Comprehending the formation and administration of entities and categories is crucial, not just for creating software that meets the rigorous requirements of sectors like banking but also for sustaining and improving current systems. The principles of object-oriented programming provide a structured way to approach software development, fostering code that is both reusable and adaptable to future changes.
Ways to Create an Object in Java
'The role of Java as a cornerstone in the realm of Object-Oriented Programming (OOP) is beyond dispute.'. It's a programming paradigm that shapes our digital interactions by simulating entities, akin to nouns in our language, which embody real-world entities with their own data and behavior. These items, or entities, are the core of the programming language, serving as the fundamental components of our software applications, much like Lego pieces that join together to form a intricate structure.
To animate these items in Java, we must first establish a blueprint referred to as a Class. This blueprint outlines the characteristics and behaviors of a thing, known as attributes and procedures, respectively. When we create an object from a category, we're essentially generating a new entity with its own state, defined by its attributes, and capabilities, as defined by its methods. For instance, consider a Person class with name and address attributes, which could then be inherited by Employee and Customer subclasses, promoting code reusability and modularity.
Oracle reports that an astonishing 90% of Fortune 500 companies depend on the programming language, which attests to its enduring importance in the tech sector. As the programming language continues to evolve, with contributions from the vibrant developer community, it remains at the forefront of programming languages. Sharat Chander, senior director at Oracle, expresses gratitude for the community's role in maintaining the vitality of the programming language. This ongoing innovation includes proposals for new JDK enhancements that aim to further streamline creation and management within applications written in the programming language.
Understanding the creation mechanisms and class relationships in the Java programming language is more than just a technical requirement; it's about embracing a mindset where entities are not just code but represent a meaningful concept with real-world parallels. Eleftheria, an experienced business analyst, underscores the power of OOP in her writings, demystifying complex technical concepts into relatable content. As you explore the world of programming, keep in mind that every instance you generate is not merely a data structure, but a representation of an entity that could exist in the real world.
Using the 'new' Keyword
Developing Java entities is a fundamental ability in software development. To create an instance, developers usually employ the 'new' keyword followed by invoking the class constructor, which establishes the initial state and allocates the required memory. For example:
java
MyClass obj = new MyClass();
This line of code not only reserves space in the heap for 'obj' but also invokes the constructor of 'MyClass'. It's important to mention that each entity can contain its own set of variables and methods, which enables the creation of complex and dynamic software systems. The practice of object creation is just one aspect of understanding the robust capabilities of a language renowned for its performance, control, and flexibility. As you explore further into the features and frameworks of this programming language, you'll uncover a diverse ecosystem created to enhance efficiency and adapt to various project complexities.
Using Class.forName(String className) Method
Java's dynamic nature allows for the flexible loading and instantiation of objects at runtime, which can be particularly useful when dealing with entities that aren't known until the program is executed. The Class.forName(String className)
approach illustrates this, by allowing the dynamic loading of a category based on its name, which is given as a string argument. For instance, consider the following code:
java
Class<?> obj class = Class.forName("com.example.MyClass");
MyClass obj = (MyClass) objClass.newInstance();
In this snippet, objClass
is a reference to the Class
object associated with com.example.MyClass
. The newInstance()
function is then utilized to create an instance of this class. This approach follows the naming conventions of the programming language, enhancing readability and maintainability. The approach plays a crucial role in scenarios where applications need to dynamically adapt to new plugins or modules without the need for recompilation.
Using Class.forName()
aligns with the principle of encapsulation in the programming language, as it allows the creation of objects from classes that may not be accessible via traditional methods. This technique is also supported by the fact that most programming language applications follow a predictable startup sequence, enabling the dynamic assembly of applications at runtime. It's an example of the expressive power developers have, allowing the construction of flexible and scalable applications that can respond to changing requirements or environments.
Using clone() Method
In Java, the clone()
function is a mechanism to replicate an existing entity, generating a new instance that preserves the original's condition. It's crucial to understand that this method generates a shallow copy. This implies that while primitive fields of the cloned element are precisely replicated, the references still indicate to the same instances as those in the original, possibly resulting in shared mutable state between the two elements. For example:
java
MyClass obj1 = new MyClass();
MyClass obj2 = obj1.clone();
It's important for developers to recognize the implications of shallow copying, especially when dealing with mutable objects that could inadvertently affect both the original and cloned instances. This understanding is essential for maintaining clean code, which, as defined by Robert C. Martin, should be expressive and straightforward, minimizing the risk of unintended side effects. Additionally, software reliability is bolstered by clear and maintainable code, aligning with Michael Feathers' perspective that code should appear as if it was written with care.
Using newInstance() Method
Generating instances in Java is a fundamental aspect of programming, and the newInstance()
method offers a dynamic approach to instance creation. Unlike the 'new' keyword, which statically creates entities, 'newInstance()' is used when you need to instantiate entities at runtime without knowing their exact type beforehand. For example:
java
Class<?> objClass = MyClass.class;
MyClass obj = (MyClass) objClass.newInstance();
Here, newInstance()
is invoked on a Class
object representing MyClass
, and the result is cast to MyClass
. This technique is particularly useful in scenarios where the class to be instantiated is determined during program execution. It is a well-liked approach used in frameworks that depend on runtime configuration to generate entities, such as those dealing with testing or modular applications. Moreover, this approach supports the principles of Object-Oriented Programming (OOP) and the adherence to SOLID design principles, by allowing for more flexible and maintainable code structures.
Constructors and Object Initialization
Constructors are the foundation of entity initialization in programming, serving as special methods invoked during the creation of an entity. These constructors are responsible for establishing the initial state of an entity by initializing its attributes with default or specified values. As constructors bear the same name as their class and return no value, their role is singular but significant. They ensure that a subject is in a valid state before it is used, which is essential for maintaining the integrity of the codebase and preventing runtime errors. The lack of a constructor would leave entities in an uncertain state, rendering the code unpredictable and challenging to debug. Therefore, mastering the art of efficiently implementing constructors is crucial in the realm of object-oriented programming, as it improves code efficiency, simplifies the creation of instances, and contributes to a more transparent and maintainable software architecture.
Common Practices and Best Practices for Creating Objects
Generating instances in the programming language is an essential undertaking for software engineers, but it is vital to tackle this procedure with a thorough comprehension of optimal techniques, which enhance both effectiveness and code management. With Object-Oriented Programming (OOP) at the heart of the language, where blueprints are used for objects, developers create instances that encapsulate data and methods into a single entity. For instance, a 'Person' class could include properties like 'name' and 'age,' with capabilities such as 'walk()' and 'talk().'
To ensure the code remains clear and maintainable, adhering to standard naming conventions is essential. Avoiding cryptic acronyms like 'Ctr,' which can stand for multiple terms, and instead opting for explicit names such as 'Customer,' contributes to code readability and ease of understanding. This practice follows the naming convention for components, which is widely accepted in the development of applications using the Java programming language.
Moreover, crafting your code to meet specific project requirements from the outset is a strategic move. Take, for example, the creation of an application for a parking lot system. It should begin with defining basic requirements such as a unique parking lot ID, the number of floors, and the allocation of slots on each floor. This structured approach to entity creation ensures that your programming components align perfectly with the intended functionality of the application.
Remember, the primary purpose of code is not to show off technical prowess but to be comprehensible and maintainable. As we step into modern coding practices, simplicity and readability take precedence, avoiding complexity for the sake of it. This mindset is imperative when dealing with objects in Java, as it leads to software that is easier to debug, maintain, and extend, which benefits all stakeholders involved in the project.
Conclusion
In conclusion, understanding classes and objects in Java is crucial for building reusable and adaptable software. Classes serve as blueprints, defining attributes and behaviors, while objects encapsulate data and functionality.
Creating objects in Java can be done using the 'new' keyword, Class.forName() method, clone() method, or newInstance() method. Each approach offers different ways to instantiate objects and manage class relationships.
Constructors play a significant role in object initialization, ensuring objects are in a valid state before use. Effective implementation of constructors enhances code efficiency and maintainability.
Following best practices, such as adhering to naming conventions and structuring code to meet project requirements, is essential for creating readable and maintainable code. Simplifying code and prioritizing readability over complexity leads to easier debugging, maintenance, and extension of software.
By understanding object and class management in Java, developers can build industry-standard software that is adaptable to future changes. Object-oriented programming principles provide a structured approach, fostering reusable and adaptable code.
In summary, mastering the fundamentals of classes and objects empowers Java developers to create robust and scalable applications, meeting industry standards and facilitating future development.
Master the fundamentals of classes and objects in Java to build reusable and adaptable software!
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.