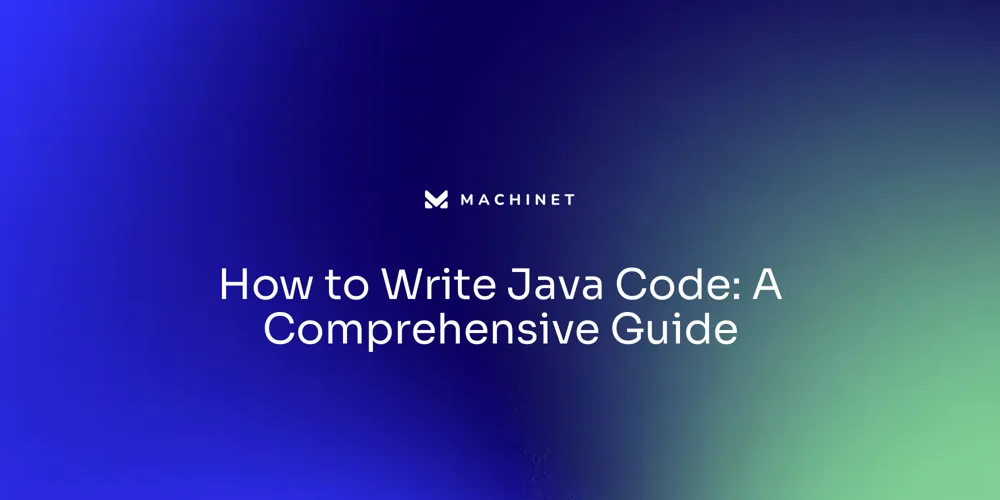
Table of Contents
- Setting Up Your Java Environment
- Basic Java Syntax and Data Types
- Functions and Methods
- Object-Oriented Programming in Java
- Error Handling and Debugging
- Advanced Java Concepts: Multithreading and Networking
- Best Practices for Writing Efficient Java Code
Introduction
Setting Up Your Java Environment
Embarking on Java programming begins with the essential step of setting up your development environment. Here's how to get started: 1.
Install Java Development Kit (JDK): Acquire the latest JDK from the official Oracle site. This kit is a collection of tools for developing and testing Java applications.
- Configure Your IDE: Choose and set up an Integrated Development Environment (IDE) that supports Java.
Some of the most popular IDEs are Eclipse, IntelliJ IDEA, and Visual Studio Code, each offering robust features for efficient coding. 3.
Verify Java Installation: To confirm that Java is installed correctly on your device, open a terminal or command prompt and enter java -version
. Successful installation will display the version of Java currently running. Java's robust platform, introduced by Sun Microsystems in 1995, is crucial for numerous applications and websites. Its cross-platform compatibility, encapsulated in the 'Write Once, Run Anywhere' principle, ensures that Java code can be executed on any device with Java support, bypassing the need for recompilation. As a high-level, class-based, object-oriented language, Java stands out for its minimal implementation dependencies, speed, security, and reliability, catering to diverse development needs from mobile apps to large-scale enterprise solutions. Understanding these Java fundamentals is the first stride in leveraging its potential to create versatile and dependable applications.
Setting Up Your Java Environment
Embarking on Java programming begins with the essential step of setting up your development environment. Here's how to get started:
1.
Install Java Development Kit (JDK): Acquire the latest JDK from the official Oracle site. This kit is a collection of tools for developing and testing Java applications.
- Configure Your IDE: Choose and set up an Integrated Development Environment (IDE) that supports Java.
Some of the most popular IDEs are Eclipse, IntelliJ IDEA, and Visual Studio Code, each offering robust features for efficient coding. 3.
Verify Java Installation: To confirm that Java is installed correctly on your device, open a terminal or command prompt and enter java -version
. Successful installation will display the version of Java currently running. Java's robust platform, introduced by Sun Microsystems in 1995, is crucial for numerous applications and websites. Its cross-platform compatibility, encapsulated in the 'Write Once, Run Anywhere' principle, ensures that Java code can be executed on any device with Java support, bypassing the need for recompilation. As a high-level, class-based, object-oriented language, Java stands out for its minimal implementation dependencies, speed, security, and reliability, catering to diverse development needs from mobile apps to large-scale enterprise solutions. Understanding the Java fundamentals is the first stride in leveraging its potential to create versatile and dependable applications.
Basic Java Syntax and Data Types
Java, an Object-oriented language created by James Gosling in 1990, is known for its readability and organization, drawing its syntax from C and C++. At the heart of Java programming lies the concept of classes and methods which structure a Java program into an organized collection of code blocks.
The Java Development Kit (JDK) is indispensable in this process, providing the necessary tools and libraries for Java application development across various platforms. Central to writing Java code is understanding its basic syntax and data types.
Variables are the fundamental building blocks, serving as placeholders for data that can be of various types, including integers for whole numbers, floating-point numbers for decimals, booleans for true/false values, and strings for text. Operators come in several forms, such as arithmetic for mathematical operations, assignment for setting values, comparison for evaluating conditions, and logical for boolean logic.
Control flow structures like if-else and switch statements, along with loops (for, while, do-while), are pivotal for directing the execution flow of your code. Moreover, methods encapsulate sets of instructions and adhere to the Single Responsibility Principle, ensuring each method is focused on a specific task, which simplifies the complexity of coding. Finally, input and output operations are essential for interacting with users, involving reading user input and displaying output via standard streams. Grasping these elements fortifies your foundation in Java, equipping you with the capabilities to tackle more intricate software development challenges. As per a global survey, an increasing number of developers are integrating AI tools like ChatGPT and GitHub Copilot in their workflow, reflecting the evolving landscape where a solid understanding of programming fundamentals remains crucial.
Functions and Methods
In the realm of programming, methods are the cornerstone of code organization and functionality, akin to mini-programs that perform specific tasks within a larger application. Java, an object-oriented language crafted by James Gosling in 1990, is a testament to this structure, drawing its syntax from C and C++, and offering readability and a clean coding style. Methods in Java are defined within classes and are fundamental for building complex software systems.
The Java Development Kit (JDK), a cross-platform toolkit, furnishes developers with the necessary tools and libraries for Java application development. Adhering to the Single Responsibility Principle ensures that each method addresses a distinct function, preventing complexity and maintaining focus. As the State of Developer Ecosystem 2023 report indicates, with insights from over 26,000 developers, the tech landscape is evolving, with 77% of developers using AI tools like ChatGPT.
This underscores the importance of understanding foundational concepts like method overloading, which allows for methods with the same name but differing parameters, and the proper use of return statements and parameters. Such knowledge is vital in a field where Java remains prevalent alongside languages like JavaScript, SQL, Python, and HTML/CSS. Proper commenting is also crucial for code clarity, serving as documentation and structuring, especially in complex algorithms or when delineating major program sections.
Object-Oriented Programming in Java
Java's object-oriented programming (OOP) is like building with Lego blocks—each piece, or 'object,' is a fundamental part of the overall structure. To master Java OOP, consider these key concepts:
1.
Classes and Objects: Imagine you're designing a software program to handle data about people. You wouldn't need every detail about a person, just the relevant data and how it's used.
In Java, a 'Class' is a blueprint for creating these 'objects,' similar to how a person might be represented in a program. A class outlines specific properties (like the parts of a human body) and functions (actions performed by the body parts).
- Inheritance: This allows you to form new classes based on existing ones, inheriting their properties and behaviors.
It's akin to a child inheriting traits from their parents. With inheritance, you can create a hierarchy of classes that share common traits, streamlining the process of building new functionalities.
- Polymorphism: It enables objects of different classes to be treated as objects of a common superclass.
Think of polymorphism as a way to use a general class type to reference specific subclass objects, allowing for more flexible code. 4. Encapsulation: This concept is about hiding the inner workings of a class and only exposing what's necessary through methods. It's like keeping the technical details of a car hidden under the hood, providing a cleaner interface for the user. By grasping these OOP principles, you'll be better equipped to write well-organized and maintainable Java code. As one expert says, 'OOP languages have become the standard for everything from web development to desktop applications,' making these concepts essential for modern software development. Armed with a solid understanding of classes, objects, inheritance, polymorphism, and encapsulation, you can approach Java programming with confidence and creativity.
Error Handling and Debugging
Understanding Java's error handling and debugging is essential for crafting resilient applications. Exception handling in Java is fundamental, and it's crucial to become proficient with try-catch blocks and the 'throws' keyword.
This allows developers to manage unexpected conditions and maintain application flow without crashing. Additionally, logging is not merely a good practice but an indispensable part of the development process.
Implementing logging frameworks such as Log4j enables developers to keep a detailed record of the program's operation, which is invaluable for tracking down elusive bugs. Remember, clear and effective communication, as highlighted by experts in software development, is as important in your code as it is with your team.
Furthermore, Integrated Development Environments (IDEs) offer a suite of debugging tools that can dramatically enhance your problem-solving capabilities. Utilizing breakpoints, stepping through code, and inspecting variables allows for a meticulous approach to dissecting and understanding how your code executes, which aligns with the principle of taking the simplest route to solve complex problems, as attributed to Bill Gates' perspective on developer efficiency. In the dynamic world of software development, the ability to swiftly identify and rectify issues is akin to navigating through a labyrinth with a clear map. Developers who master these techniques stand at the forefront, ready to tackle the challenges of today's technology landscape, defined by its unique organizational, technological, and historical contexts.
Advanced Java Concepts: Multithreading and Networking
Java's advanced capabilities, such as multithreading and networking, enable developers to craft sophisticated applications that can perform multiple tasks concurrently, communicate over networks, and handle synchronization to maintain data integrity. Multithreading allows developers to create multiple threads, or units of execution, within a single process. This concurrency can significantly improve application performance by allowing simultaneous execution of tasks, akin to taking a walk while talking on the phone—two tasks done concurrently but in parallel.
Java's networking APIs empower developers to build robust client-server applications that can exchange data over the network, a crucial aspect for real-time services like financial trading platforms, where milliseconds can mean the difference between profit and loss. Synchronization in Java ensures that when multiple threads access shared resources, they do so safely, preventing race conditions—a vital practice for maintaining the integrity and reliability of applications. By mastering these advanced Java concepts, developers can create applications that are efficient, responsive, and capable of handling the complexities of today's digital world.
Best Practices for Writing Efficient Java Code
Crafting Java code that is both efficient and maintainable requires adherence to several best practices. Firstly, embracing clear and descriptive names for variables and methods is essential, as it enhances code readability and understanding.
For example, using 'Customer' instead of an ambiguous acronym like 'Ctr' eliminates confusion and clarifies intent. Secondly, it is critical to follow Java coding conventions, which include consistent naming patterns, proper indentation, and strategic commenting.
Comments are not only for explaining what the code does but also for providing documentation and outlining the code's structure, thus aiding future development and maintenance efforts. Integrating tools like Machine, an AI plugin, can further streamline coding and testing processes.
Machine uses context-aware AI to assist in generating code, simply by describing the desired outcome. Additionally, its AI unit test agent can automatically generate unit tests for selected methods, significantly speeding up the testing phase.
Moreover, to prevent code bloating and to maintain simplicity, the Single Responsibility Principle (SRP) should guide the creation of methods. This principle asserts that a method should focus on a single task or responsibility, avoiding unnecessary complexity. Machinet's codebase-aware intelligence comes into play here as well, offering the ability to ask questions about general programming topics and your specific codebase directly from your editor, ensuring adherence to SRP and other best practices. Lastly, it is crucial to write reusable code to minimize duplication and to constantly seek ways to optimize performance by selecting the most efficient data structures and algorithms. By following these guidelines and leveraging tools like Machine, a developer can produce Java code that is not just functional but also clear, efficient, and easier to manage overtime.
Conclusion
In conclusion, setting up your Java environment is the essential first step in Java programming. This involves installing the JDK, configuring your IDE, and verifying the Java installation.
Understanding basic Java syntax and data types is crucial for writing code. Variables, operators, control flow structures, and methods provide structure to your programs.
Methods play a vital role in code organization and functionality. Adhering to the Single Responsibility Principle ensures focused methods and easier maintenance.
Object-oriented programming (OOP) is fundamental in Java. Understanding classes, objects, inheritance, polymorphism, and encapsulation allows for maintainable code.
Error handling and debugging are crucial for resilient applications. Exception handling, logging frameworks like Log4j, and debugging tools enhance problem-solving capabilities. Advanced Java concepts like multithreading and networking enable developers to create sophisticated applications that perform multiple tasks concurrently and communicate over networks. To write efficient Java code, follow best practices such as clear naming, coding conventions, strategic commenting, SRP implementation, reusable code, and optimizing performance with efficient data structures. By mastering these concepts and best practices, developers can leverage the full potential of Java to create versatile applications that meet today's technology demands.
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.