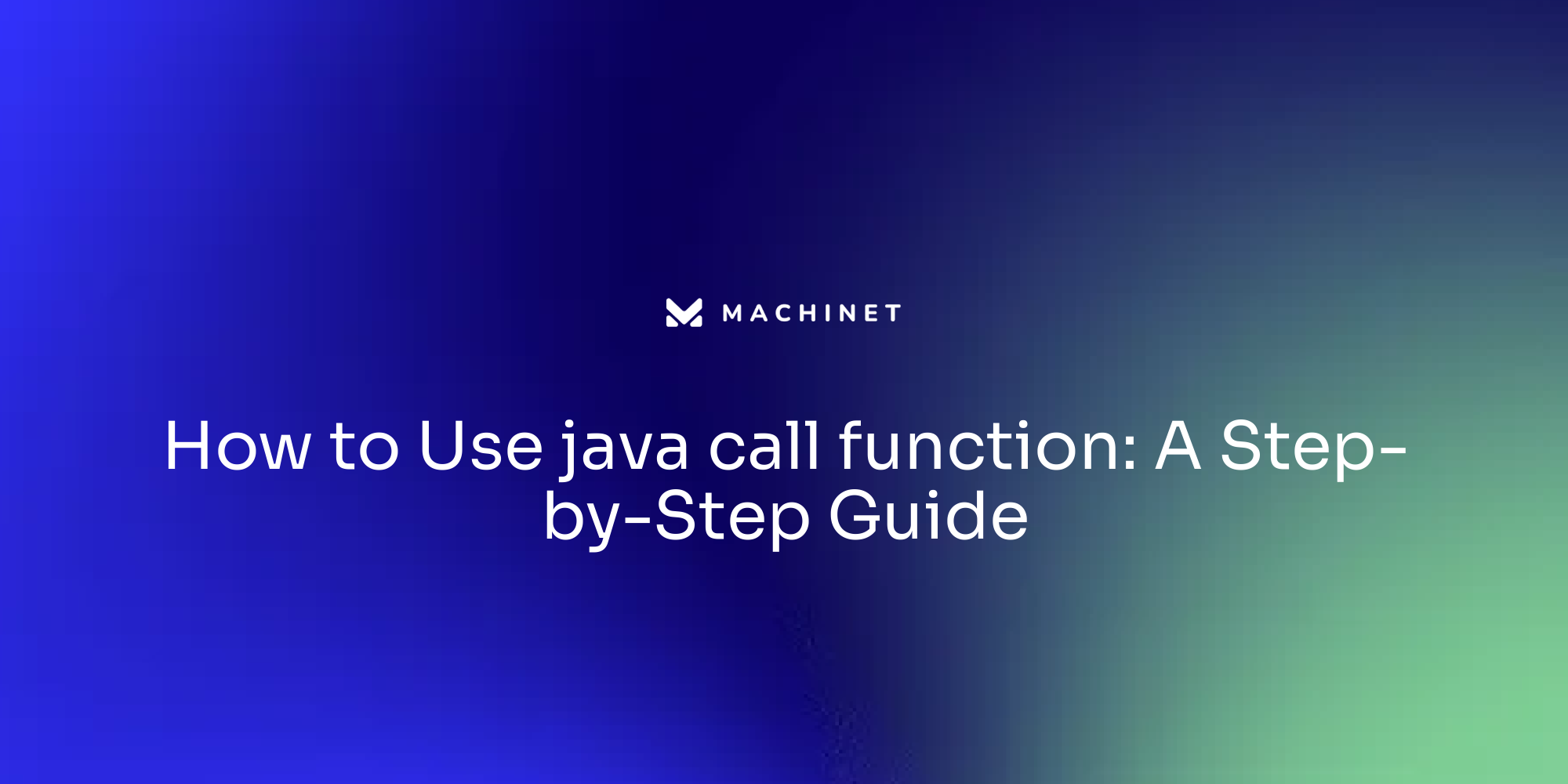
Introduction
Methods in Java are foundational to effective software development, offering a structured approach to organizing code, promoting reusability, and enhancing readability. By modularizing tasks into discrete methods, developers can manage and maintain complex codebases more efficiently. Each method encapsulates specific functionality, allowing for isolated testing and modification, thereby minimizing the risk of unintended side effects in other parts of the program.
This article delves into the significance of methods in Java, detailing their components, declaration, and usage. It also covers best practices for naming methods, understanding method parameters and return types, and troubleshooting common issues, providing a comprehensive guide for developers to harness the full potential of Java methods in their projects.
Why Use Methods in Java
Methods in Java play a crucial role in structuring programs, facilitating reuse, and improving readability. By breaking down tasks into smaller, manageable parts, approaches make understanding and maintaining the codebase substantially easier. Encapsulating functionality within functions enables developers to efficiently modify and test code, ensuring changes in one part do not adversely affect the rest of the program. This modular approach not only streamlines the development process but also enhances the overall quality of the software.
Components of a Java Method
A Java function is comprised of several essential components. First, the modifier, such as public
or private
, defines the accessibility of the function. Second, the return type indicates the kind of value that will be provided; this could be a primitive data type such as int
or boolean
, or an object type like String
or ArrayList
. The procedure name is another crucial aspect, and it should be descriptive to clearly communicate the procedure's purpose. Furthermore, the parameter list specifies the inputs that can be accepted, which are enclosed in parentheses and separated by commas.
Furthermore, the function body, enclosed in curly braces, includes the actual instructions that run when the function is invoked. 'This program can range from simple calculations to complex operations involving multiple classes and libraries.'. The modular approach of defining functions not only enhances code readability but also promotes reusability and easier debugging. For instance, user-defined functions encapsulate specific functionality that can be invoked multiple times throughout the application, thereby adhering to the DRY (Don't Repeat Yourself) principle.
Declaring a Method in Java
To declare a function in Java, you use the following syntax: returnType functionName(parameterType parameterName) { // function body }
. For instance, a straightforward approach that sums two integers might appear as follows: int add(int a, int b) { a + b; }
.
Breaking Down the Components
- access Specifier: Defines the visibility or accessibility of classes, methods, and fields within a program. Common access specifiers include
private
,default
, andprotected
. - returnType: The data type of the value that the function provides. If the approach does not return any value, the
void
keyword is used. - methodName: The title of the function, adhering to Java naming conventions.
- parameter: Input value that the function accepts.
These elements establish the basis of techniques in Java, allowing developers to produce reusable segments that execute particular functions. For example, predefined functions such as System.out.println()
and Math.max()
are part of the Java Class Library and can be utilized directly without any declaration.
This structure enables functions to perform their designated tasks efficiently when invoked, ensuring code modularity and reusability.
Understanding Method Parameters
Function parameters are essential for functions to accept input values, allowing dynamic behavior based on different inputs. Parameters are specified within the parentheses in the function declaration. For example, in void printMessage(String message)
, String message
is the parameter. When calling this function, an argument that corresponds to the parameter's category must be supplied.
Parameters in Java functions are optional, and a function can have zero or more parameters. Each parameter is declared with a specific data type and a name. For instance, in int add(int a, int b)
, int a
and int b
are parameters, allowing the function to accept two integer values. The technique then employs these values within its body to carry out its task.
Grasping function parameters is essential, as it allows for the development of adaptable and reusable code. They permit approaches to function on various data, improving overall capability and flexibility in software creation.
How to Call a Method in Java
To call a function in Java, you use the function name followed by parentheses. If the technique requires parameters, you place the necessary arguments inside the parentheses. For instance, invoking the add
function would look like this: int result = add(5, 3);
. This line runs the function and saves the return value in the variable result
. Comprehending how techniques function is essential for effective programming, particularly when managing intricate assignments or enhancing performance. Recent advancements in AI-based code assistants, such as GitHub Copilot, have made it easier to generate and comprehend function calls by providing accurate code snippets and context.
Understanding Return Types
'The output category of a function indicates the kind of value that the function will provide to the caller.'. If a technique is not intended to yield any value, its output classification is labeled as void
. For techniques that yield a value, the output classification must correspond to the data format of the value being provided. For example, a function defined as int getCount()
is anticipated to yield an integer.
Variable naming and output types that are not consistent can lead to confusion. For instance, a function named get Statistics
suggests that a collection will be returned. However, if this approach produces a single object or is void, it creates a discrepancy that should be clarified through proper documentation or renaming the function. Furthermore, techniques designed to verify conditions ought to provide a significant value signifying the success or failure of the validation procedure, or clearly document the result's storage place, instead of yielding void
without any clarification.
Properly aligning function names with their return types helps maintain code readability and reliability, ensuring that the function's purpose is clearly communicated.
Best Practices for Naming Methods
Effective naming of functions is essential for maintainability and readability in Java. Method names should be clear and descriptive, typically beginning with a verb and following camelCase conventions, such as calculate Total
or printReport
. This practice helps other developers swiftly understand the aim of the technique. Inconsistent naming conventions, like those seen when integrating Ruby on Rails (snake case) with JavaScript (camel case), can complicate the codebase and require additional manual case conversion. However, once standard naming conventions are uniformly applied, it significantly improves the efficiency of the development process, as observed in teams that implement automated case conversion.
Common Use Cases for Java Methods
Java functions are extremely adaptable and can be utilized in various situations to improve modularity and maintainability. They play a crucial role in performing calculations, processing data inputs, and encapsulating repetitive tasks. For example, utility functions for string manipulation, such as trimming whitespace or converting case, streamline text processing. Mathematical computations, such as determining the highest value in a collection or calculating factorials, are frequently organized in functions to encourage reuse of programming. Managing user input, another frequent scenario, includes techniques that validate and process input data, ensuring robustness and error handling in applications. Highlighting the modular aspect of techniques, developers can create code that is not only efficient but also simpler to read, understand, and maintain.
Troubleshooting Common Issues
'When dealing with techniques in Java, common issues such as mismatched parameter types, incorrect return types, or improper calls can arise.'. Examining function signatures thoroughly and making sure parameters are accurately transmitted is essential. For instance, a common error such as a [NullPointerException](https://javacodegeeks.com/2024/05/null-no-more-mastering-javas-most-common-error.html)
could happen if a variable isn't adequately initialized prior to a function call. Debugging tools, such as breakpoints and step-through debugging, can significantly simplify identifying these issues. By pausing execution and examining variable states, developers can pinpoint where mismatches or incorrect values occur. Moreover, understanding the flow of control through the application helps in tracing how data is passed and manipulated, ensuring methods behave as expected.
Conclusion
Methods in Java are integral to creating structured, maintainable, and reusable code. They allow developers to break down complex tasks into manageable segments, enhancing both readability and the ability to troubleshoot issues. By encapsulating specific functionalities, methods not only facilitate isolated testing but also promote a modular approach that leads to higher software quality.
The essential components of a Java method include modifiers, return types, method names, parameters, and method bodies. Understanding how to declare and call methods, as well as the significance of parameters and return types, is crucial for effective software development. Adhering to best practices in method naming and being aware of common use cases ensure that code remains clear and easy to understand.
Moreover, troubleshooting common issues related to method calls, parameter types, and return types is vital for maintaining code integrity. Utilizing debugging tools can aid in swiftly identifying and resolving problems, contributing to a smoother development process. Ultimately, mastering the use of methods in Java empowers developers to create robust applications that are both efficient and easy to maintain.
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.