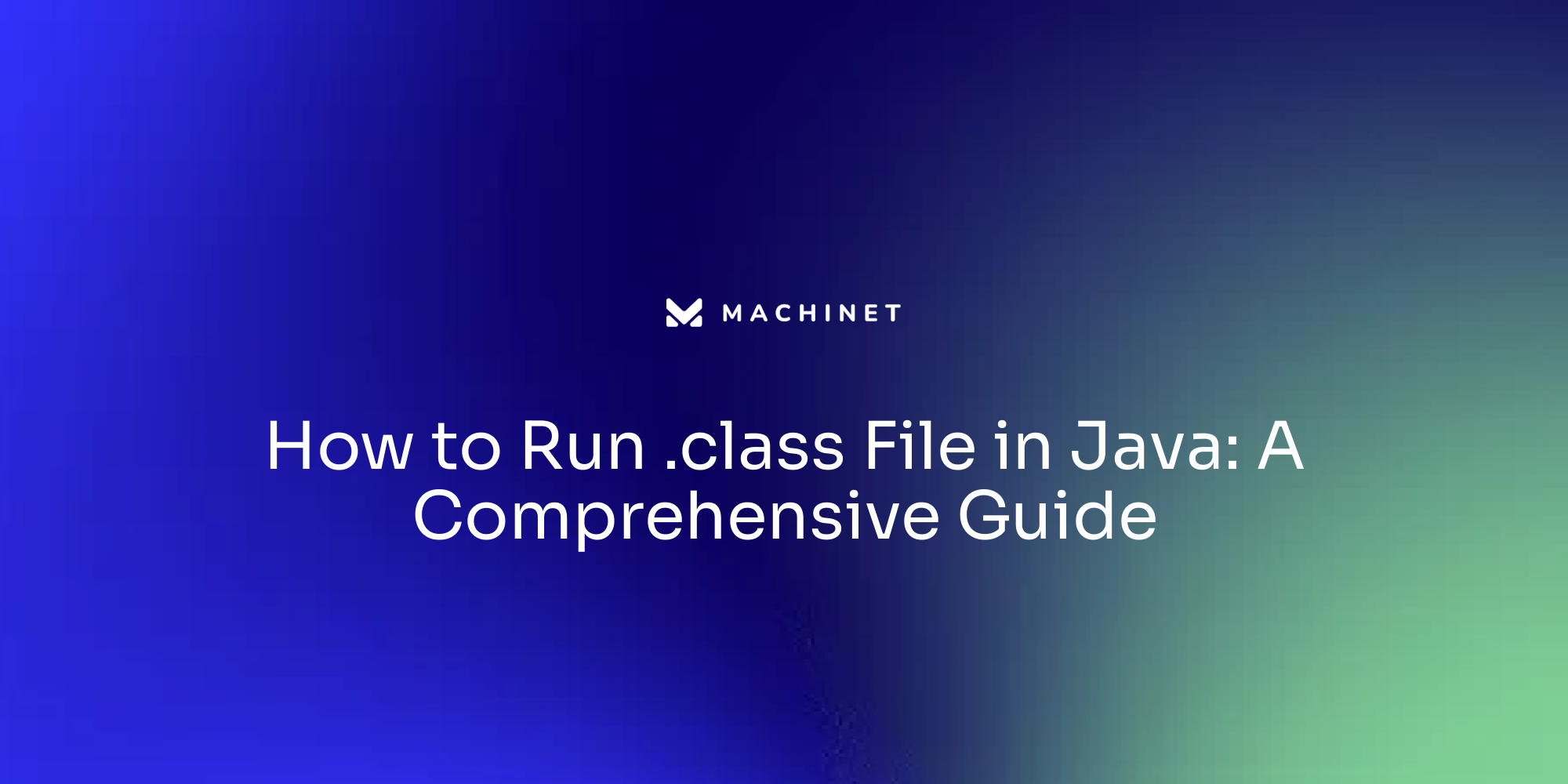
Introduction
Java, a cornerstone of modern computing, thrives on its ability to be versatile and platform-independent. At the heart of this capability lies the Java class files and bytecode, the compiled output of Java source code that the Java Virtual Machine (JVM) executes. Understanding the intricacies of these elementsβfrom the initial compilation process to running and troubleshooting the resulting filesβis crucial for any developer seeking to harness the full potential of Java.
This article delves into the detailed process of compiling Java code to create class files, running them from the command line, packaging them into JAR files for distribution, and troubleshooting common issues. Additionally, insights into the latest advancements in Java technology and best practices for optimizing performance are provided, ensuring that developers are well-equipped to build robust, scalable applications.
Understanding Java Class Files and Bytecode
Class files from the programming language are the compiled output of source material, containing bytecode that the Virtual Machine (JVM) executes. The compilation process starts with lexical analysis, where the source text is transformed into tokens. These tokens are categorized into identifiers, keywords, literals, and operators. Next, syntax examination ensures the program adheres to the language's strict syntax regulations, followed by semantic analysis that validates the program's meaning.
When you compile a programming project using the javac
command, it produces a .class
file for each class defined in the source code. This bytecode is platform-independent, allowing applications to run on any system with a compatible JVM. The bytecode is optimized to execute efficiently across different platforms without altering its output.
A noteworthy contribution to the ongoing development of the programming language was highlighted by Sharat Chander, senior director at Oracle, who emphasized the vibrant community's role in keeping the technology robust and evolving.
How to Compile Java Code to Create a .class File
To compile Java source code into a class file, follow these steps:
- Write your programming code in a text editor and save it with a
.java
extension. For example,HelloWorld.java
. - Open your command prompt or terminal.
- Navigate to the directory where your
.java
document is saved. - Run the command
javac HelloWorld.java
. This command compiles the programming file and createsHelloWorld.class
in the same directory.
Compiling programming instructions involves transforming human-readable syntax into bytecode, which the virtual machine can execute. This process is a critical step in the development lifecycle, ensuring that your applications are robust, scalable, and efficient.
Grasping the compiler's function can assist you in creating improved and more efficient programs. It performs several tasks, from lexical analysis to code generation, and optimizes the final bytecode for efficient execution. This ensures that the applications run smoothly on various platforms, leveraging the cross-platform capabilities of the programming language.
In real-world scenarios, you might encounter different JVMs or JDK versions, such as version 21. For instance, during a project transition to Spring Boot 3.x, developers faced challenges due to changes in security configurations, which often rendered existing guides obsolete. However, staying informed about the latest programming language releases can be advantageous. 'Version 21, for instance, introduced virtual threads that significantly enhance resource management and execution efficiency.'.
By following these steps and understanding the underlying mechanisms, developers can ensure their programming applications meet modern development needs and perform optimally. This approach aligns with best practices and keeps your development process streamlined and effective.
Running a .class File from the Command Line
To run a .class
file from the command line, follow these steps:
- Ensure the Java Development Kit (JDK) is installed on your system.
- Open your command prompt or terminal.
- Navigate to the directory containing the
.class
file. - Execute the command
java HelloWorld
(omit the.class
extension). This executes themain
method in theHelloWorld
type.
$ java HelloWorld
This method leverages the built-in class loaders of the JDK, ensuring a smooth execution process without needing to change application code or rely on additional tools like jlink
or jpackage
. This provides a foundation for continuous improvements in startup and warmup times, essential for optimizing performance. As noted by Sharat Chander, senior director, Product Management & Developer Engagement at Oracle, the ongoing momentum in the developer community keeps Java vibrant and evolving, ensuring that these processes remain efficient and effective.
Common Issues and Troubleshooting Tips
When running .class
files, you may encounter several common issues that can disrupt your development process. Here's how to troubleshoot them effectively:
- ClassNotFoundException: This occurs when the JVM cannot find the class definition for a given name. 'Ensure the group is included in the path and that you are using the correct ClassLoader.'. Missing JAR files or incorrect classpath settings can often be the root cause.
- NoClassDefFoundError: This error occurs when a type was present during compilation but not accessible at execution. Generally, this is caused by problems with the path or when types have been removed after compilation.
- No Main Method Error: Ensure that your type contains a
public static void main(String[] args)
method. This is the entry point for any Java application and is essential for the program to run. - ClassCastException: This exception occurs when attempting to cast an object to a type it is not an instance of. It frequently happens when the same type is loaded by different ClassLoaders, resulting in multiple definitions in memory. Ensure that classes are loaded by the same ClassLoader to avoid this issue.
- JDK Not Recognized: Ensure that the JDK is installed and the
JAVA_HOME
environment variable is set correctly. This configuration is crucial for the JVM to locate the necessary binaries.
Understanding these common issues can help you troubleshoot effectively and maintain a smooth development workflow.
Packaging .class Files into JAR Files for Distribution
To package your .class
files into a JAR (Java Archive) file for distribution, follow these steps:
-
Compile your Java programs: Begin by compiling your Java programs to generate
.class
outputs. Use thejavac
command for this purpose. -
Create the JAR archive: In your terminal, use the command
jar cf myapp.jar *.class
. This command packages all the.class
files in the current directory into a JAR archive namedmyapp.jar
. -
Run the JAR: To execute the JAR, use the command
java -jar myapp.jar
. This command runs the application contained within the JAR file.
Improving your application's startup time can significantly enhance performance. By leveraging tools like JReleaser, developers can streamline the creation of project releases, ensuring efficient deployment. "Additionally, improvements in integration with frameworks like Micronaut and compatibility with Quarkus 3.6, as seen in the latest JobRunr release, demonstrate the ongoing advancements in programming technology.". As stated by Oracle's senior director, Sharat Chander, the contributions from the programming community continue to advance the platform, making it essential for developers to remain aware of these updates.
Running a Java Class from a JAR File
To run a Java class from a JAR file, follow these steps:
- Ensure your JAR archive includes a
META-INF/MANIFEST.MF
entry that specifies the main class to execute. - Open your command prompt or terminal.
- Navigate to the directory containing the JAR file.
- Execute the command
java -jar myapp.jar
.
This straightforward approach does not require any modifications to the application programming, libraries, or frameworks. Additionally, it lays the foundation for future improvements in startup and warmup times, as most applications start up similarly each time they run. This method leverages the Hotspot JVM to optimize the application's code for peak performance without needing tools like jlink or jpackage.
Conclusion
Java class files and bytecode form the backbone of the Java development process, enabling platform-independent execution through the Java Virtual Machine (JVM). The compilation process transforms source code into bytecode, ensuring adherence to Java's syntax and semantics. This critical step not only facilitates robust and scalable applications but also underscores the importance of understanding Java's compilation mechanics to optimize performance.
Running and troubleshooting Java applications from the command line is essential for developers. By mastering commands like javac
for compiling and java
for executing class files, developers can efficiently manage their Java projects. Awareness of common issues, such as ClassNotFoundException
and NoClassDefFoundError
, allows for quick resolution and a smoother development workflow.
Additionally, packaging class files into JAR files for distribution enhances the deployment process. This method streamlines the execution of Java applications, particularly when combined with modern tools and frameworks that improve startup times and resource management. Staying updated with the latest advancements in Java technology is vital for developers, ensuring they leverage community contributions to drive their projects forward effectively.
In summary, understanding the intricacies of Java class files, compilation, execution, and packaging equips developers with the necessary skills to build efficient and high-performing applications in a continuously evolving landscape.
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.