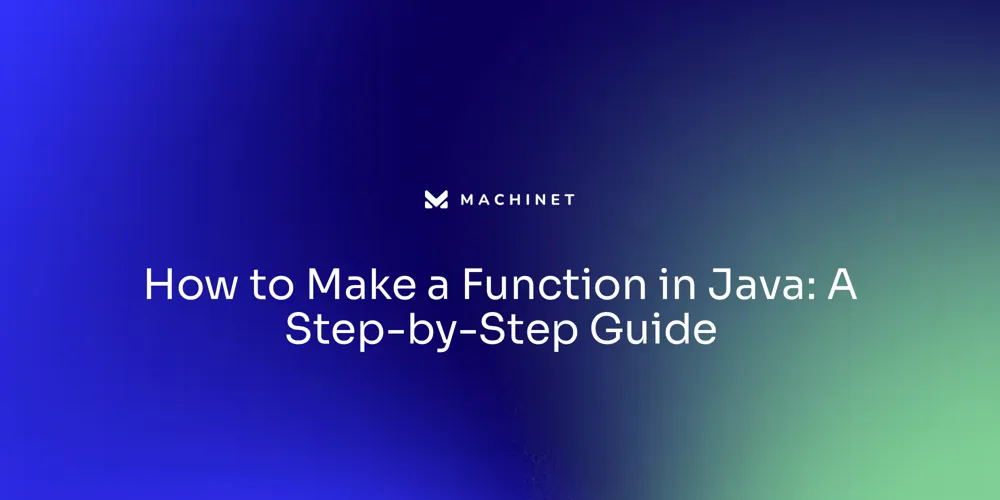
Table of Contents
- Step 1: Setting Up the Java Environment
- Step 2: Understanding Java Syntax and Semantics
- Step 3: Writing Your First Java Function
- Step 4: Testing and Debugging Your Java Function
Introduction
Embarking on Java development begins with setting up a conducive environment for your programming endeavors. This article will guide you through the process of setting up the Java Development Kit (JDK) and selecting a Java-friendly Integrated Development Environment (IDE). Once you have these essential tools in place, you'll be ready to start crafting Java applications.
Additionally, this article will cover the importance of understanding Java syntax and semantics, including variables, data types, control flow, and object-oriented programming principles. Furthermore, it will explore the process of writing your first Java function and testing and debugging your code to ensure its reliability and correctness. Whether you're a beginner or an experienced developer, this article will provide valuable insights into Java development best practices to help you succeed in the programming world.
Step 1: Setting Up the Java Environment
Embarking on Java development begins with setting up a conducive environment for your programming endeavors. This entails installing the Java Development Kit (JDK), not to be confused with the Java Runtime Environment (JRE) which is simply for running Java applications.
The JDK is vital for development, offering tools for compiling and debugging your Java code. Here's a streamlined process to get started:
1.
Obtain the latest JDK version from the Oracle website, ensuring to download the correct build for your operating system. 2.
Assign the JAVA_HOME environment variable to your JDK installation path, which informs your system where Java is located. 3.
Select a Java-friendly IDE like Eclipse, IntelliJ IDEA, or NetBeans, and configure it to recognize the newly installed JDK. After these initial steps, you're equipped to begin crafting Java applications.
If you're working with Ubuntu, there are comprehensive guides, complete with screenshots and video walkthroughs, to assist you in setting things up. Additionally, for MacOS users, OpenJDK installation is straightforward and empowers you to both run and develop Java applications seamlessly. Java's 'Write Once, Run Anywhere' philosophy is exemplified by its ability to operate across diverse platforms without recompilation—its speed, security, and reliability are suited for a range of applications, from mobile to enterprise solutions. OpenJDK, in particular, stands as the reference implementation of Java, open to contributions from the developer community, fueling innovation and ensuring security. Java's evolution is ongoing, with Oracle releasing new versions biannually, and every two years, an LTS version appears, bringing improvements in stability and performance. The introduction of Java 21 saw a surge in adoption, with a 1.4% uptake in applications within six months post-release, highlighting its growing relevance in the programming landscape.
Step 2: Understanding Java Syntax and Semantics
Java's evolution continues with the upcoming release of Java 21 on September 19, 2023, which introduces record patterns in switch blocks and expressions—a significant leap towards functional programming akin to Kotlin, Rust, or C#. This enhancement follows Java 9's slower pace, after which releases progressed every six months, demonstrating Java's rapid development over the past decade. As we prepare for these advancements, understanding Java's syntax and semantics becomes crucial.
Variables and data types remain foundational, with types such as int, double, boolean, and String requiring careful declaration and utilization. Control flow has been enriched, particularly with switch expressions introduced in Java 14 and the revolutionary Pattern Matching for Switch in Java 21, which may extend to primitive types in Java 23. This progress simplifies data manipulation, enabling more intuitive code through pattern matching, which aligns values with patterns containing variables and conditions.
Furthermore, Java's object-oriented nature necessitates proficiency in defining classes, creating objects, and employing methods—units encapsulating related instructions. These methods, adhering to the Single Responsibility Principle (SRP), should have one well-defined function to avoid complexity. Instance methods, particularly, are non-static and manipulate the object's state, using the 'this' keyword to differentiate between instance variables and parameters of the same name.
Invoked with the dot notation, these methods require an object instance. Java's parameter passing mechanism, often misunderstood as pass-by-reference, is strictly pass-by-value. When passing a primitive type, a copy of its value is transferred, leaving the original unaltered.
In contrast, passing an object reference copies the reference's value (memory address), allowing modifications to the object's properties within the method but not to the reference itself. This distinction is paramount for writing clean, maintainable Java code that is not only functionally correct but also comprehensible and adaptable. As we continue to explore Java's capabilities, the language's persistent popularity, supported by frameworks like Spring, and its top-three ranking, despite competition from languages like Kotlin and C#, is a testament to its enduring relevance and versatility in the programming world.
Step 3: Writing Your First Java Function
Embarking on your first Java coding adventure can be both exhilarating and challenging. Begin by crafting a new Java class in your preferred Integrated Development Environment (IDE), assigning it a name that captures its essence. Within this class, you'll establish a method, the heart of your function, where the logic takes shape.
The method's signature is crucial—it outlines the return type, the method's moniker, and the parameters required for operation. Delving into the method's body, you'll articulate the code necessary to bring your desired functionality to life. To enhance clarity and future-proof your work, consider annotating your code with comments that elucidate its purpose.
Upon breathing life into your function, it stands ready to be invoked from various corners of your application or to undergo rigorous testing in seclusion. Remember, compiling your Java code is a pivotal step before you set it in motion. This process not only equips you with a functional Java method but also aligns with the principles of clean Java code—ensuring readability, maintainability, and fewer bugs.
As Java continues to be a sought-after skill in the tech industry, with 915K job offers over 17 months, mastering this language opens doors to a plethora of opportunities. Whether you're solving complex problems like the 'Happy Number' or contributing to large-scale enterprise projects, Java's robustness and frameworks like Spring secure its place as a top programming language. Despite the rise of languages like Python, Java's stronghold in the market is undeniable, reinforced by the continuous evolution of projects like Babylon that aim to expand Java's reach to new programming paradigms.
Step 4: Testing and Debugging Your Java Function
Ensuring the reliability and correctness of Java functions is a multi-faceted process, involving strategic testing and debugging methodologies. To begin with, unit testing is essential. Each test case must have a clearly defined objective, which is critical for evaluating specific functionality or performance aspects of the software.
A well-structured test case includes a unique identifier (Test Case ID), a descriptive title, preconditions that must be met, specific input data, and a detailed guide on how to execute the test (Steps to Reproduce). Tools like Machine.net facilitate this process by automating unit test generation and providing best practices for structuring tests, isolating dependencies, and using effective assertions with Mockito. This approach not only accelerates development by speeding up validation of changes but also sets a high standard for code quality, contributing to a cleaner, more maintainable codebase.
When it comes to debugging, setting breakpoints is a widely used technique. It allows developers to pause the execution of code at strategic points, facilitating a thorough inspection of variable values and helping to pinpoint issues or unexpected behaviors. Machine.net enhances this process by offering resources like tutorials and FAQs that help developers optimize unit testing efficiency, including the use of Mockito for mocking dependencies.
Furthermore, their AI assistant and the 'Getting Started with Java Unit Testing' ebook serve as additional support for Java developers navigating complex debugging scenarios. Logging and error handling are indispensable for tracking the flow of execution and managing exceptions effectively. They provide a transparent view of the runtime behavior, which is invaluable when addressing complex bugs.
Machine.net underscores the importance of these practices by offering insights into best practices and techniques through their platform, thus aiding developers in instituting a reliable process for ensuring software health and longevity. In summary, by meticulously crafting test cases, utilizing breakpoints for inspection, and implementing robust logging and error management, developers can significantly bolster the integrity of their Java functions. This comprehensive approach is vital in a landscape where software is constantly evolving and where maintaining high-quality, bug-free code is paramount.
Conclusion
To succeed in Java development, it is important to set up the Java environment by installing the JDK and selecting a Java-friendly IDE. Understanding syntax and semantics, including variables, data types, control flow, and object-oriented programming principles, is crucial for writing clean and maintainable code.
Java's evolution brings advancements like record patterns in switch blocks and expressions. Its enduring popularity and versatility solidify its relevance in the programming world.
Writing your first Java function involves creating a class, defining a method, and annotating your code for clarity. Compiling your code before testing or running it is essential.
Mastering Java opens doors to numerous opportunities in the tech industry. Testing and debugging play a vital role in ensuring reliability.
Well-structured test cases evaluate functionality and performance, while breakpoints help pinpoint issues. Logging and error handling aid in tracking execution flow and managing exceptions effectively. By meticulously crafting test cases, utilizing breakpoints for inspection, and implementing robust logging practices, developers can enhance the integrity of their Java functions. This comprehensive approach is crucial in a constantly evolving software landscape where high-quality code is paramount. In conclusion, setting up the Java environment, understanding syntax and semantics, writing functions effectively, and thorough testing/debugging are key practices for successful Java development. With these best practices in mind, developers can navigate the world of Java programming confidently and achieve their goals efficiently.
Start coding in Java with Machinet and experience the power of AI-driven development!
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.