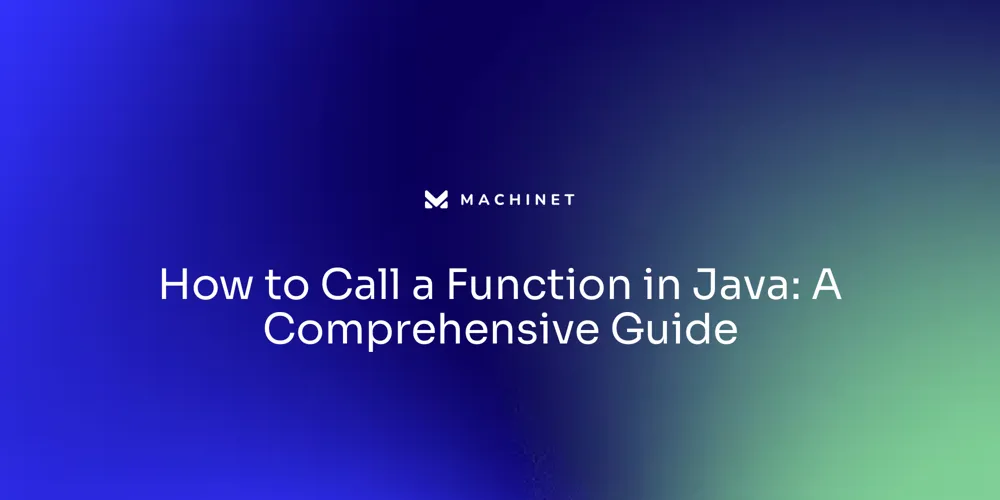
Table of Contents
- Understanding Java Functions
- Declaring and Defining Functions in Java
- Calling Functions in Java
- Passing Arguments to Functions
- Returning Values from Functions
- Common Use Cases for Function Calls
- Best Practices for Calling Functions
- Troubleshooting Common Issues
Introduction
Understanding the structure and behavior of Java functions is essential for any software development process. Java functions provide a modular approach to programming, encapsulating reusable code and simplifying code management. In this article, we will explore the concepts of Java functions, including declaring and defining functions, calling functions, passing arguments to functions, returning values from functions, and best practices for calling functions.
We will also discuss common use cases for function calls and troubleshooting common issues. By the end of this article, you will have a comprehensive understanding of Java functions and be equipped with the knowledge to write clean and efficient code. Let's dive in!
Understanding Java Functions
Understanding the structure and behavior of Java functions is essential for any software development process, as they offer a modular approach to programming. Essentially, Java functions, also known as methods, provide a way to encapsulate reusable code, allowing for easier maintenance and code management. This modular design benefits us in various ways, such as improving code readability and simplifying the debugging process.
For example, when writing code and engaging in a Slack chat simultaneously, the essence of concurrency comes into play. While not occurring in parallel, these tasks illustrate the concurrent nature of activities, just like synchronous and asynchronous requests in Java functions. The simplicity of synchronous execution - a by-the-book execution where the response immediately follows the request - contrasts with asynchronous execution, where operations continue without blocking the thread.
This is a vital concept in Java, reflecting on real-life multitasking. As highlighted by Eleftheria, an expert in the computer software industry, these concepts are key to creating efficient and accessible Java code.
Java, now a veteran language with a nearly 30-year history, continues to evolve while maintaining stability and embracing new features. Its transformative journey from a nascent language for set-top boxes to the foundation of modern software on various platforms emphasizes its adaptability and the evolving concept of Java. The journey of Java from its inception emphasizes the criticality of writing robust, future-proof code.
As the software craftsmanship philosophy underlines the significance of 'clean code', Java developers are encouraged to strive for code clarity.
Clean Java code has the benefit of being easily readable, understandable, and maintainable, adhering to best practices such as using descriptive names over confusing acronyms. Clarity in code names, as recommended by seasoned developers, eliminates the ambiguity of acronyms like 'Ctr', which could stand for multiple concepts, and instead favors a clear and meaningful designation like 'Customer'. By following Java's naming conventions and principles, developers create code that is not only correct but shines in its elegance and simplicity.
The introduction of Java has initiated a powerful legacy in the tech world, providing a framework for developers to harness their creativity and technical skill in writing efficient, understandable, and adaptable code, proving that it's not just about the result, but also about the process and practice of crafting exemplary software.
Declaring and Defining Functions in Java
To implement a function in Java, it is essential to grasp the underlying structure and the rules that govern its creation. A Java method, which embodies functionality within a class, follows a specific declaration pattern. An access specifier delineates the realm of method accessibility, ensuring control over where the method can be invoked.
Followed by this, the return type declares the data type of the output produced by the method. If no output is expected, the keyword 'void' signifies the lack of return value.
When naming a method, adherence to Java naming conventions is crucial for readability and maintainability—attributes highly regarded as best practices within the domain of 'clean code'. Parameters, constituting inputs to the method, are declared within parentheses following the method name. They range from none to many, tailored to the demands of the function.
The method body—wrapped in curly braces '{}'—houses the executable statements. Short, focused methods, embracing the Single Responsibility Principle (SRP), add clarity and reduce complexity, rendering the code easier to comprehend and modify, thereby upholding the ethos of 'clean code'. An example to illustrate this might involve parameters 'x' and 'y' of type 'Int', with the method's purpose being to compute their sum and returning the result as an 'Int'.
In essence, each aspect of the method syntax in Java ensures that developers not only deliver working solutions but also contribute to the collective codebase with code that is elegant, expressive, and enduring.
Calling Functions in Java
In the dynamic sphere of Java development, the skill of invoking functions adeptly is a fundamental yet potent tool in a developer's arsenal. This ability facilitates the execution of code in an organized and reusable fashion, contributing significantly to software that is not only functional but also exhibits exemplary attributes of clean code. Java's ethos espouses the creation of code that is readable, maintainable, and streamlined.
By embracing practices such as standard naming conventions, code in Java is transformed from mere functionality to an art form where clarity is to be expected rather than the exception.
Having trouble calling your methods effectively in Java? This could lead to concurrency issues, like blocking the main thread with synchronous requests or not managing asynchronous operations correctly. It's crucial to leverage Java's language features, such as the ability to use synchronous and asynchronous requests, to avoid such pitfalls.
It's a craft to write code that communicates clearly not just to machines, but to our human contemporaries. Consider the implications of code calling: a synchronous request may stall the thread, simple yet potentially rigid. On the other hand, an asynchronous call allows for the program to proceed without interruption, but it requires a more nuanced approach to manage the operations effectively.
Opting for simplicity and descriptiveness in our method names, as opposed to enigmatic acronyms, eases the understanding and maintenance of codebases. It's a subtle yet impactful choice that embodies the maxim of clean Java code—a philosophy advocating principles that enhance readability and modify-ease of code.
Remember, Java's landscape is continually evolving, marked by the introduction of new features and improvements with each version. With the launch of Java 21, the language welcomed enhancements to virtual threads, refined libraries, and syntax upgrades. Within six months of its release, a significant 1.4% of applications monitored by New Relic had already adopted this version, a testament to the developer community's value on advancements that bolster stability, security, and performance.
Thus, mastering the art of function calls in Java is not just about understanding its mechanics but also about knowing how to embed the language's advancements into your work rhythm.
Passing Arguments to Functions
When constructing functions in Java, parameters are essential for defining the specific information that the function will act upon. Parameters allow different pieces of data to be processed in a consistent manner by the same function, exemplifying the principle of the Single Responsibility Principle (SRP) which asserts that a function should have one and only one reason to change, corresponding to a single job.
As we delve into the intricacies of parameter passing, it becomes important to understand the distinction between value passing and reference passing. Value passing involves copying the value of an argument into the formal parameter of the function, so that any modifications within the function do not affect the original data outside the function. This approach is beneficial in cases where you want to protect the original data from being altered by the function, preserving the integrity of the data throughout the execution of your program.
On the other hand, reference passing means that the function receives a reference to the original data, not a copy, allowing the function to modify the actual data. This method is akin to giving someone direct access to edit a document as opposed to a photocopy. The powerful advantage of reference passing is observed when working with large data structures where passing by value is not efficient or even possible due to memory constraints.
To draw inspiration from a concrete example, imagine implementing an application for a parking lot system. Each parking slot might be represented as an object that contains information such as the slot ID, type of vehicle it can accommodate, and its current status. When updating the status of a given parking slot within a method, reference passing would allow us to modify the actual slot object in the parking lot system, rather than a copy, ensuring our changes reflect in the overall state of the parking lot accurately and instantly.
Garbage Collection (GC) plays an essential role in memory management in Java, especially when handling such large-scale data in applications. It automatically frees up memory that is no longer in use by the program, which can significantly reduce the risk of memory leaks and ensure that the application maintains its performance over time. By understanding the semantics of parameter passing in Java, you can write cleaner, more efficient code that harnesses the principles of clean code and the strategic advantages of Java’s robust language constructs.
Returning Values from Functions
Java's vibrant ecosystem allows developers to write functions that execute specific tasks and can return results for use in further processing. Functions in Java that yield results use the return
keyword to pass a value back to the caller. These returned values can be of various data types, including the primitive types like int
, double
, boolean
, and object references.
To declare a function that returns a value, specify the data type of the result as part of the function signature. For instance:
```java public int square(int number) { return number * number; }
``
In this example, the
square` method takes an integer as a parameter, processes it, and returns an integer. This simple yet robust functionality forms the crux of creating versatile and reusable code.
Understanding expressions and statements is foundational to mastering Java functions. Expressions are units of code that can be evaluated to produce a value and can be arithmetic, logical, or involving characters. Statements, on the other hand, are the building blocks that dictate the actions to be performed.
For example, '5 + 3' is an arithmetic expression that evaluates to 8, whereas the code inside the braces {...}
of a function comprises statements which may include return
statements, essential for handing back results.
Imagine the action of selecting a candy bar from a grocery store. The candy bar is an object you choose based on its characteristics. It stands to reason then, similarly, that functions in Java can serve as tools that perform tasks — akin to selecting a candy bar — where the return value represents the chosen item based on given attributes.
Moreover, the concept of 'clean code' is pivotal in writing functions in Java. Clean code is well-organized, understandable, and maintainable, which ultimately improves the sustainability of applications.
It's not just about writing any code that works but crafting code that stands the test of time and evolves as needs grow. With the proper method definitions that include clear intents, precise return types, and thoughtful naming conventions, developing clean Java methods becomes second nature, enhancing code quality and fostering a mature development environment.
Common Use Cases for Function Calls
For developers working with Java, comprehending asynchronous and synchronous function calls is crucial for crafting efficient applications. To elaborate, when a synchronous function is called, the thread that initiated the call is blocked until the function completes its task. This is akin to making a phone call and waiting on the line for a response.
In contrast, an asynchronous function allows the thread to proceed with other tasks while awaiting a response, much like sending a text message and carrying on with your day until you get a reply.
Delving into the realms of concurrency and parallelism is essential for Java developers, especially as applications become more complex and performance-driven. Concurrency illustrates the capability of an application to manage several activities, either sequentially or in tandem, which is pivotal when constructing responsive software systems.
Moreover, adhering to intuitive and consistent naming conventions is fundamental in Java, as underscored by the widely embraced JavaBeans naming practice. Such conventions prevent confusion and facilitate a clearer understanding of the codebase, thereby avoiding needless hurdles such as deciphering ambiguous acronyms.
Lastly, as we honor Java's impressive journey since its debut over 30 years ago, we continue to admire the enhancements that have been integrated into the language, ensuring that it remains relevant and efficient. This commitment to evolution is reflective of the broader discipline of software development, where writing code is not only about functionality but also about maintainability and clarity. Embracing the philosophy of “clean code” is integral to the craft, influencing not just the immediate project but the long-term wellness of the software at large.
Through the guidance of this article, you are invited to deepen your appreciation of Java's capabilities and to apply the principles of clean and maintainable code to your own development practices, empowering you to write Java code that is as robust as it is elegant.
Best Practices for Calling Functions
Crafting code in Java goes beyond functionality; it demands attention to clean code principles for long-term sustainability and maintainability. Calling functions effectively is a fundamental aspect of writing clean Java code. It involves incorporating practices that enhance readability and understanding, not just for the computer, but for your peers navigating the codebase.
Following standard naming conventions avoids confusion; for instance, prefer using clear names like 'Customer' over ambiguous acronyms like 'Ctr'. This clarity extends to your methods, which are the structural building blocks of your Java program. Ensuring that each method encapsulates a coherent set of actions adheres to the Single Responsibility Principle.
By keeping methods dedicated to a single task, you simplify both the debugging process and future code modifications. Ultimately, writing clean Java includes these aspects of function calling, which contribute to an overall codebase that is not only correct in functionality but also exemplary in its structure and clarity.
Troubleshooting Common Issues
When engaging with Java, understanding and correctly implementing function calls is a fundamental skill, as incorrect usage can lead to perplexing bugs or unexpected program behaviors. It's crucial to grasp the nuances of how Java handles concurrent and parallel tasks within your application. Java developers often deal with synchronous requests, which block the calling thread until a response is obtained.
This method of programming is direct: you make a request and then immediately process the received information. However, Java also allows for asynchronous function calls, where you initiate a request and your program continues to run. While your code moves ahead, the function operates quietly in the background, without interrupting the ongoing tasks.
Maintaining a clean and organized codebase becomes invaluable in this context. As methods are the building blocks of a Java application, dividing complex programs into smaller, more focused methods can dramatically simplify understanding and maintenance. The discipline of following the Single Responsibility Principle (SRP) ensures that each method addresses a single function or behavior, avoiding the pitfalls of creating monolithic, complicated code structures.
Oracle's complex relationship with open source Java has seen shifts in the developer community, with many moving away from proprietary Java solutions due to cost considerations, seeking open-source alternatives to avoid vendor lock-in. As businesses and individual developers navigate these waters, embracing clean, maintainable code practices—free from the constraints of a single vendor's policies—becomes paramount.
In this dynamic environment, Java programmers must be adept not only at writing functional code but also code that is clean and adheres to best practices, facilitating readability and future modifications. Reinforcing these practices, one becomes prepared to tackle function call intricacies effectively, keeping applications robust against the backdrop of evolving industry trends and licensing models.
Conclusion
In conclusion, understanding Java functions is essential in software development. They provide a modular approach, simplifying code management and enhancing code readability. By following syntax rules and naming conventions, declaring and defining functions becomes easier.
Effective function calls are crucial, with an understanding of synchronous and asynchronous requests to prevent concurrency issues. Adhering to standard naming conventions and clean code principles improves code readability and maintainability.
Passing arguments requires consideration of value passing and reference passing. Efficient memory management is important, especially with large-scale data.
Returning values from functions allows for versatile and reusable code. By declaring return types and using expressions and statements effectively, developers can create functions that produce desired results.
Common use cases for function calls involve asynchronous and synchronous calls, managing concurrency, and using intuitive naming conventions. Following best practices, like keeping methods focused and adhering to clean code principles, enables the creation of clear and maintainable code.
Troubleshooting function call issues requires a deep understanding of Java's management of concurrent and parallel tasks. Maintaining a clean and organized codebase, following the Single Responsibility Principle, and exploring open-source alternatives contribute to robust and adaptable code.
In summary, mastering Java functions is key to writing clean, efficient, and maintainable code. Understanding concepts and best practices enhances code readability, improves structure, and ensures the longevity of software development.
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.