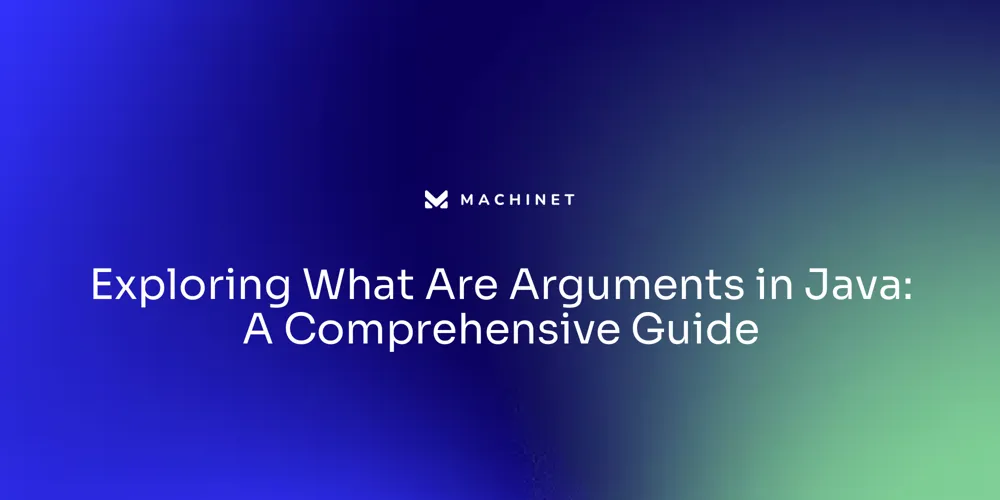
Table of Contents
- Understanding Arguments in Java
- Types of Arguments in Java
- Using Command Line Arguments in Java Programs
- Best Practices for Using Arguments in Java
Introduction
Java programming extends far beyond the confines of just writing methods; it involves a dynamic interaction with the user and the system environment. Command-line arguments in Java exemplify this interaction, as they allow programmers to input data into their applications directly from the terminal.
This feature transforms static code into a versatile tool that can respond to a variety of user needs and preferences. In this article, we will explore the different types of arguments in Java, how to use command line arguments in Java programs, and best practices for incorporating arguments in Java code.
Understanding Arguments in Java
Java programming extends far beyond the confines of just writing methods; it involves a dynamic interaction with the user and the system environment. Command-line arguments in Java exemplify this interaction, as they allow programmers to input data into their applications directly from the terminal.
This feature transforms static code into a versatile tool that can respond to a variety of user needs and preferences. For instance, a developer can take advantage of Java's flexibility by using command-line arguments to tailor a program's execution without altering the source code.
This adaptability is particularly useful in complex systems where predefined functions cannot cater to every potential scenario. As highlighted in recent updates like Java 21, the language continually evolves to offer more efficient ways to manage and process such inputs, with features like virtual threads simplifying the execution of concurrent tasks.
Moreover, the ability to manage dependencies efficiently is crucial in a language like Java, where each component can be fine-tuned for optimal performance. This level of control is a testament to the language's design, which encourages clean, maintainable code that shines in both functionality and clarity. As experts suggest, clean Java code is not only error-free but also expressive, well-organized, and easy to understand, making it essential for sustainable software development. In the realm of Java, developers often interact with a wide range of technologies, with JavaScript, SQL, Python, and HTML/CSS being the most commonly used alongside Java. Such versatility underscores the language's role in today's technology landscape, where applications must be robust, secure, and adaptable to the ever-changing demands of users and businesses alike.
Types of Arguments in Java
In Java programming, understanding the distinction between command line arguments and method arguments is crucial. Command line arguments are parameters that you pass directly to the Java program during its launch from the terminal. These arguments are handy for feeding input to your program dynamically, without altering the code itself, and they follow the program's name, separated by spaces.
For example, XPipe, an application that has undergone extensive user testing, leverages command line arguments for versatility across different platforms and use cases. On the other hand, method arguments are the parameters you pass when invoking a Java method, which enable the method to perform operations with the given data. As per the insights from JobRunr's latest release and Oracle's community engagement, such core Java features continue to be refined for performance and ease of use.
Moreover, libraries like Apache Commons CLI demonstrate the power of Java in creating efficient command line interfaces, simplifying argument parsing and validation. This is evident in its adoption by major Apache projects, confirming the practicality and robustness of such tools. The importance of using meaningful variable names, as highlighted in the case of the Args4j library, cannot be overstatedβenhancing code readability and maintainability, which is an essential practice for any Java developer.
Using Command Line Arguments in Java Programs
In Java, the main
method serves as the entry point for program execution and provides the ability to accept command line arguments. These arguments are encapsulated within the args
array, which is a parameter of the main
method.
The args
array holds the values passed from the command line when the program is started, allowing the user to influence the program's behavior at runtime. The following Java code illustrates how to process command line arguments:
java
public class CommandLineExample {
public static void main(String[] args) {
System.out.println("Number of arguments: " + args.length);
for (int i = 0; i < args.length; i++) {
System.out.println("Argument " + i + ": " + args[i]);
}
}
}
When executed with arguments, such as [java Command Line Example](https://docs.oracle.com/en/java/javase/20/docs/api/java.base/java/lang/System.html) first Art secondArg
, the program outputs the count of arguments received and lists each one.
It's important to note that Java is case-sensitive; therefore, main
differs from Main
. Additionally, proper syntax must be adhered to, such as enclosing strings in double quotes and terminating statements with semicolons, to avoid compilation errors. Furthermore, as Java evolves, features like JEP 480 and JEP 477 aim to simplify concurrency patterns and ease the learning curve for new programmers, respectively, making it even more approachable to work with command line arguments and other Java constructs.
Best Practices for Using Arguments in Java
When incorporating command line arguments in Java, it's crucial to ensure they contribute to clean, maintainable code. Validate and sanitize inputs thoroughly to prevent unforeseen behavior or security risks.
For example, the Apache Commons CLI library aids developers by offering built-in validation capabilities, which are highly regarded in the Java community, including projects like Kafka and Tomcat. Additionally, handle missing or incorrect arguments with grace; provide clear usage instructions or request the correct data, improving the user's experience and reducing potential errors.
Descriptive argument names in method parameters are not just good practice; they enhance code readability, which is a cornerstone of clean Java code. As Java technology evolves, maintaining code that's easy to read is critical for debugging and collaboration, as noted by Sharat Chander from Oracle.
Furthermore, documenting the expected arguments, especially when developing libraries or reusable components, is essential. This practice aligns with the Single Responsibility Principle, ensuring that methods are focused and comprehensible, thereby reducing the risk of bugs during updates. Real-world applications like XPipe, which has undergone extensive user testing across various platforms, exemplify these practices in action. By adhering to these guidelines, developers can create robust Java applications that are easier to maintain and less prone to errors, as evidenced by the ongoing momentum in the Java developer community and the continued advancements in Java technology.
Conclusion
In conclusion, command-line arguments in Java provide a versatile way for programmers to input data into their applications directly from the terminal. By using command line arguments, developers can make their code more adaptable and responsive to different user needs and preferences. Java offers two types of arguments: command line arguments and method arguments.
Command line arguments are parameters passed during program launch, while method arguments are parameters passed when invoking a Java method. To use command line arguments in Java programs, developers utilize the main
method as the entry point for program execution. The args
array within the main
method holds the values passed from the command line, allowing users to influence the program's behavior at runtime.
When incorporating arguments in Java, it is important to follow best practices for clean and maintainable code. This includes thorough validation and sanitization of inputs to prevent unforeseen behavior or security risks. Developers should also handle missing or incorrect arguments gracefully by providing clear usage instructions or requesting correct data.
Using descriptive argument names in method parameters enhances code readability, while documenting expected arguments is essential for developing libraries or reusable components. By adhering to these guidelines, developers can create robust Java applications that are easier to maintain and less prone to errors. The ongoing momentum in the Java developer community and advancements in Java technology highlight the importance of effective argument usage.
Overall, understanding and utilizing command-line arguments in Java enhance program versatility, user experience, and code maintainability. Incorporating these features allows developers to create dynamic applications that can adapt to various user needs and preferences. With proper validation, handling, and documentation practices, developers can ensure their Java programs are efficient, secure, and easy to understand.
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.