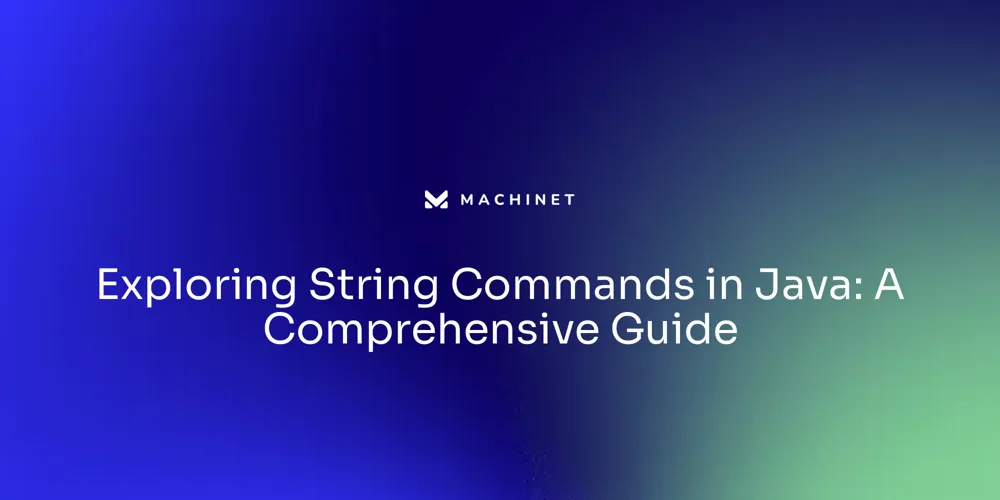
Table of Contents
- Creating Strings in Java
- String Literals and the new Keyword
- String Classes and Interfaces
- Immutable Nature of Strings
- String Methods and Operations
- String Concatenation and Formatting
- Using StringBuilder and StringBuffer
- Common Use Cases and Best Practices
Introduction
In the Java programming language, manipulating strings is a fundamental aspect of development. Whether it's creating strings using literals or objects, understanding the initialization process sets the stage for more complex string operations.
In this article, we will explore the different methods of creating strings in Java and delve into the intricacies of string manipulation. By mastering these techniques, developers can effectively communicate their intent and structure their code around well-defined data elements like strings. So, let's dive in and uncover the world of creating and manipulating strings in Java.
Creating Strings in Java
In the Java programming language, strings are fundamental components that are manipulated using the String
class. To create strings, there are primarily two methods:
- String Literals: By simply enclosing a sequence of characters within double quotes, you create a string literal.
This is the most straightforward way to create a string, as demonstrated by the following code snippet: String greeting = "Hello, World!";
. - String Objects: Alternatively, you can instantiate a new string object using the new
keyword in conjunction with the String
class constructor.
For instance: String farewell = new String("Goodbye, World!");
. Understanding the initialization of strings is akin to laying the foundation for further exploration into the intricacies of string manipulation. As with the initial steps of algorithm design, where a problem is broken down into actionable steps, creating strings is the preliminary phase before delving into more complex string operations. It sets the stage for programmers to effectively communicate their intent and structure their code around well-defined data elements like strings.
String Literals and the new Keyword
Strings in Java are a fundamental aspect of programming within the language, offering multiple ways to create and manage text. While string literals are a straightforward and efficient method, favored for their simplicity, the use of the new
keyword allows for the creation of distinct string objects.
This distinction is particularly relevant when considering memory management and object references. String literals benefit from Java's string constant pool, which promotes memory optimization by reusing identical string objects.
Contrastingly, strings instantiated with the new
keyword result in a new object each time, even if the string content is identical. This understanding is crucial for developers who aim to write memory-efficient code and manage string references effectively. As the tech industry evolves with trends like real-time services, which demand low latency and efficient data handling, grasping these nuances in Java's string handling becomes even more significant.
String Classes and Interfaces
In the vast landscape of Java programming, strings play a pivotal role, representing sequences of characters and offering a plethora of operations to manipulate them. The String
class is the most recognizable, immutable by design, ensuring stability and predictability in your code.
For scenarios demanding changeable strings, StringBuilder
steps in, providing an efficient way to append, insert, and delete characters, thus outperforming the +
operator for string concatenation in terms of efficiency. On the other hand, StringBuffer
mirrors StringBuilder
in functionality but brings the added advantage of thread safety, making it a reliable choice for concurrent execution environments.
Embracing the Single Responsibility Principle, each of these classes is designed with a clear focus, contributing to cleaner and more maintainable codebases. Moreover, Java's CharSequence
interface lays the foundation for these string-related classes, promoting consistency and interoperability across the language's ecosystem.
As stated in the realm of high-quality software development, clear requirements and effective communication are paramount. This principle transcends to code structure and commenting, where classes and interfaces like those for string manipulation in Java provide clarity and structure, serving as the building blocks of complex software systems.
According to recent statistics, developers who primarily use Java also frequently engage with languages and technologies like JavaScript, SQL, Python, and HTML/CSS. This multidisciplinary approach underscores the importance of a robust understanding of Java's string handling capabilities, ensuring developers can navigate and integrate multiple languages effectively. As we venture further into the intricacies of Java development, it's essential to appreciate the dualities of Native Java and frameworks. Native Java, with its high performance and control, contrasts with frameworks designed for productivity in complex projects. This comprehensive guide aims to equip developers and tech enthusiasts with the insights and understanding necessary to harness Java's full potential, whether through its core language or through the adoption of frameworks.
Immutable Nature of Strings
Understanding the concept of immutability in Java is pivotal for any developer, particularly when handling strings. By definition, once a string is instantiated in Java, its state is sealed; it cannot be altered.
Should any attempt to modify a string be made, Java doesn't tweak the original object but rather crafts a fresh string instance. This characteristic is not a quirk but a deliberate design choice with tangible benefits.
It ensures that strings can circulate within an application without the peril of accidental mutations, safeguarding the integrity of data across various modules. Moreover, immutability is a boon for Java's memory management.
It sanctions the reuse of identical string literals, thus streamlining resource utilization. A real-world example of immutability at play can be observed in how a major electronics company improved their video player app.
To determine if a URL was associated with a protected stream from services like WideVine, Vudu, or Netflix, the application inspected if the filename bore a specific substring. This operation, seemingly simple, underscores the importance of understanding string manipulation and immutability. The concept of immutability often provokes questions among developers. Why opt for data that resists change when software development is inherently about creating, updating, and deleting information? The answer lies in the predictability and reliability it provides, which are cornerstones of robust software. In the vast ecosystem of programming, where Java developers are also likely to engage with languages such as JavaScript, SQL, Python, and HTML/CSS, grasping the principles of immutability is essential for writing effective, error-resistant code.
String Methods and Operations
In Java, strings are a fundamental data type that are widely used in programming. To manipulate strings, Java offers a variety of methods. For instance, the length()
method computes the number of characters in a string, and the charAt(int index)
function retrieves the character at a specified position, acknowledging that each character's underlying representation is a binary sequence defined by an encoding scheme like Unicode.
Java also allows for the creation of string subsets through the substring(int beginIndex, int endIndex)
method, which extracts a section of the original string. When you need to merge strings, the concat(String str)
method seamlessly appends another string to the end. To modify content, replace(char oldChar, char newChar)
swaps all instances of one character for another, reflecting the importance of precise character encoding.
Understanding these methods is crucial for any Java programmer. As Eleftheria, an experienced business analyst, highlights, technical skills are invaluable in the software industry. Moreover, the Single Responsibility Principle (SRP) recommends that each method should perform a singular, well-defined task, preventing complexity.
Comments and documentation further elucidate the purpose and organization of code, essential for complex string manipulations. According to recent statistics, Java remains a core language for developers who also frequently use JavaScript, SQL, Python, and HTML/CSS. This illustrates the interdisciplinary nature of software development and the importance of mastering string manipulation within Java's rich ecosystem.
String Concatenation and Formatting
In Java, string concatenation is a fundamental operation that allows developers to merge multiple strings into one. By using the +
operator, such as in "Hello, " + "World! ";
, developers can easily combine strings.
Alternatively, the concat()
method offers another approach: "Hello, ".concat("World! ");
. For more sophisticated string construction, String.format()
is available, providing placeholders and format specifiers for inserting dynamic values into strings.
This method is particularly advantageous for generating strings with complex structures or variable content. As Eleftheria, an experienced Business Analyst, notes, effective communication is vital in software development, and this extends to how data is presented in string format. Clear and well-constructed strings can serve as a blueprint for understanding the purpose and functionality within a program.
Moreover, the Single Responsibility Principle (SRP) emphasizes that each method, such as those used for string concatenation, should have a single, focused task to prevent complexity. In the context of big data and traditional data management, the challenges of scalability and governance highlight the importance of clear and effective string manipulation. As we delve into integrating big data, the way we construct and handle strings in Java plays a crucial role in managing and presenting data accurately, making the understanding of string concatenation and formatting not just a technical skill, but a crucial aspect of software development.
Using StringBuilder and StringBuffer
In the realm of Java development, efficient string manipulation is a cornerstone of performance optimization. Mutable string classes like StringBuilder
and StringBuffer
come into play, offering dynamic alternatives to the immutable String
class.
Both StringBuilder
and StringBuffer
grant developers the power to alter string content through concatenation, insertion, deletion, and more, without the overhead of creating new objects each time. The distinction lies in synchronization: StringBuilder
is the go-to for single-threaded scenarios, boasting better performance due to its non-synchronized methods.
Conversely, StringBuffer
provides thread safety with synchronized methods, making it the choice for multithreaded applications where strings might be modified by concurrent threads. This nuanced understanding of when to employ StringBuilder
versus StringBuffer
is instrumental in crafting responsive Java applications that adhere to the Single Responsibility Principle, ensuring methods remain focused and maintainable. Moreover, these classes reflect the larger trend in software development towards real-time services, where quick, efficient data handling is paramount. As the software industry evolves, such knowledge is crucial for developers who aim to build systems capable of performing seamlessly in high-stakes environments like financial trading, where milliseconds can dictate success or failure.
Common Use Cases and Best Practices
In the fast-paced world of software development, strings hold a pivotal role, being the backbone of many common tasks such as parsing user input, text processing, SQL query construction, and shaping complex data structures. To optimize for clarity and efficiency, developers should adopt certain best practices. Using string literals over the new
keyword can significantly enhance performance, while leveraging StringBuilder or StringBuffer facilitates more effective string manipulation.
It's crucial to select the right methods for specific string-related tasks, ensuring a streamlined approach to handling text data. Moreover, understanding string encoding and character sets is essential to maintain the integrity of data across different systems and platforms. By embracing these best practices, developers can craft code that is not only clean and reliable but also aligned with the latest industry trends which underscore the importance of clear requirements and effective communication.
The Single Responsibility Principle (SRP) further guides developers to maintain focus in their methods, preventing the pitfalls of complexity. Proper commenting, as highlighted in recent insights, is another layer of best practice, offering clarity on the code's intent and serving as a navigational aid within the codebase. As the tech sector evolves, with a reported 13.4 million professional developers as of 2023, these practices are more than just recommendations; they are the standards that define high-quality software development in the modern era.
Conclusion
In conclusion, understanding how to create and manipulate strings in Java is crucial for effective development. By mastering the different methods of string creation, such as literals or objects, developers can structure their code around well-defined data elements.
Java provides classes like String
, StringBuilder
, and StringBuffer
for efficient string manipulation. Methods like length()
, charAt()
, substring()
, concat()
, and replace()
offer powerful tools for working with strings.
String concatenation and formatting operations allow developers to merge strings or insert dynamic values. Optimizing performance is important when manipulating strings.
Choosing between StringBuilder
and StringBuffer
based on thread safety requirements ensures efficient manipulation without unnecessary object creation. By following best practices like using literals over the new
keyword, understanding character encoding, adhering to the Single Responsibility Principle (SRP), and incorporating proper commenting, developers can write clean and reliable code that meets industry standards. In summary, mastering string manipulation in Java empowers developers to effectively communicate intent and efficiently handle text data. With the evolving tech industry demanding clear requirements, effective communication across languages, and efficient data handling, a strong understanding of Java's string handling capabilities is essential for success in software development.
Master string manipulation in Java today and take your coding skills to the next level!
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.