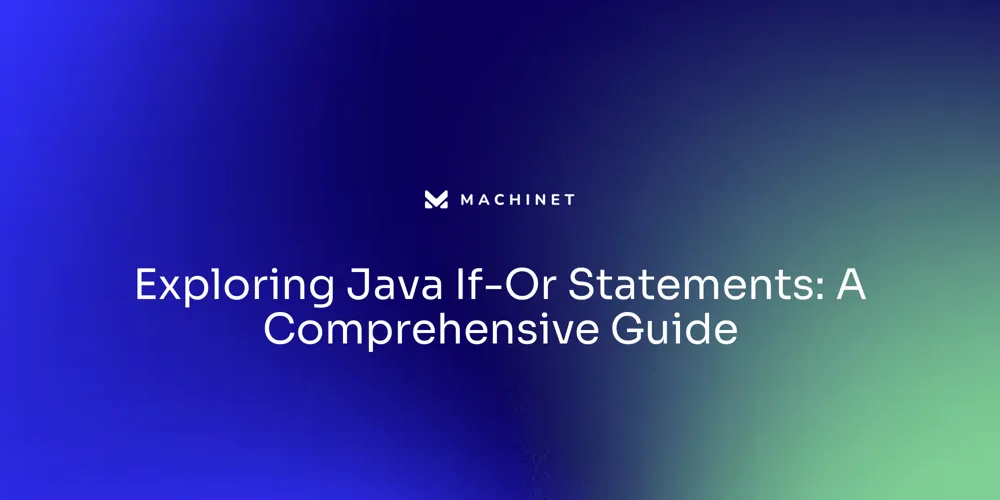
Table of Contents
- Understanding the Basics of If-Or Statements
- Syntax and Structure of If-Or Statements
- Examples of If-Or Statements in Java
- Best Practices for Using If-Or Statements
- Common Errors and Troubleshooting
- Advanced Uses of If-Or Statements
Introduction
Logical OR operators are a fundamental part of decision-making in Java programming. They allow developers to execute code based on specific conditions being met. In this article, we will explore the basics of If-Or statements in Java and their syntax and structure.
We will also delve into practical examples of If-Or statements in Java and discuss best practices for using them effectively. Additionally, we will cover common errors and troubleshooting tips, as well as advanced uses of If-Or statements. By the end of this article, you will have a solid understanding of If-Or statements and how to leverage them in your Java programs.
Understanding the Basics of If-Or Statements
Logical OR operators, symbolized by ||, are a cornerstone of decision-making in Java programming. They enable the execution of a block of code when at least one of the specified conditions is met.
This concept is akin to running a delivery company where different transportation methods are chosen based on various conditions like distance and package size. Just as a company might switch from bicycles to motorcycles or cars when the distance for deliveries increases, a Java program uses || to decide between different actions based on the truth values of expressions.
For instance, if a delivery needs to be fast, or the package is large, a car might be chosen. Translating this into Java code, an If-Or statement would look like this:
java
if (isDeliveryUrgent || isPackageLarge) {
useCar();
}
This structure is pivotal for creating flexible and responsive software that can handle various scenarios, much like a delivery company adapts to different cities' needs. By employing logical OR operators, developers craft conditions that are less rigid and more reflective of the real-world decisions, as in choosing the best transportation method for delivery services.
Syntax and Structure of If-Or Statements
When crafting an If-Or statement in Java, it's essential to grasp both its syntax and the underlying logic. The statement executes a code block only when at least one of the specified conditions is true, leveraging the logical OR operator. For instance, in a delivery system, an If-Or statement could determine the type of vehicle used for a delivery based on distance.
If the distance is less than a certain threshold, bicycles may be used; otherwise, motorcycles or cars could be considered. Here's a closer look at an If-Or statement structure:
java
if (condition1 || condition2) {
// Code to execute if either condition1 or condition2 is true
}
In this example, condition1
or condition2
represents the criteria to be met. The ||
symbol is the logical OR operator, and the enclosed code block will be executed if any of the conditions evaluate to true.
The use of If-Or statements is a fundamental aspect of decision-making in programming, allowing for flexibility and responsiveness within software applications. As noted by experienced professionals in the field, understanding and applying such logical operations is part of the foundation for successful software engineering, which aligns with the principles of modularity and abstraction. These concepts help manage complexity by breaking down tasks into smaller, cohesive units, promoting clarity and maintainability in code.
Incorporating If-Or statements effectively is akin to the meticulous planning phase of the Software Development Lifecycle, where every possible outcome is considered, and strategies are put in place to handle them. Similarly, in software risk analysis, these conditional statements can be instrumental in preempting potential issues and ensuring that applications behave as expected under varying conditions. Moreover, the adaptability of If-Or statements is reflected in the versatility of readymade software, which must cater to a broad user base with diverse needs, much like the logical operators that help tailor a program's response to different inputs.
Examples of If-Or Statements in Java
Java's If-Or statements are a cornerstone of decision-making in programming. Let's delve into practical examples to observe their utility. Consider a delivery company that uses bicycles for transportation.
In a small town, this method is flawless, but as the operation expands to a larger city, the distances become a challenge. The company adapts by introducing motorcycles and cars, illustrating the need for flexible decision-making in software as well. Similar to how the company's strategy evolved, If-Or statements can adjust to complex conditions, blending with other control structures to manage diverse data types.
Through methods, we encapsulate related instructions, enhancing modularity and adhering to the Single Responsibility Principle (SRP) for simplicity and focus. Comments play a pivotal role, clarifying code intentions and serving as documentation. Eleftheria, an experienced business analyst, emphasizes the importance of clear requirements and effective communication in software development, akin to a blueprint for successful projects.
Supporting these practices, the Software Development Lifecycle (SDLC) provides a structured approach to software creation, ensuring the final product aligns with its intended purpose and user needs. Software risk analysis, in turn, prepares us to anticipate and mitigate potential issues that could derail a project. Lastly, readymade software development demonstrates the value of pre-built functionalities for widespread use, highlighting the balance between custom and off-the-shelf solutions in the software industry.
Best Practices for Using If-Or Statements
If-Or statements are vital in Java programming, allowing developers to control the flow of execution based on certain conditions. When used wisely, they enhance code readability and maintainability.
To leverage these statements effectively, consider simplifying complex conditions to prevent the code from becoming convoluted. This can be achieved by breaking down conditions into smaller, more digestible expressions, or by employing methods that encapsulate related instructions, thereby adhering to the Single Responsibility Principle (SRP).
This principle ensures each method in your codebase has a distinct purpose, avoiding bloated and confusing logic. Moreover, nested If-Or statements should be minimized.
Deep nesting can lead to code that's difficult to follow and prone to errors. When necessary, use clear and descriptive variable names to reflect their purpose and make the code more intuitive.
Comments play a crucial role in explaining the rationale behind your conditions, especially when dealing with intricate logic. They serve as documentation and aid other developers in understanding the code's intent, promoting effective communication and collaboration. Remember, the goal of using If-Or statements is to write code that is not only functional but also clean and robust, facilitating easier testing, debugging, and future modifications. As highlighted in recent discussions on developer productivity, the efficiency of your code directly impacts the productivity of your team, especially when it comes to running automated tests. By following these best practices, you contribute to a codebase that is more maintainable and less prone to errors, ultimately supporting the success of your software development projects.
Common Errors and Troubleshooting
If statements are essential for decision-making in programming, allowing code execution based on whether a condition is true. For instance, if a user's input matches the correct password, a message can be displayed. Consider this simple code snippet:
```java int temperature = 25; if (temperature > 20) { System.out.println("It's warm outside!
"); } ```
Here, the program checks if the temperature exceeds 20 degrees and then prints a message accordingly. However, if not properly managed, such conditional logic can introduce risks into software projects, potentially impacting their objectives, schedule, budget, or quality. Software Risk Analysis assists in identifying and evaluating these risks by analyzing the project context and objectives, which is vital in preventing issues that could lead to project failure or cost overruns.
By understanding the significance of the Single Responsibility Principle, developers ensure that each method in their codebase has a clear, focused purpose, reducing the likelihood of errors. Comments also play a crucial role in maintaining code quality. They should explain the code's intent, document complex logic, and outline the code's structure, aiding both current understanding and future maintenance.
In the context of managing software risks, it's not about eliminating all potential issues—since that's often impractical—but rather assessing their impact and likelihood to develop effective management strategies. This involves identifying risks through brainstorming, historical data analysis, and stakeholder input, followed by evaluating their significance. Such a proactive approach is critical in the dynamic environment of software development, ensuring that teams can address uncertainties and navigate the complexities of their projects effectively.
Advanced Uses of If-Or Statements
Diving deeper into the Java language, we encounter scenarios that demand a nuanced understanding of If-Or statements. These conditional constructs are not just about making decisions; they are about making smart, efficient decisions.
Short-circuit evaluation, for instance, is a technique where Java evaluates a compound condition from left to right and stops as soon as the outcome is determined. This not only saves processing time but also prevents unnecessary computation, akin to a business analyzing risks and halting a project's phase when a potential issue is identified—thereby avoiding cost overruns.
When combining multiple conditions, it’s essential to adhere to the Single Responsibility Principle, ensuring each condition serves a clear purpose without overcomplicating the logic. Just as a business analyst like Eleftheria might dissect a complex market trend into understandable segments, a developer should approach compound conditions methodically, ensuring each part is coherent and justifiable.
Moreover, If-Or statements often work in concert with other control structures, forming a cohesive workflow. This is similar to a company expanding its delivery methods from bicycles to include motorcycles or cars, optimizing the process based on the context and requirements. In programming, this might mean using loops or switch statements alongside If-Or conditions to handle more sophisticated tasks. By mastering these advanced techniques, developers can craft code that is not just functional but also elegant and efficient, mirroring the evolution of a business that grows and adapts its strategies to succeed in a competitive environment. It's a journey from understanding the syntax to appreciating the nuances that make Java a robust and versatile programming language.
Conclusion
Logical OR operators, symbolized by ||, are a fundamental part of decision-making in Java programming. If-Or statements provide flexibility and responsiveness within software applications, allowing developers to execute code based on specific conditions being met.
By embracing If-Or statements effectively, developers can create code that is more modular, clear, and maintainable. Practical examples illustrate the utility of If-Or statements in Java programming and their alignment with software engineering principles such as modularity and abstraction.
To use If-Or statements effectively, it is recommended to simplify complex conditions by breaking them down into smaller expressions or employing methods that encapsulate related instructions. Clear variable names and comments enhance code readability.
Common errors in using If-Or statements can be mitigated through proper management of conditional logic and adherence to software risk analysis practices. Advanced uses of If-Or statements involve techniques like short-circuit evaluation and combining multiple conditions while adhering to the Single Responsibility Principle. By mastering If-Or statements, developers can write clean, robust, and efficient code that reflects the adaptability and versatility of Java as a programming language. In conclusion, If-Or statements play a crucial role in Java programming by providing flexible decision-making capabilities. By following best practices and understanding advanced techniques, developers contribute to maintainable codebases and successful software development projects.
Start using Machinet today and experience the power of AI in coding and unit testing!
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.