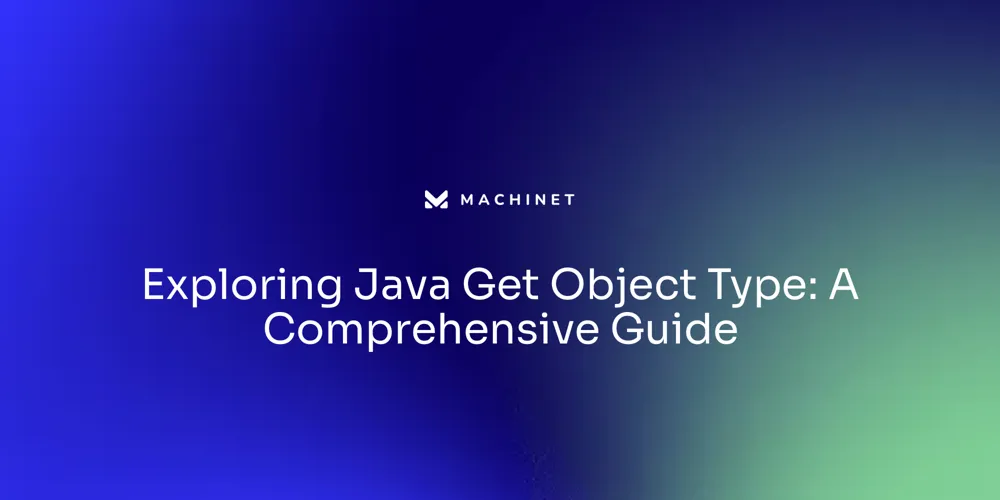
Table of Contents
- Understanding the Object Class in Java
- Using getClass() Method to Get Object Type
- Practical Examples of Getting Object Type
- Best Practices for Working with Object Types in Java
Introduction
The Object class in Java serves as the foundation of the class hierarchy, providing blueprints for all other classes and enabling the creation of objects with properties and abilities. This article explores the significance of the Object class in Java's object-oriented programming paradigm and its role in the latest Java Development Kit (JDK) versions.
It also delves into the use of the getClass()
method to determine an object's type at runtime and showcases practical examples and best practices for working with object types in Java. Whether you're a beginner or an experienced Java developer, this article will provide valuable insights into the Object class and its applications in Java programming.
Understanding the Object Class in Java
In Java, the Object class is the pinnacle of the class hierarchy, akin to the foundation of a pyramid that supports all structures above it. Every class created in Java draws upon the blueprints provided by the Object class, inheriting fundamental methods and attributes. This inheritance is a cornerstone of Java's object-oriented programming (OOP) paradigm, where classes represent blueprints for objects—akin to creating various objects like 'John' and 'Mary' from a 'Person' class template, each with properties like 'name' and 'age' and abilities such as 'walk()' and 'talk()'.
The Object class is not just a theoretical concept; it's actively utilized in the latest Java Development Kit (JDK) versions, where new features continue to evolve Java's capabilities. For instance, JDK 23 has introduced enhancements like the Vector API for expressing vector computations, which ultimately rely on the Object class and its derivatives. These updates reflect Java's ongoing commitment to modularity and reusability in code, allowing developers to extend and modify classes without altering their original implementations.
While Java does not support multiple inheritance, where a class extends more than one parent class—an approach possible in languages like C++ and Python—it champions the use of interfaces to achieve similar outcomes. The Object class, therefore, forms the genetic code for all Java objects, encapsulating their properties and methods, and enabling the creation of complex yet organized software that mirrors real-world entities with attributes and behavior. As one might say, objects in Java are like Lego blocks, each with its own color and shape, coming together to build a cohesive structure.
Using getClass() Method to Get Object Type
The getClass()
method in Java serves as a powerful tool for understanding the type of an object at runtime. By returning an instance of the Class
class, it opens up a world of possibilities for developers to interact with their objects.
For instance, with pattern matching introduced in Java, developers can match objects against patterns, simplifying the process of extracting data and performing operations on complex data structures. This enhances code readability and intuitiveness, as it allows parts of the object that match the pattern to be bound to variables, making the code more concise.
Moreover, the control afforded by Native Java allows for custom solutions and optimizations that are essential in complex projects. The use of the getClass()
method aligns with this adaptability, enabling developers to dynamically invoke methods at runtime based on the object's actual type, which is a cornerstone of polymorphism.
This flexibility and extensibility in code are critical for creating stable and reliable applications. For example, consider a case where a method's return type or parameter types are unknown at compile time. By utilizing the getClass()
method, one can retrieve these details dynamically, as illustrated by this code snippet:
java
System.out.println("Public Field: " + myObject.getClass().getName());
In this scenario, myObject
might be an instance of any class, and yet we're able to ascertain its type at runtime, which can then inform subsequent operations or method calls. As the landscape of Java development evolves, with updates like the release of EclipseStore and enhancements in Quarkus, the ability to determine object types at runtime remains an indispensable skill for developers navigating the intricacies of Java.
Practical Examples of Getting Object Type
In Java, the ability to discern an object's type during runtime is a foundational skill for developers. The getClass() method serves as a straightforward tool to achieve this, allowing for dynamic operations based on the object's class.
For instance, consider a Driver class designed to operate any type of Vehicle. When the drive() method is invoked, the method not only outputs the driver's name but also calls upon the drive() method of the specific Vehicle instance, be it a Car or a Bike.
This elegant use of polymorphism enables the Driver to handle the general Vehicle type, thereby enhancing code legibility, as type casting or conditional logic is unnecessary unless specific Car or Bike details are required. Furthermore, understanding object types in Java is critical when dealing with a vast array of entities, such as those in a database managed by a call center.
With over a thousand different entities to audit, tracking each field edited by users or applications becomes a monumental task. Leveraging Java Generics and Reflections API, a developer can craft a library to compare objects of the same type efficiently, streamlining the process of auditing field changes without relying on external libraries, which may be restricted due to security concerns. As Java continues to evolve, with JDK 21 introducing features like virtual threads, and the latest JDK 23 previewing the Vector API and primitive types in patterns, the language equips developers with the tools to write more expressive and efficient code. These advancements underline the importance of staying current with Java's capabilities, such as pattern matching and the use of sealed classes, which simplify data extraction and operation execution, leading to more intuitive and readable code.
Best Practices for Working with Object Types in Java
In the world of Java, understanding and managing object types is crucial for writing clean, efficient, and maintainable code. To enhance maintainability, consider using interfaces which act as a central point of control.
When the structure of an object changes, updating the interface ensures all objects align with the new structure, simplifying maintenance and augmenting early error detection. The finalize method in Java serves as a last resort for garbage collection, allowing objects to clean up resources before collection, although its execution is not guaranteed.
For representing complex behaviors, classes are the go-to structure, encapsulating both data and behavior, which is particularly useful for modeling real-world entities. When dealing with collections, the choice between Lists and Sets depends on the need for ordered elements or uniqueness, respectively.
Lists maintain a sequence and allow duplicates, while Sets ensure each element is unique. With Java's evolving landscape, as highlighted by the latest release of JobRunr and Oracle's acknowledgment of the vibrant Java community, staying abreast of best practices and emerging trends is key. Leveraging pattern matching can lead to more readable code, especially when dealing with complex data structures, by binding parts of a value to variables if it matches a certain pattern. This approach, along with the strategic use of classes and interfaces, can significantly enhance the clarity and maintainability of your Java applications.
Conclusion
The Object class in Java is the foundation of the class hierarchy, enabling the creation of objects and providing blueprints for all other classes. The getClass()
method allows developers to understand an object's type at runtime, enhancing code legibility and simplifying complex operations.
By leveraging Java Generics and Reflections API, developers can efficiently compare objects of the same type and streamline processes like auditing field changes. Following best practices, such as using interfaces for maintainability and classes for complex behaviors, leads to clean and efficient code.
Staying current with Java's capabilities, like pattern matching and sealed classes, improves code readability. By embracing emerging trends and best practices, developers can create maintainable Java applications that leverage the language's features. In conclusion, understanding the Object class in Java and effectively working with object types are fundamental skills for developers. By utilizing the getClass()
method, leveraging Java Generics and Reflections API, following best practices, and staying current with Java's capabilities, developers can create robust applications with clean code that is easy to understand and maintain.
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.