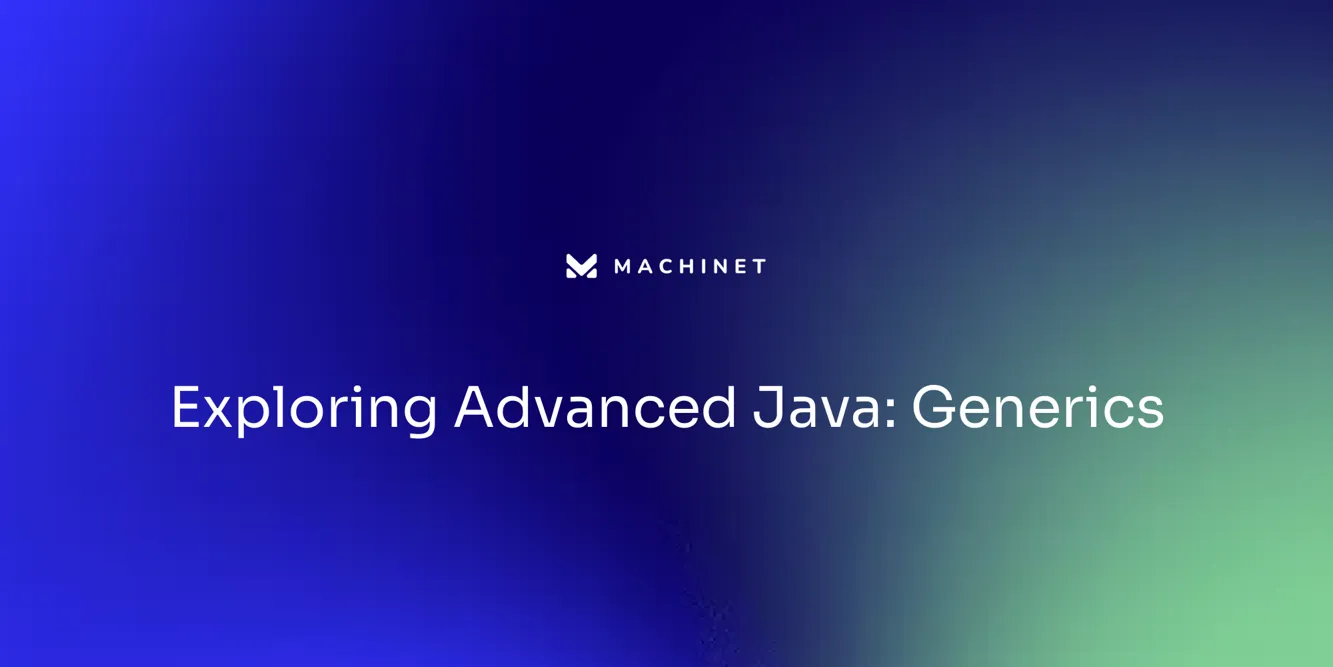
Table of Contents
- Understanding Generics
- Benefits of Using Generics
- Declaring and Using Generic Types
Introduction
Java's Generics are a robust feature that facilitates the creation of adaptable code components. They enable defining classes, interfaces, and methods that can interact with diverse data types, providing compile-time type safety.
Generics use type parameters, acting as substitutes for real data types, to parameterize classes and methods, enhancing their flexibility and reusability. This article will explore the benefits of using generics in Java, as well as how to declare and use generic types. By mastering this feature, developers can elevate the quality of their code and enhance their proficiency in Java development.
Understanding Generics
Java's Generics are a robust feature that facilitates the creation of adaptable code components. They enable defining classes, interfaces, and methods that can interact with diverse data types, providing compile-time type safety. Generics use type parameters, acting as substitutes for real data types, to parameterize classes and methods, enhancing their flexibility and reusability.
This enhances code adaptability while maintaining type safety. Two pivotal concepts in understanding generics are type erasure and type bounds. Type erasure refers to the compiler's process of eliminating generic type information at runtime, a crucial step for backward compatibility with older Java versions.
Type bounds impose restrictions on the types that can be applied to a generic class or method, enabling developers to enforce constraints on generic parameters. The introduction of JDK 21, as per Oracle's biannual standard Java updates, has brought about features like virtual threads and a generational Z garbage collector. These updates have continued to enhance Java's versatility, evident in the ease of upgrading from Java 17 to Java 21.
The case of pattern matching exemplifies this, as it aids in extracting data from data structures and performing operations on them, leading to more intuitive code. However, it's essential to navigate potential challenges such as performance overhead, the need for tuning, and the learning curve. Balancing these advantages and drawbacks based on project requirements is crucial.
The principles of the Dependency Inversion Principle (DIP) can be applied in Java through dependency injection frameworks, where interfaces or abstract classes define dependencies, and concrete implementations are injected at runtime. After nearly 30 years since its inception, Java continues to evolve, offering language enhancements for intuitive coding and top-notch performance. It remains a mature language, yet young at heart, and mastering its features such as generics can significantly elevate the quality of your code, leading to proficient Java development.
Benefits of Using Generics
Java's generics feature offers several significant advantages. It provides compile-time type checking, ensuring type-related errors are caught during development rather than runtime, increasing code robustness and catching potential issues early.
Generics enhance code reusability. By creating generic classes and methods, developers can write code that works with various data types, promoting reusability and reducing duplication.
This leads to more efficient, maintainable codebases, as emphasized by the OpenJDK project's dedication to developer productivity. Generics also contribute to code readability.
With type parameters, the intended purpose of a class or method can be clearly expressed, making it easier for developers to understand and use the code. This is in line with the best practices outlined to enhance the readability, reliability, and scalability of Java applications.
Furthermore, generics allow for performance optimization. By using specialized algorithms and data structures, developers can tailor their implementations to specific data types, enhancing efficiency and reducing memory overhead. The control afforded by Native Java over codebases allows for such optimizations, contributing to stable and reliable applications. In conclusion, generics are a powerful tool in Java, offering numerous benefits that contribute to high-quality, adaptable code. As Java continues to evolve, features like generics will continue to strengthen Java's position as a preferred programming language for business applications.
Declaring and Using Generic Types
Generics in Java are a mechanism that allows for type parameterization, enhancing code reusability and ensuring type safety. Consider the creation of a 'Box' class, employing a type parameter 'T'. This 'T' parameter can represent any valid Java type, offering flexibility.
```java
public class Box
public T get content() {
return content;
}
public void set content(T content) {
this.content = content;
}
} ```
While creating an instance of the 'Box' class, the type for 'T' can be specified. For instance, 'String' could be used as the type parameter:
```java
Box
String content = stringBox.getContent(); System.out.println(content); ```
This example demonstrates setting and retrieving the content of the box using the 'getContent()' method.
Methods can also employ generics, allowing them to operate with diverse types, thereby augmenting flexibility. For example, a generic method 'printArray' might be declared to accept an array of type 'T' and print each element:
java
public <T> void printArray(T[] array) {
for (T element : array) {
System.out.println(element);
}
}
Generics in Java offer a powerful tool for creating reusable classes and methods capable of working with various data types. This helps developers write flexible, adaptable, and type-safe code.
Conclusion
Java's Generics are a robust feature that enables the creation of adaptable code components. They provide compile-time type safety, enhance code reusability and readability, and allow for performance optimization. By mastering generics, developers can elevate the quality of their code and enhance their proficiency in Java development.
Start using Machinet today and revolutionize your coding experience!
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.