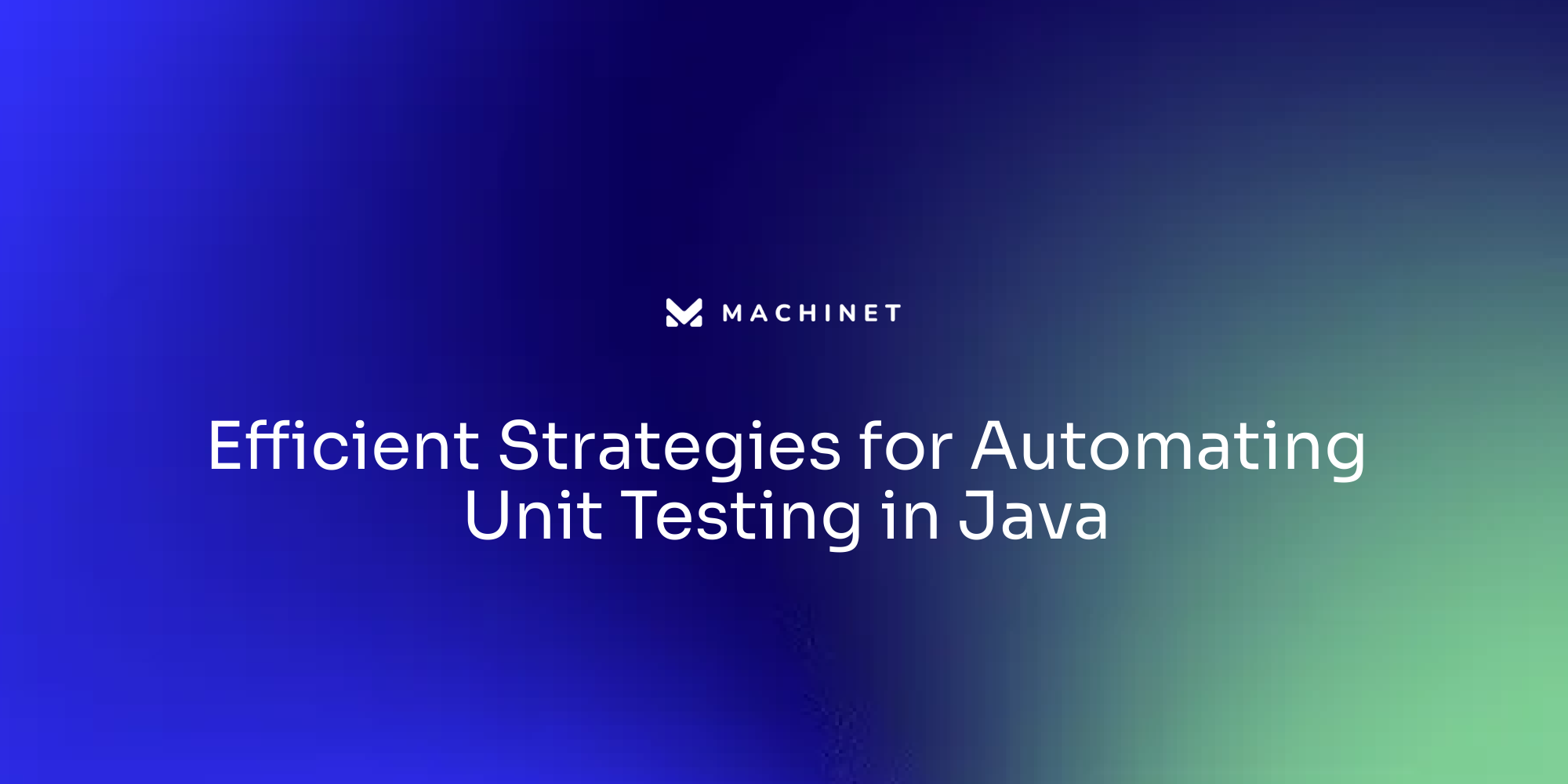
Table of contents
- Understanding Automated Testing in Java
- Key Concepts of Unit Testing in Java
- Selection of Suitable Unit Testing Frameworks for Java
- Strategies for Implementing Automated Unit Testing in Java
- Managing Technical Debt and Legacy Code during Unit Test Automation
- Adapting to Changing Requirements with Flexible Testing Frameworks
- Balancing Workload and Deadlines in Automated Unit Testing
- Best Practices for Automating Unit Tests in Java
Introduction
Automated unit testing is a crucial aspect of software development in Java. It ensures code reliability and functionality by automating test execution and minimizing the time and effort required for manual testing. By creating independent tests that validate the smallest pieces of code, developers can confirm their functionality and identify any potential issues.
In this article, we will explore the importance of automated unit testing in Java and the key concepts involved in the process. We will discuss strategies for implementing unit testing, selecting suitable testing frameworks, managing technical debt and legacy code, adapting to changing requirements, and balancing workload and deadlines. By following best practices and utilizing flexible testing frameworks, developers can enhance the efficiency and reliability of their software development process
1. Understanding Automated Testing in Java
Java, a statically-typed, object-oriented programming language, is known for its robustness and versatility. A critical aspect of software development in this language is systematic and automated testing. It ensures code reliability and functionality by leveraging a variety of tools and frameworks to automate test execution, thereby minimizing the time and effort required for manual testing.
In Java, automated unit testing is a process involving the creation of independent tests that validate the smallest pieces of code, or 'units', confirming their functionality as expected. Essentially, a unit test can be seen as the smallest testable part of an application.
Writing testable code and conducting unit testing without relying on libraries necessitates a basic understanding of the unit testing process. This three-step process involves preparing test inputs, invoking the code to be tested, and verifying the behavior and result.
Dependency injection, a key concept in this process, helps make code more testable by separating configuration from use. This separation increases code flexibility and testability, especially when swapping implementations for test and production code. Dependency on abstractions, such as interfaces, further decouples code and simplifies this process.
Test doubles, like fake implementations of interfaces, are beneficial in unit tests. For instance, testing a username validation logic without using any libraries can be guided by the code examples in this context.
The introduction of JUnit 5 has simplified the setup and execution of unit tests. For example, tests for a decision maker class can be easily written using JUnit 5.
While striving for 100% code coverage and practicing test-driven development (TDD) can be debatable, it's crucial to provide balanced perspectives on these topics. As a software engineer, you should be open to experimenting with different testing approaches and understanding the importance of incorporating regular test writing into your practice.
In the world of unit testing in Java, resources like Baeldung offer various guides and tutorials, including best practices for unit testing in Java. They also offer courses on related topics such as Spring, Spring Security, and Spring Data JPA.
Explore Baeldung's guides and tutorials for unit testing in Java
For those interested in AI-assisted coding and automated unit test generation, Machinet AI is a useful tool.
To enhance the efficiency of unit testing in Java, there are several tips worth considering. One crucial tip is to design your code to be testable, which includes breaking down complex methods into smaller, more focused ones, and minimizing dependencies.
Design your code to be more testable for efficient unit testing
Mocking frameworks like Mockito can be used to simulate external dependencies and isolate the unit under test, enabling the testing of the unit's behavior without relying on the actual implementation of the dependencies.
It's also important to prioritize tests based on their significance and impact. Start by writing tests for critical and complex parts of the code, then gradually add tests for less critical areas. This ensures that the most important parts of the code are thoroughly tested.
Effective use of assertions in unit tests is recommended. Assertions help verify that the code's expected behavior is met. By using specific assertions, the code's functionality can be assured, and potential issues or bugs can be identified.
Lastly, automating the execution of unit tests using build tools like Maven or Gradle is beneficial. This enables easy integration of unit tests into the build and deployment process, ensuring that tests are run consistently and providing immediate feedback on the code's quality.
In summary, automated unit testing in Java is not just a necessity, but a pathway to efficient and reliable software development
2. Key Concepts of Unit Testing in Java
Unit testing in Java is a strategic approach for verifying the functionality of individual software components in isolation. This practice is instrumental in fostering software reliability and quality, and it encompasses several pivotal concepts such as test coverage, test-driven development (TDD), assertions, and mocking.
Test coverage quantifies the portion of code exercised by the tests, thereby acting as a gauge of the software's validation. The significance of test coverage in unit testing lies in its ability to pinpoint untested code segments, thereby facilitating comprehensive software validation.
Test-driven development (TDD) is a software development strategy that advocates for the creation of tests before the actual code. Implementing TDD in Java provides several advantages. It not only assists in spotting and rectifying bugs early in the development process but also encourages the creation of modular and loosely coupled code. Furthermore, TDD ensures that the code fulfills the desired specifications and fosters improved collaboration between developers and testers by providing a clear specification of the code's functionality. Ultimately, TDD can lead to the development of more robust and maintainable code.
Assertions are integral to unit testing, serving as statements that validate whether the code yields the expected outcomes. They act as a safeguard for code refactoring and maintenance, guaranteeing that the existing functionality is preserved despite changes in the code. Assertions also double as living documentation for the codebase, offering a precise and current depiction of the code's intended behavior.
Mocking is a technique employed to isolate the code under test by substituting dependencies with objects that mimic their behavior. This is generally facilitated by a mocking framework like Mockito, which enables the creation of mock objects. These mock objects allow developers to concentrate on the logic and functionality of the component under test, thereby fostering a more efficient unit testing process.
JUnit serves as the standard unit testing framework in Java, with JUnit 5 being the latest release. It boasts a more modular and adaptable architecture compared to its predecessor, JUnit 4, which is now obsolete and lacks support for newer testing patterns and Java features.
Upgrade to JUnit 5 for a more modular and adaptable unit testing framework
To set up JUnit 5, one must add the Jupiter engine and Jupiter API dependencies.
In JUnit, it is recommended to create test classes in the src/test/java directory, adhering to the same package convention as the class being tested. Test methods should be annotated with @Test from the JUnit Jupiter API, while the @BeforeEach and @AfterEach annotations can be used to establish and dismantle resources before and after each test method. JUnit also supports parameterized tests, enabling a single test method to be executed multiple times with varying parameters.
To utilize Mockito, one must add the Mockito Core and Mockito JUnit Jupiter dependencies. Mock objects can be created using the @Mock annotation, and their behavior can be specified using the Mockito.when() method. The Mockito.verify() method is also available to confirm interactions with mock objects, assuring that they behave as expected.
In sum, unit testing in Java is a harmonious blend of key concepts and tools aimed at ensuring software quality and reliability. By comprehending and effectively employing these elements, developers are empowered to create robust and maintainable software applications
3. Selection of Suitable Unit Testing Frameworks for Java
Selecting the most appropriate unit testing framework is a key factor in establishing efficient automation. JUnit has earned a high reputation in Java's testing sphere, primarily due to its simplicity and user-friendliness. It provides annotations to define test methods (@Test
) and assertions for validating the results of tests.
Nevertheless, other frameworks, such as TestNG, offer a wider variety of features. TestNG is a potent framework that surpasses JUnit by introducing advanced features like parallel test execution and flexible test configuration.
Mockito, a mocking framework, is another valuable tool that enables developers to create and configure mock objects. This is particularly useful when you wish to isolate dependencies in your code during testing. Mockito's simple API allows for the creation of mock objects and the definition of their behavior, thereby aiding in the development of effective and reliable unit tests for Java applications.
Let's explore the features of JUnit and TestNG more deeply. JUnit 5, the newest version of the JUnit framework, is made up of three parts: JUnit Platform, JUnit Jupiter, and JUnit Vintage. It supports parameterized testing using the @ParameterizedTest
annotation and enables test prioritization with the @Order
annotation. Furthermore, JUnit 5 allows for tests to be ignored using the @Ignore
annotation and offers options for grouping tests with the @Tag
annotation. Although JUnit 5 does not include built-in reporting capabilities, it can be paired with plugins to generate reports.
Conversely, TestNG is an open-source Java-based unit testing framework that offers comprehensive control over test cases and their execution. It supports parameterization with the @DataProvider
annotation and allows for test prioritization with the priority attribute in the @Test
annotation. TestNG also provides options for ignoring tests using the enabled attribute and for grouping tests using the groups attribute. Unlike JUnit 5, TestNG automatically generates an HTML report after the execution of tests.
When it comes to deciding between JUnit 5 and TestNG, the choice should be based on the specific requirements and features of each framework. The unique abilities of each framework cater to different testing needs, making the selection process heavily reliant on the specifics of the software being tested. Therefore, it's essential to carefully consider the specific testing requirements, the features provided by each framework, and the ease of integration into the existing development environment
4. Strategies for Implementing Automated Unit Testing in Java
Unit testing forms the bedrock of software development, serving as a quality assurance mechanism that verifies the performance and validity of individual code segments. This meticulous scrutiny of individual methods or code snippets ensures that their output aligns with the anticipated outcomes. Libraries like JUnit and Mockito are widely adopted within the Java ecosystem for unit testing, offering a sturdy base for productive and efficient testing strategies.
Before undertaking unit testing with JUnit in a Java project, it is common to install IntelliJ IDE and follow a sequence of specific steps to set up the project and incorporate the necessary dependencies. This process is crucial for early bug detection during the development process, ensuring the code meets quality standards, and driving the production of superior software.
A key best practice in unit testing involves creating tests that cover anticipated outputs. This encompasses checking if function calls occur as expected and using assertions to compare expected and actual values. Assertions such as assertEquals
and assertArrayEquals
play a central role in this comparison process, enhancing the precision and reliability of the tests.
In certain instances, unit testing also involves mocking external dependencies like database connections or network requests. Libraries like Mockito facilitate this isolation of the code under scrutiny by generating mock objects that mimic the behavior of external dependencies. In this context, method stubbing is a technique used to stub the behavior of getter methods or set certain properties of the mock object.
Organizing unit testing cases in separate classes and methods improves the structure and readability of the tests. Annotations such as @Test
and @DisplayName
provide additional information about the tests, enhancing their understandability and maintainability.
Unit testing strategies can be employed in a wide range of scenarios, such as comparing numbers, sorting arrays, or testing methods that involve database queries or updates. The process typically involves initializing necessary parameters, creating mock objects, invoking the method under test with the initialized parameters, and adding assertions to verify the test outcome.
Running tests frequently is another important strategy in unit testing. This practice facilitates early issue detection and promotes continuous code improvement. Continuous integration tools can automate this process, running tests whenever code alterations occur, thereby guaranteeing the robustness and reliability of the code.
Regular review and analysis of test results is vital for identifying and rectifying failing tests, fostering a robust and effective codebase. This practice, coupled with the previously mentioned strategies, ensures that new functionality does not disrupt existing functionality and boosts the overall quality of the software.
In essence, unit testing in Java involves writing simple, modular code with a single responsibility, creating tests for all new features or bug fixes, running tests frequently, and regularly reviewing test results. By adhering to these strategies and best practices, developers can ensure the reliability and correctness of their code, thereby delivering high-quality software solutions
5. Managing Technical Debt and Legacy Code during Unit Test Automation
The challenges of technical debt and legacy code are well-known hurdles in the software development landscape, especially when it comes to automating unit testing. Technical debt, a term that encapsulates the cumulative complications arising from the use of outdated technologies or sub-standard code quality, is a critical aspect that every developer must understand and manage effectively. Similarly, legacy applications, often a significant source of profit for companies, can become increasingly challenging to maintain and scale with advancing technology.
Addressing technical debt effectively often requires allocating time to tackle urgent issues and organizing initiatives such as "quality weeks" or hackathons to mitigate the underlying causes of debt. Minimizing the creation of new technical debt is also essential, which can be achieved by maintaining the status quo or migrating to newer technologies as required. A culture of code craftsmanship and engineering excellence is crucial for managing technical debt effectively.
Legacy code can often be a source of numerous bugs and issues, with even minor changes resulting in weeks of labor-intensive problem-solving. Therefore, management of legacy applications and technical debt should ideally be undertaken by senior engineers who possess a comprehensive understanding of the entire system.
One method to ensure that automated tests cover legacy code is to follow a systematic approach. Start by identifying the critical functionalities and areas of the legacy code that need to be covered by tests. This can be done by performing a thorough code analysis to understand the dependencies and interactions of different components. Once the critical areas are identified, prioritize them based on their importance and impact on the overall system. Design and implement appropriate test cases that cover these identified areas. This may involve creating test scripts and test data that exercise different scenarios and edge cases. It is important to ensure that the tests are comprehensive and cover all possible paths through the legacy code.
Tools and frameworks that facilitate automated testing, such as unit testing frameworks or test automation tools, can be incredibly helpful in this process. These tools can automate the execution of test cases and generate test reports, making it easier to track the coverage of the legacy code. Regular reviews and updates to the test suite ensure that the automated tests continue to cover the evolving legacy code. This can be done by incorporating feedback from stakeholders, monitoring the test coverage metrics, and addressing any gaps or areas that are not adequately covered.
Technical debt in performance testing can lead to long-term issues if not addressed promptly. Continuous integration and testing practices can help mitigate technical debt in performance testing. Automation plays a crucial role in achieving "hands-free" performance testing, but it can be challenging to implement. Performance test suites require regular evaluation and adaptation to changing conditions. Existing tests must be regularly reviewed to determine their relevance and necessity, and missing tests should be addressed. Metrics and logs should be collected and analyzed to validate performance test results. Slow tests that can impact the overall performance of the test suite should be addressed. Technical debt in performance testing should be tracked, prioritized, and addressed according to cost, impact, and risk.
In conclusion, managing technical debt and legacy code effectively requires strategic planning, careful analysis, and continuous monitoring and improvement. By following these steps, organizations can enhance the quality and maintainability of their legacy code through automated testing
6. Adapting to Changing Requirements with Flexible Testing Frameworks
Embracing automated testing within software development is akin to navigating through a dynamic landscape, constantly shifting to align with ever-evolving project requirements. A robust tool that aids in this journey is a flexible testing framework. It provides the agility to add, modify, or discard tests as the project evolves, ensuring the testing process remains relevant and effective.
One of the notable attributes of flexible testing frameworks is their support for parameterized tests. These tests are designed to run with varied inputs, thereby enhancing their versatility and adaptability. Consequently, a single test can verify multiple scenarios by merely adjusting the input parameters, leading to an uptick in testing efficiency.
Flexible testing frameworks also harmonize well with behavior-driven development (BDD). BDD pivots around the behavior of the system rather than its implementation, making the tests resilient to changes in the codebase. By formulating tests that mirror the desired behavior of the system, modifications to the underlying code are less likely to disrupt the test outcomes.
Moreover, the power of a flexible testing framework can be harnessed optimally when used in a framework-agnostic manner. This approach empowers developers and QA teams to explore various testing frameworks without being tethered to a particular one. It also supports custom tools and configurations, allowing teams to run tests in their preferred environment.
This sort of approach reaps benefits for managers and teams alike by providing a common tool that can be used across different frameworks and tools. Furthermore, it future-proofs organizations by facilitating the adaptation of workflows to changing technical and testing requirements. It also paves the way for organizations to leverage new features and beta versions of operating systems without disturbing existing workflows.
Given the information from the solution context, it's worth considering Machinet as a valuable resource for managing the dynamic nature of software requirements. It provides a systematic approach for handling software requirement changes, including documentation, impact analysis, plan development, stakeholder communication, and quality assurance. This rigorous process ensures the adapted software meets the evolving needs of its users, thereby enhancing the overall software development lifecycle.
Moreover, Machinet provides a wealth of knowledge on unit testing in Java, which can be beneficial for software engineers looking to improve their testing skills and knowledge. In essence, flexible testing frameworks, particularly when used in a framework-agnostic manner, offer a formidable solution for navigating the dynamic landscape of software requirements, ensuring the testing process remains efficient, effective, and adaptable
7. Balancing Workload and Deadlines in Automated Unit Testing
Striking a balance between workload and impending deadlines is a perennial issue in software development. The introduction of automated unit testing can aid in addressing this concern by minimizing the time and resources necessary for testing. However, it is paramount to reserve enough time for the composition and upkeep of tests.
Unit tests are instrumental in detecting regression issues that may inadvertently surface during refactoring, bug fixing, or the integration of new features into existing codebases. As such, the prioritization of tests based on risk and impact is a valuable strategy. Code areas with high-risk or frequent modifications should be the first to undergo testing.
The creation of a useful unit test suite follows several best practices. Among these is the need for a fast execution time for the entire suite, ideally taking seconds rather than minutes. Unit tests should also be independent of each other and not share state. This means they should be repeatable, relying solely on the code and not on external factors such as databases or file systems. Self-validating unit tests are also crucial, producing a boolean value post-test execution, indicating whether the test was passed or failed.
The unit test suite should evolve over time with the application code. Non-determinism in unit tests, where tests sometimes pass and sometimes fail, should be avoided. Developers should carefully examine the unit test code and logic under test to find the root cause of non-deterministic behavior.
The concept of "sustainable pace" is integral in agile software development. A sustainable pace involves delivering small chunks of value to customers frequently. Practices such as test-driven development, pair and ensemble programming, continuous integration, and acceptance test-driven development are seen as part of the agile approach. However, many software organizations still fail to understand the importance of working at a sustainable pace.
Pressure from company leaders to meet unrealistic deadlines often leads to teams working longer hours and sacrificing quality. It is important for teams to step back and evaluate their process to avoid falling into the trap of overworking and accumulating technical debt. Slicing stories into small, consistently-sized increments and limiting work in progress can help teams achieve a predictable and consistent cadence.
Automated test development should be seen as a long-term investment. While it may initially increase the workload, it can lead to significant time savings in the future. It is important for teams to discuss their workload and potential burnout in retrospectives and brainstorm small experiments to address the issue gradually. Not maintaining a sustainable pace can lead to burnout and high turnover, which is costly for organizations.
In conclusion, balancing workload and deadlines in automated unit testing is not only about managing the present but also planning for the future. By adhering to best practices and maintaining a sustainable pace, teams can ensure the quality and effectiveness of their unit tests, leading to more robust and reliable software solutions
8. Best Practices for Automating Unit Tests in Java
Unit testing automation in Java is a practice that necessitates a careful adherence to certain principles. The independence and isolation of each test is paramount, meaning they should not be dependent on the state of other tests or external variables. This autonomy permits each test to be executed in any sequence without influencing the result of other tests.
A recommended strategy is to keep tests straightforward and centered on a single functionality. This method aids in maintaining clarity and makes it easier to pinpoint the specific feature or function the test is validating. In the event of a test failure, it should be evident which part of the codebase is to blame, facilitating quick and effective debugging.
A key principle is determinism. A deterministic test is one that will yield the same results consistently, no matter how many times it is run. This consistency is crucial in guaranteeing the reliability of the tests and the codebase as a whole.
The provision of clear and meaningful feedback is another vital component of effective unit testing. If a test fails, it should provide insightful feedback that aids in swiftly identifying and resolving issues. This method not only enhances the debugging process but also improves overall code quality by making problems easier to identify and resolve.
The integration of tests into the build process is a practice that ensures they are executed regularly and automatically. This integration verifies every modification to the codebase, helping to preserve the product's integrity while also identifying any potential issues early on.
Apart from these fundamental principles, there are other practices that can amplify the efficacy of unit testing. One such practice is partitioning the test code into manageable sections, which simplifies understanding and maintenance. Similarly, employing a consistent naming convention and structure for test methods can make the test suite more navigable and comprehensible.
A given-when-then style can be employed for structuring tests, where each test comprises logical steps. The "given" step establishes a certain condition or creates objects needed for the test, the "when" step initiates the action being tested, and the "then" step asserts that the application's state is as expected. This style of structuring tests not only simplifies their understanding but also serves as living documentation for the project.
Generating code coverage reports is another practice that can assist in quantifying progress and ensuring every part of the codebase is adequately tested. Tools like Jacoco can be used to generate these reports, providing a visual depiction of what parts of the code are covered by tests.
Compliance with these guidelines can make the process of writing unit tests more streamlined and enjoyable. Furthermore, a robust and comprehensive suite of unit tests can significantly simplify future code refactoring, since the code is supported by consistent, self-documenting tests. Consequently, these practices not only aid in delivering a high-quality software product, but they also make the process of writing unit tests more efficient and enjoyable
Conclusion
Automated unit testing in Java is a crucial aspect of software development, ensuring code reliability and functionality by automating test execution. By creating independent tests that validate the smallest pieces of code, developers can confirm their functionality and identify any potential issues. The key concepts involved in the process include dependency injection, test doubles, assertions, and mocking frameworks like Mockito. These concepts enhance code testability and simplify the unit testing process.
The broader significance of automated unit testing lies in its ability to enhance the efficiency and reliability of the software development process. By following best practices and utilizing flexible testing frameworks like JUnit and TestNG, developers can create a comprehensive suite of tests that cover critical functionalities. This helps in detecting regression issues, improving code quality, and facilitating future code refactoring. Additionally, developers should prioritize tests based on their significance and impact, design testable code, and automate test execution using build tools like Maven or Gradle.
Boost your productivity with Machinet. Experience the power of AI-assisted coding and automated unit test generation. Visit Machinet here
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.