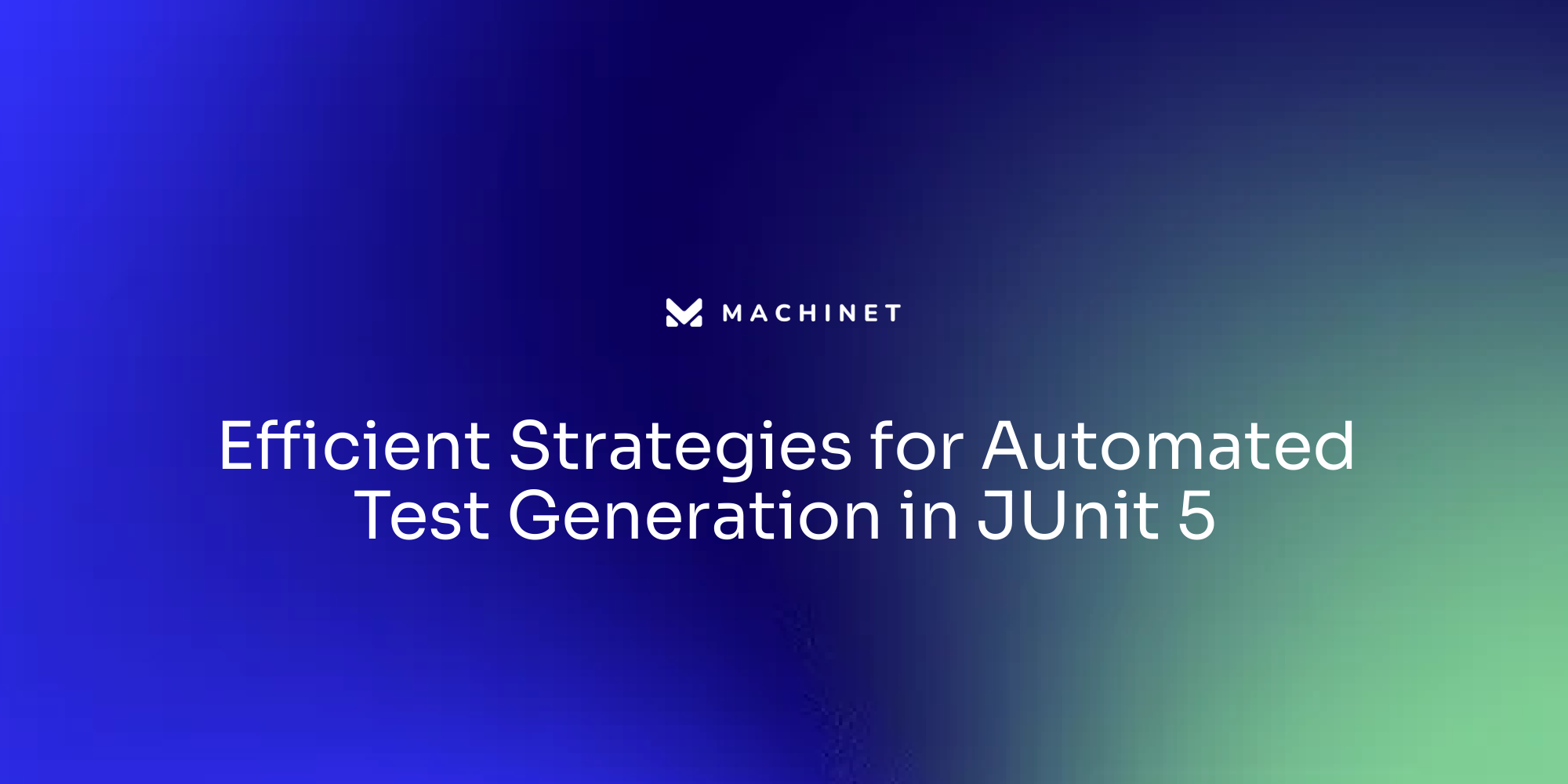
Table of contents
- Understanding Automated Test Generation
- Advantages of Automated Test Generation in JUnit 5
- Setting up the Environment for Automated Test Generation in JUnit 5
- Creating Dynamic Tests in JUnit 5
- Improving Dynamic Tests Using Java Features
- Effective Strategies for Managing Workload and Balancing Deadlines
- Advanced Examples: Adaptability to Changing Requirements
- Refactoring and Managing Legacy Code in JUnit Testing
Introduction
Automated test generation is revolutionizing the field of software development, especially in the context of unit testing. By leveraging advanced tools and frameworks like JUnit 5, developers can automatically generate test cases, reducing manual effort and improving the effectiveness of the testing process. This article explores the concept of automated test generation and its benefits, with a focus on JUnit 5's capabilities in this area.
In the realm of software development, ensuring the correctness and reliability of code is of utmost importance. Manually written test cases can be challenging to validate, as they may not cover the entire input domain and can be prone to errors. In contrast, automated tests, such as property-based testing, provide a broader range of inputs and can uncover unexpected failure cases. JUnit 5 offers a comprehensive framework for generating and executing automated tests, enabling developers to validate their code more effectively. By following a set of defined steps, developers can generate automated tests that enhance the reliability and quality of their software applications
1. Understanding Automated Test Generation
In the realm of modern software development, automated test generation plays a pivotal role, particularly within the scope of unit testing. This process utilizes advanced tools and frameworks to automatically craft test cases, thereby reducing manual effort and enhancing the efficacy of the testing procedure. JUnit5's automated test generation serves as a prime example, offering a substantial upgrade over its predecessor, JUnit4, by providing superior features and capabilities that elevate the entire testing process.
The widely accepted 'test pyramid' model in testing suggests a proportionate distribution of tests along the isolation-integration spectrum.
This model presumes that test cases are manually crafted. However, it's crucial to view test generation as an essential component, with generated tests forming the pyramid's base and hand-written scenarios at the pinnacle. The ultimate aim of testing is to validate correctness, which includes verifying that new features meet expectations before release and ensuring that existing functionality remains unaltered between releases.
Manually written test cases, or test scenarios, can pose a challenge to validate for correctness due to the 'test oracle problem' and limited coverage of the input domain. Conversely, generated tests, such as property-based testing, can inspect for properties and generate a wide range of inputs to test against, thereby offering the advantage of uncovering unexpected failure cases.
To generate automated tests with JUnit 5, you can follow these steps:
- Define your test class: Create a new class and annotate it with
@Test
and@ExtendWith
annotations. This will mark the class as a test class and enable JUnit 5 extensions. - Write your test methods: Inside the test class, write individual test methods and annotate them with
@Test
annotation. These methods will represent different test scenarios. - Use assertions: Within each test method, use JUnit 5's assertion methods to verify the expected behavior of your code. Assertions like
assertEquals()
,assertTrue()
, etc., are available. - Set up and tear down: If you need to perform certain setup or teardown actions before/after each test method or the entire test class, you can use
@BeforeEach
,@AfterEach
,@BeforeAll
, and@AfterAll
annotations. - Run the tests: Finally, you can run your automated tests by executing the test class using an IDE or build tool that supports JUnit 5. The test results will be displayed, indicating whether the tests passed or failed.
By following these steps, you can generate automated tests using JUnit 5, which will help you ensure the correctness and reliability of your code.
It is advisable to write both test scenarios and generated tests, with a preference for generated tests due to their ability to uncover failures and cover a larger portion of the input domain. Continually and concurrently running generated tests can enhance coverage of the input domain and increase the probability of detecting edge cases.
The automation of test cases should not be approached merely as a percentage of tests automated, as this can lead to quality engineering anti-patterns. The costs of automated tests, which include initial development and ongoing maintenance, should be weighed against the benefits, such as time saved in executing the tests. The value of automated tests fluctuates over time and can be either positive or negative, depending on various factors.
Different types of automated tests have diverse costs and benefits. For instance, unit tests have minimal upfront costs and maintenance but provide small incremental value with each execution. Conversely, end-to-end (E2E) tests have higher upfront costs and maintenance but demonstrate the full integrated system's value.
A balanced approach to test automation involves assessing automation options across all types of tests, updating existing tests, and removing obsolete tests. Test automation should be considered a vital part of the software development process and should inform system design and architecture. The role of the automator is to communicate system designers' automation requirements and create the most effective and efficient suite of automation. It is imperative to continually evaluate the health of the test suite and consider automatability in system design
2. Advantages of Automated Test Generation in JUnit 5
JUnit 5.4 has ushered in a new era of automated test generation for the Java programming language, introducing a myriad of novel features that significantly enhance developer testing capabilities. One of the most notable advancements is dynamic tests creation at runtime, which provides a more adaptive and flexible approach to testing, accommodating a variety of testing strategies.
Dynamic tests in JUnit 5 can be effectively utilized by following best practices such as using the @TestFactory annotation, generating dynamic test cases programmatically, utilizing the DynamicTest class, leveraging parameterized tests, and test templates. The @TestFactory annotation allows the creation of dynamic tests by returning a Stream, Collection, or Iterator of dynamic test cases, providing flexibility in generating and executing tests at runtime. The DynamicTest class allows the definition of dynamic tests programmatically, specifying the display name, executable, and tags for each dynamic test case. This is particularly useful when generating tests with different inputs or configurations.
JUnit 5.4 also offers substantial improvement to parameterized tests, simplifying the task of testing a method or function with an array of input values, even accommodating null and empty values. With the @ParameterizedTest
annotation, a test method can be defined that will be executed multiple times with different sets of parameters, using sources such as method arguments, CSV files, or custom sources. The process of including dependencies has been streamlined too, with the junit-jupiter dependency being the only requirement.
Another key feature introduced in JUnit 5.4 is the support for temporary directories during testing. This simplifies the creation and cleanup of temporary files during the testing process, ensuring a cleaner test environment.
The display names of the tests can now also be dynamically generated, based on the nested class or method name, which allows for more intuitive test identification. Furthermore, the new testmethodorder annotation allows developers to specify the order of test method execution, providing more control over the testing process.
JUnit 5.4 is designed with a more modular and extensible architecture, allowing developers to use only the features they require and extend the framework as needed. This modularity contributes to the efficiency and performance of the testing process.
Lastly, comprehensive documentation for JUnit 5.4 includes illustrative code examples, making it easy for developers to understand and leverage the new features. These advancements make upgrading to JUnit 5.4 highly recommended for developers seeking enhanced testing capabilities
3. Setting up the Environment for Automated Test Generation in JUnit 5
The journey to implementing JUnit 5 automated test generation begins with several key steps, each pivotal in establishing a functional testing framework.
The first step in this journey is the installation of JUnit 5 and its corresponding dependencies. This installation process can be seamlessly handled using build tools like Maven or Gradle, both of which are highly regarded in the realm of Java project builds.
To install JUnit 5 with Maven, for instance, you would begin by opening your project in an Integrated Development Environment (IDE) or navigating to your project folder via the command line. The pom.xml file, which manages dependencies and build configurations for your Maven project, is the next point of focus. Inside the <dependencies>
tag of this file, you can add the JUnit 5 dependency:
xml
<dependency>
<groupId>org.junit.jupiter</groupId>
<artifactId>junit-jupiter-api</artifactId>
<version>5.8.1</version>
<scope>test</scope>
</dependency>
After saving the pom.xml file, you can build your project using Maven. Running the command mvn clean install
will prompt Maven to download the JUnit 5 dependency and add it to your project's classpath. This completes the installation of JUnit 5 via Maven.
The next phase involves the configuration of your IDE to support JUnit 5. Most modern IDEs, including industry frontrunners like IntelliJ IDEA and Eclipse, come with built-in support for JUnit 5, which simplifies this process.
To configure IntelliJ IDEA for JUnit 5, you would start by opening IntelliJ IDEA and navigating to the project settings. Here, you can add the JUnit 5 library to your project dependencies under the "Libraries" section. Ensuring that the JUnit 5 library is properly configured and all necessary dependencies are included is crucial. You can then add the JUnit 5 library to your module dependencies under the "Modules" section. Creating a new JUnit 5 test class allows you to begin writing your tests using the JUnit 5 annotations and assertions. You can run your JUnit 5 tests by right-clicking on the test class or individual test methods and selecting the "Run" option.
Setting up Eclipse for JUnit 5 follows a similar process. After opening or creating a new Java project in Eclipse, you would right-click on the project in the Project Explorer and select "Build Path" > "Configure Build Path". In the "Libraries" tab, you can add the JUnit 5 library by clicking on "Add Library", selecting "JUnit", and choosing "JUnit 5". Clicking "Apply and Close" will save these changes.
The final steps in setting up an environment for JUnit 5 automated test generation involve modifying the Gradle build file to include necessary dependencies for JUnit 5, such as the JUnit Jupiter API, JUnit Jupiter Params, and JUnit Jupiter Engine. Enabling Gradle's native support for JUnit 5 involves ensuring the test task uses JUnit 5 instead of JUnit 4. Once these steps are completed, a simple test class can be created, followed by the addition of a test method and running the unit test with JUnit 5. This can be accomplished using the "gradle clean test" command, which initiates the Gradle task for running unit tests.
JUnit 5, with its design focus on Java 8 and above, supports various testing styles and provides developers with a versatile tool to streamline their testing processes. With JUnit 5 artifacts readily available from Maven Central, developers have easy access to the resources needed for setting up their testing environment. The JUnit team's commitment to regular updates and improvements to the framework, along with the use of tools like GitHub for version control, project management, and continuous integration, and Develocity for build analysis and acceleration, underscores the efficiency and effectiveness of the framework.
The steps outlined above offer a comprehensive guide for setting up a JUnit 5 automated test generation environment, equipping developers with the necessary tools and resources to implement effective and efficient unit testing processes
4. Creating Dynamic Tests in JUnit 5
JUnit 5 ushers in an era of dynamic testing, a transformative feature that allows tests to be constructed and executed on-the-fly. These tests, unlike their static counterparts, are created during runtime rather than being predefined. Encapsulated within a method carrying the @TestFactory annotation, dynamic tests pave the way for a sequence of tests to be generated dynamically.
A dynamic test comprises two essential elements: a unique name and a TestExecutionBody. The latter is a lambda expression that delineates the test's behavior. This configuration grants extensive flexibility, facilitating the formulation of tests based on a plethora of factors, including input data and runtime conditions.
Dynamic testing in JUnit 5 marks a significant advancement in the unit testing domain. It equips developers with a more versatile and adaptive testing strategy, empowering them to engineer tests that can adapt to diverse data inputs and runtime conditions. The dynamism of these tests enables them to cater to a wide array of scenarios, thereby becoming an indispensable tool for developers aiming to bolster the robustness and reliability of their software applications.
JUnit 5 also debuts a collection of extensions, providing a means to augment the behavior of test classes and methods. These extensions afford added functionality such as parameter resolution, exception handling, and more. They can be implemented via interfaces like TestInstancePostProcessor, ExecutionCondition, ParameterResolver, or TestExecutionExceptionHandler, and can be registered using the @ExtendWith annotation or through a configuration file.
Moreover, JUnit 5 enables the creation of parameterized tests, a potent tool that permits the same test to be executed multiple times with varying input parameters. This proves especially beneficial when testing a method or function expected to yield the same result for different input values. To create parameterized tests in JUnit 5, specific dependencies like "junit-jupiter-params" are necessary.
In essence, JUnit 5 brings a wealth of enhancements over JUnit 4, with dynamic tests, extensions, and parameterized tests being some of the most notable. These features foster a more flexible and adaptive testing strategy, thereby enabling developers to fortify the robustness and reliability of their software applications
5. Improving Dynamic Tests Using Java Features
JUnit 5 continues to lead the way in modern testing capabilities, with a particular emphasis on dynamic testing. The most recent release, JUnit 5.4, which was launched on October 19, 2021, delivers a host of appealing features designed to streamline dynamic testing. By leveraging the Java Stream API, developers can construct a series of dynamic tests, paving the way for more complex testing scenarios. Furthermore, the brevity and readability of dynamic tests are greatly improved through the application of Java's lambda expressions and method references.
The introduction of the @TempDir
annotation in JUnit 5.4 is a significant enhancement. This feature simplifies the management of temporary directories during testing, providing a sturdy solution for temporary data management. Furthermore, this feature eases the process of integrating dependencies by enabling the use of a single junit-jupiter
dependency.
Parameterized tests have been considerably enhanced in JUnit 5.4. The software now supports null and empty values, allowing for more thorough and rigorous testing. Another new feature, the @DisplayName
annotation, generates dynamic test names based on nested classes or method names, promoting clear and descriptive test identification.
The @TestMethodOrder
annotation, another significant addition, allows developers to specify the order of test method execution. This feature is essential in cases where one test's outcome depends on the execution of another.
JUnit 5.4 also includes a variety of other features outlined in the release notes, making it a recommended update for those looking to augment their testing capabilities. The detailed and high-quality JUnit 5 documentation, complete with comprehensive explanations and code examples, is a valuable resource for developers aiming to master these new features.
In terms of handling optional values in tests, Java's Optional class can be utilized alongside JUnit 5's assertions. This feature contributes to the development of more resilient tests by ensuring that optional values are adequately addressed in various testing scenarios.
To sum up, the combination of JUnit 5.4 and Java's robust features provides a powerful arsenal for dynamic testing. These capabilities can significantly augment the quality and effectiveness of your test suite. Furthermore, method references in JUnit 5.4 provide an effective way to define behavior in dynamic tests. By referencing an existing method that has the desired behavior, tests can be defined with more concise and readable code
6. Effective Strategies for Managing Workload and Balancing Deadlines
In the realm of software development, especially when dealing with automated test generation in JUnit 5, the art of managing workload and adhering to deadlines can often pose as a formidable challenge. In such a scenario, strategic planning and prioritization become key to successfully navigating these hurdles.
The process of prioritizing tests should be guided by several factors, including the inherent complexity of the code, the potential risk of defects, and the consequential impact of possible failures. The ultimate goal is to ensure the integrity of the software while minimizing the risk of issues that could compromise the user experience.
One effective strategy to enhance efficiency and reduce manual effort is the automation of as much of the testing process as feasible. This not only accelerates the testing process but also frees up human resources to focus on more complex tasks that require a human touch.
Another important consideration is the need to optimize build speeds. Slow builds can have a detrimental impact on team productivity, and this applies to any tech stack, not just Kotlin. Techniques such as running tests concurrently, using more threads than CPUs, and avoiding time-consuming setup and cleanup in tests can significantly improve test performance.
Moreover, it's advisable to differentiate between proper unit tests and scenario-driven integration tests. The former are smaller, faster, and more focused, while the latter tend to be larger and more complex. Flaky tests, which are tests that exhibit both a passing and a failing result with the same code, are particularly troublesome. Fixing these tests involves understanding why they are flaky and addressing the root causes.
Furthermore, it's important to keep your build tools up to date and take advantage of their performance improvements. Also, consider investing in faster Continuous Integration (CI) machines to further enhance build speeds. It's also worth noting that sleep calls in tests can slow down the process, so it's better to use polling instead.
The Agile methodology, with its dynamic approach and emphasis on reducing effort and delivering high-quality features in each iteration, can also be a valuable tool in managing workload and balancing deadlines. Agile testing, an integral part of this methodology, focuses on early engagement, synchronized efforts with development, a customer-centric approach, feedback-driven development, test-driven development, vigilant regression testing, streamlined documentation, and collaboration.
In conclusion, maintaining a sustainable pace of work is crucial to avoid burnout and ensure consistent productivity. By implementing these strategies, you can effectively manage your workload, meet your deadlines, and deliver a high-quality software product
7. Advanced Examples: Adaptability to Changing Requirements
The dynamic testing capabilities of JUnit 5 introduce a remarkable level of flexibility, fitting seamlessly with the fluid nature of software development. This adaptability is evident in the ease at which tests can be updated in response to changes in input data, eliminating the need to overhaul the entire test. Moreover, when a new requirement emerges, JUnit 5's dynamic testing enables swift generation of a new test to verify this requirement.
Let's take a scenario where National Information Solutions Cooperative (NISC), a technology solutions provider for utility cooperatives and telecommunications providers, sought to upgrade its customer-facing application suite, iVUE AppSuite. The upgrade involved enhancing the application's code, modernizing it for iOS compatibility, and adding desired functionality while maintaining existing features. In this situation, JUnit 5's dynamic testing capabilities would have been instrumental in ensuring that the new features and modifications meet the required standards.
JUnit 5 allows for quick generation of tests to verify the functionality of new features. This is achieved through the use of the @TestFactory
annotation provided by JUnit 5, which allows for the dynamic generation and execution of tests at runtime. A method annotated with @TestFactory
returns a Stream, Iterable, Collection, or an array of dynamic tests. Within this method, you can define the logic to generate the desired tests based on new requirements. Each dynamically generated test can be represented by an instance of the DynamicTest class, which provides methods to set the display name, define the test execution logic, and specify any dependencies or assumptions.
Additionally, given that the project was a modernization of an existing application, any changes in the input data would necessitate corresponding modifications in the tests. To make these tests more resilient to changes, you can use parameterized tests, which allow you to run the same test with different inputs. This can be particularly useful when requirements change and you need to test the same functionality with different sets of input data. Moreover, you can use test interfaces or test templates to easily modify or extend tests when requirements change. This helps avoid duplicating code and makes it easier to maintain your test suite.
JUnit 5 also provides test annotations such as @Disabled
or @EnabledIf
to selectively enable or disable tests based on certain conditions. This can be helpful when requirements change and certain tests become irrelevant or need to be skipped.
In the software development industry where requirements are constantly evolving, the dynamic testing capabilities of JUnit 5 provide an invaluable tool. This adaptability not only streamlines the testing process but also enhances the reliability of the software products delivered, as evidenced by the success of iVUE AppSuite's upgrade
8. Refactoring and Managing Legacy Code in JUnit Testing
Working with legacy code and refactoring is an essential aspect of JUnit5 automated test generation. JUnit 5 offers a range of features aimed at easing this process. For instance, annotations such as @Disabled and @Tag provide a controlled mechanism for test execution during the refactoring phase.
JUnit 5 also introduces a set of assertions specifically tailored for exception testing. This enhancement proves pivotal when testing legacy code that has a propensity for throwing exceptions. JUnit 5's versatility also shines through in its support for both vintage and contemporary test engines, smoothing the path from JUnit 4 to JUnit 5.
In the context of real-world applications, the case of Diffblue, a company that excels in AI testing, continuous integration, and DevOps, is noteworthy. Upon announcing Citi as a strategic investor, Diffblue has been drawing comparisons between their product, Diffblue Cover, and GitHub Copilot. Both leverage generative AI for code, but they serve different purposes for developers. This comparison underscores the importance of managing legacy code and refactoring in automated test generation.
In a survey conducted by Diffblue involving 450 IT leaders, the focus was to understand their approach towards Java application modernization. The survey results indicated a substantial amount of work that needs to be done in this area, thereby emphasizing the need for effective strategies for managing legacy code.
As Mathew Lodge, an expert in the field, aptly states, "Diffblue announces Citi as a strategic investor" and "Diffblue Cover vs GitHub Copilot: What's the difference?" These statements underline the significance of legacy code management and the role of automated testing tools like JUnit 5 in this process.
To summarize, the management and refactoring of legacy code form a critical part of automated test generation in JUnit 5. With its enhanced features, JUnit 5 simplifies this process, thus proving to be an invaluable asset for developers.
When transitioning from JUnit 4 to JUnit 5, it is advised to adhere to best practices to ensure a successful transition. This approach not only ensures a smooth transition but also helps to fully leverage the new features and improvements offered by JUnit 5.
While the context information does not directly mention the use of the JUnit 5 vintage test engine for legacy code migration, it's worth noting that JUnit 5 does offer support for both vintage and modern test engines. This feature is particularly beneficial during the transition from JUnit 4 to JUnit 5.
Furthermore, although there's no direct mention of JUnit 5 assertions for exception handling in legacy code in the provided context, JUnit does offer several built-in assertion methods suitable for exception handling in unit tests. These methods allow for specific exceptions to be asserted during the execution of test code, enabling verification that the expected exceptions are being correctly thrown in legacy code
Conclusion
Automated test generation, particularly in the context of unit testing, has revolutionized software development. By leveraging advanced tools and frameworks like JUnit 5, developers can automatically generate test cases, reducing manual effort and improving the effectiveness of the testing process. The benefits of automated tests, such as property-based testing, include broader input coverage and the ability to uncover unexpected failure cases. JUnit 5 offers a comprehensive framework for generating and executing automated tests, enabling developers to validate their code more effectively. By following defined steps, developers can generate automated tests that enhance the reliability and quality of their software applications.
The significance of automated test generation extends beyond the immediate benefits of reducing manual effort and improving test coverage. It allows developers to adapt to changing requirements more easily by enabling quick generation of new tests or modification of existing ones. Furthermore, automation frees up human resources to focus on more complex tasks that require a human touch. By embracing automated test generation with JUnit 5, developers can boost their productivity and deliver high-quality software applications. Boost your productivity with Machinet. Experience the power of AI-assisted coding and automated unit test generation. Boost your productivity with Machinet
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.