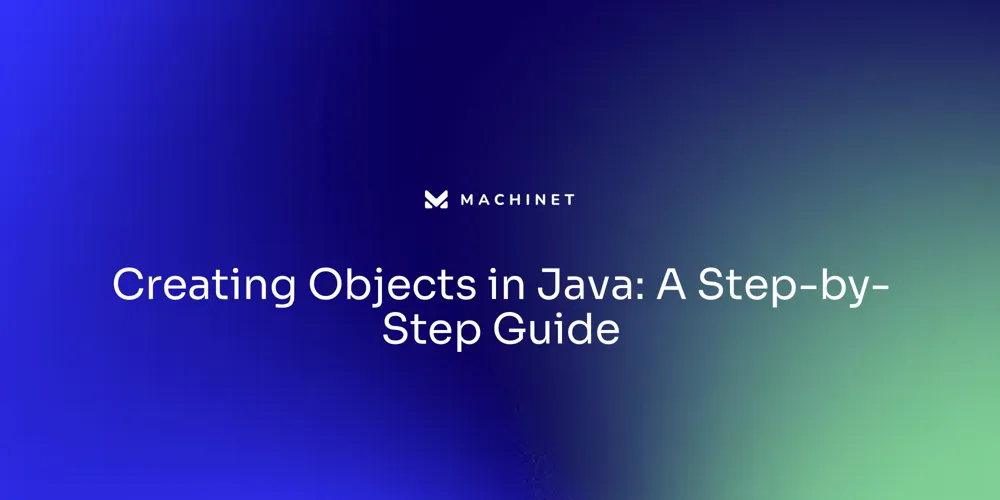
Table of Contents
- Understanding Object Creation in Java
- Using Constructors to Create Objects
- Creating Objects with the 'new' Keyword
- Object Creation Using Factory Methods
Introduction
Creating objects in Java is a pivotal skill for developers, as objects are the cornerstone of Java's object-oriented paradigm. This article explores the process of object creation in Java, including the use of constructors, the "new" keyword, and factory methods.
Understanding these concepts is essential for Java developers to write adaptable and efficient code. Additionally, the article highlights the importance of adhering to Java conventions and staying up-to-date with the language's evolution. Whether you're new to Java or an experienced developer, this article provides valuable insights into object creation in Java.
Understanding Object Creation in Java
Creating objects in Java is a pivotal skill for developers, as objects are the cornerstone of Java's object-oriented paradigm. To instantiate an object, one must first define a class that serves as a blueprint.
For example, in a simple parking lot system application, you might have classes like Parking Lot and Vehicle, each with their unique attributes and behaviors. A Parking Lot class might have properties such as a parking lot ID, number of floors, and slots per floor, while a Vehicle class could include types like car, bike, and truck.
When an object is created, it's allocated memory in the heap, a dynamic memory pool managed by the Java Virtual Machine (JVM). Unlike local variables that use stack memory, objects reside in the heap because their size may only be determined at runtime.
This memory management is crucial for optimizing system performance and is automatically handled by the JVM without explicit intervention. Moreover, adhering to Java's conventions, such as camelCase naming for methods and variables, enhances readability and maintainability.
Clear naming avoids confusion, especially for those new to the codebase. For instance, method names should be action-oriented verbs like calculateTotalPrice(), and variables should be indicative nouns like total Price. Best practices also recommend the Single Responsibility Principle (SRP), ensuring that each method is dedicated to a single task, thus simplifying the code structure. Recent updates, like in the Java 20 release, continue to introduce features that improve the language's capabilities, such as virtual threads for better concurrency management. The vibrant Java community, recognized by industry leaders, continually contributes to the language's evolution, ensuring that Java remains a robust platform for modern software development.
Using Constructors to Create Objects
In Java, constructors are pivotal for initializing new objects and setting up their initial state. They are invoked at the moment an object is created and have the same name as the class they reside in.
Understanding constructors is essential, particularly with Java's evolving landscape where new features are introduced as preview features, and feedback from developers can influence their final implementation. A constructor's absence leads to the automatic creation of a default constructor by the compiler, which is parameterless, adhering to the principle that a constructor should be comprehensive enough to handle all initialization scenarios.
Java's object-oriented nature is exemplified by constructors that do not have a return type and cannot be called like normal methods; they are unique to object creation. For instance, if you create a class named 'Product', the constructor will also be named 'Product'.
Overloading constructors is common practice, allowing for different initialization scenarios based on varying parameters. This flexibility is crucial, especially when considering the introduction of new language features such as virtual threads in Java 21, which have their own set of initialization considerations, such as potential deadlocks that might occur unexpectedly. Moreover, the Java community continually contributes to the language's growth, as seen in the recent updates to JobRunr, which exemplifies the importance of maintaining up-to-date and compatible code. As Java evolves with features like the Vector API in JDK 23, enhancing computational efficiency, constructors remain a foundational concept that every Java developer must master to write adaptable and efficient code.
Creating Objects with the 'new' Keyword
Java's journey from its inception as 'Oak' to the robust, widely-used language it is today is a testament to its adaptability and enduring relevance in the world of software development. The language's evolution has been marked by the careful addition of features that enhance its capabilities without compromising its core principles. One such foundational feature is the use of the 'new' keyword, which is essential for creating new objects.
When a developer uses the 'new' keyword, they are instructing Java to allocate memory for a new object of a specified class. This is a crucial step in object-oriented programming, as it allows for the instantiation of objects that can then be manipulated within the code. For example, consider the String class, which enables the creation of a sequence of characters without the need for a terminating null character, as seen in String s1 = "Scaler";
.
Similarly, arrays in Java, akin to a bookshelf, hold elements of the same data type, such as int[] arr1 = {1, 2, 3};
. The significance of the 'new' keyword becomes even more apparent when we look at the statistics revealing the rapid adoption of new Java versions. Within six months of its release, Java 21 was used by 1.4% of applications monitored by New Relic, a significant increase from the 0.37% uptake of Java 17 in its initial six months.
This showcases the language's ongoing refinement and the importance of understanding its core features, including object creation. As developers continue to build and maintain Java applications, from small-scale projects to large server boxes, mastering the use of the 'new' keyword and the principles of object creation is indispensable. It's a skill that echoes the language's history—adapting to new environments while staying true to the essence that has made Java an enduring choice for programmers around the globe.
Object Creation Using Factory Methods
The Java programming language is rich with patterns that simplify software development, and among these, the Factory Method pattern stands out for its ability to create objects while hiding the instantiation logic. This pattern is particularly useful when your application needs to manage the creation of various types of products. For instance, in a drawing application, the Factory Method allows for the creation of different shapes like circles and rectangles through a factory interface, without the caller needing to understand the intricacies of each shape's creation.
This abstraction fosters a well-organized codebase that is easier to maintain and extend with new shapes, as it adheres to the Single Responsibility Principle, ensuring each method has a clear purpose. In another example, a document editor application can leverage the Factory Method to instantiate different document types—PDF, Word, and HTML—without coupling the client code to specific document classes. This approach not only encapsulates the creation process but also improves the maintainability of the code by allowing new document types to be added with minimal changes.
As Java has evolved over the past three decades, patterns like the Factory Method continue to be integral in writing clean, reliable, and scalable Java applications. To implement the Factory Method in Java, you would start by defining an abstract 'Product' interface, followed by concrete implementations for each object type. The factory itself is also defined, typically as an abstract class or interface, with concrete factories responsible for the actual object creation.
This design ensures a consistent and predictable structure, which is crucial for collaboration and comprehension within a development team. According to best practices, methods in Java should be clear in their responsibility, as they serve as the building blocks of your application. With the proper use of access specifiers and method signatures, Java methods can be a powerful tool for developers to create flexible and maintainable code.
Conclusion
In conclusion, understanding object creation in Java is crucial for developers as it forms the foundation of Java's object-oriented paradigm. By defining classes, using constructors, and utilizing the "new" keyword and factory methods, developers can instantiate objects and manipulate them within their code.
Adhering to Java conventions, such as clear naming and adhering to the Single Responsibility Principle, enhances code readability and maintainability. Staying up-to-date with Java's evolution is also important, as new features are continually introduced to improve the language's capabilities.
Constructors play a pivotal role in initializing objects and setting up their initial state. Overloading constructors allows for flexibility in different initialization scenarios.
The "new" keyword is essential for creating new objects and allocating memory for them. It is a foundational feature of Java that enables object instantiation.
Factory methods provide an abstraction layer that simplifies object creation by hiding the instantiation logic. This pattern improves code organization, maintainability, and extensibility. Mastering object creation in Java is indispensable for developers working on small-scale projects or large server boxes. It is a skill that reflects the adaptability and enduring relevance of Java as a programming language. Overall, by understanding the concepts of object creation in Java and following best practices, developers can write adaptable, efficient, and maintainable code that leverages the power of the language's features.
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.