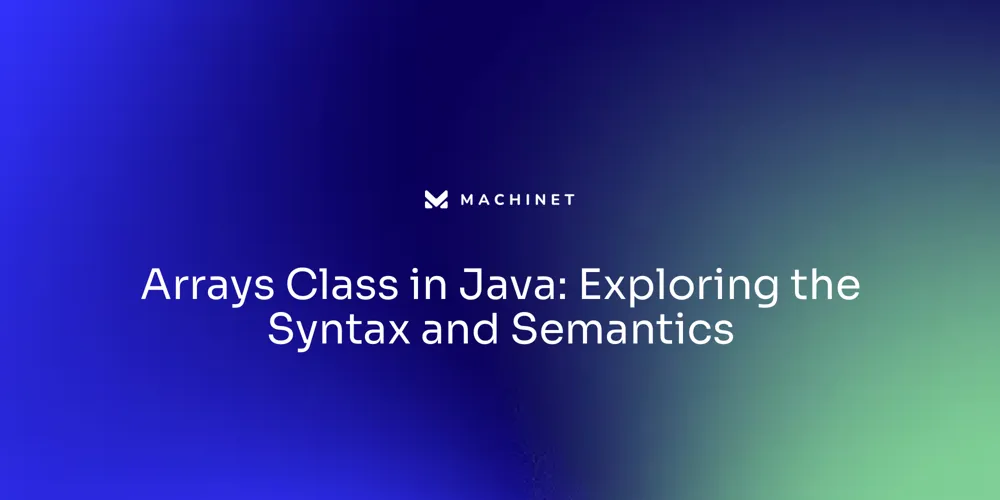
Table of Contents
- Declaring and Defining Arrays
- Accessing and Modifying Array Elements
- Array Length and Capacity
- Array Methods: Sorting, Searching, and Copying
- Using the Arrays Class for Advanced Operations
- Common Applications of Arrays in Java Programming
- Best Practices for Working with Arrays in Java
Introduction
Arrays are fundamental data structures in Java that allow for the storage and manipulation of collections of elements. Whether you're a beginner or an experienced Java developer, understanding arrays is essential for writing efficient and optimized code.
In this article, we will explore various aspects of arrays in Java, including declaring and defining arrays, accessing and modifying array elements, array length and capacity, array methods for sorting and searching, using the Arrays class for advanced operations, common applications of arrays in Java programming, and best practices for working with arrays. By the end of this article, you will have a comprehensive understanding of arrays in Java and how to effectively use them in your programming endeavors. So let's dive in and explore the world of Java arrays!
Declaring and Defining Arrays
In the realm of Java development, arrays are fundamental structures that store collections of elements of the same type. Arrays are zero-indexed, meaning the first element is located at index 0, which is a vital concept to grasp for efficient programming.
Here's how to declare an array in Java: use the type of the elements, the array name, and square brackets ([]). For example, an integer array named 'numbers' would be declared as:
java
int[] numbers;
To instantiate this array with a capacity for five elements, use the 'new' keyword followed by the type and the desired size:
java
numbers = new int[5];
Alternatively, combine declaration and instantiation in one succinct line:
java
int[] numbers = new int[5];
Understanding and utilizing arrays is a stepping stone to mastering Java's syntax and semantics, which are essential for writing coherent and optimized code.
As Eleftheria, an experienced business analyst and tech writer, suggests, conveying intricate programming concepts in an accessible manner is key to knowledge sharing in the tech community. This aligns with the Single Responsibility Principle, a software design tenet that advocates for methods to have one well-defined purpose, simplifying complexity and maintaining clarity. Furthermore, proper commenting is crucial for elucidating the logic behind code, serving as a navigational aid for current and future developers. As we delve deeper into Java's capabilities, remember that a solid understanding of its native features can empower you with performance, flexibility, and control, while frameworks can streamline and expedite development processes for more ambitious projects.
Accessing and Modifying Array Elements
In Java, arrays are a fundamental data structure that allows you to store multiple values of the same type under a single variable name. They are stored in contiguous memory locations, which means accessing and modifying elements is a swift operation. For instance, you can retrieve the first element of an array called 'numbers' with int firstElement = numbers[0];
.
This line of code uses the index 0
to access the first position in the array. If you want to update an element, Java makes it just as straightforward. To alter the second element in the 'numbers' array to the value 10, you would write numbers[1] = 10;
.
This statement assigns the new value to the element at index 1
. Arrays in Java are zero-indexed, so the first element is always at index 0
, and the last element is at an index that is one less than the size of the array. It's crucial to remember that when you're working with arrays, the indices must be valid.
An attempt to access or modify an element outside the bounds of the array will trigger an 'ArrayIndexOutOfBoundsException'. This adherence to boundaries is essential to prevent runtime errors and maintain the integrity of your data. Arrays, being pivotal in handling data, make managing and manipulating large datasets more organized and efficient, which is vital for solving complex programming challenges.
Array Length and Capacity
In Java, arrays are fundamental structures that store fixed-size sequential collections of elements of the same type. The size of an array, known as its length, is accessible via the 'length' property. To determine how many elements an array named 'numbers' contains, you would use the code int array Length = numbers.length;
.
It's crucial to understand that the length of an array is the count of elements it currently contains, not the total capacity it can hold. Array capacity is a concept that signifies the maximum number of elements an array can accommodate, dictated at the time of its creation. Unlike length, capacity is immutable; to adjust it, one must instantiate a new array with the required capacity and migrate the existing elements to it.
This distinction is vital in Java programming, as it aligns with the Single Responsibility Principle (SRP)βensuring that each component of your code, like an array, has one well-defined task. Properly managing array sizes and capacities contributes to clearer and more maintainable code. Moreover, understanding these concepts aids in writing comments that elucidate the structure and purpose of the code, thereby enhancing its readability and serving as a guide for other developers.
Array Methods: Sorting, Searching, and Copying
Java's Arrays class equips developers with an array of methods for effective array management. Among these, the 'sort()' method streamlines the ordering of array elements. By invoking Arrays.sort(numbers);
, one can effortlessly organize the 'numbers' array in ascending sequence.
Moreover, the 'binarySearch()' method enhances the efficiency of locating elements within sorted arrays. By using int index = Arrays.binarySearch(numbers, 10);
, developers can swiftly pinpoint the index of '10' in the 'numbers' array, or receive a negative outcome should the element be absent. Additionally, the 'copyOf()' method offers the convenience of duplicating arrays while customizing their size.
For instance, creating a truncated copy of the 'numbers' array, limited to three elements, is as simple as int[] newArray = Arrays.copyOf(numbers, 3);
. These methods not only simplify routine tasks but also embody the Single Responsibility Principle, ensuring each method addresses a unique aspect of array manipulation. As arrays are pivotal in managing sizeable datasets, these tools provided by the Java Arrays class are indispensable for fostering organized and efficient coding practices.
Using the Arrays Class for Advanced Operations
Java's Arrays class provides a suite of tools for handling collections of elements, known as arrays, which are vital in programming for organizing large datasets efficiently. These elements, stored in contiguous memory spaces, can be accessed and manipulated effectively, contributing to the ease of operations and computations.
One-dimensional or multi-dimensional arrays, defined by the number of indices needed to pinpoint an element, are essential for solving complex problems across different programming languages. For instance, the fill()
method in Java initializes all elements within an array with a single value, streamlining the process of setting up your data structure.
Here's how you can apply it:
java
Arrays.fill(numbers, 0);
When it comes to comparing arrays, the equals()
method evaluates if two arrays contain identical elements, which is crucial in ensuring data integrity. You can check the equality of 'array1' and 'array2' using:
java
boolean isEqual = Arrays.equals(array1, array2);
Moreover, representing an array as a string is simplified with the ToString()
method, which joins array elements in a formatted string, enclosed in brackets and separated by commas. This is particularly useful for displaying array contents in a readable format:
java
String arrayString = Arrays.toString(numbers);
These functionalities of the Arrays class not only enhance code efficiency but also underscore the significance of arrays in managing and representing information in programming.
Common Applications of Arrays in Java Programming
Arrays in Java serve as a foundational structure for storing and managing collections of data. They are essential for various operations, such as data analysis, which begins with the collection of relevant data from multiple sources.
Arrays allow for the efficient storage of such data, enabling quick access and manipulation. For example, an e-commerce company might use arrays to handle vast amounts of customer transaction data, streamlining the analysis process.
When it comes to implementing algorithms and data structures, arrays are particularly powerful. They are the backbone for building more complex structures like Binary Trees.
Take LeetCode's coding problems, which often involve array representations of trees. Arrays might be challenging for humans to interpret, but they offer a compact format that's optimal for computational processing.
Furthermore, arrays are not just for data storage; they are also instrumental in data cleaning and preprocessing, which is critical for ensuring the accuracy and integrity of data before analysis. For instance, arrays can be used to identify and correct errors or fill in missing values within datasets. Java developers frequently use arrays in conjunction with other languages and technologies such as JavaScript, SQL, Python, and HTML/CSS. Arrays provide the structure needed to organize and manage data across these platforms, proving their versatility and importance in software development. In essence, arrays encapsulate related data into a single unit, adhering to the Single Responsibility Principle by allowing methods to remain focused and manageable. Whether for sorting, searching, or iterating over elements, arrays are a fundamental tool that offers a structured approach to handling data, which is indispensable in programming.
Best Practices for Working with Arrays in Java
Adhering to best practices when working with Java arrays is crucial for writing efficient, understandable, and error-free code. Initializing arrays with default values is fundamental; for numeric types, this is typically 0, and for boolean types, false.
This simple step can prevent unpredictable behaviors during execution. For iteration, the enhanced 'for-each' loop is recommended.
It streamlines the process by handling indices behind the scenes, allowing you to focus on the logic of what you're doing with the elements rather than on the mechanics of accessing them. Being mindful of array bounds is vital to avoid the dreaded 'ArrayIndexOutOfBoundsException'.
Validating indices before using them is a practice that safeguards against runtime errors. While traditional C-style arrays have their place, the Java Collections Framework offers a more modern approach with structures like ArrayList and LinkedList, which provide additional functionality and flexibility. Performance considerations are especially important when dealing with large data sets or in performance-sensitive scenarios. In such cases, data structures like ArrayList or HashMap may offer more efficient alternatives to arrays, thanks to their optimized implementations and algorithmic advantages. By embracing these best practices, developers can ensure their array handling in Java is robust and efficient, leading to cleaner and more maintainable code.
Conclusion
To conclude, arrays are fundamental data structures in Java that allow for the storage and manipulation of collections of elements. They are zero-indexed and provide efficient access and modification of elements.
It is important to understand how to declare and define arrays, as well as their length and capacity. The Arrays class in Java provides useful methods for sorting, searching, and copying arrays, which simplify routine tasks and adhere to the Single Responsibility Principle.
Additionally, the Arrays class offers advanced operations such as filling arrays with a single value, comparing arrays for equality, and converting arrays to strings. Arrays have a wide range of applications in Java programming, including data storage and management, algorithm implementation, data cleaning and preprocessing, and integration with other languages and technologies.
They encapsulate related data into a single unit and promote structured approaches to handling data. When working with arrays in Java, it is important to follow best practices such as initializing arrays with default values, using the enhanced 'for-each' loop for iteration, validating array bounds to avoid runtime errors, considering alternative data structures from the Java Collections Framework for added functionality and flexibility, and optimizing performance for large datasets or performance-sensitive scenarios. By understanding the various aspects of arrays in Java and following best practices, developers can effectively use arrays in their programming endeavors. Arrays provide efficiency, organization, and control when working with collections of elements in Java.
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.