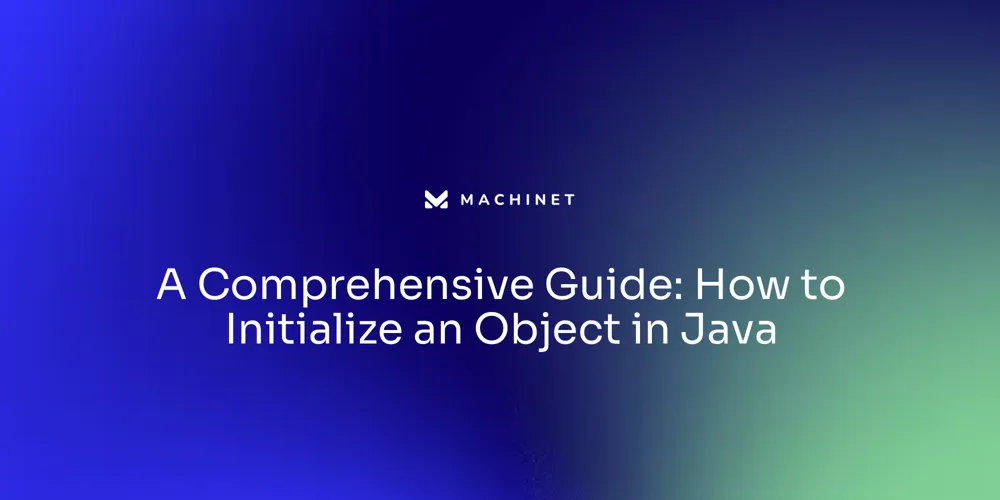
Table of Contents
- Using the new Keyword
- Using Class.forName() Method
- Using clone() Method
- Using Deserialization
Introduction
Java, one of the most popular programming languages, offers various methods and techniques for object initialization and manipulation. In this article, we will explore four different approaches: using the new keyword, the Class.forName() method, the clone() method, and deserialization.
Each method has its advantages and considerations, and understanding them is crucial for writing clean, maintainable Java code. So, let's dive in and discover the intricacies of object initialization in Java.
Using the new Keyword
In Java, object initialization is a fundamental concept that allows developers to create instances of classes. Initiation typically involves the new
keyword, which serves to allocate memory and set up the object.
Following this, the constructor of the class is invoked, which may require passing specific arguments if the constructor is parameterized. For instance:
java
ClassName objectName = new ClassName();
This line of code demonstrates how ClassName
represents the class being instantiated, while object
is the identifier for the new object.
This process not only allocates memory but also ensures that the instance variables are ready for use. Java's approach to object creation has evolved since its inception nearly three decades ago.
It has maintained core functionalities while also adapting new features, keeping the language both modern and flexible. The recent release of Java 20 introduces advancements such as virtual threads, which promise to revolutionize the handling of concurrent applications by simplifying their development and maintenance.
Embracing the principles of Native Java development, programmers have the flexibility to design applications that are tailored to specific needs, leading to better maintainability and control over their codebases. This adaptability is crucial in complex projects where bespoke solutions are required. The use of named constants, for example, exemplifies Java's emphasis on reducing errors and improving code clarity for collaboration. As Java continues to evolve, it remains a powerful tool for developers. Mark Reinhold, chief architect of the Java Platform Group at Oracle, has indicated that Java's adaptability and innovative features, such as checked exceptions, are key to its enduring popularity and relevance in the software development landscape.
Using Class.forName() Method
Java's dynamic class loading capabilities are exemplified by the Class.forName()
method, which allows for the runtime loading of classes. This method shines when you need to create instances of classes without knowing their type at compile time. Consider this snippet of code:
java
ClassName objectName = (ClassName) Class.forName("ClassName").newInstance();
Here, ClassName
represents the class you intend to instantiate.
First, Class.forName()
locates and loads the class, then newInstance()
is called to create an instance of the loaded class. Finally, the new object is cast to its proper type, ensuring that you can work with it as expected. The power of this approach is accentuated when dealing with complex data structures, as it aligns with Java's recent enhancements in pattern matching, which aims to bind values to variables within a pattern, thereby increasing code readability and intuitiveness.
For instance, pattern matching can simplify the extraction of data and execution of operations on them, a feature that continues to evolve with Java's ongoing development. Recent updates in the Java ecosystem, such as the release of JobRunr 6.3.4 and Spring Shell's new versions, reflect the continuous advancements and the dedication of the Java community to keep the language robust. These updates often include improvements in compatibility and performance, underlining the importance of staying current with Java's development to leverage its full potential.
Moreover, understanding how dependencies are resolved at runtime versus compile-time is crucial. As demonstrated by issues encountered when overriding library versions, such as with the Jackson-core
library, developers must be aware of how class dependencies impact the execution of their applications. This knowledge is essential to avoid runtime errors like java.lang.NoClassDefFoundError
, ensuring smooth operation and stability of Java applications.
Using clone() Method
Java's clone()
method offers a means to replicate an existing object, but it's not as straightforward as it may seem. To utilize clone()
, the class must implement the Cloneable
interface, signaling that it is legally allowable to clone instances of the class. Here's how you can use it in your code:
java
ClassName clonedObject = (ClassName) originalObject.clone();
In this snippet, ClassName
represents your class that implements Cloneable
, and originalObject
is the instance you wish to duplicate.
The cast to ClassName
is necessary because clone()
returns a reference of type Object
. This technique, while useful, has its intricacies. For example, the clone()
method performs a shallow copy, not a deep copy, meaning that any object references inside the cloned object will still point to the original objects.
Developers should be wary of this behavior to avoid unintended side effects. Furthermore, with the advent of new Java features, such as the Vector API previewed in JDK 23, developers are equipped with more robust tools for performance optimization. The Vector API, for instance, allows for expressing complex vector computations that are optimized at runtime, showcasing Java's continuous evolution and its commitment to performance.
The Java ecosystem is also enriched by frameworks like Hibernate, which uses Java Persistence API (JPA) annotations to map database entries to objects, simplifying data manipulation. As seen in practical applications like the Conway's Game of Life, the flexibility of Java enables developers to build applications with ease and efficiency, even when dealing with version-controlled databases like Dolt. However, it's essential to manage code clones effectively, as they constitute a significant portion of codebasesβon average, 18.5%βand can complicate maintenance and evolution of software projects.
Using Deserialization
Java's deserialization mechanism allows for the reconstruction of an object's state from its serialized form, which is essential in scenarios where objects need to be transmitted or stored. For instance, within an event-driven architecture like Kafka, a producer serializes Java objects into JSON messages for a messaging topic, and a consumer deserializes them for further processing. Here's a simple illustration of deserialization in Java:
java
ObjectInputStream inputStream = new ObjectInputStream(new FileInputStream("filename.txt"));
ClassName objectName = (ClassName) inputStream.readObject();
In this snippet, ClassName
represents the class of the object we are reconstructing.
We initiate an ObjectInputStream
linked to a file, "filename.txt", which contains the serialized object data. The readObject()
method then reads and deserializes the object, recreating it in memory. Recent developments in the Java ecosystem, such as the updates in Spring Integration and Spring Vault, emphasize the ongoing improvements in Java's robust libraries.
These advancements not only enhance performance but also provide a deeper level of control and flexibility for developers. With Java's enduring popularity and its third-place ranking in job market demand, understanding deserialization and serialization processes is more relevant than ever. It's not just about writing code that functions; it's about crafting clean, maintainable code that stands the test of time and aligns with the principles of clean Java code, such as the Single Responsibility Principle.
Conclusion
In conclusion, object initialization in Java can be achieved through various methods, each with its own advantages and considerations. The use of the new
keyword is a fundamental approach that allocates memory and sets up the object by invoking the class constructor.
Java's dynamic class loading capabilities are exemplified by the Class.forName()
method, which allows for runtime loading of classes without knowing their type at compile time. The clone()
method provides a means to replicate an existing object but requires implementing the Cloneable
interface and performs a shallow copy.
Lastly, Java's deserialization mechanism enables the reconstruction of an object's state from its serialized form, making it essential for scenarios involving object transmission or storage. Understanding these different approaches to object initialization is crucial for writing clean and maintainable Java code.
It allows developers to leverage Java's evolving features, such as pattern matching and performance optimization tools like the Vector API. Additionally, staying current with updates in the Java ecosystem ensures compatibility, performance improvements, and stability in application execution. Overall, Java remains a powerful tool for developers due to its adaptability, innovative features, and robust libraries. By mastering object initialization techniques in Java, developers can craft code that stands the test of time while adhering to clean coding principles.
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.