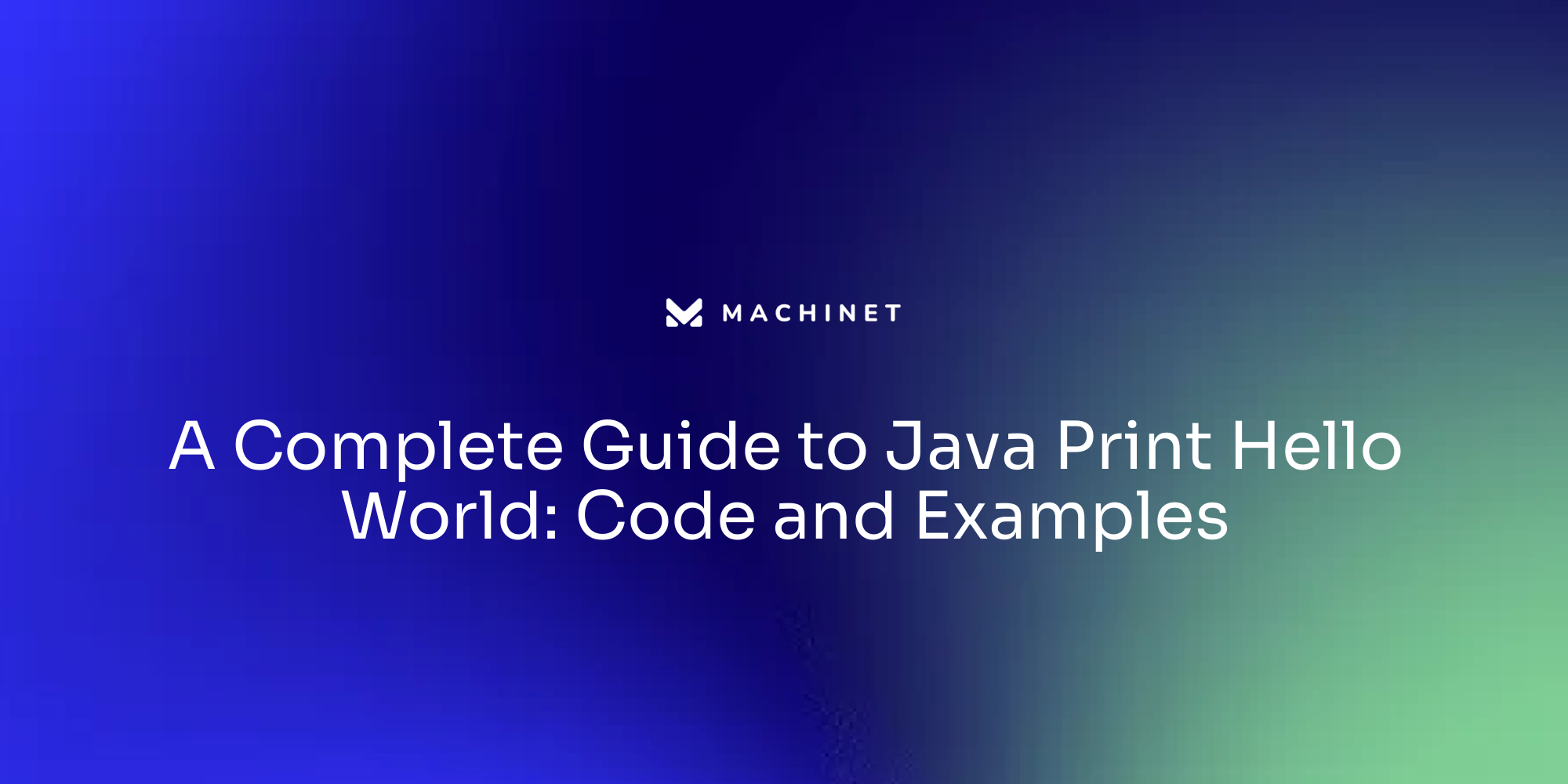
Introduction
Introducing the world of Java programming begins with the iconic "Hello, World!" program, a rite of passage for aspiring developers. This simple yet powerful example not only demonstrates the basic syntax of Java but also lays the groundwork for understanding its object-oriented principles.
The program consists of essential components: a class definition, the main method, and a print statement, each playing a crucial role in execution. By exploring these foundational elements, developers can familiarize themselves with Java's structure and functionality. From defining classes to handling output, this article delves into the intricacies of creating and running a Java Hello World program, providing insights that will pave the way for more complex coding endeavors.
Components of a Java Hello World Program
A Hello World program in the programming language is often the first step for many developers learning it. It usually includes several key elements: a type definition, the main method, and a print statement.
- Class Definition: In Java, every piece of code exists within a structure. The group functions as a blueprint for creating objects and encapsulates data and behavior. The syntax for defining a class is straightforward:
java
public class HelloWorld {
}
- Main Method: The
main()
method is crucial as it serves as the entry point for program execution. When the Virtual Machine (JVM) runs a programming application, it looks for this method to start the execution process. The typical structure looks like this:
java
public static void main(String[] args) {
// code here
}
- Print Statement: Finally, to display output, a print statement is used. In the programming language, this is accomplished using
System.out.println()
. The complete Hello World program would look like this:
java
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
This simple program demonstrates basic Java syntax and allows developers to ensure their development environment is correctly set up. Comprehending these components is essential for grasping the broader structure of programming applications, which are built upon object-oriented principles. For instance, the syntax of this programming language is derived from C and C++, making it accessible for developers familiar with those languages, while promoting a clean and organized coding style.
Understanding Java Class Definition
In Java, every application is organized around types, which serve as blueprints for creating objects. A blueprint encapsulates related methods and properties, forming the foundation of object-oriented programming. To declare a type, the keyword class
is utilized, followed by the type name, which conventionally starts with an uppercase letter. For instance, a simple class declaration would look like this:
java
public class HelloWorld {
// Class body goes here
}
This structure not only organizes code but also enhances its readability and maintainability—key principles in modern coding practices. As Brian Goetz, a Language Architect at Oracle, emphasizes, "A class is a template or blueprint that defines the properties (attributes) and behaviors (methods) that objects of that class will have." This encapsulation allows developers to create modular and reusable code, which is crucial for efficient software development.
The Main Method in Java
The main
method serves as the crucial entry point for any programming application, defined by the signature public static void main(String[] args)
. When the Java Virtual Machine (JVM) runs an application, it starts its journey in this method, illustrating its foundational role in the ecosystem of this programming language. The String[] args
parameter is particularly noteworthy, as it allows the program to accept command-line arguments, enhancing the flexibility and adaptability of the application. This means that users can supply various inputs when launching the application, enabling a dynamic interaction that can cater to different needs and scenarios.
The programming language, launched in 1995 by Sun Microsystems and now owned by Oracle Corporation, is renowned for its versatility and platform independence. The mantra "write once, run anywhere" encapsulates the power of the language, as code can execute on any device or platform equipped with a JVM. This characteristic has solidified the language's position as one of the most popular programming languages globally, utilized across diverse fields ranging from web development to enterprise applications.
Printing to the Console with System.out.println()
In the programming language, showing results to the console is achieved through the System.out.println()
method. 'This function is essential for communicating information during the execution of a task, making it a foundational tool for debugging and user interaction.'. When invoked, it prints the specified message followed by a newline, ensuring that each output appears on a new line for clarity.
For instance, the command System.out.println("Hello, World!");
results in the output "Hello, World!" appearing in the console. This method not only facilitates the display of strings but can also handle various data types, making it versatile for different programming scenarios.
Grasping how to use System.out.println()
effectively is essential for any software developer, as it helps in tracking the flow of the application and confirming output during the development phase.
Creating and Running a Java Hello World Program
Creating a Java Hello World program involves a few straightforward steps that introduce you to the basics of Java programming. Start by selecting a text editor or an Integrated Development Environment (IDE) to write your program. Here’s a concise breakdown of the essential steps:
- Class Specification: Begin by outlining a structure. In Java, all code must be contained within a structure. You can name your class
HelloWorld
as follows:
java
public class HelloWorld {
}
- Main Method: Inside your class, add the
main
method. This method acts as the entry point for any programming application. It should look like this:
java
public static void main(String[] args) {
}
- Print Statement: Within the
main
method, insert a print statement to output the message. For instance:
java
System.out.println("Hello, World!");
}
-
File Saving: Save your file with a
.java
extension, for example,HelloWorld.java
. This step is essential as it informs the compiler that the file includes programming instructions. -
Compilation: To convert your programming code into bytecode, you need to compile it. Use the following command in your terminal or command prompt:
bash
javac HelloWorld.java
- Execution: After successful compilation, run your program with the command:
bash
java HelloWorld
Upon running, you should see the output:
Hello, World!
This simple program not only serves as a foundational exercise in Java but also illustrates the fundamental structure of Java applications, which is crucial for understanding more complex concepts in Object-Oriented Programming (OOP). As you explore further into the programming language, you'll discover that OOP principles boost the modularity and reusability of your code, facilitating management and scalability.
Example Code for Java Hello World Program
A Java Hello World program serves as the simplest introduction to Java programming. Below is a basic example:
java
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
When this code is compiled and executed, it outputs "Hello, World!" in the console. This software demonstrates the fundamental framework of an application created with Java. The public class HelloWorld
declaration defines a class named HelloWorld
, which is the entry point of the program. The main
method, marked as public static void
, is where the programming runtime begins execution. Within this method, the System.out.println
statement is utilized to display text to the console, demonstrating how simple the syntax can be for newcomers.
The versatility of this programming language is a key feature that contributes to its popularity. Created by Sun Microsystems and currently overseen by Oracle Corporation, this programming language is renowned for its platform independence—developers can write code once and execute it anywhere that has a compatible Virtual Machine (JVM). This characteristic, along with the strong security features of the programming language, has made it a preferred choice among developers worldwide.
According to a recent report, the programming language remains one of the most widely-used, with over 77% of developers actively utilizing AI tools like ChatGPT and GitHub Copilot to enhance their coding efficiency. This reflects a broader trend where tools are increasingly integrated into the software development life cycle, empowering developers to innovate and optimize their programming more effectively.
Tips for Writing and Compiling Java Code
When writing Java programs, adhering to best practices is crucial for creating maintainable and understandable applications. Utilize meaningful class and method names that convey their purpose clearly, as this promotes readability and facilitates easier debugging and future modifications. Proper indentation is equally important; it enhances the visual structure of your work, making it easier for yourself and others to follow the logic and flow.
Adding comments to your work is not merely a courtesy; it acts as documentation that clarifies the functionality of complex logic, ensuring that anyone reviewing it can understand its intent swiftly. As the saying goes, the main goal of programming is to be comprehended and preserved, not to display technical skill. This focus on clarity helps reduce frustration and promotes a healthier development environment.
To compile your programming instructions, ensure that the Development Kit (JDK) is properly installed and configured on your system. This includes setting environment variables and ensuring that your IDE or command line is correctly pointed to the JDK. After writing your code, always check the console for any syntax errors during compilation. Missing a simple semicolon or typo can lead to frustrating debugging sessions. As a best practice, strive to catch these errors early to maintain a smooth development workflow.
Common Issues and Solutions in Java Hello World Programs
When working with a Hello World program, several common issues may arise that can hinder execution. Here are some key problems and their solutions:
-
Class Not Found: This error typically occurs when the class name does not precisely match the file name, including its case. The programming language is case-sensitive, so ensure that the name of your public class aligns perfectly with the filename.
-
Syntax Errors: Syntax errors are often caused by overlooked mistakes such as missing semicolons at the end of statements or mismatched braces. These errors can prevent the program from compiling, so it’s essential to carefully review your code for such discrepancies.
-
Programming Language Not Recognized: If you encounter this issue, it indicates that the programming environment might not be set up correctly. Verify that the programming language is installed on your system and check that the PATH environment variable is configured to include the binaries. This setup is crucial for your operating system to recognize Java commands.
Conclusion
The exploration of the Java Hello World program serves as an essential introduction to the fundamentals of Java programming. By dissecting its core components—class definition, main method, and print statement—developers gain a clear understanding of how Java applications are structured. The simplicity of the Hello World program not only demonstrates basic syntax but also reinforces the importance of object-oriented principles that underpin the language.
Understanding the significance of each component is crucial for effective programming. The class serves as a blueprint for creating objects, while the main method acts as the entry point for execution. The use of System.out.println()
for output is a foundational skill that facilitates communication within the program, aiding in debugging and user interaction.
Mastering these elements lays the groundwork for tackling more complex programming tasks.
As developers progress in their Java journey, adhering to best practices in coding, such as meaningful naming conventions and proper indentation, becomes increasingly important. Recognizing common issues and knowing how to resolve them is key to maintaining a smooth development experience. Ultimately, the knowledge gained from creating and running a simple Hello World program equips developers with the confidence to delve deeper into the vast capabilities of Java programming.
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.